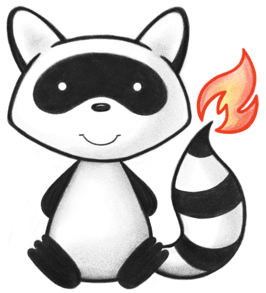
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Address10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Attachment10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.HumanName10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Period10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Date10_50; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class Patient10_50 { 017 018 public static org.hl7.fhir.dstu2.model.Patient.AnimalComponent convertAnimalComponent(org.hl7.fhir.r5.model.Extension src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.dstu2.model.Patient.AnimalComponent tgt = new org.hl7.fhir.dstu2.model.Patient.AnimalComponent(); 022 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 023 if (src.hasExtension("species")) 024 tgt.setSpecies(CodeableConcept10_50.convertCodeableConcept((org.hl7.fhir.r5.model.CodeableConcept) src.getExtensionByUrl("species").getValue())); 025 if (src.hasExtension("breed")) 026 tgt.setBreed(CodeableConcept10_50.convertCodeableConcept((org.hl7.fhir.r5.model.CodeableConcept) src.getExtensionByUrl("breed").getValue())); 027 if (src.hasExtension("genderStatus")) 028 tgt.setGenderStatus(CodeableConcept10_50.convertCodeableConcept((org.hl7.fhir.r5.model.CodeableConcept) src.getExtensionByUrl("genderStatus").getValue())); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.r5.model.Extension convertAnimalComponent(org.hl7.fhir.dstu2.model.Patient.AnimalComponent src) throws FHIRException { 033 if (src == null) 034 return null; 035 org.hl7.fhir.r5.model.Extension tgt = new org.hl7.fhir.r5.model.Extension(); 036 tgt.setUrl("http://hl7.org/fhir/StructureDefinition/patient-animal"); 037 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 038 if (src.hasSpecies()) 039 tgt.addExtension("species", CodeableConcept10_50.convertCodeableConcept(src.getSpecies())); 040 if (src.hasBreed()) 041 tgt.addExtension("breed", CodeableConcept10_50.convertCodeableConcept(src.getBreed())); 042 if (src.hasGenderStatus()) 043 tgt.addExtension("genderStatus", CodeableConcept10_50.convertCodeableConcept(src.getGenderStatus())); 044 return tgt; 045 } 046 047 public static org.hl7.fhir.r5.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.dstu2.model.Patient.ContactComponent src) throws FHIRException { 048 if (src == null || src.isEmpty()) 049 return null; 050 org.hl7.fhir.r5.model.Patient.ContactComponent tgt = new org.hl7.fhir.r5.model.Patient.ContactComponent(); 051 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 052 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getRelationship()) 053 tgt.addRelationship(CodeableConcept10_50.convertCodeableConcept(t)); 054 if (src.hasName()) 055 tgt.setName(HumanName10_50.convertHumanName(src.getName())); 056 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 057 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 058 if (src.hasAddress()) 059 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 060 if (src.hasGender()) 061 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 062 if (src.hasOrganization()) 063 tgt.setOrganization(Reference10_50.convertReference(src.getOrganization())); 064 if (src.hasPeriod()) 065 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.dstu2.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.r5.model.Patient.ContactComponent src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.dstu2.model.Patient.ContactComponent tgt = new org.hl7.fhir.dstu2.model.Patient.ContactComponent(); 073 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 074 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRelationship()) 075 tgt.addRelationship(CodeableConcept10_50.convertCodeableConcept(t)); 076 if (src.hasName()) 077 tgt.setName(HumanName10_50.convertHumanName(src.getName())); 078 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 079 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 080 if (src.hasAddress()) 081 tgt.setAddress(Address10_50.convertAddress(src.getAddress())); 082 if (src.hasGender()) 083 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 084 if (src.hasOrganization()) 085 tgt.setOrganization(Reference10_50.convertReference(src.getOrganization())); 086 if (src.hasPeriod()) 087 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 088 return tgt; 089 } 090 091 