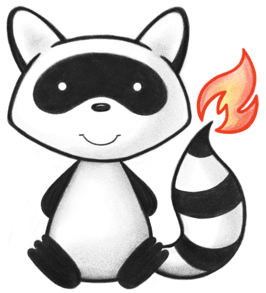
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_50; 004import org.hl7.fhir.convertors.context.ConversionContext10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Signature10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Decimal10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Instant10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.UnsignedInt10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Bundle.LinkRelationTypes; 013import org.hl7.fhir.utilities.FhirPublication; 014 015public class Bundle10_50 { 016 017 public static org.hl7.fhir.r5.model.Bundle convertBundle(org.hl7.fhir.dstu2.model.Bundle src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.r5.model.Bundle tgt = new org.hl7.fhir.r5.model.Bundle(); 021 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyResource(src, tgt); 022 if (src.hasType()) 023 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 024 if (src.hasTotal()) 025 tgt.setTotalElement(UnsignedInt10_50.convertUnsignedInt(src.getTotalElement())); 026 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 027 tgt.addLink(convertBundleLinkComponent(t)); 028 for (org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent t : src.getEntry()) 029 tgt.addEntry(convertBundleEntryComponent(t)); 030 if (src.hasSignature()) 031 tgt.setSignature(Signature10_50.convertSignature(src.getSignature())); 032 return tgt; 033 } 034 035 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.r5.model.Bundle src) throws FHIRException { 036 return convertBundle(src, null); 037 } 038 039 public static org.hl7.fhir.dstu2.model.Bundle convertBundle(org.hl7.fhir.r5.model.Bundle src, BaseAdvisor_10_50 advisor) throws FHIRException { 040 if (src == null || src.isEmpty()) 041 return null; 042 org.hl7.fhir.dstu2.model.Bundle tgt = new org.hl7.fhir.dstu2.model.Bundle(); 043 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyResource(src, tgt); 044 if (src.hasType()) 045 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 046 if (src.hasTotal()) 047 tgt.setTotalElement(UnsignedInt10_50.convertUnsignedInt(src.getTotalElement())); 048 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 049 for (org.hl7.fhir.r5.model.Bundle.BundleEntryComponent t : src.getEntry()) 050 tgt.addEntry(convertBundleEntryComponent(t, advisor)); 051 if (src.hasSignature()) 052 tgt.setSignature(Signature10_50.convertSignature(src.getSignature())); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.r5.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.r5.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryComponent(); 060 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 061 for (org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent t : src.getLink()) 062 tgt.addLink(convertBundleLinkComponent(t)); 063 if (src.hasFullUrlElement()) 064 tgt.setFullUrlElement(Uri10_50.convertUri(src.getFullUrlElement())); 065 if (src.hasResource()) 066 tgt.setResource(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertResource(src.getResource())); 067 if (src.hasSearch()) 068 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 069 if (src.hasRequest()) 070 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 071 if (src.hasResponse()) 072 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 073 return tgt; 074 } 075 076 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryComponent src, BaseAdvisor_10_50 advisor) throws FHIRException { 077 if (src == null || src.isEmpty()) 078 return null; 079 if (advisor.ignoreEntry(src, FhirPublication.DSTU2)) 080 return null; 081 org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent(); 082 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 083 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 084 if (src.hasFullUrlElement()) 085 tgt.setFullUrlElement(Uri10_50.convertUri(src.getFullUrlElement())); 086 if (src.hasResource()) 087 tgt.setResource(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertResource(src.getResource())); 088 if (src.hasSearch()) 089 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 090 if (src.hasRequest()) 091 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 092 if (src.hasResponse()) 093 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 094 return tgt; 095 } 096 097 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryComponent src) throws FHIRException { 098 return convertBundleEntryComponent(src, null); 099 } 100 101 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 102 if (src == null || src.isEmpty()) 103 return null; 104 org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent(); 105 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 106 if (src.hasMethod()) 107 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 108 if (src.hasUrlElement()) 109 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 110 if (src.hasIfNoneMatchElement()) 111 tgt.setIfNoneMatchElement(String10_50.convertString(src.getIfNoneMatchElement())); 112 if (src.hasIfModifiedSinceElement()) 113 tgt.setIfModifiedSinceElement(Instant10_50.convertInstant(src.getIfModifiedSinceElement())); 