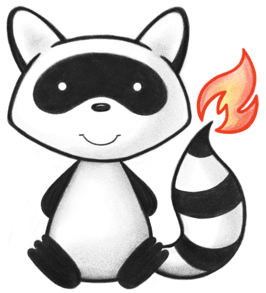
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Coding10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.MarkDown10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 013import org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.*; 016import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireAnswerConstraint; 017import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent; 018 019public class Questionnaire10_50 { 020 021 public static org.hl7.fhir.r5.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2.model.Questionnaire src) throws FHIRException { 022 if (src == null || src.isEmpty()) 023 return null; 024 org.hl7.fhir.r5.model.Questionnaire tgt = new org.hl7.fhir.r5.model.Questionnaire(); 025 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 026 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 027 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 028 if (src.hasVersionElement()) 029 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 030 if (src.hasStatus()) 031 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 032 if (src.hasDate()) 033 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 034 if (src.hasPublisherElement()) 035 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 036 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 037 tgt.addContact(convertQuestionnaireContactComponent(t)); 038 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = src.getGroup(); 039 tgt.setTitle(root.getTitle()); 040 for (org.hl7.fhir.dstu2.model.Coding t : root.getConcept()) tgt.addCode(Coding10_50.convertCoding(t)); 041 for (org.hl7.fhir.dstu2.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 042 tgt.addItem(convertQuestionnaireGroupComponent(root)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu2.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r5.model.Questionnaire src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu2.model.Questionnaire tgt = new org.hl7.fhir.dstu2.model.Questionnaire(); 050 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 053 if (src.hasVersionElement()) 054 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 057 if (src.hasDate()) 058 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 059 if (src.hasPublisherElement()) 060 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 061 for (ContactDetail t : src.getContact()) 062 for (org.hl7.fhir.r5.model.ContactPoint t1 : t.getTelecom()) 063 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t1)); 064 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent root = tgt.getGroup(); 065 root.setTitle(src.getTitle()); 066 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) { 067 root.addConcept(Coding10_50.convertCoding(t)); 068 } 069 for (CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 070 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 071 if (t.getType() == org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType.GROUP) 072 root.addGroup(convertQuestionnaireGroupComponent(t)); 073 else 074 root.addQuestion(convertQuestionnaireQuestionComponent(t)); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r5.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2.model.ContactPoint src) throws FHIRException { 079 if (src == null || src.isEmpty()) 080 return null; 081 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 082 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 083 tgt.addTelecom(ContactPoint10_50.convertContactPoint(src)); 084 return tgt; 085 } 086 087 public static org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent convertQuestionnaireGroupComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent(); 091 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 092 if (src.hasLinkIdElement()) 093 tgt.setLinkIdElement(String10_50.convertString(src.getLinkIdElement())); 094 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addConcept(Coding10_50.convertCoding(t)); 095 if (src.hasTextElement()) 096 tgt.setTextElement(String10_50.convertString(src.getTextElement())); 097 if (src.hasRequiredElement()) 098 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 099 if (src.hasRepeatsElement()) 100 tgt.setRepeatsElement(Boolean10_50.convertBoolean(src.getRepeatsElement())); 101 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 102 if (t.getType() == org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType.GROUP) 103 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 104 else 105 tgt.addQuestion(convertQuestionnaireQuestionComponent(t)); 106 return tgt; 107 } 108 109 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireGroupComponent(org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent(); 113 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 114 if (src.hasLinkIdElement()) 115 tgt.setLinkIdElement(String10_50.convertString(src.getLinkIdElement())); 116 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_50.convertCoding(t)); 117 if (src.hasTextElement()) 118 tgt.setTextElement(MarkDown10_50.convertStringToMarkdown(src.getTextElement())); 119 tgt.setType(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType.GROUP); 120 if (src.hasRequiredElement()) 121 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 122 if (src.hasRepeatsElement()) 123 tgt.setRepeatsElement(Boolean10_50.convertBoolean(src.getRepeatsElement())); 124 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 125 tgt.addItem(convertQuestionnaireGroupComponent(t)); 126 for (org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent t : src.getQuestion()) 127 tgt.addItem(convertQuestionnaireQuestionComponent(t)); 128 return tgt; 129 } 130 131 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> convertQuestionnaireItemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> src, QuestionnaireAnswerConstraint constraint) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormatEnumFactory()); 135 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt, VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL); 136 if (src.hasExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)) { 137 tgt.setValueAsString(src.getExtensionString(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)); 138 } else { 139 if (src.getValue() == null) { 140 tgt.setValue(null); 141 } else { 142 switch (src.getValue()) { 143 case BOOLEAN: 144 tgt.setValue(AnswerFormat.BOOLEAN); 145 break; 146 case DECIMAL: 147 tgt.setValue(AnswerFormat.DECIMAL); 148 break; 149 case INTEGER: 150 tgt.setValue(AnswerFormat.INTEGER); 151 break; 152 case DATE: 153 tgt.setValue(AnswerFormat.DATE); 154 break; 155 case DATETIME: 156 tgt.setValue(AnswerFormat.DATETIME); 157 break; 158 case TIME: 159 tgt.setValue(AnswerFormat.TIME); 160 break; 161 case STRING: 162 tgt.setValue(AnswerFormat.STRING); 163 break; 164 case TEXT: 165 tgt.setValue(AnswerFormat.TEXT); 166 break; 167 case URL: 168 tgt.setValue(AnswerFormat.URL); 169 break; 170 case CODING: 171 if (constraint == QuestionnaireAnswerConstraint.OPTIONSORSTRING) 172 tgt.setValue(AnswerFormat.OPENCHOICE); 