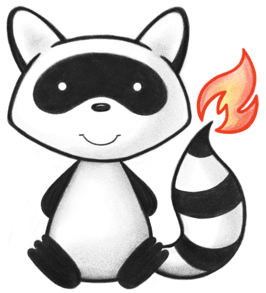
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Period10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.CodeableReference; 011 012public class Schedule10_50 { 013 014 public static org.hl7.fhir.dstu2.model.Schedule convertSchedule(org.hl7.fhir.r5.model.Schedule src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.dstu2.model.Schedule tgt = new org.hl7.fhir.dstu2.model.Schedule(); 018 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 019 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 020 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 021 for (CodeableReference t : src.getServiceType()) 022 if (t.hasConcept()) 023 tgt.addType(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 024 if (src.hasActor()) 025 tgt.setActor(Reference10_50.convertReference(src.getActorFirstRep())); 026 if (src.hasPlanningHorizon()) 027 tgt.setPlanningHorizon(Period10_50.convertPeriod(src.getPlanningHorizon())); 028 if (src.hasCommentElement()) 029 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 030 return tgt; 031 } 032 033 public static org.hl7.fhir.r5.model.Schedule convertSchedule(org.hl7.fhir.dstu2.model.Schedule src) throws FHIRException { 034 if (src == null || src.isEmpty()) 035 return null; 036 org.hl7.fhir.r5.model.Schedule tgt = new org.hl7.fhir.r5.model.Schedule(); 037 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 038 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 039 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 040 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getType()) 041 tgt.addServiceType(new CodeableReference().setConcept(CodeableConcept10_50.convertCodeableConcept(t))); 042 if (src.hasActor()) 043 tgt.addActor(Reference10_50.convertReference(src.getActor())); 044 if (src.hasPlanningHorizon()) 045 tgt.setPlanningHorizon(Period10_50.convertPeriod(src.getPlanningHorizon())); 046 if (src.hasCommentElement()) 047 tgt.setCommentElement(String10_50.convertStringToMarkdown(src.getCommentElement())); 048 return tgt; 049 } 050}