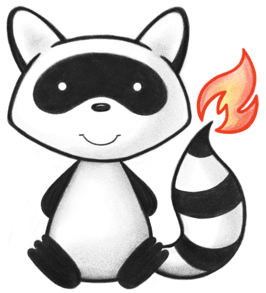
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_50; 005import org.hl7.fhir.convertors.context.ConversionContext10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Coding10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Code10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 014import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.UnsignedInt10_50; 015import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 016import org.hl7.fhir.dstu2.model.Conformance; 017import org.hl7.fhir.dstu2.model.Enumeration; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r5.model.CapabilityStatement; 020import org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent; 021import org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent; 022import org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction; 023import org.hl7.fhir.r5.model.Enumerations; 024 025public class Conformance10_50 { 026 027 private static final String[] IGNORED_EXTENSION_URLS = new String[]{ 028 VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL 029 }; 030 031 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> src) throws FHIRException { 032 if (src == null || src.isEmpty()) 033 return null; 034 Enumeration<Conformance.ConditionalDeleteStatus> tgt = new Enumeration<>(new Conformance.ConditionalDeleteStatusEnumFactory()); 035 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 036 if (src.getValue() == null) { 037 tgt.setValue(null); 038 } else { 039 switch (src.getValue()) { 040 case NOTSUPPORTED: 041 tgt.setValue(Conformance.ConditionalDeleteStatus.NOTSUPPORTED); 042 break; 043 case SINGLE: 044 tgt.setValue(Conformance.ConditionalDeleteStatus.SINGLE); 045 break; 046 case MULTIPLE: 047 tgt.setValue(Conformance.ConditionalDeleteStatus.MULTIPLE); 048 break; 049 default: 050 tgt.setValue(Conformance.ConditionalDeleteStatus.NULL); 051 break; 052 } 053 } 054 return tgt; 055 } 056 057 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ConditionalDeleteStatus> convertConditionalDeleteStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConditionalDeleteStatus> src) throws FHIRException { 058 if (src == null || src.isEmpty()) 059 return null; 060 org.hl7.fhir.r5.model.Enumeration<CapabilityStatement.ConditionalDeleteStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.ConditionalDeleteStatusEnumFactory()); 061 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 062 if (src.getValue() == null) { 063 tgt.setValue(null); 064 } else { 065 switch (src.getValue()) { 066 case NOTSUPPORTED: 067 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NOTSUPPORTED); 068 break; 069 case SINGLE: 070 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.SINGLE); 071 break; 072 case MULTIPLE: 073 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.MULTIPLE); 074 break; 075 default: 076 tgt.setValue(CapabilityStatement.ConditionalDeleteStatus.NULL); 077 break; 078 } 079 } 080 return tgt; 081 } 082 083 public static org.hl7.fhir.dstu2.model.Conformance convertConformance(org.hl7.fhir.r5.model.CapabilityStatement src) throws FHIRException { 084 return convertConformance(src, new BaseAdvisor_10_50()); 085 } 086 087 public static org.hl7.fhir.dstu2.model.Conformance convertConformance(org.hl7.fhir.r5.model.CapabilityStatement src, BaseAdvisor_10_50 advisor) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.dstu2.model.Conformance tgt = new org.hl7.fhir.dstu2.model.Conformance(); 091 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt, IGNORED_EXTENSION_URLS); 092 if (src.hasUrlElement()) 093 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 094 if (src.hasVersionElement()) 095 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 096 if (src.hasNameElement()) 097 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 098 if (src.hasStatus()) 099 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 100 if (src.hasExperimental()) 101 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 102 if (src.hasDate()) 103 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 104 if (src.hasPublisherElement()) 105 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 106 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 107 tgt.addContact(convertConformanceContactComponent(t)); 108 if (src.hasDescription()) 109 tgt.setDescription(src.getDescription()); 110 if (src.hasPurpose()) 111 tgt.setRequirements(src.getPurpose()); 112 if (src.hasCopyright()) 113 tgt.setCopyright(src.getCopyright()); 114 if (src.hasKind()) 115 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 116 if (src.hasSoftware()) 117 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 118 if (src.hasImplementation()) 119 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 120 if (src.hasFhirVersion()) 121 tgt.setFhirVersion(src.getFhirVersion().toCode()); 122 if (src.hasExtension(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL)) 123 tgt.setAcceptUnknown(org.hl7.fhir.dstu2.model.Conformance.UnknownContentCode.fromCode(src.getExtensionByUrl(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL).getValue().primitiveValue())); 124 for (org.hl7.fhir.r5.