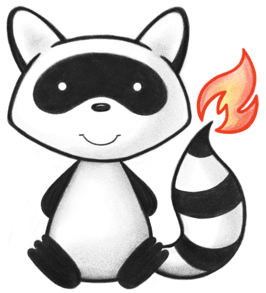
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 008import org.hl7.fhir.dstu2.model.Communication; 009import org.hl7.fhir.dstu2.model.Enumeration; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012import org.hl7.fhir.r5.model.Enumerations; 013 014public class Communication10_50 { 015 016 public static org.hl7.fhir.r5.model.Communication convertCommunication(org.hl7.fhir.dstu2.model.Communication src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.r5.model.Communication tgt = new org.hl7.fhir.r5.model.Communication(); 020 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 023 if (src.hasCategory()) 024 tgt.addCategory(CodeableConcept10_50.convertCodeableConcept(src.getCategory())); 025 if (src.hasSender()) 026 tgt.setSender(Reference10_50.convertReference(src.getSender())); 027 for (org.hl7.fhir.dstu2.model.Reference t : src.getRecipient()) 028 tgt.addRecipient(Reference10_50.convertReference(t)); 029 for (org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 030 tgt.addPayload(convertCommunicationPayloadComponent(t)); 031 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getMedium()) 032 tgt.addMedium(CodeableConcept10_50.convertCodeableConcept(t)); 033 if (src.hasStatus()) 034 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 035 if (src.hasEncounter()) 036 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 037 if (src.hasSentElement()) 038 tgt.setSentElement(DateTime10_50.convertDateTime(src.getSentElement())); 039 if (src.hasReceivedElement()) 040 tgt.setReceivedElement(DateTime10_50.convertDateTime(src.getReceivedElement())); 041 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReason()) 042 tgt.addReason(CodeableConcept10_50.convertCodeableConceptToCodableReference(t)); 043 if (src.hasSubject()) 044 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 045 return tgt; 046 } 047 048 public static org.hl7.fhir.dstu2.model.Communication convertCommunication(org.hl7.fhir.r5.model.Communication src) throws FHIRException { 049 if (src == null || src.isEmpty()) 050 return null; 051 org.hl7.fhir.dstu2.model.Communication tgt = new org.hl7.fhir.dstu2.model.Communication(); 052 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 055 if (src.hasCategory()) 056 tgt.setCategory(CodeableConcept10_50.convertCodeableConcept(src.getCategoryFirstRep())); 057 if (src.hasSender()) 058 tgt.setSender(Reference10_50.convertReference(src.getSender())); 059 for (org.hl7.fhir.r5.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference10_50.convertReference(t)); 060 for (org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 061 tgt.addPayload(convertCommunicationPayloadComponent(t)); 062 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getMedium()) 063 tgt.addMedium(CodeableConcept10_50.convertCodeableConcept(t)); 064 if (src.hasStatus()) 065 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 066 if (src.hasEncounter()) 067 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 068 if (src.hasSentElement()) 069 tgt.setSentElement(DateTime10_50.convertDateTime(src.getSentElement())); 070 if (src.hasReceivedElement()) 071 tgt.setReceivedElement(DateTime10_50.convertDateTime(src.getReceivedElement())); 072 for (CodeableReference t : src.getReason()) 073 if (t.hasConcept()) 074 tgt.addReason(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 075 if (src.hasSubject()) 076 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 081 if (src == null || src.isEmpty()) 082 return null; 083 org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent(); 084 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 085 if (src.hasContent()) 086 tgt.setContent(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getContent())); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.dstu2.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 091 if (src == null || src.isEmpty()) 092 return null; 093 org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent(); 094 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 095 if (src.hasContent()) 096 tgt.setContent(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getContent())); 097 return tgt; 098 } 099 100 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Communication.CommunicationStatus> convertCommunicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> src) throws FHIRException { 101 if (src == null || src.isEmpty()) 102 return null; 103 Enumeration<Communication.CommunicationStatus> tgt = new Enumeration<>(new Communication.CommunicationStatusEnumFactory()); 104 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 105 if (src.getValue() == null) { 106 tgt.setValue(null); 107 } else { 108 switch (src.getValue()) { 109 case INPROGRESS: 110 tgt.setValue(Communication.CommunicationStatus.INPROGRESS); 111 break; 112 case COMPLETED: 113 tgt.setValue(Communication.CommunicationStatus.COMPLETED); 114 break; 115 case ONHOLD: 116 tgt.setValue(Communication.CommunicationStatus.SUSPENDED); 117 break; 118 case ENTEREDINERROR: 119 tgt.setValue(Communication.CommunicationStatus.REJECTED); 120 break; 121 case NOTDONE: 122 tgt.setValue(Communication.CommunicationStatus.FAILED); 123 break; 124 default: 125 tgt.setValue(Communication.CommunicationStatus.NULL); 126 break; 127 } 128 } 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> convertCommunicationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Communication.CommunicationStatus> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 org.hl7.fhir.r5.model.Enumeration<Enumerations.EventStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.EventStatusEnumFactory()); 136 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case INPROGRESS: 142 tgt.setValue(Enumerations.EventStatus.INPROGRESS); 143 break; 144 case COMPLETED: 145 tgt.setValue(Enumerations.EventStatus.COMPLETED); 146 break; 147 case SUSPENDED: 148 tgt.setValue(Enumerations.EventStatus.ONHOLD); 149 break; 150 case REJECTED: 151 tgt.setValue(Enumerations.EventStatus.ENTEREDINERROR); 152 break; 153 case FAILED: 154 tgt.setValue(Enumerations.EventStatus.NOTDONE); 155 break; 156 default: 157 tgt.setValue(Enumerations.EventStatus.NULL); 158 break; 159 } 160 } 161 return tgt; 162 } 163}