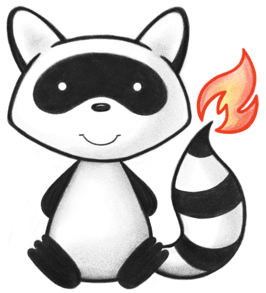
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Address10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Attachment10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.HumanName10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Period10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Date10_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.Practitioner.PractitionerCommunicationComponent; 016 017public class Practitioner10_50 { 018 019 public static org.hl7.fhir.r5.model.Practitioner convertPractitioner(org.hl7.fhir.dstu2.model.Practitioner src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.r5.model.Practitioner tgt = new org.hl7.fhir.r5.model.Practitioner(); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 024 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 025 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 026 if (src.hasActiveElement()) 027 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 028 if (src.hasName()) 029 tgt.addName(HumanName10_50.convertHumanName(src.getName())); 030 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 031 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 032 for (org.hl7.fhir.dstu2.model.Address t : src.getAddress()) tgt.addAddress(Address10_50.convertAddress(t)); 033 if (src.hasGender()) 034 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 035 if (src.hasBirthDateElement()) 036 tgt.setBirthDateElement(Date10_50.convertDate(src.getBirthDateElement())); 037 for (org.hl7.fhir.dstu2.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_50.convertAttachment(t)); 038 for (org.hl7.fhir.dstu2.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 039 tgt.addQualification(convertPractitionerQualificationComponent(t)); 040 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getCommunication()) 041 tgt.addCommunication().setLanguage(CodeableConcept10_50.convertCodeableConcept(t)); 042 return tgt; 043 } 044 045 public static org.hl7.fhir.dstu2.model.Practitioner convertPractitioner(org.hl7.fhir.r5.model.Practitioner src) throws FHIRException { 046 if (src == null || src.isEmpty()) 047 return null; 048 org.hl7.fhir.dstu2.model.Practitioner tgt = new org.hl7.fhir.dstu2.model.Practitioner(); 049 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 052 if (src.hasActiveElement()) 053 tgt.setActiveElement(Boolean10_50.convertBoolean(src.getActiveElement())); 054 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.setName(HumanName10_50.convertHumanName(t)); 055 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 056 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 057 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address10_50.convertAddress(t)); 058 if (src.hasGender()) 059 tgt.setGenderElement(Enumerations10_50.convertAdministrativeGender(src.getGenderElement())); 060 if (src.hasBirthDateElement()) 061 tgt.setBirthDateElement(Date10_50.convertDate(src.getBirthDateElement())); 062 for (org.hl7.fhir.r5.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment10_50.convertAttachment(t)); 063 for (org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent t : src.getQualification()) 064 tgt.addQualification(convertPractitionerQualificationComponent(t)); 065 for (PractitionerCommunicationComponent t : src.getCommunication()) 066 tgt.addCommunication(CodeableConcept10_50.convertCodeableConcept(t.getLanguage())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.dstu2.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent(); 074 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 075 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 076 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 077 if (src.hasCode()) 078 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 079 if (src.hasPeriod()) 080 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 081 if (src.hasIssuer()) 082 tgt.setIssuer(Reference10_50.convertReference(src.getIssuer())); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.dstu2.model.Practitioner.PractitionerQualificationComponent convertPractitionerQualificationComponent(org.hl7.fhir.r5.model.Practitioner.PractitionerQualificationComponent src) throws FHIRException { 087 if (src == null || src.isEmpty()) 088 return null; 089 org.hl7.fhir.dstu2.model.Practitioner.PractitionerQualificationComponent tgt = new org.hl7.fhir.dstu2.model.Practitioner.PractitionerQualificationComponent(); 090 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 091 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 092 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 093 if (src.hasCode()) 094 tgt.setCode(CodeableConcept10_50.convertCodeableConcept(src.getCode())); 095 if (src.hasPeriod()) 096 tgt.setPeriod(Period10_50.convertPeriod(src.getPeriod())); 097 if (src.hasIssuer()) 098 tgt.setIssuer(Reference10_50.convertReference(src.getIssuer())); 099 return tgt; 100 } 101}