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 125 for (CapabilityStatementRestComponent r : src.getRest()) 126 for (CapabilityStatementRestResourceComponent rr : r.getResource()) 127 for (org.hl7.fhir.r5.model.CanonicalType t : rr.getSupportedProfile()) 128 tgt.addProfile(Reference10_50.convertCanonicalToReference(t)); 129 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent t : src.getRest()) 130 tgt.addRest(convertConformanceRestComponent(t)); 131 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent t : src.getMessaging()) 132 tgt.addMessaging(convertConformanceMessagingComponent(t)); 133 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent t : src.getDocument()) 134 tgt.addDocument(convertConformanceDocumentComponent(t)); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.r5.model.CapabilityStatement convertConformance(org.hl7.fhir.dstu2.model.Conformance src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.r5.model.CapabilityStatement tgt = new org.hl7.fhir.r5.model.CapabilityStatement(); 142 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 143 if (src.hasUrlElement()) 144 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 145 if (src.hasVersionElement()) 146 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 147 if (src.hasNameElement()) 148 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 149 if (src.hasStatus()) 150 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 151 if (src.hasExperimental()) 152 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 153 if (src.hasDate()) 154 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 155 if (src.hasPublisherElement()) 156 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 157 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent t : src.getContact()) 158 tgt.addContact(convertConformanceContactComponent(t)); 159 if (src.hasDescription()) 160 tgt.setDescription(src.getDescription()); 161 if (src.hasRequirements()) 162 tgt.setPurpose(src.getRequirements()); 163 if (src.hasCopyright()) 164 tgt.setCopyright(src.getCopyright()); 165 if (src.hasKind()) 166 tgt.setKindElement(convertConformanceStatementKind(src.getKindElement())); 167 if (src.hasSoftware()) 168 tgt.setSoftware(convertConformanceSoftwareComponent(src.getSoftware())); 169 if (src.hasImplementation()) 170 tgt.setImplementation(convertConformanceImplementationComponent(src.getImplementation())); 171 if (src.hasFhirVersion()) 172 tgt.setFhirVersion(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 173 if (src.hasAcceptUnknown()) 174 tgt.addExtension().setUrl(VersionConvertorConstants.EXT_ACCEPT_UNKNOWN_EXTENSION_URL).setValue(new org.hl7.fhir.r5.model.CodeType(src.getAcceptUnknownElement().asStringValue())); 175 for (org.hl7.fhir.dstu2.model.CodeType t : src.getFormat()) tgt.addFormat(t.getValue()); 176 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent t : src.getRest()) 177 tgt.addRest(convertConformanceRestComponent(t)); 178 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent t : src.getMessaging()) 179 tgt.addMessaging(convertConformanceMessagingComponent(t)); 180 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent t : src.getDocument()) 181 tgt.addDocument(convertConformanceDocumentComponent(t)); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent convertConformanceContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 186 if (src == null || src.isEmpty()) 187 return null; 188 org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent(); 189 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 190 if (src.hasNameElement()) 191 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 192 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 193 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 194 return tgt; 195 } 196 197 public static org.hl7.fhir.r5.model.ContactDetail convertConformanceContactComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceContactComponent src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 201 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 202 if (src.hasNameElement()) 203 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 204 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 205 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent src) throws FHIRException { 210 if (src == null || src.isEmpty()) 211 return null; 212 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent(); 213 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 214 if (src.hasMode()) 215 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 216 if (src.hasDocumentation()) 217 tgt.setDocumentation(src.getDocumentation()); 218 if (src.hasProfile()) 219 tgt.setProfileElement(Reference10_50.convertReferenceToCanonical(src.getProfile())); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent convertConformanceDocumentComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementDocumentComponent src) throws FHIRException { 224 if (src == null || src.isEmpty()) 225 return null; 226 org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceDocumentComponent(); 227 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 228 if (src.hasMode()) 229 tgt.setModeElement(convertDocumentMode(src.getModeElement())); 230 if (src.hasDocumentation()) 231 tgt.setDocumentation(src.getDocumentation()); 232 if (src.hasProfileElement()) 233 tgt.setProfile(Reference10_50.convertCanonicalToReference(src.getProfileElement())); 234 return tgt; 235 } 236 237 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent(); 241 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 242 if (src.hasDescriptionElement()) 243 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 244 if (src.hasUrl()) 245 tgt.setUrl(src.getUrl()); 246 return tgt; 247 } 248 249 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent convertConformanceImplementationComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceImplementationComponent src) throws FHIRException { 250 if (src == null || src.isEmpty()) 251 return null; 252 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementImplementationComponent(); 253 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 254 if (src.hasDescriptionElement()) 255 