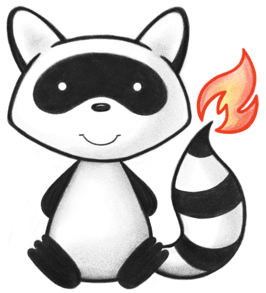
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Attachment10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.dstu2.model.Enumeration; 012import org.hl7.fhir.dstu2.model.HealthcareService; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.Enumerations; 015import org.hl7.fhir.r5.model.ExtendedContactDetail; 016 017public class HealthcareService10_50 { 018 019 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 Enumeration<HealthcareService.DaysOfWeek> tgt = new Enumeration<>(new HealthcareService.DaysOfWeekEnumFactory()); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 024 if (src.getValue() == null) { 025 tgt.setValue(null); 026 } else { 027 switch (src.getValue()) { 028 case MON: 029 tgt.setValue(HealthcareService.DaysOfWeek.MON); 030 break; 031 case TUE: 032 tgt.setValue(HealthcareService.DaysOfWeek.TUE); 033 break; 034 case WED: 035 tgt.setValue(HealthcareService.DaysOfWeek.WED); 036 break; 037 case THU: 038 tgt.setValue(HealthcareService.DaysOfWeek.THU); 039 break; 040 case FRI: 041 tgt.setValue(HealthcareService.DaysOfWeek.FRI); 042 break; 043 case SAT: 044 tgt.setValue(HealthcareService.DaysOfWeek.SAT); 045 break; 046 case SUN: 047 tgt.setValue(HealthcareService.DaysOfWeek.SUN); 048 break; 049 default: 050 tgt.setValue(HealthcareService.DaysOfWeek.NULL); 051 break; 052 } 053 } 054 return tgt; 055 } 056 057 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.HealthcareService.DaysOfWeek> src) throws FHIRException { 058 if (src == null || src.isEmpty()) 059 return null; 060 org.hl7.fhir.r5.model.Enumeration<Enumerations.DaysOfWeek> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.DaysOfWeekEnumFactory()); 061 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 062 if (src.getValue() == null) { 063 tgt.setValue(null); 064 } else { 065 switch (src.getValue()) { 066 case MON: 067 tgt.setValue(Enumerations.DaysOfWeek.MON); 068 break; 069 case TUE: 070 tgt.setValue(Enumerations.DaysOfWeek.TUE); 071 break; 072 case WED: 073 tgt.setValue(Enumerations.DaysOfWeek.WED); 074 break; 075 case THU: 076 tgt.setValue(Enumerations.DaysOfWeek.THU); 077 break; 078 case FRI: 079 tgt.setValue(Enumerations.DaysOfWeek.FRI); 080 break; 081 case SAT: 082 tgt.setValue(Enumerations.DaysOfWeek.SAT); 083 break; 084 case SUN: 085 tgt.setValue(Enumerations.DaysOfWeek.SUN); 086 break; 087 default: 088 tgt.setValue(Enumerations.DaysOfWeek.NULL); 089 break; 090 } 091 } 092 return tgt; 093 } 094 095 public static org.hl7.fhir.dstu2.model.HealthcareService convertHealthcareService(org.hl7.fhir.r5.model.HealthcareService src) throws FHIRException { 096 if (src == null || src.isEmpty()) 097 return null; 098 org.hl7.fhir.dstu2.model.HealthcareService tgt = new org.hl7.fhir.dstu2.model.HealthcareService(); 099 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 100 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 101 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 102 if (src.hasProvidedBy()) 103 tgt.setProvidedBy(Reference10_50.convertReference(src.getProvidedBy())); 104 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) { 105 if (!tgt.hasServiceType()) 106 tgt.addServiceType(); 107 tgt.getServiceType().get(0).addSpecialty(CodeableConcept10_50.convertCodeableConcept(t)); 108 } 109 for (org.hl7.fhir.r5.model.Reference t : src.getLocation()) tgt.setLocation(Reference10_50.convertReference(t)); 110 if (src.hasCommentElement()) 111 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 112 if (src.hasExtraDetails()) 113 tgt.setExtraDetails(src.getExtraDetails()); 114 if (src.hasPhoto()) 115 tgt.setPhoto(Attachment10_50.convertAttachment(src.getPhoto())); 116 for (ExtendedContactDetail t1 : src.getContact()) 117 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 118 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 119 for (org.hl7.fhir.r5.model.Reference t : src.getCoverageArea()) 120 tgt.addCoverageArea(Reference10_50.convertReference(t)); 121 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getServiceProvisionCode()) 122 tgt.addServiceProvisionCode(CodeableConcept10_50.convertCodeableConcept(t)); 123 tgt.setEligibility(CodeableConcept10_50.convertCodeableConcept(src.getEligibilityFirstRep().getCode())); 124 if (src.hasCommentElement()) 125 tgt.setEligibilityNoteElement(String10_50.convertString(src.getCommentElement())); 126 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getProgram()) 127 if (t.hasText()) 128 tgt.addProgramName(t.getText()); 129 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCharacteristic()) 130 tgt.addCharacteristic(CodeableConcept10_50.convertCodeableConcept(t)); 131 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getReferralMethod()) 132 tgt.addReferralMethod(CodeableConcept10_50.convertCodeableConcept(t)); 133 if (src.hasAppointmentRequiredElement()) 134 tgt.setAppointmentRequiredElement(Boolean10_50.convertBoolean(src.getAppointmentRequiredElement())); 135// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 136// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 137// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 138// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 139// if (src.hasAvailabilityExceptionsElement()) 140// tgt.setAvailabilityExceptionsElement(String10_50.convertString(src.getAvailabilityExceptionsElement())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.r5.model.HealthcareService