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Patient.LinkType> convertLinkType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Patient.LinkType> src) throws FHIRException { 092 if (src == null || src.isEmpty()) 093 return null; 094 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Patient.LinkType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Patient.LinkTypeEnumFactory()); 095 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 096 switch (src.getValue()) { 097 case REPLACE: 098 tgt.setValue(org.hl7.fhir.r5.model.Patient.LinkType.REPLACEDBY); 099 break; 100 case REFER: 101 tgt.setValue(org.hl7.fhir.r5.model.Patient.LinkType.REFER); 102 break; 103 case SEEALSO: 104 tgt.setValue(org.hl7.fhir.r5.model.Patient.LinkType.SEEALSO); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.r5.model.Patient.LinkType.NULL); 108 break; 109 } 110 return tgt; 111 } 112 113 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Patient.LinkType> convertLinkType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Patient.LinkType> src) throws FHIRException { 114 if (src == null || src.isEmpty()) 115 return null; 116 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Patient.LinkType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Patient.LinkTypeEnumFactory()); 117 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 118 switch (src.getValue()) { 119 case REPLACEDBY: 120 tgt.setValue(org.hl7.fhir.dstu2.model.Patient.LinkType.REPLACE); 121 break; 122 case REPLACES: 123 tgt.setValue(org.hl7.fhir.dstu2.model.Patient.LinkType.REPLACE); 124 break; 125 case REFER: 126 tgt.setValue(org.hl7.fhir.dstu2.model.Patient.LinkType.REFER); 127 break; 128 case SEEALSO: 129 tgt.setValue(org.hl7.fhir.dstu2.model.Patient.LinkType.SEEALSO); 130 break; 131 default: 132 tgt.setValue(org.hl7.fhir.dstu2.model.Patient.LinkType.NULL); 133 break; 134 } 135 return tgt; 136 } 137 138 public static org.hl7.fhir.r5.model.Patient convertPatient(org.hl7.fhir.dstu2.model.Patient src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.r5.model.Patient tgt = new org.hl7.fhir.r5.model.Patient(); 142 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 143 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 144 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 145 if (src.hasActiveElement()) 146 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 147 for (org.hl7.fhir.dstu2.model.HumanName t : src.getName()) tgt.addName(HumanName10_50.convertHumanName(t)); 148 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 149 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 150 if (src.hasGender()) 151 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 152 if (src.hasBirthDateElement()) 153 tgt.setBirthDateElement(Date10_50.convertDate(src.getBirthDateElement())); 154 if (src.hasDeceased()) 155 tgt.setDeceased(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getDeceased())); 156 for (org.hl7.fhir.dstu2.model.Address t : src.getAddress()) tgt.addAddress(Address10_50.convertAddress(t)); 157 if (src.hasMaritalStatus()) 158 tgt.setMaritalStatus(CodeableConcept10_50.convertCodeableConcept(src.getMaritalStatus())); 159 if (src.hasMultipleBirth()) 160 tgt.setMultipleBirth(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getMultipleBirth())); 161 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_50.convertAttachment(t)); 162 for (org.hl7.fhir.dstu2.model.Patient.ContactComponent t : src.getContact()) 163 tgt.addContact(convertContactComponent(t)); 164 if (src.hasAnimal()) 165 tgt.addExtension(convertAnimalComponent(src.getAnimal())); 166 for (org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 167 tgt.addCommunication(convertPatientCommunicationComponent(t)); 168 for (org.hl7.fhir.dstu2.model.Reference t : src.getCareProvider()) 169 tgt.addGeneralPractitioner(Reference10_50.convertReference(t)); 170 if (src.hasManagingOrganization()) 171 tgt.setManagingOrganization(Reference10_50.convertReference(src.getManagingOrganization())); 172 for (org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent t : src.getLink()) 173 tgt.addLink(convertPatientLinkComponent(t)); 174 return tgt; 175 } 176 177 public static org.hl7.fhir.dstu2.model.Patient convertPatient(org.hl7.fhir.r5.model.Patient src) throws FHIRException { 178 if (src == null || src.isEmpty()) 179 return