tgt.setDescriptionElement(String10_50.convertStringToMarkdown(src.getDescriptionElement())); 256 if (src.hasUrl()) 257 tgt.setUrl(src.getUrl()); 258 return tgt; 259 } 260 261 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent src) throws FHIRException { 262 if (src == null || src.isEmpty()) 263 return null; 264 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent(); 265 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 266 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent t : src.getEndpoint()) 267 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 268 if (src.hasReliableCacheElement()) 269 tgt.setReliableCacheElement(UnsignedInt10_50.convertUnsignedInt(src.getReliableCacheElement())); 270 if (src.hasDocumentation()) 271 tgt.setDocumentation(src.getDocumentation()); 272 for (org.hl7.fhir.r5.model.Extension e : src.getExtensionsByUrl(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT)) { 273 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent event = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent(); 274 tgt.addEvent(event); 275 event.setCode(Coding10_50.convertCoding((org.hl7.fhir.r5.model.Coding) e.getExtensionByUrl("code").getValue())); 276 if (e.hasExtension("category")) 277 event.setCategory(org.hl7.fhir.dstu2.model.Conformance.MessageSignificanceCategory.fromCode(e.getExtensionByUrl("category").getValue().toString())); 278 event.setMode(org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode.fromCode(e.getExtensionByUrl("mode").getValue().toString())); 279 event.setCode(Coding10_50.convertCoding((org.hl7.fhir.r5.model.Coding) e.getExtensionByUrl("code").getValue())); 280 org.hl7.fhir.r5.model.Extension focusE = e.getExtensionByUrl("focus"); 281 if (focusE.getValue().hasPrimitiveValue()) 282 event.setFocus(focusE.getValue().toString()); 283 else { 284 event.setFocusElement(new org.hl7.fhir.dstu2.model.CodeType()); 285 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(focusE.getValue(), event.getFocusElement()); 286 } 287 event.setRequest(Reference10_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("request").getValue())); 288 event.setResponse(Reference10_50.convertReference((org.hl7.fhir.r5.model.Reference) e.getExtensionByUrl("response").getValue())); 289 if (e.hasExtension("documentation")) 290 event.setDocumentation(e.getExtensionByUrl("documentation").getValue().toString()); 291 } 292 return tgt; 293 } 294 295 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent convertConformanceMessagingComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingComponent src) throws FHIRException { 296 if (src == null || src.isEmpty()) 297 return null; 298 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingComponent(); 299 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 300 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent t : src.getEndpoint()) 301 tgt.addEndpoint(convertConformanceMessagingEndpointComponent(t)); 302 if (src.hasReliableCacheElement()) 303 tgt.setReliableCacheElement(UnsignedInt10_50.convertUnsignedInt(src.getReliableCacheElement())); 304 if (src.hasDocumentation()) 305 tgt.setDocumentation(src.getDocumentation()); 306 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEventComponent t : src.getEvent()) { 307 org.hl7.fhir.r5.model.Extension e = new org.hl7.fhir.r5.model.Extension(VersionConvertorConstants.EXT_IG_CONFORMANCE_MESSAGE_EVENT); 308 e.addExtension(new org.hl7.fhir.r5.model.Extension("code", Coding10_50.convertCoding(t.getCode()))); 309 if (t.hasCategory()) 310 e.addExtension(new org.hl7.fhir.r5.model.Extension("category", new org.hl7.fhir.r5.model.CodeType(t.getCategory().toCode()))); 311 e.addExtension(new org.hl7.fhir.r5.model.Extension("mode", new org.hl7.fhir.r5.model.CodeType(t.getMode().toCode()))); 312 if (t.getFocusElement().hasValue()) 313 e.addExtension(new org.hl7.fhir.r5.model.Extension("focus", new org.hl7.fhir.r5.model.StringType(t.getFocus()))); 314 else { 315 org.hl7.fhir.r5.model.CodeType focus = new org.hl7.fhir.r5.model.CodeType(); 316 org.hl7.fhir.r5.model.Extension focusE = new org.hl7.fhir.r5.model.Extension("focus", focus); 317 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(t.getFocusElement(), focus); 318 e.addExtension(focusE); 319 } 320 e.addExtension(new org.hl7.fhir.r5.model.Extension("request", Reference10_50.convertReference(t.getRequest()))); 321 e.addExtension(new org.hl7.fhir.r5.model.Extension("response", Reference10_50.convertReference(t.getResponse()))); 322 if (t.hasDocumentation()) 323 e.addExtension(new org.hl7.fhir.r5.model.Extension("documentation", new org.hl7.fhir.r5.model.StringType(t.getDocumentation()))); 324 tgt.addExtension(e); 325 } 326 return tgt; 327 } 328 329 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent src) throws FHIRException { 330 if (src == null || src.isEmpty()) 331 return null; 332 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent(); 333 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 334 if (src.hasProtocol()) 335 tgt.setProtocol(Coding10_50.convertCoding(src.getProtocol())); 336 if (src.hasAddress()) 337 tgt.setAddress(src.getAddress()); 338 return tgt; 339 } 340 341 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent convertConformanceMessagingEndpointComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementMessagingEndpointComponent src) throws FHIRException { 342 if (src == null || src.isEmpty()) 343 return null; 344 org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceMessagingEndpointComponent(); 345 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 346 if (src.hasProtocol()) 347 tgt.setProtocol(Coding10_50.convertCoding(src.getProtocol())); 348 if (src.hasAddress()) 349 tgt.setAddress(src.getAddress()); 350 