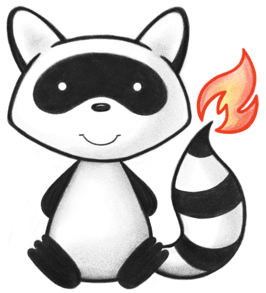
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Address10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.HumanName10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.ExtendedContactDetail; 014 015public class Organization10_50 { 016 017 public static org.hl7.fhir.r5.model.Organization convertOrganization(org.hl7.fhir.dstu2.model.Organization src) throws FHIRException { 018 if (src == null || src.isEmpty()) 019 return null; 020 org.hl7.fhir.r5.model.Organization tgt = new org.hl7.fhir.r5.model.Organization(); 021 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 022 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 023 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 024 if (src.hasActiveElement()) 025 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 026 if (src.hasType()) 027 tgt.addType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 028 if (src.hasNameElement()) 029 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 030 for (org.hl7.fhir.dstu2.model.Address t : src.getAddress()) tgt.addContact().setAddress(Address10_50.convertAddress(t)); 031 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 032 tgt.getContactFirstRep().addTelecom(ContactPoint10_50.convertContactPoint(t)); 033 if (src.hasPartOf()) 034 tgt.setPartOf(Reference10_50.convertReference(src.getPartOf())); 035 for (org.hl7.fhir.dstu2.model.Organization.OrganizationContactComponent t : src.getContact()) 036 tgt.addContact(convertOrganizationContactComponent(t)); 037 return tgt; 038 } 039 040 public static org.hl7.fhir.dstu2.model.Organization convertOrganization(org.hl7.fhir.r5.model.Organization src) throws FHIRException { 041 if (src == null || src.isEmpty()) 042 return null; 043 org.hl7.fhir.dstu2.model.Organization tgt = new org.hl7.fhir.dstu2.model.Organization(); 044 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 045 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 046 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 047 if (src.hasActiveElement()) 048 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 049 if (src.hasType()) 050 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getTypeFirstRep())); 051 if (src.hasNameElement()) 052 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 053 for (ExtendedContactDetail t1 : src.getContact()) 054 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 055 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 056 for (ExtendedContactDetail t : src.getContact()) 057 if (t.hasAddress()) 058 tgt.addAddress(Address10_50.convertAddress(t.getAddress())); 059 if (src.hasPartOf()) 060 tgt.setPartOf(Reference10_50.convertReference(src.getPartOf())); 061 for (org.hl7.fhir.r5.model.ExtendedContactDetail t : src.getContact()) 062 tgt.addContact(convertOrganizationContactComponent(t)); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.dstu2.model.Organization.OrganizationContactComponent convertOrganizationContactComponent(org.hl7.fhir.r5.model.ExtendedContactDetail src) throws FHIRException { 067 if (src == null || src.isEmpty()) 068 return null; 069 org.hl7.fhir.dstu2.model.Organization.OrganizationContactComponent tgt = new org.hl7.fhir.dstu2.model.Organization.OrganizationContactComponent(); 070 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 071 if (src.hasPurpose()) 072 tgt.setPurpose(CodeableConcept10_50.convertCodeableConcept(src.getPurpose())); 073 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) 074 tgt.setName(HumanName10_50.convertHumanName(t)); 075 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 076 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 077 if (src.hasAddress()) 078 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 079 return tgt; 080 } 081 082 public static org.hl7.fhir.r5.model.ExtendedContactDetail convertOrganizationContactComponent(org.hl7.fhir.dstu2.model.Organization.OrganizationContactComponent src) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.r5.model.ExtendedContactDetail tgt = new org.hl7.fhir.r5.model.ExtendedContactDetail(); 086 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 087 if (src.hasPurpose()) 088 tgt.setPurpose(CodeableConcept10_50.convertCodeableConcept(src.getPurpose())); 089 if (src.hasName()) 090 tgt.addName(HumanName10_50.convertHumanName(src.getName())); 091 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 092 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 093 if (src.hasAddress()) 094 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 095 return tgt; 096 } 097}