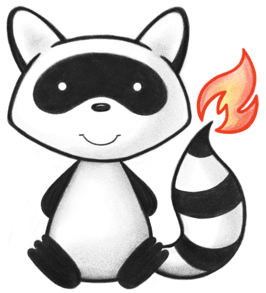
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Attachment10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Period10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.MarkDown10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.dstu2.model.CodeableConcept; 012import org.hl7.fhir.dstu2.model.Enumerations; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableReference; 015import org.hl7.fhir.r5.model.Coding; 016import org.hl7.fhir.r5.model.DocumentReference; 017import org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceAttesterComponent; 018import org.hl7.fhir.r5.model.Enumeration; 019 020public class DocumentReference10_50 { 021 022 static public CodeableConcept convertDocStatus(org.hl7.fhir.r5.model.Enumerations.CompositionStatus docStatus) { 023 CodeableConcept cc = new CodeableConcept(); 024 switch (docStatus) { 025 case AMENDED: 026 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("amended"); 027 break; 028 case ENTEREDINERROR: 029 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("entered-in-error"); 030 break; 031 case FINAL: 032 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("final"); 033 break; 034 case PRELIMINARY: 035 cc.addCoding().setSystem("http://hl7.org/fhir/composition-status").setCode("preliminary"); 036 break; 037 default: 038 return null; 039 } 040 return cc; 041 } 042 043 static public org.hl7.fhir.r5.model.Enumerations.CompositionStatus convertDocStatus(CodeableConcept cc) { 044 if (CodeableConcept10_50.hasConcept(cc, "http://hl7.org/fhir/composition-status", "preliminary")) 045 return org.hl7.fhir.r5.model.Enumerations.CompositionStatus.PRELIMINARY; 046 if (CodeableConcept10_50.hasConcept(cc, "http://hl7.org/fhir/composition-status", "final")) 047 return org.hl7.fhir.r5.model.Enumerations.CompositionStatus.FINAL; 048 if (CodeableConcept10_50.hasConcept(cc, "http://hl7.org/fhir/composition-status", "amended")) 049 return org.hl7.fhir.r5.model.Enumerations.CompositionStatus.AMENDED; 050 if (CodeableConcept10_50.hasConcept(cc, "http://hl7.org/fhir/composition-status", "entered-in-error")) 051 return org.hl7.fhir.r5.model.Enumerations.CompositionStatus.ENTEREDINERROR; 052 return null; 053 } 054 055 public static org.hl7.fhir.dstu2.model.DocumentReference convertDocumentReference(org.hl7.fhir.r5.model.DocumentReference src) throws FHIRException { 056 if (src == null || src.isEmpty()) 057 return null; 058 org.hl7.fhir.dstu2.model.DocumentReference tgt = new org.hl7.fhir.dstu2.model.DocumentReference(); 059 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 060 // if (src.hasMasterIdentifier()) 061 // tgt.setMasterIdentifier(VersionConvertor_10_50.convertIdentifier(src.getMasterIdentifier())); 062 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 063 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 064 if (src.hasSubject()) 065 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 066 if (src.hasType()) 067 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 068 if (src.hasCategory()) 069 tgt.setClass_(CodeableConcept10_50.convertCodeableConcept(src.getCategoryFirstRep())); 070 if (src.hasCustodian()) 071 tgt.setCustodian(Reference10_50.convertReference(src.getCustodian())); 072 for (DocumentReferenceAttesterComponent t : src.getAttester()) { 073 if (t.getMode().hasCoding("http://hl7.org/fhir/composition-attestation-mode", "official")) 074 tgt.setAuthenticator(Reference10_50.convertReference(t.getParty())); 075 } 076 if (src.hasDate()) 077 tgt.setCreated(src.getDate()); 078 if (src.hasStatus()) 079 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 080 if (src.hasDocStatus()) 081 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 082 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 083 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 084 if (src.hasDescriptionElement()) 085 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 086 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSecurityLabel()) 087 tgt.addSecurityLabel(CodeableConcept10_50.convertCodeableConcept(t)); 088 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 089 tgt.addContent(convertDocumentReferenceContentComponent(t)); 090 convertDocumentReferenceContextComponent(tgt.getContext(), src); 091 return tgt; 092 } 093 094 public static org.hl7.fhir.r5.model.DocumentReference convertDocumentReference(org.hl7.fhir.dstu2.model.DocumentReference src) throws FHIRException { 095 if (src == null || src.isEmpty()) 096 return null; 097 org.hl7.fhir.r5.model.DocumentReference tgt = new org.hl7.fhir.r5.model.DocumentReference(); 098 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 099 if (src.hasMasterIdentifier()) 100 tgt.addIdentifier(Identifier10_50.convertIdentifier(src.getMasterIdentifier())); 