return tgt; 351 } 352 353 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent convertConformanceRestComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent src) throws FHIRException { 354 if (src == null || src.isEmpty()) 355 return null; 356 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent(); 357 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 358 if (src.hasMode()) 359 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 360 if (src.hasDocumentation()) 361 tgt.setDocumentation(src.getDocumentation()); 362 if (src.hasSecurity()) 363 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 364 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent t : src.getResource()) 365 tgt.addResource(convertConformanceRestResourceComponent(t)); 366 for (org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent t : src.getInteraction()) 367 tgt.addInteraction(convertSystemInteractionComponent(t)); 368 if (src.getTransactionMode() == org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BATCH || src.getTransactionMode() == org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BOTH) 369 tgt.addInteraction().setCode(SystemRestfulInteraction.BATCH); 370 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 371 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 372 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent t : src.getOperation()) 373 tgt.addOperation(convertConformanceRestOperationComponent(t)); 374 for (org.hl7.fhir.dstu2.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 375 return tgt; 376 } 377 378 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent convertConformanceRestComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestComponent src) throws FHIRException { 379 if (src == null || src.isEmpty()) 380 return null; 381 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestComponent(); 382 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 383 if (src.hasMode()) 384 tgt.setModeElement(convertRestfulConformanceMode(src.getModeElement())); 385 if (src.hasDocumentation()) 386 tgt.setDocumentation(src.getDocumentation()); 387 if (src.hasSecurity()) 388 tgt.setSecurity(convertConformanceRestSecurityComponent(src.getSecurity())); 389 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent t : src.getResource()) 390 tgt.addResource(convertConformanceRestResourceComponent(t)); 391 boolean batch = false; 392 boolean transaction = false; 393 for (org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent t : src.getInteraction()) { 394 if (t.getCode().equals(SystemRestfulInteraction.BATCH)) 395 batch = true; 396 else 397 tgt.addInteraction(convertSystemInteractionComponent(t)); 398 if (t.getCode().equals(SystemRestfulInteraction.TRANSACTION)) 399 transaction = true; 400 } 401 if (batch) 402 tgt.setTransactionMode(transaction ? org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BOTH : org.hl7.fhir.dstu2.model.Conformance.TransactionMode.BATCH); 403 else 404 tgt.setTransactionMode(transaction ? org.hl7.fhir.dstu2.model.Conformance.TransactionMode.TRANSACTION : org.hl7.fhir.dstu2.model.Conformance.TransactionMode.NOTSUPPORTED); 405 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 406 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 407 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent t : src.getOperation()) 408 tgt.addOperation(convertConformanceRestOperationComponent(t)); 409 for (org.hl7.fhir.r5.model.UriType t : src.getCompartment()) tgt.addCompartment(t.getValue()); 410 return tgt; 411 } 412 413 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent src) throws FHIRException { 414 if (src == null || src.isEmpty()) 415 return null; 416 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent(); 417 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 418 if (src.hasNameElement()) 419 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 420 if (src.hasDefinitionElement()) 421 tgt.setDefinition(Reference10_50.convertCanonicalToReference(src.getDefinitionElement())); 422 return tgt; 423 } 424 425 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent convertConformanceRestOperationComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestOperationComponent src) throws FHIRException { 426 if (src == null || src.isEmpty()) 427 return null; 428 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceOperationComponent(); 429 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 430 if (src.hasNameElement()) 431 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 432 if (src.hasDefinition()) 433 tgt.setDefinitionElement(Reference10_50.convertReferenceToCanonical(src.getDefinition())); 434 return tgt; 435 } 436 437 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent src) throws FHIRException { 438 if (src == null || src.isEmpty()) 439 return null; 440 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent(); 441 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 442 if (src.hasTypeElement()) 443 tgt.setTypeElement(Code10_50.convertCode(src.getTypeElement())); 444 if (src.hasProfile()) 445 tgt.setProfileElement(Reference10_50.convertReferenceToCanonical(src.getProfile())); 446 for (org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent t : src.getInteraction()) 447 tgt.addInteraction(convertResourceInteractionComponent(t)); 448 if (src.hasVersioning()) 449 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 450 if (src.hasReadHistoryElement()) 451 tgt.setReadHistoryElement(Boolean10_50.convertBoolean(src.getReadHistoryElement())); 452 if (src.hasUpdateCreateElement()) 453 tgt.setUpdateCreateElement(Boolean10_50.convertBoolean(src.getUpdateCreateElement())); 454 if (src.hasConditionalCreateElement()) 455 tgt.setConditionalCreateElement(Boolean10_50.convertBoolean(src.getConditionalCreateElement())); 456 if (src.hasConditionalUpdateElement()) 457 tgt.setConditionalUpdateElement(Boolean10_50.convertBoolean(src.getConditionalUpdateElement())); 458 if (src.hasConditionalDelete()) 459 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 460 for (org.hl7.fhir.dstu2.