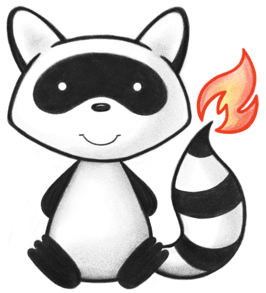
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.exceptions.FHIRException; 005import org.hl7.fhir.r5.model.Enumerations; 006 007public class Enumerations10_50 { 008 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> src) throws FHIRException { 009 if (src == null || src.isEmpty()) return null; 010 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.BindingStrengthEnumFactory()); 011 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 012 if (src.getValue() == null) { 013 tgt.setValue(null); 014} else { 015 switch(src.getValue()) { 016 case REQUIRED: 017 tgt.setValue(Enumerations.BindingStrength.REQUIRED); 018 break; 019 case EXTENSIBLE: 020 tgt.setValue(Enumerations.BindingStrength.EXTENSIBLE); 021 break; 022 case PREFERRED: 023 tgt.setValue(Enumerations.BindingStrength.PREFERRED); 024 break; 025 case EXAMPLE: 026 tgt.setValue(Enumerations.BindingStrength.EXAMPLE); 027 break; 028 default: 029 tgt.setValue(Enumerations.BindingStrength.NULL); 030 break; 031 } 032} 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> src) throws FHIRException { 037 if (src == null || src.isEmpty()) return null; 038 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.BindingStrengthEnumFactory()); 039 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 040 if (src.getValue() == null) { 041 tgt.setValue(null); 042} else { 043 switch(src.getValue()) { 044 case REQUIRED: 045 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.REQUIRED); 046 break; 047 case EXTENSIBLE: 048 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXTENSIBLE); 049 break; 050 case PREFERRED: 051 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.PREFERRED); 052 break; 053 case EXAMPLE: 054 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.EXAMPLE); 055 break; 056 default: 057 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.BindingStrength.NULL); 058 break; 059 } 060} 061 return tgt; 062 } 063 064 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> src) throws FHIRException { 065 if (src == null || src.isEmpty()) return null; 066 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.AdministrativeGenderEnumFactory()); 067 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(null); 070} else { 071 switch(src.getValue()) { 072 case MALE: 073 tgt.setValue(Enumerations.AdministrativeGender.MALE); 074 break; 075 case FEMALE: 076 tgt.setValue(Enumerations.AdministrativeGender.FEMALE); 077 break; 078 case OTHER: 079 tgt.setValue(Enumerations.AdministrativeGender.OTHER); 080 break; 081 case UNKNOWN: 082 tgt.setValue(Enumerations.AdministrativeGender.UNKNOWN); 083 break; 084 default: 085 tgt.setValue(Enumerations.AdministrativeGender.NULL); 086 break; 087 } 088} 089 return tgt; 090 } 091 092 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> src) throws FHIRException { 093 if (src == null || src.isEmpty()) return null; 094 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGenderEnumFactory()); 095 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 096 if (src.getValue() == null) { 097 tgt.setValue(null); 098} else { 099 switch(src.getValue()) { 100 case MALE: 101 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.MALE); 102 break; 103 case FEMALE: 104 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.FEMALE); 105 break; 106 case OTHER: 107 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.OTHER); 108 break; 109 case UNKNOWN: 110 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.UNKNOWN); 111 break; 112 default: 113 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.AdministrativeGender.NULL); 114 break; 115 } 116} 117 return tgt; 118 } 119 120 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> src) throws FHIRException { 121 if (src == null || src.isEmpty()) return null; 122 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.SearchParamTypeEnumFactory()); 123 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 124 if (src.getValue() == null) { 125 tgt.setValue(null); 126} else { 127 switch(src.getValue()) { 128 case NUMBER: 129 tgt.setValue(Enumerations.SearchParamType.NUMBER); 130 break; 131 case DATE: 132 tgt.setValue(Enumerations.SearchParamType.DATE); 133 break; 134 case STRING: 135 tgt.setValue(Enumerations.SearchParamType.STRING); 136 break; 137 case TOKEN: 138 tgt.setValue(Enumerations.SearchParamType.TOKEN); 139 break; 140 case REFERENCE: 141 tgt.setValue(Enumerations.SearchParamType.REFERENCE); 142 break; 143 case COMPOSITE: 144 tgt.setValue(Enumerations.SearchParamType.COMPOSITE); 145 break; 146 case QUANTITY: 147 tgt.setValue(Enumerations.SearchParamType.QUANTITY); 148 break; 149 case URI: 150 tgt.setValue(Enumerations.SearchParamType.URI); 151 break; 152 default: 153 tgt.setValue(Enumerations.SearchParamType.NULL); 154 break; 155 } 156} 157 return tgt; 158 } 159 160 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> src) throws FHIRException { 161 if (src == null || src.isEmpty()) return null; 162 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.SearchParamTypeEnumFactory()); 163 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 164 if (src.getValue() == null) { 165 tgt.setValue(null); 166} else { 167 switch(src.getValue()) { 168 case NUMBER: 169 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.NUMBER); 170 break; 171 case DATE: 172 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.DATE); 173 break; 174 case STRING: 175 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.STRING); 176 break; 177 case TOKEN: 178 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.TOKEN); 179 break; 180 case REFERENCE: 181 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.REFERENCE); 182 break; 183 case COMPOSITE: 184 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.COMPOSITE); 185 break; 186 case QUANTITY: 187 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.QUANTITY); 188 break; 189 case URI: 190 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.URI); 191 break; 192 default: 193 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.SearchParamType.NULL); 194 break; 195 } 196} 197 return tgt; 198 } 199 200 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> convertConformanceResourceStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> src) throws FHIRException { 201 if (src == null || src.isEmpty()) return null; 202 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.PublicationStatusEnumFactory()); 203 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 204 if (src.getValue() == null) { 205 tgt.setValue(null); 206} else { 207 switch(src.getValue()) { 208 case DRAFT: 209 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 210 break; 211 case ACTIVE: 212 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 213 break; 214 case RETIRED: 215 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 216 break; 217 default: 218 tgt.setValue(Enumerations.PublicationStatus.NULL); 219 break; 220 } 221} 222 return tgt; 223 } 224 225 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> convertConformanceResourceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> src) throws FHIRException { 226 if (src == null || src.isEmpty()) return null; 227 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatusEnumFactory()); 228 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 229 if (src.getValue() == null) { 230 tgt.setValue(null); 231} else { 232 switch(src.getValue()) { 233 case DRAFT: 234 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.DRAFT); 235 break; 236 case ACTIVE: 237 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.ACTIVE); 238 break; 239 case RETIRED: 240 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.RETIRED); 241 break; 242 default: 243 tgt.setValue(org.hl7.fhir.dstu2.model.Enumerations.ConformanceResourceStatus.NULL); 244 break; 245 } 246} 247 return tgt; 248 } 249}