convertHealthcareService(org.hl7.fhir.dstu2.model.HealthcareService src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.r5.model.HealthcareService tgt = new org.hl7.fhir.r5.model.HealthcareService(); 148 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 149 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 150 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 151 if (src.hasProvidedBy()) 152 tgt.setProvidedBy(Reference10_50.convertReference(src.getProvidedBy())); 153 for (org.hl7.fhir.dstu2.model.HealthcareService.ServiceTypeComponent t : src.getServiceType()) { 154 for (org.hl7.fhir.dstu2.model.CodeableConcept tj : t.getSpecialty()) 155 tgt.addSpecialty(CodeableConcept10_50.convertCodeableConcept(tj)); 156 } 157 if (src.hasLocation()) 158 tgt.addLocation(Reference10_50.convertReference(src.getLocation())); 159 if (src.hasCommentElement()) 160 tgt.setCommentElement(String10_50.convertStringToMarkdown(src.getCommentElement())); 161 if (src.hasExtraDetails()) 162 tgt.setExtraDetails(src.getExtraDetails()); 163 if (src.hasPhoto()) 164 tgt.setPhoto(Attachment10_50.convertAttachment(src.getPhoto())); 165 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 166 tgt.getContactFirstRep().addTelecom(ContactPoint10_50.convertContactPoint(t)); 167 for (org.hl7.fhir.dstu2.model.Reference t : src.getCoverageArea()) 168 tgt.addCoverageArea(Reference10_50.convertReference(t)); 169 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getServiceProvisionCode()) 170 tgt.addServiceProvisionCode(CodeableConcept10_50.convertCodeableConcept(t)); 171 if (src.hasEligibility()) 172 tgt.getEligibilityFirstRep().setCode(CodeableConcept10_50.convertCodeableConcept(src.getEligibility())); 173 if (src.hasEligibilityNote()) 174 tgt.getEligibilityFirstRep().setComment(src.getEligibilityNote()); 175 for (org.hl7.fhir.dstu2.model.StringType t : src.getProgramName()) tgt.addProgram().setText(t.getValue()); 176 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCharacteristic()) 177 tgt.addCharacteristic(CodeableConcept10_50.convertCodeableConcept(t)); 178 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getReferralMethod()) 179 tgt.addReferralMethod(CodeableConcept10_50.convertCodeableConcept(t)); 180 if (src.hasAppointmentRequiredElement()) 181 tgt.setAppointmentRequiredElement(Boolean10_50.convertBoolean(src.getAppointmentRequiredElement())); 182// for (org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 183// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 184// for (org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 185// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 186// if (src.hasAvailabilityExceptionsElement()) 187// tgt.setAvailabilityExceptionsElement(String10_50.convertString(src.getAvailabilityExceptionsElement())); 188 return tgt; 189 } 190 191// public static org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 192// if (src == null || src.isEmpty()) 193// return null; 194// org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 195// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 196// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 197// .map(HealthcareService10_50::convertDaysOfWeek) 198// .collect(Collectors.toList())); 199// if (src.hasAllDayElement()) 200// tgt.setAllDayElement(Boolean10_50.convertBoolean(src.getAllDayElement())); 201// if (src.hasAvailableStartTimeElement()) 202// tgt.setAvailableStartTimeElement(Time10_50.convertTime(src.getAvailableStartTimeElement())); 203// if (src.hasAvailableEndTimeElement()) 204// tgt.setAvailableEndTimeElement(Time10_50.convertTime(src.getAvailableEndTimeElement())); 205// return tgt; 206// } 207// 208// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 209// if (src == null || src.isEmpty()) 210// return null; 211// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 212// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 213// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 214// .map(HealthcareService10_50::convertDaysOfWeek) 215// .collect(Collectors.toList())); 216// if (src.hasAllDayElement()) 217// tgt.setAllDayElement(Boolean10_50.convertBoolean(src.getAllDayElement())); 218// if (src.hasAvailableStartTimeElement()) 219// tgt.setAvailableStartTimeElement(Time10_50.convertTime(src.getAvailableStartTimeElement())); 220// if (src.hasAvailableEndTimeElement()) 221// tgt.setAvailableEndTimeElement(Time10_50.convertTime(src.getAvailableEndTimeElement())); 222// return tgt; 223// } 224// 225// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 226// if (src == null || src.isEmpty()) 227// return null; 228// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 229// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 230// if (src.hasDescriptionElement()) 231// tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 232// if (src.hasDuring()) 233// tgt.setDuring(Period10_50.convertPeriod(src.getDuring())); 234// return tgt; 235// } 236// 237// public static org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 238// if (src == null || src.isEmpty()) 239// return null; 240// org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.dstu2.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 241// ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 242// if (src.hasDescriptionElement()) 243// tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 244// if (src.hasDuring()) 245// tgt.setDuring(Period10_50.convertPeriod(src.getDuring())); 246// return tgt; 247// } 248}