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 461 for (org.hl7.fhir.dstu2.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 462 for (org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent t : src.getSearchParam()) 463 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 464 return tgt; 465 } 466 467 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent convertConformanceRestResourceComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceComponent src) throws FHIRException { 468 if (src == null || src.isEmpty()) 469 return null; 470 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceComponent(); 471 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 472 if (src.hasType()) { 473 if (src.hasTypeElement()) 474 tgt.setTypeElement(Code10_50.convertCode(src.getTypeElement())); 475 } 476 if (src.hasProfile()) 477 tgt.setProfile(Reference10_50.convertCanonicalToReference(src.getProfileElement())); 478 for (org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent t : src.getInteraction()) 479 tgt.addInteraction(convertResourceInteractionComponent(t)); 480 if (src.hasVersioning()) 481 tgt.setVersioningElement(convertResourceVersionPolicy(src.getVersioningElement())); 482 if (src.hasReadHistoryElement()) 483 tgt.setReadHistoryElement(Boolean10_50.convertBoolean(src.getReadHistoryElement())); 484 if (src.hasUpdateCreateElement()) 485 tgt.setUpdateCreateElement(Boolean10_50.convertBoolean(src.getUpdateCreateElement())); 486 if (src.hasConditionalCreateElement()) 487 tgt.setConditionalCreateElement(Boolean10_50.convertBoolean(src.getConditionalCreateElement())); 488 if (src.hasConditionalUpdateElement()) 489 tgt.setConditionalUpdateElement(Boolean10_50.convertBoolean(src.getConditionalUpdateElement())); 490 if (src.hasConditionalDelete()) 491 tgt.setConditionalDeleteElement(convertConditionalDeleteStatus(src.getConditionalDeleteElement())); 492 for (org.hl7.fhir.r5.model.StringType t : src.getSearchInclude()) tgt.addSearchInclude(t.getValue()); 493 for (org.hl7.fhir.r5.model.StringType t : src.getSearchRevInclude()) tgt.addSearchRevInclude(t.getValue()); 494 for (org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent t : src.getSearchParam()) 495 tgt.addSearchParam(convertConformanceRestResourceSearchParamComponent(t)); 496 return tgt; 497 } 498 499 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent src) throws FHIRException { 500 if (src == null || src.isEmpty()) 501 return null; 502 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent(); 503 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 504 if (src.hasNameElement()) 505 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 506 if (src.hasDefinition()) 507 tgt.setDefinition(src.getDefinition()); 508 if (src.hasType()) 509 tgt.setTypeElement(Enumerations10_50.convertSearchParamType(src.getTypeElement())); 510 if (src.hasDocumentation()) 511 tgt.setDocumentation(src.getDocumentation()); 512 return tgt; 513 } 514 515 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent convertConformanceRestResourceSearchParamComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestResourceSearchParamComponent src) throws FHIRException { 516 if (src == null || src.isEmpty()) 517 return null; 518 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestResourceSearchParamComponent(); 519 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 520 if (src.hasNameElement()) 521 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 522 if (src.hasDefinition()) 523 tgt.setDefinition(src.getDefinition()); 524 if (src.hasType()) 525 tgt.setTypeElement(Enumerations10_50.convertSearchParamType(src.getTypeElement())); 526 if (src.hasDocumentation()) 527 tgt.setDocumentation(src.getDocumentation()); 528 return tgt; 529 } 530 531 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent src) throws FHIRException { 532 if (src == null || src.isEmpty()) 533 return null; 534 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent(); 535 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 536 if (src.hasCorsElement()) 537 tgt.setCorsElement(Boolean10_50.convertBoolean(src.getCorsElement())); 538 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getService()) 539 tgt.addService(CodeableConcept10_50.convertCodeableConcept(t)); 540 if (src.hasDescription()) 541 tgt.setDescription(src.getDescription()); 542 return tgt; 543 } 544 545 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent convertConformanceRestSecurityComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementRestSecurityComponent src) throws FHIRException { 546 if (src == null || src.isEmpty()) 547 return null; 548 org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceRestSecurityComponent(); 549 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 550 if (src.hasCorsElement()) 551 tgt.setCorsElement(Boolean10_50.convertBoolean(src.getCorsElement())); 552 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getService()) 553 tgt.addService(CodeableConcept10_50.convertCodeableConcept(t)); 554 if (src.hasDescription()) 555 tgt.setDescription(src.getDescription()); 556 return tgt; 557 } 558 559 public static org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent src) throws FHIRException { 560 if (src == null || src.isEmpty()) 561 return null; 562 