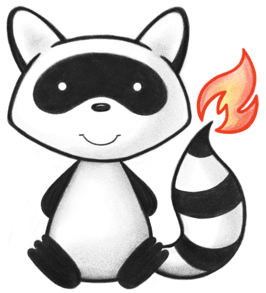
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Code10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 010import org.hl7.fhir.dstu2.utils.ToolingExtensions; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAll; 014import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAllEnumFactory; 015import org.hl7.fhir.r5.model.SearchParameter; 016 017public class SearchParameter10_50 { 018 019 public static org.hl7.fhir.dstu2.model.SearchParameter convertSearchParameter(org.hl7.fhir.r5.model.SearchParameter src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.dstu2.model.SearchParameter tgt = new org.hl7.fhir.dstu2.model.SearchParameter(); 023 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 024 if (src.hasUrlElement()) 025 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 026 if (src.hasNameElement()) 027 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 028 if (src.hasStatus()) 029 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 030 if (src.hasExperimental()) 031 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 032 if (src.hasDate()) 033 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 034 if (src.hasPublisherElement()) 035 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 036 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 037 tgt.addContact(convertSearchParameterContactComponent(t)); 038 if (src.hasPurpose()) 039 tgt.setRequirements(src.getPurpose()); 040 if (src.hasCodeElement()) 041 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 042 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getBase()) tgt.setBaseElement(Code10_50.convertCode(t.getCodeType())); 043 if (src.hasType()) 044 tgt.setTypeElement(Enumerations10_50.convertSearchParamType(src.getTypeElement())); 045 if (src.hasDescription()) 046 tgt.setDescription(src.getDescription()); 047 org.hl7.fhir.dstu2.utils.ToolingExtensions.setStringExtension(tgt, ToolingExtensions.EXT_EXPRESSION, src.getExpression()); 048// if (src.hasXpathElement()) 049// tgt.setXpathElement(String10_50.convertString(src.getXpathElement())); 050 if (src.hasProcessingMode()) 051 tgt.setXpathUsageElement(convertXPathUsageType(src.getProcessingModeElement())); 052 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getTarget()) tgt.getTarget().add(Code10_50.convertCode(t.getCodeType())); 053 return tgt; 054 } 055 056 public static org.hl7.fhir.r5.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu2.model.SearchParameter src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 org.hl7.fhir.r5.model.SearchParameter tgt = new org.hl7.fhir.r5.model.SearchParameter(); 060 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 061 if (src.hasUrlElement()) 062 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 063 if (src.hasNameElement()) 064 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 065 if (src.hasStatus()) 066 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 067 if (src.hasExperimental()) 068 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 069 if (src.hasDate()) 070 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 071 if (src.hasPublisherElement()) 072 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 073 for (org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent t : src.getContact()) 074 tgt.addContact(convertSearchParameterContactComponent(t)); 075 if (src.hasRequirements()) 076 tgt.setPurpose(src.getRequirements()); 077 if (src.hasCodeElement()) 078 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 079 tgt.getBase().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), Code10_50.convertCode(src.getBaseElement()))); 080 if (src.hasType()) 081 tgt.setTypeElement(Enumerations10_50.convertSearchParamType(src.getTypeElement())); 082 if (src.hasDescription()) 083 tgt.setDescription(src.getDescription()); 084 tgt.setExpression(ToolingExtensions.readStringExtension(src, ToolingExtensions.EXT_EXPRESSION)); 085// if (src.hasXpathElement()) 086// tgt.setXpathElement(String10_50.convertString(src.getXpathElement())); 087 if (src.hasXpathUsage()) 088 tgt.setProcessingModeElement(convertXPathUsageType(src.getXpathUsageElement())); 089 for (org.hl7.fhir.dstu2.model.CodeType t : src.getTarget()) tgt.getTarget().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), t.getValue())); 090 return tgt; 091 } 092 093 public static org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent convertSearchParameterContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 094 if (src == null || src.isEmpty()) 095 return null; 096 org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent tgt = new org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent(); 097 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 098 if (src.hasNameElement()) 099 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 100 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 101 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 102 return tgt; 103 } 104 105 public static org.hl7.fhir.r5.model.ContactDetail convertSearchParameterContactComponent(org.hl7.fhir.dstu2.model.SearchParameter.SearchParameterContactComponent src) throws FHIRException { 106 if (src == null || src.isEmpty()) 107 return null; 108 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 109 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 110 if (src.hasNameElement()) 111 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 112 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 113 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 114 return tgt; 115 } 116 117 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> convertXPathUsageType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType> src) throws FHIRException { 118 if (src == null || src.isEmpty()) 119 return null; 120 Enumeration<SearchParameter.SearchProcessingModeType> tgt = new Enumeration<>(new SearchParameter.SearchProcessingModeTypeEnumFactory()); 121 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 122 if (src.getValue() == null) { 123 tgt.setValue(null); 124 } else { 125 switch (src.getValue()) { 126 case NORMAL: 127 tgt.setValue(SearchParameter.SearchProcessingModeType.NORMAL); 128 break; 129 case PHONETIC: 130 tgt.setValue(SearchParameter.SearchProcessingModeType.PHONETIC); 131 break; 132 case NEARBY: 133 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 134 break; 135 case DISTANCE: 136 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 137 break; 138 case OTHER: 139 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 140 break; 141 default: 142 tgt.setValue(SearchParameter.SearchProcessingModeType.NULL); 143 break; 144 } 145 } 146 return tgt; 147 } 148 149 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageTypeEnumFactory()); 153 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 154 if (src.getValue() == null) { 155 tgt.setValue(null); 156 } else { 157 switch (src.getValue()) { 158 case NORMAL: 159 tgt.setValue(org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType.NORMAL); 160 break; 161 case PHONETIC: 162 tgt.setValue(org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType.PHONETIC); 163 break; 164 case OTHER: 165 tgt.setValue(org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType.OTHER); 166 break; 167 default: 168 tgt.setValue(org.hl7.fhir.dstu2.model.SearchParameter.XPathUsageType.NULL); 169 break; 170 } 171 } 172 return tgt; 173 } 174}