114 if (src.hasIfMatchElement()) 115 tgt.setIfMatchElement(String10_50.convertString(src.getIfMatchElement())); 116 if (src.hasIfNoneExistElement()) 117 tgt.setIfNoneExistElement(String10_50.convertString(src.getIfNoneExistElement())); 118 return tgt; 119 } 120 121 public static org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 122 if (src == null || src.isEmpty()) 123 return null; 124 org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent(); 125 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 126 if (src.hasMethod()) 127 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 128 if (src.hasUrlElement()) 129 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 130 if (src.hasIfNoneMatchElement()) 131 tgt.setIfNoneMatchElement(String10_50.convertString(src.getIfNoneMatchElement())); 132 if (src.hasIfModifiedSinceElement()) 133 tgt.setIfModifiedSinceElement(Instant10_50.convertInstant(src.getIfModifiedSinceElement())); 134 if (src.hasIfMatchElement()) 135 tgt.setIfMatchElement(String10_50.convertString(src.getIfMatchElement())); 136 if (src.hasIfNoneExistElement()) 137 tgt.setIfNoneExistElement(String10_50.convertString(src.getIfNoneExistElement())); 138 return tgt; 139 } 140 141 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 142 if (src == null || src.isEmpty()) 143 return null; 144 org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent(); 145 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 146 if (src.hasStatusElement()) 147 tgt.setStatusElement(String10_50.convertString(src.getStatusElement())); 148 if (src.hasLocationElement()) 149 tgt.setLocationElement(Uri10_50.convertUri(src.getLocationElement())); 150 if (src.hasEtagElement()) 151 tgt.setEtagElement(String10_50.convertString(src.getEtagElement())); 152 if (src.hasLastModifiedElement()) 153 tgt.setLastModifiedElement(Instant10_50.convertInstant(src.getLastModifiedElement())); 154 return tgt; 155 } 156 157 public static org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 158 if (src == null || src.isEmpty()) 159 return null; 160 org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent(); 161 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 162 if (src.hasStatusElement()) 163 tgt.setStatusElement(String10_50.convertString(src.getStatusElement())); 164 if (src.hasLocationElement()) 165 tgt.setLocationElement(Uri10_50.convertUri(src.getLocationElement())); 166 if (src.hasEtagElement()) 167 tgt.setEtagElement(String10_50.convertString(src.getEtagElement())); 168 if (src.hasLastModifiedElement()) 169 tgt.setLastModifiedElement(Instant10_50.convertInstant(src.getLastModifiedElement())); 170 return tgt; 171 } 172 173 public static org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 174 if (src == null || src.isEmpty()) 175 return null; 176 org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent(); 177 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 178 if (src.hasMode()) 179 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 180 if (src.hasScoreElement()) 181 tgt.setScoreElement(Decimal10_50.convertDecimal(src.getScoreElement())); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 186 if (src == null || src.isEmpty()) 187 return null; 188 org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent(); 189 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 190 if (src.hasMode()) 191 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 192 if (src.hasScoreElement()) 193 tgt.setScoreElement(Decimal10_50.convertDecimal(src.getScoreElement())); 194 return tgt; 195 } 196 197 public static org.hl7.fhir.r5.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r5.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleLinkComponent(); 201 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 202 if (src.hasRelationElement()) 203 tgt.setRelation(LinkRelationTypes.fromCode(src.getRelation())); 204 if (src.hasUrlElement()) 205 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r5.model.Bundle.BundleLinkComponent src) throws FHIRException { 210 if (src == null || src.isEmpty()) 211 return null; 212 org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent(); 213 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 214 if (src.hasRelationElement()) 215 tgt.setRelation((src.getRelation().toCode())); 216 if (src.hasUrlElement()) 217 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 218 return tgt; 219 } 220 221 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> src) throws FHIRException { 222 if (src == null || src.isEmpty()) 223 return null; 224 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.BundleTypeEnumFactory()); 225 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 226 switch (src.getValue()) { 227 case DOCUMENT: 228 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.DOCUMENT); 229 break; 230 case MESSAGE: 231 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.MESSAGE); 232 break; 233 case TRANSACTION: 234 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTION); 235 break; 236 case TRANSACTIONRESPONSE: 237 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.TRANSACTIONRESPONSE); 