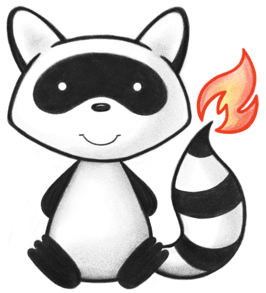
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Address10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Decimal10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.ContactPoint; 013import org.hl7.fhir.r5.model.Enumeration; 014import org.hl7.fhir.r5.model.ExtendedContactDetail; 015import org.hl7.fhir.r5.model.Location; 016 017public class Location10_50 { 018 019 public static org.hl7.fhir.dstu2.model.Location convertLocation(org.hl7.fhir.r5.model.Location src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.dstu2.model.Location tgt = new org.hl7.fhir.dstu2.model.Location(); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 028 if (src.hasNameElement()) 029 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 030 if (src.hasDescriptionElement()) 031 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 032 if (src.hasMode()) 033 tgt.setModeElement(convertLocationMode(src.getModeElement())); 034 if (src.hasType()) 035 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getTypeFirstRep())); 036 for (ExtendedContactDetail t1 : src.getContact()) 037 for (ContactPoint t : t1.getTelecom()) 038 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 039 if (src.hasAddress()) 040 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 041 if (src.hasForm()) 042 tgt.setPhysicalType(CodeableConcept10_50.convertCodeableConcept(src.getForm())); 043 if (src.hasPosition()) 044 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 045 if (src.hasManagingOrganization()) 046 tgt.setManagingOrganization(Reference10_50.convertReference(src.getManagingOrganization())); 047 if (src.hasPartOf()) 048 tgt.setPartOf(Reference10_50.convertReference(src.getPartOf())); 049 return tgt; 050 } 051 052 public static org.hl7.fhir.r5.model.Location convertLocation(org.hl7.fhir.dstu2.model.Location src) throws FHIRException { 053 if (src == null || src.isEmpty()) 054 return null; 055 org.hl7.fhir.r5.model.Location tgt = new org.hl7.fhir.r5.model.Location(); 056 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 057 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 059 if (src.hasStatus()) 060 tgt.setStatusElement(convertLocationStatus(src.getStatusElement())); 061 if (src.hasNameElement()) 062 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 063 if (src.hasDescriptionElement()) 064 tgt.setDescriptionElement(String10_50.convertStringToMarkdown(src.getDescriptionElement())); 065 if (src.hasMode()) 066 tgt.setModeElement(convertLocationMode(src.getModeElement())); 067 if (src.hasType()) 068 tgt.addType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 069 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 070 tgt.getContactFirstRep().addTelecom(ContactPoint10_50.convertContactPoint(t)); 071 if (src.hasAddress()) 072 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 073 if (src.hasPhysicalType()) 074 tgt.setForm(CodeableConcept10_50.convertCodeableConcept(src.getPhysicalType())); 075 if (src.hasPosition()) 076 tgt.setPosition(convertLocationPositionComponent(src.getPosition())); 077 if (src.hasManagingOrganization()) 078 tgt.setManagingOrganization(Reference10_50.convertReference(src.getManagingOrganization())); 079 if (src.hasPartOf()) 080 tgt.setPartOf(Reference10_50.convertReference(src.getPartOf())); 081 return tgt; 082 } 083 084 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationMode> src) throws FHIRException { 085 if (src == null || src.isEmpty()) 086 return null; 087 Enumeration<Location.LocationMode> tgt = new Enumeration<>(new Location.LocationModeEnumFactory()); 088 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 089 if (src.getValue() == null) { 090 tgt.setValue(null); 091 } else { 092 switch (src.getValue()) { 093 case INSTANCE: 094 tgt.setValue(Location.LocationMode.INSTANCE); 095 break; 096 case KIND: 097 tgt.setValue(Location.LocationMode.KIND); 098 break; 099 default: 100 tgt.setValue(Location.LocationMode.NULL); 101 break; 102 } 103 } 104 return tgt; 105 } 106 107 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationMode> convertLocationMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationMode> src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationMode> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Location.LocationModeEnumFactory()); 111 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 112 if (src.getValue() == null) { 113 tgt.setValue(null); 114 } else { 115 switch (src.getValue()) { 116 case INSTANCE: 117 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationMode.INSTANCE); 118 break; 119 case KIND: 120 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationMode.KIND); 121 break; 122 default: 123 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationMode.NULL); 124 break; 125 } 126 } 127 return tgt; 128 } 129 130 public static org.hl7.fhir.r5.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.dstu2.model.Location.LocationPositionComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.r5.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.r5.model.Location.LocationPositionComponent(); 134 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 135 if (src.hasLongitudeElement()) 136 tgt.setLongitudeElement(Decimal10_50.convertDecimal(src.getLongitudeElement())); 137 if (src.hasLatitudeElement()) 138 tgt.setLatitudeElement(Decimal10_50.convertDecimal(src.getLatitudeElement())); 139 if (src.hasAltitudeElement()) 140 tgt.setAltitudeElement(Decimal10_50.convertDecimal(src.getAltitudeElement())); 141 return tgt; 142 } 143 144 public static org.hl7.fhir.dstu2.model.Location.LocationPositionComponent convertLocationPositionComponent(org.hl7.fhir.r5.model.Location.LocationPositionComponent src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.dstu2.model.Location.LocationPositionComponent tgt = new org.hl7.fhir.dstu2.model.Location.LocationPositionComponent(); 148 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 149 if (src.hasLongitudeElement()) 150 tgt.setLongitudeElement(Decimal10_50.convertDecimal(src.getLongitudeElement())); 151 if (src.hasLatitudeElement()) 152 tgt.setLatitudeElement(Decimal10_50.convertDecimal(src.getLatitudeElement())); 153 if (src.hasAltitudeElement()) 154 tgt.setAltitudeElement(Decimal10_50.convertDecimal(src.getAltitudeElement())); 155 return tgt; 156 } 157 158 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationStatus> src) throws FHIRException { 159 if (src == null || src.isEmpty()) 160 return null; 161 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Location.LocationStatusEnumFactory()); 162 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 163 if (src.getValue() == null) { 164 tgt.setValue(null); 165 } else { 166 switch (src.getValue()) { 167 case ACTIVE: 168 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationStatus.ACTIVE); 169 break; 170 case SUSPENDED: 171 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationStatus.SUSPENDED); 172 break; 173 case INACTIVE: 174 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationStatus.INACTIVE); 175 break; 176 default: 177 tgt.setValue(org.hl7.fhir.dstu2.model.Location.LocationStatus.NULL); 178 break; 179 } 180 } 181 return tgt; 182 } 183 184 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Location.LocationStatus> convertLocationStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Location.LocationStatus> src) throws FHIRException { 185 if (src == null || src.isEmpty()) 186 return null; 187 Enumeration<Location.LocationStatus> tgt = new Enumeration<>(new Location.LocationStatusEnumFactory()); 188 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 189 if (src.getValue() == null) { 190 tgt.setValue(null); 191 } else { 192 switch (src.getValue()) { 193 case ACTIVE: 194 tgt.setValue(Location.LocationStatus.ACTIVE); 195 break; 196 case SUSPENDED: 197 tgt.setValue(Location.LocationStatus.SUSPENDED); 198 break; 199 case INACTIVE: 200 tgt.setValue(Location.LocationStatus.INACTIVE); 201 break; 202 default: 203 tgt.setValue(Location.LocationStatus.NULL); 204 break; 205 } 206 } 207 return tgt; 208 } 209}