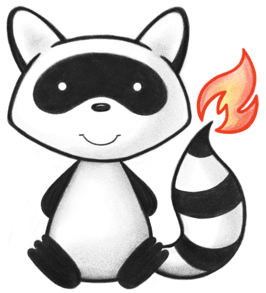
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Extension10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.exceptions.FHIRException; 012 013public class List10_50 { 014 015 public static org.hl7.fhir.dstu2.model.List_ convertList(org.hl7.fhir.r5.model.ListResource src) throws FHIRException { 016 if (src == null || src.isEmpty()) 017 return null; 018 org.hl7.fhir.dstu2.model.List_ tgt = new org.hl7.fhir.dstu2.model.List_(); 019 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 020 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 021 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 022 if (src.hasTitleElement()) 023 tgt.setTitleElement(String10_50.convertString(src.getTitleElement())); 024 if (src.hasCode()) 025 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 026 if (src.hasSubject()) 027 tgt.setSubject(Reference10_50.convertReference(src.getSubjectFirstRep())); 028 if (src.hasSource()) 029 tgt.setSource(Reference10_50.convertReference(src.getSource())); 030 if (src.hasEncounter()) 031 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 032 if (src.hasStatus()) 033 tgt.setStatusElement(convertListStatus(src.getStatusElement())); 034 if (src.hasDate()) 035 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 036 if (src.hasOrderedBy()) 037 tgt.setOrderedBy(CodeableConcept10_50.convertCodeableConcept(src.getOrderedBy())); 038 if (src.hasMode()) 039 tgt.setModeElement(convertListMode(src.getModeElement())); 040 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.setNote(t.getText()); 041 for (org.hl7.fhir.r5.model.ListResource.ListResourceEntryComponent t : src.getEntry()) 042 tgt.addEntry(convertListEntry(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.r5.model.ListResource convertList(org.hl7.fhir.dstu2.model.List_ src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.r5.model.ListResource tgt = new org.hl7.fhir.r5.model.ListResource(); 050 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 053 if (src.hasTitleElement()) 054 tgt.setTitleElement(String10_50.convertString(src.getTitleElement())); 055 if (src.hasCode()) 056 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 057 if (src.hasSubject()) 058 tgt.addSubject(Reference10_50.convertReference(src.getSubject())); 059 if (src.hasSource()) 060 tgt.setSource(Reference10_50.convertReference(src.getSource())); 061 if (src.hasEncounter()) 062 tgt.setEncounter(Reference10_50.convertReference(src.getEncounter())); 063 if (src.hasStatus()) 064 tgt.setStatusElement(convertListStatus(src.getStatusElement())); 065 if (src.hasDate()) 066 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 067 if (src.hasOrderedBy()) 068 tgt.setOrderedBy(CodeableConcept10_50.convertCodeableConcept(src.getOrderedBy())); 069 if (src.hasMode()) 070 tgt.setModeElement(convertListMode(src.getModeElement())); 071 if (src.hasNote()) 072 tgt.addNote(new org.hl7.fhir.r5.model.Annotation().setText(src.getNote())); 073 for (org.hl7.fhir.dstu2.model.List_.ListEntryComponent t : src.getEntry()) tgt.addEntry(convertListEntry(t)); 074 return tgt; 075 } 076 077 public static org.hl7.fhir.dstu2.model.List_.ListEntryComponent convertListEntry(org.hl7.fhir.r5.model.ListResource.ListResourceEntryComponent src) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 org.hl7.fhir.dstu2.model.List_.ListEntryComponent tgt = new org.hl7.fhir.dstu2.model.List_.ListEntryComponent(); 081 copyBackboneElement(src, tgt); 082 if (src.hasFlag()) 083 tgt.setFlag(CodeableConcept10_50.convertCodeableConcept(src.getFlag())); 084 if (src.hasDeletedElement()) 085 tgt.setDeletedElement(Boolean10_50.convertBoolean(src.getDeletedElement())); 086 if (src.hasDate()) 087 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 088 if (src.hasItem()) 089 tgt.setItem(Reference10_50.convertReference(src.getItem())); 090 return tgt; 091 } 092 093 public static org.hl7.fhir.r5.model.ListResource.ListResourceEntryComponent convertListEntry(org.hl7.fhir.dstu2.model.List_.ListEntryComponent src) throws FHIRException { 094 if (src == null || src.isEmpty()) 095 return null; 096 org.hl7.fhir.r5.model.ListResource.ListResourceEntryComponent tgt = new org.hl7.fhir.r5.model.ListResource.ListResourceEntryComponent(); 097 copyBackboneElement(src, tgt); 098 if (src.hasFlag()) 099 tgt.setFlag(CodeableConcept10_50.convertCodeableConcept(src.getFlag())); 