238 break; 239 case BATCH: 240 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCH); 241 break; 242 case BATCHRESPONSE: 243 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.BATCHRESPONSE); 244 break; 245 case HISTORY: 246 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.HISTORY); 247 break; 248 case SEARCHSET: 249 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.SEARCHSET); 250 break; 251 case COLLECTION: 252 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.COLLECTION); 253 break; 254 default: 255 tgt.setValue(org.hl7.fhir.r5.model.Bundle.BundleType.NULL); 256 break; 257 } 258 return tgt; 259 } 260 261 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> src) throws FHIRException { 262 if (src == null || src.isEmpty()) 263 return null; 264 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.BundleTypeEnumFactory()); 265 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 266 switch (src.getValue()) { 267 case DOCUMENT: 268 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.DOCUMENT); 269 break; 270 case MESSAGE: 271 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.MESSAGE); 272 break; 273 case TRANSACTION: 274 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.TRANSACTION); 275 break; 276 case TRANSACTIONRESPONSE: 277 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.TRANSACTIONRESPONSE); 278 break; 279 case BATCH: 280 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.BATCH); 281 break; 282 case BATCHRESPONSE: 283 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.BATCHRESPONSE); 284 break; 285 case HISTORY: 286 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.HISTORY); 287 break; 288 case SEARCHSET: 289 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.SEARCHSET); 290 break; 291 case COLLECTION: 292 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.COLLECTION); 293 break; 294 default: 295 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.BundleType.NULL); 296 break; 297 } 298 return tgt; 299 } 300 301 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> src) throws FHIRException { 302 if (src == null || src.isEmpty()) 303 return null; 304 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.HTTPVerbEnumFactory()); 305 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 306 switch (src.getValue()) { 307 case GET: 308 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.GET); 309 break; 310 case POST: 311 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.POST); 312 break; 313 case PUT: 314 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.PUT); 315 break; 316 case DELETE: 317 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.DELETE); 318 break; 319 default: 320 tgt.setValue(org.hl7.fhir.r5.model.Bundle.HTTPVerb.NULL); 321 break; 322 } 323 return tgt; 324 } 325 326 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> src) throws FHIRException { 327 if (src == null || src.isEmpty()) 328 return null; 329 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.HTTPVerbEnumFactory()); 330 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 331 switch (src.getValue()) { 332 case GET: 333 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.GET); 334 break; 335 case POST: 336 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.POST); 337 break; 338 case PUT: 339 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.PUT); 340 break; 341 case DELETE: 342 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.DELETE); 343 break; 344 default: 345 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.HTTPVerb.NULL); 346 break; 347 } 348 return tgt; 349 } 350 351 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> src) throws FHIRException { 352 if (src == null || src.isEmpty()) 353 return null; 354 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Bundle.SearchEntryModeEnumFactory()); 355 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 356 switch (src.getValue()) { 357 case MATCH: 358 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.MATCH); 359 break; 360 case INCLUDE: 361 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.INCLUDE); 362 break; 363 case OUTCOME: 364 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.OUTCOME); 365 break; 366 default: 367 tgt.setValue(org.hl7.fhir.r5.model.Bundle.SearchEntryMode.NULL); 368 break; 369 } 370 return tgt; 371 } 372 373 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> src) throws FHIRException { 374 if (src == null || src.isEmpty()) 375 return null; 376 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Bundle.SearchEntryModeEnumFactory()); 377 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 378 switch (src.getValue()) { 379 case MATCH: 380 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.MATCH); 381 break; 382 case INCLUDE: 383 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.INCLUDE); 384 break; 385 case OUTCOME: 386 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.OUTCOME); 387 break; 388 default: 389 tgt.setValue(org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode.NULL); 390 break; 391 } 392 return tgt; 393 } 394}