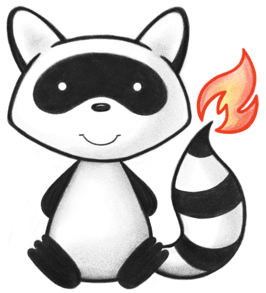
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.Objects; 006 007import org.hl7.fhir.convertors.SourceElementComponentWrapper; 008import org.hl7.fhir.convertors.VersionConvertorConstants; 009import org.hl7.fhir.convertors.context.ConversionContext10_50; 010import org.hl7.fhir.convertors.conv10_50.VersionConvertor_10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 014import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 015import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Code10_50; 016import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 017import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 018import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 019import org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence; 020import org.hl7.fhir.exceptions.FHIRException; 021import org.hl7.fhir.r5.model.CanonicalType; 022import org.hl7.fhir.r5.model.ConceptMap; 023import org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent; 024import org.hl7.fhir.r5.model.DataType; 025import org.hl7.fhir.r5.model.Enumeration; 026import org.hl7.fhir.r5.model.Enumerations; 027import org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship; 028import org.hl7.fhir.r5.utils.ToolingExtensions; 029 030public class ConceptMap10_50 { 031 032 public static org.hl7.fhir.r5.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu2.model.ConceptMap src) throws FHIRException { 033 if (src == null || src.isEmpty()) 034 return null; 035 org.hl7.fhir.r5.model.ConceptMap tgt = new org.hl7.fhir.r5.model.ConceptMap(); 036 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 037 if (src.hasUrlElement()) 038 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 039 if (src.hasIdentifier()) 040 tgt.addIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 041 if (src.hasVersionElement()) 042 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 043 if (src.hasNameElement()) 044 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 045 if (src.hasStatus()) 046 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 047 if (src.hasExperimental()) 048 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 049 if (src.hasPublisherElement()) 050 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 051 for (org.hl7.fhir.dstu2.model.ConceptMap.ConceptMapContactComponent t : src.getContact()) 052 tgt.addContact(convertConceptMapContactComponent(t)); 053 if (src.hasDate()) 054 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 055 if (src.hasDescription()) 056 tgt.setDescription(src.getDescription()); 057 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 058 if (VersionConvertor_10_50.isJurisdiction(t)) 059 tgt.addJurisdiction(CodeableConcept10_50.convertCodeableConcept(t)); 060 else 061 tgt.addUseContext(CodeableConcept10_50.convertCodeableConceptToUsageContext(t)); 062 if (src.hasRequirements()) 063 tgt.setPurpose(src.getRequirements()); 064 if (src.hasCopyright()) 065 tgt.setCopyright(src.getCopyright()); 066 if (src.hasSource()) { 067 org.hl7.fhir.r5.model.DataType r = ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getSource()); 068 tgt.setSourceScope(r instanceof org.hl7.fhir.r5.model.Reference ? new CanonicalType(((org.hl7.fhir.r5.model.Reference) r).getReference()) : r); 069 } 070 if (src.hasTarget()) { 071 DataType r = ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getTarget()); 072 tgt.setTargetScope(r instanceof org.hl7.fhir.r5.model.Reference ? new CanonicalType(((org.hl7.fhir.r5.model.Reference) r).getReference()) : r); 073 } 074 for (org.hl7.fhir.dstu2.model.ConceptMap.SourceElementComponent t : src.getElement()) { 075 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> ws = convertSourceElementComponent(t, tgt); 076 for (SourceElementComponentWrapper<ConceptMap.SourceElementComponent> w : ws) 077 getGroup(tgt, w.getSource(), w.getTarget()).addElement(w.getComp()); 078 } 079 return tgt; 080 } 081 082 public static org.hl7.fhir.dstu2.model.ConceptMap convertConceptMap(org.hl7.fhir.r5.model.ConceptMap src) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.dstu2.model.ConceptMap tgt = new org.hl7.fhir.dstu2.model.ConceptMap(); 086 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 087 if (src.hasUrlElement()) 088 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 089 if (src.hasIdentifier()) { 090 if (src.hasIdentifier()) 091 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifierFirstRep())); 092 } 093 if (src.hasVersionElement()) 094 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 095 if (src.hasNameElement()) 096 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 097 if (src.hasStatus()) 098 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 099 if (src.hasExperimental()) 100 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 101 if (src.hasPublisherElement()) 102 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 103 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) tgt.addContact(convertConceptMapContactComponent(t)); 104 if (src.hasDate()) 105 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 106 if (src.hasDescription()) 107 tgt.setDescription(src.getDescription()); 108 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 109 if (t.hasValueCodeableConcept()) 110 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t.getValueCodeableConcept())); 111 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 112 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t)); 113 if (src.hasPurpose()) 114 tgt.setRequirements(src.getPurpose()); 115 if (src.hasCopyright()) 116 tgt.setCopyright(src.getCopyright()); 117 if (src.hasSourceScope()) 118 tgt.setSource(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getSourceScope())); 119 if (src.hasTargetScope()) 120 tgt.setTarget(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getTargetScope())); 121 for (org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g : src.getGroup()) 122 for (org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent t : g.getElement()) 123 tgt.addElement(convertSourceElementComponent(t, g, src)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.r5.model.ContactDetail convertConceptMapContactComponent(org.hl7.fhir.dstu2.model.ConceptMap.ConceptMapContactComponent src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 131 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 132 if (src.hasNameElement()) 133 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 134 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 135 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 136 return tgt; 137 } 138 139 public static org.hl7.fhir.dstu2.model.ConceptMap.ConceptMapContactComponent convertConceptMapContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 140 if (src == null || src.isEmpty()) 141 return null; 142 org.hl7.fhir.dstu2.model.ConceptMap.ConceptMapContactComponent tgt = new org.hl7.fhir.dstu2.model.ConceptMap.ConceptMapContactComponent(); 143 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 144 if (src.hasNameElement()) 145 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 146 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 147 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 148 return tgt; 149 } 150 151 public static org.hl7.fhir.dstu2.model.Enumeration<ConceptMapEquivalence> convertConceptMapEquivalence(Enumeration<ConceptMapRelationship> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent ccm) throws FHIRException { 152 if (src == null || src.isEmpty()) 153 return null; 154 org.hl7.fhir.dstu2.model.Enumeration<ConceptMapEquivalence> tgt = new org.hl7.fhir.dstu2.model.Enumeration<ConceptMapEquivalence>(new org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 155 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 156 if (ccm.hasExtension(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)) { 157 tgt.setValueAsString(ccm.getExtensionString(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)); 158 } else { 159 switch (src.getValue()) { 160 case EQUIVALENT: 161 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 162 break; 163 case SOURCEISNARROWERTHANTARGET: 164 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.WIDER); 165 break; 166 case SOURCEISBROADERTHANTARGET: 167 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.NARROWER); 168 break; 169 case NOTRELATEDTO: 170 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.DISJOINT); 171 break; 172 default: 173 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.NULL); 174 break; 175 } 176 } 177 return tgt; 178 } 179 180 public static Enumeration<ConceptMapRelationship> convertConceptMapRelationship(org.hl7.fhir.dstu2.model.Enumeration<ConceptMapEquivalence> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgtCtxt) throws FHIRException { 181 if (src == null || src.isEmpty()) 182 return null; 183 Enumeration<ConceptMapRelationship> tgt = new Enumeration<ConceptMapRelationship>(new Enumerations.ConceptMapRelationshipEnumFactory()); 184 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 185 ToolingExtensions.setCodeExtensionMod(tgtCtxt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE, src.getValueAsString()); 186 switch (src.getValue()) { 187 case EQUIVALENT: 188 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.EQUIVALENT); 189 break; 190 case EQUAL: 191 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.EQUIVALENT); 192 break; 193 case WIDER: 194 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 195 break; 196 case SUBSUMES: 197 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 198 break; 199 case NARROWER: 200 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 201 break; 202 case SPECIALIZES: 203 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 204 break; 205 case INEXACT: 206 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.RELATEDTO); 207 break; 208 case UNMATCHED: 209 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.NULL); 210 break; 211 case DISJOINT: 212 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship.NOTRELATEDTO); 