101 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 102 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 103 if (src.hasSubject()) 104 tgt.setSubject(Reference10_50.convertReference(src.getSubject())); 105 if (src.hasType()) 106 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 107 if (src.hasClass_()) 108 tgt.addCategory(CodeableConcept10_50.convertCodeableConcept(src.getClass_())); 109 if (src.hasCustodian()) 110 tgt.setCustodian(Reference10_50.convertReference(src.getCustodian())); 111 if (src.hasAuthenticator()) 112 tgt.addAttester().setMode(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(new Coding("http://hl7.org/fhir/composition-attestation-mode","official", "Official"))) 113 .setParty(Reference10_50.convertReference(src.getAuthenticator())); 114 if (src.hasCreated()) 115 tgt.setDate(src.getCreated()); 116 if (src.hasStatus()) 117 tgt.setStatusElement(convertDocumentReferenceStatus(src.getStatusElement())); 118 if (src.hasDocStatus()) 119 tgt.setDocStatus(convertDocStatus(src.getDocStatus())); 120 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 121 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 122 if (src.hasDescriptionElement()) 123 tgt.setDescriptionElement(MarkDown10_50.convertStringToMarkdown(src.getDescriptionElement())); 124 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getSecurityLabel()) 125 tgt.addSecurityLabel(CodeableConcept10_50.convertCodeableConcept(t)); 126 for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 127 tgt.addContent(convertDocumentReferenceContentComponent(t)); 128 convertDocumentReferenceContextComponent(src.getContext(), tgt); 129 return tgt; 130 } 131 132 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent(); 136 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 137 if (src.hasAttachment()) 138 tgt.setAttachment(Attachment10_50.convertAttachment(src.getAttachment())); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 143 if (src == null || src.isEmpty()) 144 return null; 145 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent(); 146 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 147 if (src.hasAttachment()) 148 tgt.setAttachment(Attachment10_50.convertAttachment(src.getAttachment())); 149 return tgt; 150 } 151 152 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r5.model.DocumentReference src, org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent tgt) throws FHIRException { 153 if (src.hasContext()) 154 tgt.setEncounter(Reference10_50.convertReference(src.getContextFirstRep())); 155 for (CodeableReference t : src.getEvent()) 156 if (t.hasConcept()) 157 tgt.addEvent(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 158 if (src.hasPeriod()) 159 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 160 if (src.hasFacilityType()) 161 tgt.setFacilityType(CodeableConcept10_50.convertCodeableConcept(src.getFacilityType())); 162 if (src.hasPracticeSetting()) 163 tgt.setPracticeSetting(CodeableConcept10_50.convertCodeableConcept(src.getPracticeSetting())); 164// if (src.hasSourcePatientInfo()) 165// tgt.setSourcePatientInfo(Reference10_50.convertReference(src.getSourcePatientInfo())); 166// for (org.hl7.fhir.r5.model.Reference t : src.getRelated()) 167// tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 168 } 169 170 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextComponent src, org.hl7.fhir.r5.model.DocumentReference tgt) throws FHIRException { 171 if (src.hasEncounter()) 172 tgt.addContext(Reference10_50.convertReference(src.getEncounter())); 173 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getEvent()) 174 tgt.addEvent(new CodeableReference().setConcept(CodeableConcept10_50.convertCodeableConcept(t))); 175 if (src.hasPeriod()) 176 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 177 if (src.hasFacilityType()) 178 tgt.setFacilityType(CodeableConcept10_50.convertCodeableConcept(src.getFacilityType())); 179 if (src.hasPracticeSetting()) 180 tgt.setPracticeSetting(CodeableConcept10_50.convertCodeableConcept(src.getPracticeSetting())); 181// if (src.hasSourcePatientInfo()) 182// tgt.setSourcePatientInfo(Reference10_50.convertReference(src.getSourcePatientInfo())); 183// for (org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent t : src.getRelated()) 184// tgt.addRelated(convertDocumentReferenceContextRelatedComponent(t)); 185 } 186 187 public static org.hl7.fhir.r5.model.Reference convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent src) throws FHIRException { 188 if (src == null || src.isEmpty()) 189 return null; 190 org.hl7.fhir.r5.model.Reference tgt = Reference10_50.convertReference(src.getRef()); 191 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 192 if (src.hasIdentifier()) 193 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 