org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent(); 563 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 564 if (src.hasNameElement()) 565 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 566 if (src.hasVersionElement()) 567 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 568 if (src.hasReleaseDateElement()) 569 tgt.setReleaseDateElement(DateTime10_50.convertDateTime(src.getReleaseDateElement())); 570 return tgt; 571 } 572 573 public static org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent convertConformanceSoftwareComponent(org.hl7.fhir.dstu2.model.Conformance.ConformanceSoftwareComponent src) throws FHIRException { 574 if (src == null || src.isEmpty()) 575 return null; 576 org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.CapabilityStatementSoftwareComponent(); 577 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 578 if (src.hasNameElement()) 579 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 580 if (src.hasVersionElement()) 581 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 582 if (src.hasReleaseDateElement()) 583 tgt.setReleaseDateElement(DateTime10_50.convertDateTime(src.getReleaseDateElement())); 584 return tgt; 585 } 586 587 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceStatementKind> convertConformanceStatementKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> src) throws FHIRException { 588 if (src == null || src.isEmpty()) 589 return null; 590 Enumeration<Conformance.ConformanceStatementKind> tgt = new Enumeration<>(new Conformance.ConformanceStatementKindEnumFactory()); 591 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 592 if (src.getValue() == null) { 593 tgt.setValue(null); 594 } else { 595 switch (src.getValue()) { 596 case INSTANCE: 597 tgt.setValue(Conformance.ConformanceStatementKind.INSTANCE); 598 break; 599 case CAPABILITY: 600 tgt.setValue(Conformance.ConformanceStatementKind.CAPABILITY); 601 break; 602 case REQUIREMENTS: 603 tgt.setValue(Conformance.ConformanceStatementKind.REQUIREMENTS); 604 break; 605 default: 606 tgt.setValue(Conformance.ConformanceStatementKind.NULL); 607 break; 608 } 609 } 610 return tgt; 611 } 612 613 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CapabilityStatementKind> convertConformanceStatementKind(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceStatementKind> src) throws FHIRException { 614 if (src == null || src.isEmpty()) 615 return null; 616 org.hl7.fhir.r5.model.Enumeration<Enumerations.CapabilityStatementKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.CapabilityStatementKindEnumFactory()); 617 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 618 if (src.getValue() == null) { 619 tgt.setValue(null); 620 } else { 621 switch (src.getValue()) { 622 case INSTANCE: 623 tgt.setValue(Enumerations.CapabilityStatementKind.INSTANCE); 624 break; 625 case CAPABILITY: 626 tgt.setValue(Enumerations.CapabilityStatementKind.CAPABILITY); 627 break; 628 case REQUIREMENTS: 629 tgt.setValue(Enumerations.CapabilityStatementKind.REQUIREMENTS); 630 break; 631 default: 632 tgt.setValue(Enumerations.CapabilityStatementKind.NULL); 633 break; 634 } 635 } 636 return tgt; 637 } 638 639 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.DocumentMode> convertDocumentMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> src) throws FHIRException { 640 if (src == null || src.isEmpty()) 641 return null; 642 Enumeration<Conformance.DocumentMode> tgt = new Enumeration<>(new Conformance.DocumentModeEnumFactory()); 643 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 644 if (src.getValue() == null) { 645 tgt.setValue(null); 646 } else { 647 switch (src.getValue()) { 648 case PRODUCER: 649 tgt.setValue(Conformance.DocumentMode.PRODUCER); 650 break; 651 case CONSUMER: 652 tgt.setValue(Conformance.DocumentMode.CONSUMER); 653 break; 654 default: 655 tgt.setValue(Conformance.DocumentMode.NULL); 656 break; 657 } 658 } 659 return tgt; 660 } 661 662 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.DocumentMode> convertDocumentMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.DocumentMode> src) throws FHIRException { 663 if (src == null || src.isEmpty()) 664 return null; 665 org.hl7.fhir.r5.model.Enumeration<CapabilityStatement.DocumentMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.DocumentModeEnumFactory()); 666 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 667 if (src.getValue() == null) { 668 tgt.setValue(null); 669 } else { 670 switch (src.getValue()) { 671 case PRODUCER: 672 tgt.setValue(CapabilityStatement.DocumentMode.PRODUCER); 673 break; 674 case CONSUMER: 675 tgt.setValue(CapabilityStatement.DocumentMode.CONSUMER); 676 break; 677 default: 678 tgt.setValue(CapabilityStatement.DocumentMode.NULL); 679 break; 680 } 681 } 682 return tgt; 683 } 684 685 public static org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent src) throws FHIRException { 686 if (src == null || src.isEmpty()) 687 return null; 688 org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent(); 689 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 690 if (src.hasCode()) 691 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 692 if (src.hasDocumentation()) 693 tgt.setDocumentation(src.getDocumentation()); 694 return tgt; 695 } 696 697 public static org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent convertResourceInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.ResourceInteractionComponent src) throws FHIRException { 698 if (src == null || src.isEmpty()) 699 return null; 700 org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.ResourceInteractionComponent(); 701 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 702 if (src.hasCode()) 703 