213 break; 214 default: 215 tgt.setValue(null); 216 break; 217 } 218 return tgt; 219 } 220 221 public static org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 222 if (src == null || src.isEmpty()) 223 return null; 224 org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent(); 225 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 226 if (src.hasAttribute()) 227 tgt.setElement(srcMap.getAttributeUri(src.getAttribute())); 228 if (src.hasValueCoding()) { 229 tgt.setCode(src.getValueCoding().getCode()); 230 } else if (src.hasValue()) { 231 tgt.setCode(src.getValue().primitiveValue()); 232 } 233 return tgt; 234 } 235 236 public static org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent(); 240 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 241 if (src.hasElementElement()) 242 tgt.setAttribute(tgtMap.registerAttribute(src.getElement())); 243 tgt.setValue(String10_50.convertString(src.getCodeElement())); 244 return tgt; 245 } 246 247 public static org.hl7.fhir.dstu2.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 248 if (src == null || src.isEmpty()) 249 return null; 250 org.hl7.fhir.dstu2.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu2.model.ConceptMap.SourceElementComponent(); 251 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 252 tgt.setCodeSystem(g.getSource()); 253 if (src.hasCodeElement()) 254 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 255 if (src.hasNoMap() && src.getNoMap() == true) { 256 tgt.addTarget(new org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent().setEquivalence(org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.UNMATCHED)); 257 } else { 258 for (org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent t : src.getTarget()) 259 tgt.addTarget(convertTargetElementComponent(t, g, srcMap)); 260 } 261 return tgt; 262 } 263 264 public static List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> convertSourceElementComponent(org.hl7.fhir.dstu2.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 265 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> res = new ArrayList<>(); 266 if (src == null || src.isEmpty()) 267 return res; 268 for (org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent t : src.getTarget()) { 269 org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent(); 270 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 271 tgt.setCode(src.getCode()); 272 if (t.getEquivalence() == org.hl7.fhir.dstu2.model.Enumerations.ConceptMapEquivalence.UNMATCHED) { 273 tgt.setNoMap(true); 274 } else { 275 tgt.addTarget(convertTargetElementComponent(t, tgtMap)); 276 } 277 res.add(new SourceElementComponentWrapper<>(tgt, src.getCodeSystem(), t.getCodeSystem())); 278 } 279 return res; 280 } 281 282 public static org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent g, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 283 if (src == null || src.isEmpty()) 284 return null; 285 org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent(); 286 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE); 287 tgt.setCodeSystem(g.getTarget()); 288 if (src.hasCodeElement()) 289 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 290 if (src.hasRelationship()) 291 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getRelationshipElement(), src)); 292 if (src.hasCommentElement()) 293 tgt.setCommentsElement(String10_50.convertString(src.getCommentElement())); 294 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 295 tgt.addDependsOn(convertOtherElementComponent(t, srcMap)); 296 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getProduct()) 297 tgt.addProduct(convertOtherElementComponent(t, srcMap)); 298 return tgt; 299 } 300 301 public static org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu2.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 302 if (src == null || src.isEmpty()) 303 return null; 304 org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent(); 305 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 306 if (src.hasCodeElement()) 307 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 308 if (src.hasEquivalence()) 309 tgt.setRelationshipElement(convertConceptMapRelationship(src.getEquivalenceElement(), tgt)); 310 if (src.hasCommentsElement()) 311 tgt.setCommentElement(String10_50.convertString(src.getCommentsElement())); 312 for (org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 313 tgt.addDependsOn(convertOtherElementComponent(t, tgtMap)); 314 for (org.hl7.fhir.dstu2.model.ConceptMap.OtherElementComponent t : src.getProduct()) 315 tgt.addProduct(convertOtherElementComponent(t, tgtMap)); 316 return tgt; 317 } 318 319 static public ConceptMapGroupComponent getGroup(ConceptMap map, String srcs, String tgts) { 320 for (ConceptMapGroupComponent grp : map.getGroup()) { 321 if (Objects.equals(grp.getSource(), srcs) && Objects.equals(grp.getTarget(), tgts)) 322 return grp; 323 } 324 ConceptMapGroupComponent grp = map.addGroup(); 325 grp.setSource(srcs); 326 grp.setTarget(tgts); 327 return grp; 328 } 329}