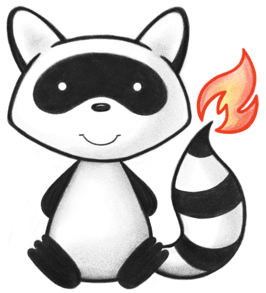
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Attachment10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Instant10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.DiagnosticReport; 012import org.hl7.fhir.r5.model.Enumeration; 013 014public class DiagnosticReport10_50 { 015 016 public static org.hl7.fhir.r5.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.dstu2.model.DiagnosticReport src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.r5.model.DiagnosticReport tgt = new org.hl7.fhir.r5.model.DiagnosticReport(); 020 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 023 if (src.hasStatus()) 024 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 025 if (src.hasCategory()) 026 tgt.addCategory(CodeableConcept10_50.convertCodeableConcept(src.getCategory())); 027 if (src.hasCode()) 028 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 029 if (src.hasSubject()) 030 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 031 if (src.hasEncounter()) 032 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 033 if (src.hasEffective()) 034 tgt.setEffective(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getEffective())); 035 if (src.hasIssuedElement()) 036 tgt.setIssuedElement(Instant10_50.convertInstant(src.getIssuedElement())); 037 for (org.hl7.fhir.dstu2.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_50.convertReference(t)); 038 for (org.hl7.fhir.dstu2.model.Reference t : src.getResult()) tgt.addResult(Reference10_50.convertReference(t)); 039 for (org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent t : src.getImage()) 040 tgt.addMedia(convertDiagnosticReportImageComponent(t)); 041 if (src.hasConclusionElement()) 042 tgt.setConclusionElement(String10_50.convertStringToMarkdown(src.getConclusionElement())); 043 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCodedDiagnosis()) 044 tgt.addConclusionCode(CodeableConcept10_50.convertCodeableConcept(t)); 045 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPresentedForm()) 046 tgt.addPresentedForm(Attachment10_50.convertAttachment(t)); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.dstu2.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r5.model.DiagnosticReport src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu2.model.DiagnosticReport tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport(); 054 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 055 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 056 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 057 if (src.hasStatus()) 058 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 059 if (src.hasCategory()) 060 tgt.setCategory(CodeableConcept10_50.convertCodeableConcept(src.getCategoryFirstRep())); 061 if (src.hasCode()) 062 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 063 if (src.hasSubject()) 064 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 065 if (src.hasEncounter()) 066 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 067 if (src.hasEffective()) 068 tgt.setEffective(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getEffective())); 069 if (src.hasIssuedElement()) 070 tgt.setIssuedElement(Instant10_50.convertInstant(src.getIssuedElement())); 071 for (org.hl7.fhir.r5.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference10_50.convertReference(t)); 072 for (org.hl7.fhir.r5.model.Reference t : src.getResult()) tgt.addResult(Reference10_50.convertReference(t)); 073 for (org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 074 tgt.addImage(convertDiagnosticReportImageComponent(t)); 075 if (src.hasConclusionElement()) 076 tgt.setConclusionElement(String10_50.convertString(src.getConclusionElement())); 077 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getConclusionCode()) 078 tgt.addCodedDiagnosis(CodeableConcept10_50.convertCodeableConcept(t)); 079 for (org.hl7.fhir.r5.model.Attachment t : src.getPresentedForm()) 080 tgt.addPresentedForm(Attachment10_50.convertAttachment(t)); 081 return tgt; 082 } 083 084 public static org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportImageComponent(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent src) throws FHIRException { 085 if (src == null || src.isEmpty()) 086 return null; 087 org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent(); 088 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 089 if (src.hasCommentElement()) 090 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 091 if (src.hasLink()) 092 tgt.setLink(Reference10_50.convertReference(src.getLink())); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent convertDiagnosticReportImageComponent(org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 097 if (src == null || src.isEmpty()) 098 return null; 099 org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent tgt = new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportImageComponent(); 100 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 101 if (src.hasCommentElement()) 102 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 103 if (src.hasLink()) 104 tgt.setLink(Reference10_50.convertReference(src.getLink())); 105 return tgt; 106 } 107 108 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 112 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 113 if (src.getValue() == null) { 114 tgt.setValue(null); 115 } else { 116 switch (src.getValue()) { 117 case REGISTERED: 118 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 119 break; 120 case PARTIAL: 121 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 122 break; 123 case FINAL: 124 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 125 break; 126 case CORRECTED: 127 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 128 break; 129 case APPENDED: 130 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 131 break; 132 case CANCELLED: 133 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 134 break; 135 case ENTEREDINERROR: 136 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 137 break; 138 default: 139 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 140 break; 141 } 142 } 143 return tgt; 144 } 145 146 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 147 if (src == null || src.isEmpty()) 148 return null; 149 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 150 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 151 if (src.getValue() == null) { 152 tgt.setValue(null); 153 } else { 154 switch (src.getValue()) { 155 case REGISTERED: 156 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 157 break; 158 case PARTIAL: 159 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 160 break; 161 case FINAL: 162 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 163 break; 164 case CORRECTED: 165 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 166 break; 167 case APPENDED: 168 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 169 break; 170 case CANCELLED: 171 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 172 break; 173 case ENTEREDINERROR: 174 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 175 break; 176 default: 177 tgt.setValue(org.hl7.fhir.dstu2.model.DiagnosticReport.DiagnosticReportStatus.NULL); 178 break; 179 } 180 } 181 return tgt; 182 } 183}