null; 180 org.hl7.fhir.dstu2.model.Patient tgt = new org.hl7.fhir.dstu2.model.Patient(); 181 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 182 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 183 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 184 if (src.hasActiveElement()) 185 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 186 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.addName(HumanName10_50.convertHumanName(t)); 187 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 188 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 189 if (src.hasGender()) 190 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 191 if (src.hasBirthDateElement()) 192 tgt.setBirthDateElement(Date10_50.convertDate(src.getBirthDateElement())); 193 if (src.hasDeceased()) 194 tgt.setDeceased(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getDeceased())); 195 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address10_50.convertAddress(t)); 196 if (src.hasMaritalStatus()) 197 tgt.setMaritalStatus(CodeableConcept10_50.convertCodeableConcept(src.getMaritalStatus())); 198 if (src.hasMultipleBirth()) 199 tgt.setMultipleBirth(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getMultipleBirth())); 200 for (org.hl7.fhir.r5.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_50.convertAttachment(t)); 201 for (org.hl7.fhir.r5.model.Patient.ContactComponent t : src.getContact()) 202 tgt.addContact(convertContactComponent(t)); 203 if (src.hasExtension("http://hl7.org/fhir/StructureDefinition/patient-animal")) 204 tgt.setAnimal(convertAnimalComponent(src.getExtensionByUrl("http://hl7.org/fhir/StructureDefinition/patient-animal"))); 205 for (org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 206 tgt.addCommunication(convertPatientCommunicationComponent(t)); 207 for (org.hl7.fhir.r5.model.Reference t : src.getGeneralPractitioner()) 208 tgt.addCareProvider(Reference10_50.convertReference(t)); 209 if (src.hasManagingOrganization()) 210 tgt.setManagingOrganization(Reference10_50.convertReference(src.getManagingOrganization())); 211 for (org.hl7.fhir.r5.model.Patient.PatientLinkComponent t : src.getLink()) 212 tgt.addLink(convertPatientLinkComponent(t)); 213 return tgt; 214 } 215 216 public static org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent(); 220 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 221 if (src.hasLanguage()) 222 tgt.setLanguage(CodeableConcept10_50.convertCodeableConcept(src.getLanguage())); 223 if (src.hasPreferredElement()) 224 tgt.setPreferredElement(Boolean10_50.convertBoolean(src.getPreferredElement())); 225 return tgt; 226 } 227 228 public static org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.dstu2.model.Patient.PatientCommunicationComponent src) throws FHIRException { 229 if (src == null || src.isEmpty()) 230 return null; 231 org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent(); 232 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 233 if (src.hasLanguage()) 234 tgt.setLanguage(CodeableConcept10_50.convertCodeableConcept(src.getLanguage())); 235 if (src.hasPreferredElement()) 236 tgt.setPreferredElement(Boolean10_50.convertBoolean(src.getPreferredElement())); 237 return tgt; 238 } 239 240 public static org.hl7.fhir.r5.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent src) throws FHIRException { 241 if (src == null || src.isEmpty()) 242 return null; 243 org.hl7.fhir.r5.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.r5.model.Patient.PatientLinkComponent(); 244 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 245 if (src.hasOther()) 246 tgt.setOther(Reference10_50.convertReference(src.getOther())); 247 if (src.hasType()) 248 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 249 return tgt; 250 } 251 252 public static org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.r5.model.Patient.PatientLinkComponent src) throws FHIRException { 253 if (src == null || src.isEmpty()) 254 return null; 255 org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.dstu2.model.Patient.PatientLinkComponent(); 256 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 257 if (src.hasOther()) 258 tgt.setOther(Reference10_50.convertReference(src.getOther())); 259 if (src.hasType()) 260 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 261 return tgt; 262 } 263}