100 if (src.hasDeletedElement()) 101 tgt.setDeletedElement(Boolean10_50.convertBoolean(src.getDeletedElement())); 102 if (src.hasDate()) 103 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 104 if (src.hasItem()) 105 tgt.setItem(Reference10_50.convertReference(src.getItem())); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> convertListMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListMode> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ListModeEnumFactory()); 113 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 114 switch (src.getValue()) { 115 case WORKING: 116 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.WORKING); 117 break; 118 case SNAPSHOT: 119 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.SNAPSHOT); 120 break; 121 case CHANGES: 122 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.CHANGES); 123 break; 124 default: 125 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.NULL); 126 break; 127 } 128 return tgt; 129 } 130 131 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListMode> convertListMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> src) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.List_.ListModeEnumFactory()); 135 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 136 switch (src.getValue()) { 137 case WORKING: 138 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListMode.WORKING); 139 break; 140 case SNAPSHOT: 141 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListMode.SNAPSHOT); 142 break; 143 case CHANGES: 144 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListMode.CHANGES); 145 break; 146 default: 147 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListMode.NULL); 148 break; 149 } 150 return tgt; 151 } 152 153 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ListResource.ListStatus> convertListStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListStatus> src) throws FHIRException { 154 if (src == null || src.isEmpty()) 155 return null; 156 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ListResource.ListStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ListResource.ListStatusEnumFactory()); 157 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 158 switch (src.getValue()) { 159 case CURRENT: 160 tgt.setValue(org.hl7.fhir.r5.model.ListResource.ListStatus.CURRENT); 161 break; 162 case RETIRED: 163 tgt.setValue(org.hl7.fhir.r5.model.ListResource.ListStatus.RETIRED); 164 break; 165 case ENTEREDINERROR: 166 tgt.setValue(org.hl7.fhir.r5.model.ListResource.ListStatus.ENTEREDINERROR); 167 break; 168 default: 169 tgt.setValue(org.hl7.fhir.r5.model.ListResource.ListStatus.NULL); 170 break; 171 } 172 return tgt; 173 } 174 175 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListStatus> convertListStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ListResource.ListStatus> src) throws FHIRException { 176 if (src == null || src.isEmpty()) 177 return null; 178 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.List_.ListStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.List_.ListStatusEnumFactory()); 179 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 180 switch (src.getValue()) { 181 case CURRENT: 182 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListStatus.CURRENT); 183 break; 184 case RETIRED: 185 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListStatus.RETIRED); 186 break; 187 case ENTEREDINERROR: 188 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListStatus.ENTEREDINERROR); 189 break; 190 default: 191 tgt.setValue(org.hl7.fhir.dstu2.model.List_.ListStatus.NULL); 192 break; 193 } 194 return tgt; 195 } 196 197 public static void copyBackboneElement(org.hl7.fhir.dstu2.model.BackboneElement src, org.hl7.fhir.r5.model.BackboneElement tgt) throws FHIRException { 198 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 199 for (org.hl7.fhir.dstu2.model.Extension e : src.getModifierExtension()) { 200 tgt.addModifierExtension(Extension10_50.convertExtension(e)); 201 } 202 } 203 204 public static void copyBackboneElement(org.hl7.fhir.r5.model.BackboneElement src, org.hl7.fhir.dstu2.model.BackboneElement tgt) throws FHIRException { 205 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 206 for (org.hl7.fhir.r5.model.Extension e : src.getModifierExtension()) { 207 tgt.addModifierExtension(Extension10_50.convertExtension(e)); 208 } 209 } 210}