173 else 174 tgt.setValue(AnswerFormat.CHOICE); 175 break; 176 case ATTACHMENT: 177 tgt.setValue(AnswerFormat.ATTACHMENT); 178 break; 179 case REFERENCE: 180 tgt.setValue(AnswerFormat.REFERENCE); 181 break; 182 case QUANTITY: 183 tgt.setValue(AnswerFormat.QUANTITY); 184 break; 185 default: 186 tgt.setValue(AnswerFormat.NULL); 187 break; 188 } 189 } 190 } 191 return tgt; 192 } 193 194 public static org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 195 if (src == null || src.isEmpty()) 196 return null; 197 org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent tgt = new org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent(); 198 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 199 if (src.hasLinkIdElement()) 200 tgt.setLinkIdElement(String10_50.convertString(src.getLinkIdElement())); 201 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addConcept(Coding10_50.convertCoding(t)); 202 if (src.hasTextElement()) 203 tgt.setTextElement(String10_50.convertString(src.getTextElement())); 204 if (src.hasType()) 205 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement(), src.getAnswerConstraint())); 206 if (src.hasRequiredElement()) 207 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 208 if (src.hasRepeatsElement()) 209 tgt.setRepeatsElement(Boolean10_50.convertBoolean(src.getRepeatsElement())); 210 if (src.hasAnswerValueSetElement()) 211 tgt.setOptions(Reference10_50.convertCanonicalToReference(src.getAnswerValueSetElement())); 212 for (QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 213 if (t.hasValueCoding()) 214 try { 215 tgt.addOption(Coding10_50.convertCoding(t.getValueCoding())); 216 } catch (org.hl7.fhir.exceptions.FHIRException e) { 217 throw new FHIRException(e); 218 } 219 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 220 tgt.addGroup(convertQuestionnaireGroupComponent(t)); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireQuestionComponent(org.hl7.fhir.dstu2.model.Questionnaire.QuestionComponent src) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent(); 228 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 229 if (src.hasLinkIdElement()) 230 tgt.setLinkIdElement(String10_50.convertString(src.getLinkIdElement())); 231 for (org.hl7.fhir.dstu2.model.Coding t : src.getConcept()) tgt.addCode(Coding10_50.convertCoding(t)); 232 if (src.hasTextElement()) 233 tgt.setTextElement(MarkDown10_50.convertStringToMarkdown(src.getTextElement())); 234 if (src.hasType()) { 235 tgt.setTypeElement(convertQuestionnaireQuestionType(src.getTypeElement())); 236 if (src.getType() == AnswerFormat.CHOICE) { 237 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSONLY); 238 } else if (src.getType() == AnswerFormat.OPENCHOICE) { 239 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSORSTRING); 240 } 241 } 242 if (src.hasRequiredElement()) 243 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 244 if (src.hasRepeatsElement()) 245 tgt.setRepeatsElement(Boolean10_50.convertBoolean(src.getRepeatsElement())); 246 if (src.hasOptions()) 247 tgt.setAnswerValueSetElement(Reference10_50.convertReferenceToCanonical(src.getOptions())); 248 for (org.hl7.fhir.dstu2.model.Coding t : src.getOption()) 249 tgt.addAnswerOption().setValue(Coding10_50.convertCoding(t)); 250 for (org.hl7.fhir.dstu2.model.Questionnaire.GroupComponent t : src.getGroup()) 251 tgt.addItem(convertQuestionnaireGroupComponent(t)); 252 return tgt; 253 } 254 255 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireQuestionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.AnswerFormat> src) throws FHIRException { 256 if (src == null || src.isEmpty()) 257 return null; 258 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 259 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 260 tgt.addExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL, new CodeType(src.getValueAsString())); 261 if (src.getValue() == null) { 262 tgt.setValue(null); 263 } else { 264 switch (src.getValue()) { 265 case BOOLEAN: 266 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 267 break; 268 case DECIMAL: 269 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 270 break; 271 case INTEGER: 272 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 273 break; 274 case DATE: 275 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 276 break; 277 case DATETIME: 278 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 279 break; 280 case INSTANT: 281 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 282 break; 283 case TIME: 284 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 285 break; 286 case STRING: 287 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 288 break; 289 case TEXT: 290 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 291 break; 292 case URL: 293 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 294 break; 295 case CHOICE: 296 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 297 break; 298 case OPENCHOICE: 299 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 300 break; 301 case ATTACHMENT: 302 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 303 break; 304 case REFERENCE: 305 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 306 break; 307 case QUANTITY: 308 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 309 break; 310 default: 311 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 312 break; 313 } 314 } 315 return tgt; 316 } 317 318 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> src) throws FHIRException { 319 if (src == null || src.isEmpty()) 320 return null; 321 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatusEnumFactory()); 322 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 323 if (src.getValue() == null) { 324 tgt.setValue(null); 325 } else { 326 switch (src.getValue()) { 327 case DRAFT: 328 tgt.setValue(org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus.DRAFT); 329 break; 330 case ACTIVE: 331 tgt.setValue(org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus.PUBLISHED); 332 break; 333 case RETIRED: 334 tgt.setValue(org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus.RETIRED); 335 break; 336 default: 337 tgt.setValue(org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus.NULL); 338 break; 339 } 340 } 341 return tgt; 342 } 343 344 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 345 if (src == null || src.isEmpty()) 346 return null; 347 Enumeration<Enumerations.PublicationStatus> tgt = new Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 348 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 349 if (src.getValue() == null) { 350 tgt.setValue(null); 351 } else { 352 switch (src.getValue()) { 353 case DRAFT: 354 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 355 break; 356 case PUBLISHED: 357 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 358 break; 359 case RETIRED: 360 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 361 break; 362 default: 363 tgt.setValue(Enumerations.PublicationStatus.NULL); 364 break; 365 } 366 } 367 return tgt; 368 } 369}