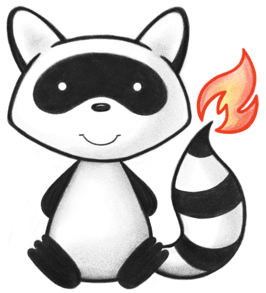
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Annotation10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Period10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 010import org.hl7.fhir.dstu2.model.Reference; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.CodeableReference; 013 014public class CarePlan10_50 { 015 016 public static org.hl7.fhir.r5.model.CarePlan convertCarePlan(org.hl7.fhir.dstu2.model.CarePlan src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.r5.model.CarePlan tgt = new org.hl7.fhir.r5.model.CarePlan(); 020 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 023 if (src.hasSubject()) 024 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 025 if (src.hasStatus()) 026 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 027 if (src.hasContext()) 028 tgt.setEncounter(Reference10_50.convertReference(src.getContext())); 029 if (src.hasPeriod()) 030 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 031 for (org.hl7.fhir.dstu2.model.Reference t : src.getAuthor()) 032 if (!tgt.hasCustodian()) 033 tgt.setCustodian(Reference10_50.convertReference(t)); 034 else 035 tgt.addContributor(Reference10_50.convertReference(t)); 036 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCategory()) 037 tgt.addCategory(CodeableConcept10_50.convertCodeableConcept(t)); 038 if (src.hasDescriptionElement()) 039 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 040 for (org.hl7.fhir.dstu2.model.Reference t : src.getAddresses()) 041 tgt.addAddresses(convertReferenceToCodableReference(t)); 042 for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_50.convertReference(t)); 043 for (org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 044 tgt.addActivity(convertCarePlanActivityComponent(t)); 045 return tgt; 046 } 047 048 public static org.hl7.fhir.dstu2.model.CarePlan convertCarePlan(org.hl7.fhir.r5.model.CarePlan src) throws FHIRException { 049 if (src == null || src.isEmpty()) 050 return null; 051 org.hl7.fhir.dstu2.model.CarePlan tgt = new org.hl7.fhir.dstu2.model.CarePlan(); 052 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 055 if (src.hasSubject()) 056 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 057 if (src.hasStatus()) 058 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 059 if (src.hasEncounter()) 060 tgt.setContext(Reference10_50.convertReference(src.getEncounter())); 061 if (src.hasPeriod()) 062 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 063 if (src.hasCustodian()) 064 tgt.addAuthor(Reference10_50.convertReference(src.getCustodian())); 065 for (org.hl7.fhir.r5.model.Reference t : src.getContributor()) tgt.addAuthor(Reference10_50.convertReference(t)); 066 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 067 tgt.addCategory(CodeableConcept10_50.convertCodeableConcept(t)); 068 if (src.hasDescriptionElement()) 069 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 070 for (CodeableReference t : src.getAddresses()) { 071 if (t.hasReference()) { 072 tgt.addAddresses(Reference10_50.convertReference(t.getReference())); 073 } 074 } 075 for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_50.convertReference(t)); 076 for (org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 077 tgt.addActivity(convertCarePlanActivityComponent(t)); 078 return tgt; 079 } 080 081 public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 082 if (src == null || src.isEmpty()) 083 return null; 084 org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent(); 085 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 086 for (org.hl7.fhir.r5.model.Annotation t : src.getProgress()) tgt.addProgress(Annotation10_50.convertAnnotation(t)); 087 if (src.hasPlannedActivityReference()) 088 tgt.setReference(Reference10_50.convertReference(src.getPlannedActivityReference())); 089// if (src.hasPlannedActivityDetail()) 090// tgt.setDetail(convertCarePlanActivityDetailComponent(src.getPlannedActivityDetail())); 091 return tgt; 092 } 093 094 public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 095 if (src == null || src.isEmpty()) 096 return null; 097 org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent(); 098 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 099 for (org.hl7.fhir.dstu2.model.Annotation t : src.getProgress()) 100 tgt.addProgress(Annotation10_50.convertAnnotation(t)); 101 if (src.hasReference()) 102 tgt.setPlannedActivityReference(Reference10_50.convertReference(src.getReference())); 103// if (src.hasDetail()) 104// tgt.setPlannedActivityDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 105 return tgt; 106 } 107 108// public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 109// if (src == null || src.isEmpty()) 110// return null; 111// org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent(); 112// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 113// if (src.hasCode()) 114// tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 115// for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReasonCode()) 116// tgt.addReason(CodeableConcept10_50.convertCodeableConceptToCodableReference(t)); 117// for (org.hl7.fhir.dstu2.model.Reference t : src.getReasonReference()) 118// tgt.addReason(convertReferenceToCodableReference(t)); 119// for (org.hl7.fhir.dstu2.