tgt.setCodeElement(convertTypeRestfulInteraction(src.getCodeElement())); 704 if (src.hasDocumentation()) 705 tgt.setDocumentation(src.getDocumentation()); 706 return tgt; 707 } 708 709 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ResourceVersionPolicy> src) throws FHIRException { 710 if (src == null || src.isEmpty()) 711 return null; 712 org.hl7.fhir.r5.model.Enumeration<CapabilityStatement.ResourceVersionPolicy> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.ResourceVersionPolicyEnumFactory()); 713 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 714 if (src.getValue() == null) { 715 tgt.setValue(null); 716 } else { 717 switch (src.getValue()) { 718 case NOVERSION: 719 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NOVERSION); 720 break; 721 case VERSIONED: 722 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONED); 723 break; 724 case VERSIONEDUPDATE: 725 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.VERSIONEDUPDATE); 726 break; 727 default: 728 tgt.setValue(CapabilityStatement.ResourceVersionPolicy.NULL); 729 break; 730 } 731 } 732 return tgt; 733 } 734 735 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ResourceVersionPolicy> convertResourceVersionPolicy(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.ResourceVersionPolicy> src) throws FHIRException { 736 if (src == null || src.isEmpty()) 737 return null; 738 Enumeration<Conformance.ResourceVersionPolicy> tgt = new Enumeration<>(new Conformance.ResourceVersionPolicyEnumFactory()); 739 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 740 if (src.getValue() == null) { 741 tgt.setValue(null); 742 } else { 743 switch (src.getValue()) { 744 case NOVERSION: 745 tgt.setValue(Conformance.ResourceVersionPolicy.NOVERSION); 746 break; 747 case VERSIONED: 748 tgt.setValue(Conformance.ResourceVersionPolicy.VERSIONED); 749 break; 750 case VERSIONEDUPDATE: 751 tgt.setValue(Conformance.ResourceVersionPolicy.VERSIONEDUPDATE); 752 break; 753 default: 754 tgt.setValue(Conformance.ResourceVersionPolicy.NULL); 755 break; 756 } 757 } 758 return tgt; 759 } 760 761 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> convertRestfulConformanceMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.RestfulConformanceMode> src) throws FHIRException { 762 if (src == null || src.isEmpty()) 763 return null; 764 org.hl7.fhir.r5.model.Enumeration<CapabilityStatement.RestfulCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.RestfulCapabilityModeEnumFactory()); 765 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 766 if (src.getValue() == null) { 767 tgt.setValue(null); 768 } else { 769 switch (src.getValue()) { 770 case CLIENT: 771 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.CLIENT); 772 break; 773 case SERVER: 774 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.SERVER); 775 break; 776 default: 777 tgt.setValue(CapabilityStatement.RestfulCapabilityMode.NULL); 778 break; 779 } 780 } 781 return tgt; 782 } 783 784 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.RestfulConformanceMode> convertRestfulConformanceMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.RestfulCapabilityMode> src) throws FHIRException { 785 if (src == null || src.isEmpty()) 786 return null; 787 Enumeration<Conformance.RestfulConformanceMode> tgt = new Enumeration<>(new Conformance.RestfulConformanceModeEnumFactory()); 788 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 789 if (src.getValue() == null) { 790 tgt.setValue(null); 791 } else { 792 switch (src.getValue()) { 793 case CLIENT: 794 tgt.setValue(Conformance.RestfulConformanceMode.CLIENT); 795 break; 796 case SERVER: 797 tgt.setValue(Conformance.RestfulConformanceMode.SERVER); 798 break; 799 default: 800 tgt.setValue(Conformance.RestfulConformanceMode.NULL); 801 break; 802 } 803 } 804 return tgt; 805 } 806 807 public static org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent src) throws FHIRException { 808 if (src == null || src.isEmpty()) 809 return null; 810 org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent tgt = new org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent(); 811 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 812 if (src.hasCode()) 813 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 814 if (src.hasDocumentation()) 815 tgt.setDocumentation(src.getDocumentation()); 816 return tgt; 817 } 818 819 public static org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent convertSystemInteractionComponent(org.hl7.fhir.dstu2.model.Conformance.SystemInteractionComponent src) throws FHIRException { 820 if (src == null || src.isEmpty()) 821 return null; 822 org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent tgt = new org.hl7.fhir.r5.model.CapabilityStatement.SystemInteractionComponent(); 823 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 824 if (src.hasCode()) 825 tgt.setCodeElement(convertSystemRestfulInteraction(src.getCodeElement())); 826 if (src.hasDocumentation()) 827 tgt.setDocumentation(src.getDocumentation()); 828 return tgt; 829 } 830 831 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.SystemRestfulInteraction> src) throws FHIRException { 832 if (src == null || src.isEmpty()) 833 return null; 834 org.hl7.fhir.r5.model.Enumeration<SystemRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.SystemRestfulInteractionEnumFactory()); 835 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 836 if (src.getValue() == null) { 837 tgt.setValue(null); 838 } else { 839 switch (src.getValue()) { 840 case TRANSACTION: 841 tgt.setValue(SystemRestfulInteraction.TRANSACTION); 842 break; 843 case SEARCHSYSTEM: 844 tgt.setValue(SystemRestfulInteraction.SEARCHSYSTEM); 845 break; 846 case