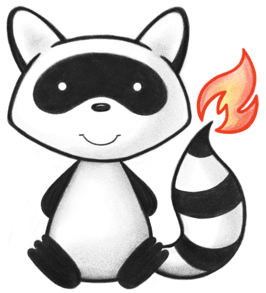
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Ratio10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Timing10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Dosage.DosageDoseAndRateComponent; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.MedicationStatement; 016 017public class MedicationStatement10_50 { 018 019 public static org.hl7.fhir.dstu2.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r5.model.MedicationStatement src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.dstu2.model.MedicationStatement tgt = new org.hl7.fhir.dstu2.model.MedicationStatement(); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 026 if (src.hasStatus()) 027 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 028 if (src.getMedication().hasConcept()) { 029 tgt.setMedication(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getMedication().getConcept())); 030 } 031 if (src.getMedication().hasReference()) { 032 tgt.setMedication(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getMedication().getReference())); 033 } 034 if (src.hasSubject()) 035 tgt.setPatient(Reference10_50.convertReference(src.getSubject())); 036 if (src.hasEffective()) 037 tgt.setEffective(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getEffective())); 038 if (src.hasInformationSource()) 039 tgt.setInformationSource(Reference10_50.convertReference(src.getInformationSourceFirstRep())); 040 for (org.hl7.fhir.r5.model.Reference t : src.getDerivedFrom()) 041 tgt.addSupportingInformation(Reference10_50.convertReference(t)); 042 if (src.hasDateAsserted()) 043 tgt.setDateAssertedElement(DateTime10_50.convertDateTime(src.getDateAssertedElement())); 044 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.setNote(t.getText()); 045 for (org.hl7.fhir.r5.model.Dosage t : src.getDosage()) tgt.addDosage(convertMedicationStatementDosageComponent(t)); 046 return tgt; 047 } 048 049 public static org.hl7.fhir.r5.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.dstu2.model.MedicationStatement src) throws FHIRException { 050 if (src == null || src.isEmpty()) 051 return null; 052 org.hl7.fhir.r5.model.MedicationStatement tgt = new org.hl7.fhir.r5.model.MedicationStatement(); 053 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 058 if (src.hasMedicationCodeableConcept()) { 059 tgt.getMedication().setConcept(CodeableConcept10_50.convertCodeableConcept(src.getMedicationCodeableConcept())); 060 } 061 if (src.hasMedicationReference()) { 062 tgt.getMedication().setReference(Reference10_50.convertReference(src.getMedicationReference())); 063 } 064 if (src.hasPatient()) 065 tgt.setSubject(Reference10_50.convertReference(src.getPatient())); 066 if (src.hasEffective()) 067 tgt.setEffective(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getEffective())); 068 if (src.hasInformationSource()) 069 tgt.addInformationSource(Reference10_50.convertReference(src.getInformationSource())); 070 for (org.hl7.fhir.dstu2.model.Reference t : src.getSupportingInformation()) 071 tgt.addDerivedFrom(Reference10_50.convertReference(t)); 072 if (src.hasDateAsserted()) 073 tgt.setDateAssertedElement(DateTime10_50.convertDateTime(src.getDateAssertedElement())); 074 if (src.hasNote()) 075 tgt.addNote().setText(src.getNote()); 076 for (org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent t : src.getDosage()) 077 tgt.addDosage(convertMedicationStatementDosageComponent(t)); 078 return tgt; 079 } 080 081 public static org.hl7.fhir.r5.model.Dosage convertMedicationStatementDosageComponent(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent src) throws FHIRException { 082 if (src == null || src.isEmpty()) 083 return null; 084 org.hl7.fhir.r5.model.Dosage tgt = new org.hl7.fhir.r5.model.Dosage(); 085 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 086 if (src.hasTextElement()) 087 tgt.setTextElement(String10_50.convertString(src.getTextElement())); 088 if (src.hasTiming()) 089 tgt.setTiming(Timing10_50.convertTiming(src.getTiming())); 090 if (src.hasAsNeededBooleanType()) 091 tgt.setAsNeededElement(Boolean10_50.convertBoolean(src.getAsNeededBooleanType())); 092 if (src.hasSiteCodeableConcept()) 093 tgt.setSite(CodeableConcept10_50.convertCodeableConcept(src.getSiteCodeableConcept())); 094 if (src.hasRoute()) 095 tgt.setRoute(CodeableConcept10_50.convertCodeableConcept(src.getRoute())); 096 if (src.hasMethod()) 097 tgt.setMethod(CodeableConcept10_50.convertCodeableConcept(src.getMethod())); 098 if (src.hasRate()) { 099 DosageDoseAndRateComponent dr = tgt.addDoseAndRate(); 100 if (src.hasRate()) 101 dr.setRate(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getRate())); 102 } 103 if (src.hasMaxDosePerPeriod()) 104 tgt.addMaxDosePerPeriod(Ratio10_50.convertRatio(src.getMaxDosePerPeriod())); 105 return tgt; 106 } 107 108 public static org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent convertMedicationStatementDosageComponent(org.hl7.fhir.r5.model.Dosage src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent tgt = new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementDosageComponent(); 112 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 113 if (src.hasTextElement()) 114 tgt.setTextElement(String10_50.convertString(src.getTextElement())); 115 if (src.hasTiming()) 116 tgt.setTiming(Timing10_50.convertTiming(src.getTiming())); 117 if (src.hasAsNeeded()) 118 tgt.setAsNeeded(Boolean10_50.convertBoolean(src.getAsNeededElement())); 119 if (src.hasSite()) 120 tgt.setSite(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getSite())); 121 if (src.hasRoute()) 122 tgt.setRoute(CodeableConcept10_50.convertCodeableConcept(src.getRoute())); 123 if (src.hasMethod()) 124 tgt.setMethod(CodeableConcept10_50.convertCodeableConcept(src.getMethod())); 125 if (src.hasDoseAndRate() && src.getDoseAndRate().get(0).hasRate()) 126 tgt.setRate(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getDoseAndRate().get(0).getRate())); 127 if (src.hasMaxDosePerPeriod()) 128 tgt.setMaxDosePerPeriod(Ratio10_50.convertRatio(src.getMaxDosePerPeriodFirstRep())); 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> convertMedicationStatementStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 Enumeration<MedicationStatement.MedicationStatementStatusCodes> tgt = new Enumeration<>(new MedicationStatement.MedicationStatementStatusCodesEnumFactory()); 136 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case ACTIVE: 142 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 143 break; 144 case COMPLETED: 145 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 146 break; 147 case ENTEREDINERROR: 148 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.ENTEREDINERROR); 149 break; 150 case INTENDED: 151 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 152 break; 153 default: 154 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.NULL); 155 break; 156 } 157 } 158 return tgt; 159 } 160 161 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatusEnumFactory()); 165 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 166 if (src.getValue() == null) { 167 tgt.setValue(null); 168 } else { 169 switch (src.getValue()) { 170 // case ACTIVE: 171// tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ACTIVE); 172// break; 173 case RECORDED: 174 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.COMPLETED); 175 break; 176 case ENTEREDINERROR: 177 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 178 break; 179 case DRAFT: 180 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.ACTIVE); 181 break; 182 default: 183 tgt.setValue(org.hl7.fhir.dstu2.model.MedicationStatement.MedicationStatementStatus.NULL); 184 break; 185 } 186 } 187 return tgt; 188 } 189}