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_50.convertReference(t)); 120// if (src.hasStatus()) 121// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 122// if (src.hasProhibitedElement()) 123// tgt.setDoNotPerformElement(Boolean10_50.convertBoolean(src.getProhibitedElement())); 124// if (src.hasScheduled()) 125// tgt.setScheduled(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getScheduled())); 126// if (src.hasLocation()) 127// tgt.getLocation().setReference(Reference10_50.convertReference(src.getLocation())); 128// for (org.hl7.fhir.dstu2.model.Reference t : src.getPerformer()) 129// tgt.addPerformer(Reference10_50.convertReference(t)); 130// if (src.hasProduct()) 131// tgt.setProduct(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getProduct())); 132// if (src.hasDailyAmount()) 133// tgt.setDailyAmount(SimpleQuantity10_50.convertSimpleQuantity(src.getDailyAmount())); 134// if (src.hasQuantity()) 135// tgt.setQuantity(SimpleQuantity10_50.convertSimpleQuantity(src.getQuantity())); 136// if (src.hasDescriptionElement()) 137// tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 138// return tgt; 139// } 140// 141// public static org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent src) throws FHIRException { 142// if (src == null || src.isEmpty()) 143// return null; 144// org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityDetailComponent(); 145// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 146// if (src.hasCode()) 147// tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 148// for (CodeableReference t : src.getReason()) 149// if (t.hasConcept()) 150// tgt.addReasonCode(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 151// for (CodeableReference t : src.getReason()) 152// if (t.hasReference()) 153// tgt.addReasonReference(Reference10_50.convertReference(t.getReference())); 154// for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) tgt.addGoal(Reference10_50.convertReference(t)); 155// if (src.hasStatus()) 156// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 157// if (src.hasDoNotPerformElement()) 158// tgt.setProhibitedElement(Boolean10_50.convertBoolean(src.getDoNotPerformElement())); 159// if (src.hasScheduled()) 160// tgt.setScheduled(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getScheduled())); 161// if (src.getLocation().hasReference()) 162// tgt.setLocation(Reference10_50.convertReference(src.getLocation().getReference())); 163// for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference10_50.convertReference(t)); 164// if (src.hasProduct()) 165// tgt.setProduct(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getProduct())); 166// if (src.hasDailyAmount()) 167// tgt.setDailyAmount(SimpleQuantity10_50.convertSimpleQuantity(src.getDailyAmount())); 168// if (src.hasQuantity()) 169// tgt.setQuantity(SimpleQuantity10_50.convertSimpleQuantity(src.getQuantity())); 170// if (src.hasDescriptionElement()) 171// tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 172// return tgt; 173// } 174// 175// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 176// if (src == null || src.isEmpty()) 177// return null; 178// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatusEnumFactory()); 179// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 180// switch (src.getValue()) { 181// case NOTSTARTED: 182// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 183// break; 184// case SCHEDULED: 185// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 186// break; 187// case INPROGRESS: 188// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 189// break; 190// case ONHOLD: 191// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.ONHOLD); 192// break; 193// case COMPLETED: 194// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.COMPLETED); 195// break; 196// case CANCELLED: 197// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.CANCELLED); 198// break; 199// default: 200// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NULL); 201// break; 202// } 203// return tgt; 204// } 205// 206// static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 207// if (src == null || src.isEmpty()) 208// return null; 209// org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatusEnumFactory()); 210// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 211// switch (src.getValue()) { 212// case NOTSTARTED: 213// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 214// break; 215// case SCHEDULED: 216// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 217// break; 218// case INPROGRESS: 219// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 220// break; 221// case ONHOLD: 222// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.ONHOLD); 223// break; 224// case COMPLETED: 225// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.COMPLETED); 226// break; 227// case CANCELLED: 228// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.CANCELLED); 229// break; 230// default: 231// tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanActivityStatus.NULL); 232// break; 233// } 234// return tgt; 235// } 236 237 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> convertCarePlanStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatusEnumFactory()); 241 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 242 switch (src.getValue()) { 243 case DRAFT: 244 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.DRAFT); 245 break; 246 case ACTIVE: 247 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.ACTIVE); 248 break; 249 case COMPLETED: 250 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.COMPLETED); 251 break; 252 case REVOKED: 253 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.CANCELLED); 254 break; 255 default: 256 tgt.setValue(org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus.NULL); 257 break; 258 } 259 return tgt; 260 } 261 262 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertCarePlanStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.CarePlan.CarePlanStatus> src) throws FHIRException { 263 if (src == null || src.isEmpty()) 264 return null; 265 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.RequestStatusEnumFactory()); 266 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 267 switch (src.getValue()) { 268 case PROPOSED: 269 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.DRAFT); 270 break; 271 case DRAFT: 272 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.DRAFT); 273 break; 274 case ACTIVE: 275 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.ACTIVE); 276 break; 277 case COMPLETED: 278 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.COMPLETED); 279 break; 280 case CANCELLED: 281 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.REVOKED); 282 break; 283 default: 284 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.NULL); 285 break; 286 } 287 return tgt; 288 } 289 290 static public CodeableReference convertReferenceToCodableReference(Reference src) { 291 CodeableReference tgt = new CodeableReference(); 292 tgt.setReference(Reference10_50.convertReference(src)); 293 return tgt; 294 } 295}