HISTORYSYSTEM: 847 tgt.setValue(SystemRestfulInteraction.HISTORYSYSTEM); 848 break; 849 default: 850 tgt.setValue(SystemRestfulInteraction.NULL); 851 break; 852 } 853 } 854 return tgt; 855 } 856 857 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.SystemRestfulInteraction> convertSystemRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.SystemRestfulInteraction> src) throws FHIRException { 858 if (src == null || src.isEmpty()) 859 return null; 860 Enumeration<Conformance.SystemRestfulInteraction> tgt = new Enumeration<>(new Conformance.SystemRestfulInteractionEnumFactory()); 861 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 862 if (src.getValue() == null) { 863 tgt.setValue(null); 864 } else { 865 switch (src.getValue()) { 866 case TRANSACTION: 867 tgt.setValue(Conformance.SystemRestfulInteraction.TRANSACTION); 868 break; 869 case SEARCHSYSTEM: 870 tgt.setValue(Conformance.SystemRestfulInteraction.SEARCHSYSTEM); 871 break; 872 case HISTORYSYSTEM: 873 tgt.setValue(Conformance.SystemRestfulInteraction.HISTORYSYSTEM); 874 break; 875 default: 876 tgt.setValue(Conformance.SystemRestfulInteraction.NULL); 877 break; 878 } 879 } 880 return tgt; 881 } 882 883 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> src) throws FHIRException { 884 if (src == null || src.isEmpty()) 885 return null; 886 Enumeration<Conformance.TypeRestfulInteraction> tgt = new Enumeration<>(new Conformance.TypeRestfulInteractionEnumFactory()); 887 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 888 if (src.getValue() == null) { 889 tgt.setValue(null); 890 } else { 891 switch (src.getValue()) { 892 case READ: 893 tgt.setValue(Conformance.TypeRestfulInteraction.READ); 894 break; 895 case VREAD: 896 tgt.setValue(Conformance.TypeRestfulInteraction.VREAD); 897 break; 898 case UPDATE: 899 tgt.setValue(Conformance.TypeRestfulInteraction.UPDATE); 900 break; 901 case DELETE: 902 tgt.setValue(Conformance.TypeRestfulInteraction.DELETE); 903 break; 904 case HISTORYINSTANCE: 905 tgt.setValue(Conformance.TypeRestfulInteraction.HISTORYINSTANCE); 906 break; 907 case HISTORYTYPE: 908 tgt.setValue(Conformance.TypeRestfulInteraction.HISTORYTYPE); 909 break; 910 case CREATE: 911 tgt.setValue(Conformance.TypeRestfulInteraction.CREATE); 912 break; 913 case SEARCHTYPE: 914 tgt.setValue(Conformance.TypeRestfulInteraction.SEARCHTYPE); 915 break; 916 default: 917 tgt.setValue(Conformance.TypeRestfulInteraction.NULL); 918 break; 919 } 920 } 921 return tgt; 922 } 923 924 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.TypeRestfulInteraction> convertTypeRestfulInteraction(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.TypeRestfulInteraction> src) throws FHIRException { 925 if (src == null || src.isEmpty()) 926 return null; 927 org.hl7.fhir.r5.model.Enumeration<CapabilityStatement.TypeRestfulInteraction> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new CapabilityStatement.TypeRestfulInteractionEnumFactory()); 928 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 929 if (src.getValue() == null) { 930 tgt.setValue(null); 931 } else { 932 switch (src.getValue()) { 933 case READ: 934 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.READ); 935 break; 936 case VREAD: 937 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.VREAD); 938 break; 939 case UPDATE: 940 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.UPDATE); 941 break; 942 case DELETE: 943 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.DELETE); 944 break; 945 case HISTORYINSTANCE: 946 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYINSTANCE); 947 break; 948 case HISTORYTYPE: 949 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.HISTORYTYPE); 950 break; 951 case CREATE: 952 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.CREATE); 953 break; 954 case SEARCHTYPE: 955 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.SEARCHTYPE); 956 break; 957 default: 958 tgt.setValue(CapabilityStatement.TypeRestfulInteraction.NULL); 959 break; 960 } 961 } 962 return tgt; 963 } 964 965 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> convertConformanceEventMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> src) throws FHIRException { 966 if (src == null || src.isEmpty()) return null; 967 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityModeEnumFactory()); 968 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 969 if (src.getValue() == null) { 970 tgt.setValue(null); 971} else { 972 switch(src.getValue()) { 973 case SENDER: 974 tgt.setValue(CapabilityStatement.EventCapabilityMode.SENDER); 975 break; 976 case RECEIVER: 977 tgt.setValue(CapabilityStatement.EventCapabilityMode.RECEIVER); 978 break; 979 default: 980 tgt.setValue(CapabilityStatement.EventCapabilityMode.NULL); 981 break; 982 } 983} 984 return tgt; 985 } 986 987 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> convertConformanceEventMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CapabilityStatement.EventCapabilityMode> src) throws FHIRException { 988 if (src == null || src.isEmpty()) return null; 989 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Conformance.ConformanceEventMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Conformance.ConformanceEventModeEnumFactory()); 990 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 991 if (src.getValue() == null) { 992 tgt.setValue(null); 993} else { 994 switch(src.getValue()) { 995 case SENDER: 996 tgt.setValue(Conformance.ConformanceEventMode.SENDER); 997 break; 998 case RECEIVER: 999 tgt.setValue(Conformance.ConformanceEventMode.RECEIVER); 1000 break; 1001 default: 1002 tgt.setValue(Conformance.ConformanceEventMode.NULL); 1003 break; 1004 } 1005} 1006 return tgt; 1007 } 1008}