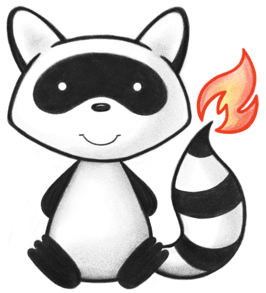
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Instant10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012import org.hl7.fhir.r5.model.Slot; 013 014public class Slot10_50 { 015 016 public static org.hl7.fhir.r5.model.Slot convertSlot(org.hl7.fhir.dstu2.model.Slot src) throws FHIRException { 017 if (src == null || src.isEmpty()) 018 return null; 019 org.hl7.fhir.r5.model.Slot tgt = new org.hl7.fhir.r5.model.Slot(); 020 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 021 for (org.hl7.fhir.dstu2.model.Identifier t : src.getIdentifier()) 022 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 023 if (src.hasType()) 024 tgt.addServiceType(new CodeableReference().setConcept(CodeableConcept10_50.convertCodeableConcept(src.getType()))); 025 if (src.hasSchedule()) 026 tgt.setSchedule(Reference10_50.convertReference(src.getSchedule())); 027 if (src.hasStartElement()) 028 tgt.setStartElement(Instant10_50.convertInstant(src.getStartElement())); 029 if (src.hasEndElement()) 030 tgt.setEndElement(Instant10_50.convertInstant(src.getEndElement())); 031 if (src.hasOverbookedElement()) 032 tgt.setOverbookedElement(Boolean10_50.convertBoolean(src.getOverbookedElement())); 033 if (src.hasCommentElement()) 034 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 035 return tgt; 036 } 037 038 public static org.hl7.fhir.dstu2.model.Slot convertSlot(org.hl7.fhir.r5.model.Slot src) throws FHIRException { 039 if (src == null || src.isEmpty()) 040 return null; 041 org.hl7.fhir.dstu2.model.Slot tgt = new org.hl7.fhir.dstu2.model.Slot(); 042 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 043 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 044 tgt.addIdentifier(Identifier10_50.convertIdentifier(t)); 045 for (CodeableReference t : src.getServiceType()) 046 if (t.hasConcept()) 047 tgt.setType(CodeableConcept10_50.convertCodeableConcept(t.getConcept())); 048 if (src.hasSchedule()) 049 tgt.setSchedule(Reference10_50.convertReference(src.getSchedule())); 050 if (src.hasStartElement()) 051 tgt.setStartElement(Instant10_50.convertInstant(src.getStartElement())); 052 if (src.hasEndElement()) 053 tgt.setEndElement(Instant10_50.convertInstant(src.getEndElement())); 054 if (src.hasOverbookedElement()) 055 tgt.setOverbookedElement(Boolean10_50.convertBoolean(src.getOverbookedElement())); 056 if (src.hasCommentElement()) 057 tgt.setCommentElement(String10_50.convertString(src.getCommentElement())); 058 return tgt; 059 } 060 061 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Slot.SlotStatus> src) throws FHIRException { 062 if (src == null || src.isEmpty()) return null; 063 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Slot.SlotStatusEnumFactory()); 064 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 065 if (src.getValue() == null) { 066 tgt.setValue(null); 067} else { 068 switch(src.getValue()) { 069 case BUSY: 070 tgt.setValue(Slot.SlotStatus.BUSY); 071 break; 072 case FREE: 073 tgt.setValue(Slot.SlotStatus.FREE); 074 break; 075 case BUSYUNAVAILABLE: 076 tgt.setValue(Slot.SlotStatus.BUSYUNAVAILABLE); 077 break; 078 case BUSYTENTATIVE: 079 tgt.setValue(Slot.SlotStatus.BUSYTENTATIVE); 080 break; 081 default: 082 tgt.setValue(Slot.SlotStatus.NULL); 083 break; 084 } 085} 086 return tgt; 087 } 088 089 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Slot.SlotStatus> convertSlotStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Slot.SlotStatus> src) throws FHIRException { 090 if (src == null || src.isEmpty()) return null; 091 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.Slot.SlotStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.Slot.SlotStatusEnumFactory()); 092 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 093 if (src.getValue() == null) { 094 tgt.setValue(null); 095} else { 096 switch(src.getValue()) { 097 case BUSY: 098 tgt.setValue(org.hl7.fhir.dstu2.model.Slot.SlotStatus.BUSY); 099 break; 100 case FREE: 101 tgt.setValue(org.hl7.fhir.dstu2.model.Slot.SlotStatus.FREE); 102 break; 103 case BUSYUNAVAILABLE: 104 tgt.setValue(org.hl7.fhir.dstu2.model.Slot.SlotStatus.BUSYUNAVAILABLE); 105 break; 106 case BUSYTENTATIVE: 107 tgt.setValue(org.hl7.fhir.dstu2.model.Slot.SlotStatus.BUSYTENTATIVE); 108 break; 109 default: 110 tgt.setValue(org.hl7.fhir.dstu2.model.Slot.SlotStatus.NULL); 111 break; 112 } 113} 114 return tgt; 115 } 116}