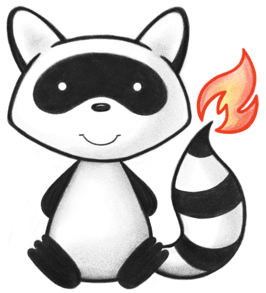
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 007import org.hl7.fhir.dstu2.model.Enumeration; 008import org.hl7.fhir.dstu2.model.SupplyDelivery; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.SupplyRequest; 011 012public class SupplyDelivery10_50 { 013 014 public static org.hl7.fhir.dstu2.model.SupplyDelivery convertSupplyDelivery(org.hl7.fhir.r5.model.SupplyDelivery src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.dstu2.model.SupplyDelivery tgt = new org.hl7.fhir.dstu2.model.SupplyDelivery(); 018 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 019 if (src.hasIdentifier()) 020 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifierFirstRep())); 021 if (src.hasStatus()) 022 tgt.setStatusElement(convertSupplyDeliveryStatus(src.getStatusElement())); 023 if (src.hasPatient()) 024 tgt.setPatient(Reference10_50.convertReference(src.getPatient())); 025 if (src.hasType()) 026 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 027 if (src.hasSupplier()) 028 tgt.setSupplier(Reference10_50.convertReference(src.getSupplier())); 029 if (src.hasDestination()) 030 tgt.setDestination(Reference10_50.convertReference(src.getDestination())); 031 for (org.hl7.fhir.r5.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference10_50.convertReference(t)); 032 return tgt; 033 } 034 035 public static org.hl7.fhir.r5.model.SupplyDelivery convertSupplyDelivery(org.hl7.fhir.dstu2.model.SupplyDelivery src) throws FHIRException { 036 if (src == null || src.isEmpty()) 037 return null; 038 org.hl7.fhir.r5.model.SupplyDelivery tgt = new org.hl7.fhir.r5.model.SupplyDelivery(); 039 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 040 if (src.hasIdentifier()) 041 tgt.addIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 042 if (src.hasStatus()) 043 tgt.setStatusElement(convertSupplyDeliveryStatus(src.getStatusElement())); 044 if (src.hasPatient()) 045 tgt.setPatient(Reference10_50.convertReference(src.getPatient())); 046 if (src.hasType()) 047 tgt.setType(CodeableConcept10_50.convertCodeableConcept(src.getType())); 048 if (src.hasSupplier()) 049 tgt.setSupplier(Reference10_50.convertReference(src.getSupplier())); 050 if (src.hasDestination()) 051 tgt.setDestination(Reference10_50.convertReference(src.getDestination())); 052 for (org.hl7.fhir.dstu2.model.Reference t : src.getReceiver()) tgt.addReceiver(Reference10_50.convertReference(t)); 053 return tgt; 054 } 055 056 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyDelivery.SupplyDeliveryStatus> convertSupplyDeliveryStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus> src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 Enumeration<SupplyDelivery.SupplyDeliveryStatus> tgt = new Enumeration<>(new SupplyDelivery.SupplyDeliveryStatusEnumFactory()); 060 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 061 if (src.getValue() == null) { 062 tgt.setValue(null); 063 } else { 064 switch (src.getValue()) { 065 case INPROGRESS: 066 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.INPROGRESS); 067 break; 068 case COMPLETED: 069 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.COMPLETED); 070 break; 071 case ABANDONED: 072 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.ABANDONED); 073 break; 074 default: 075 tgt.setValue(SupplyDelivery.SupplyDeliveryStatus.NULL); 076 break; 077 } 078 } 079 return tgt; 080 } 081 082 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus> convertSupplyDeliveryStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyDelivery.SupplyDeliveryStatus> src) throws FHIRException { 083 if (src == null || src.isEmpty()) 084 return null; 085 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatusEnumFactory()); 086 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 087 if (src.getValue() == null) { 088 tgt.setValue(null); 089 } else { 090 switch (src.getValue()) { 091 case INPROGRESS: 092 tgt.setValue(org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus.INPROGRESS); 093 break; 094 case COMPLETED: 095 tgt.setValue(org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus.COMPLETED); 096 break; 097 case ABANDONED: 098 tgt.setValue(org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus.ABANDONED); 099 break; 100 default: 101 tgt.setValue(org.hl7.fhir.r5.model.SupplyDelivery.SupplyDeliveryStatus.NULL); 102 break; 103 } 104 } 105 return tgt; 106 } 107 108 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 109 if (src == null || src.isEmpty()) return null; 110 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatusEnumFactory()); 111 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 112 if (src.getValue() == null) { 113 tgt.setValue(null); 114} else { 115 switch(src.getValue()) { 116 case REQUESTED: 117 tgt.setValue(SupplyRequest.SupplyRequestStatus.ACTIVE); 118 break; 119 case COMPLETED: 120 tgt.setValue(SupplyRequest.SupplyRequestStatus.COMPLETED); 121 break; 122 case FAILED: 123 tgt.setValue(SupplyRequest.SupplyRequestStatus.CANCELLED); 124 break; 125 case CANCELLED: 126 tgt.setValue(SupplyRequest.SupplyRequestStatus.CANCELLED); 127 break; 128 default: 129 tgt.setValue(SupplyRequest.SupplyRequestStatus.NULL); 130 break; 131 } 132} 133 return tgt; 134 } 135 136 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 137 if (src == null || src.isEmpty()) return null; 138 org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus> tgt = new org.hl7.fhir.dstu2.model.Enumeration<>(new org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatusEnumFactory()); 139 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 140 if (src.getValue() == null) { 141 tgt.setValue(null); 142} else { 143 switch(src.getValue()) { 144 case ACTIVE: 145 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.REQUESTED); 146 break; 147 case COMPLETED: 148 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.COMPLETED); 149 break; 150 case CANCELLED: 151 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.CANCELLED); 152 break; 153 default: 154 tgt.setValue(org.hl7.fhir.dstu2.model.SupplyRequest.SupplyRequestStatus.NULL); 155 break; 156 } 157} 158 return tgt; 159 } 160}