194 return tgt; 195 } 196 197 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent convertDocumentReferenceContextRelatedComponent(org.hl7.fhir.r5.model.Reference src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceContextRelatedComponent(); 201 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 202 if (src.hasIdentifier()) 203 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 204 tgt.setRef(Reference10_50.convertReference(src)); 205 return tgt; 206 } 207 208 public static org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 209 if (src == null || src.isEmpty()) 210 return null; 211 org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent(); 212 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 213 if (src.hasCode()) 214 tgt.setCodeElement(convertDocumentRelationshipType(src.getCode())); 215 if (src.hasTarget()) 216 tgt.setTarget(Reference10_50.convertReference(src.getTarget())); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.dstu2.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent(); 224 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 225 if (src.hasCode()) 226 tgt.setCode(convertDocumentRelationshipType(src.getCodeElement())); 227 if (src.hasTarget()) 228 tgt.setTarget(Reference10_50.convertReference(src.getTarget())); 229 return tgt; 230 } 231 232 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 233 if (src == null || src.isEmpty()) 234 return null; 235 Enumeration<DocumentReference.DocumentReferenceStatus> tgt = new Enumeration<>(new DocumentReference.DocumentReferenceStatusEnumFactory()); 236 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 237 if (src.getValue() == null) { 238 tgt.setValue(null); 239 } else { 240 switch (src.getValue()) { 241 case CURRENT: 242 tgt.setValue(DocumentReference.DocumentReferenceStatus.CURRENT); 243 break; 244 case SUPERSEDED: 245 tgt.setValue(DocumentReference.DocumentReferenceStatus.SUPERSEDED); 246 break; 247 case ENTEREDINERROR: 248 tgt.setValue(DocumentReference.DocumentReferenceStatus.ENTEREDINERROR); 249 break; 250 default: 251 tgt.setValue(DocumentReference.DocumentReferenceStatus.NULL); 252 break; 253 } 254 } 255 return tgt; 256 } 257 258 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> src) throws FHIRException { 259 if (src == null || src.isEmpty()) 260 return null; 261 org.hl7.fhir.dstu2.model.Enumeration<Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new Enumerations.DocumentReferenceStatusEnumFactory()); 262 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 263 if (src.getValue() == null) { 264 tgt.setValue(null); 265 } else { 266 switch (src.getValue()) { 267 case CURRENT: 268 tgt.setValue(Enumerations.DocumentReferenceStatus.CURRENT); 269 break; 270 case SUPERSEDED: 271 tgt.setValue(Enumerations.DocumentReferenceStatus.SUPERSEDED); 272 break; 273 case ENTEREDINERROR: 274 tgt.setValue(Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 275 break; 276 default: 277 tgt.setValue(Enumerations.DocumentReferenceStatus.NULL); 278 break; 279 } 280 } 281 return tgt; 282 } 283 284 static public org.hl7.fhir.r5.model.CodeableConcept convertDocumentRelationshipType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 285 if (src == null || src.isEmpty()) 286 return null; 287 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 288 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 289 if (src.getValue() == null) { 290 // Add nothing 291 } else { 292 switch (src.getValue()) { 293 case REPLACES: 294 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("replaces"); 295 break; 296 case TRANSFORMS: 297 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("transforms"); 298 break; 299 case SIGNS: 300 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("signs"); 301 break; 302 case APPENDS: 303 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("appends"); 304 break; 305 default: 306 break; 307 } 308 } 309 310 return tgt; 311 } 312 313 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 314 if (src == null || src.isEmpty()) 315 return null; 316 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 317 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 318 switch (src.getCode("http://hl7.org/fhir/document-relationship-type")) { 319 case "replaces": 320 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.REPLACES); 321 break; 322 case "transforms": 323 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 324 break; 325 case "signs": 326 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.SIGNS); 327 break; 328 case "appends": 329 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.APPENDS); 330 break; 331 default: 332 tgt.setValue(org.hl7.fhir.dstu2.model.DocumentReference.DocumentRelationshipType.NULL); 333 break; 334 } 335 336 return tgt; 337 } 338}