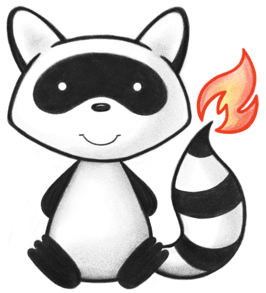
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext10_50; 004import org.hl7.fhir.convertors.conv10_50.VersionConvertor_10_50; 005import org.hl7.fhir.convertors.conv10_50.datatypes10_50.Reference10_50; 006import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 007import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Coding10_50; 008import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Id10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Integer10_50; 014import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 015import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 016import org.hl7.fhir.dstu2.model.Enumeration; 017import org.hl7.fhir.dstu2.model.TestScript; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r5.model.CanonicalType; 020 021public class TestScript10_50 { 022 023 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> src) throws FHIRException { 024 if (src == null || src.isEmpty()) 025 return null; 026 Enumeration<TestScript.AssertionDirectionType> tgt = new Enumeration<>(new TestScript.AssertionDirectionTypeEnumFactory()); 027 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 028 if (src.getValue() == null) { 029 tgt.setValue(null); 030 } else { 031 switch (src.getValue()) { 032 case RESPONSE: 033 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 034 break; 035 case REQUEST: 036 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 037 break; 038 default: 039 tgt.setValue(TestScript.AssertionDirectionType.NULL); 040 break; 041 } 042 } 043 return tgt; 044 } 045 046 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionDirectionType> src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionDirectionTypeEnumFactory()); 050 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 051 if (src.getValue() == null) { 052 tgt.setValue(null); 053 } else { 054 switch (src.getValue()) { 055 case RESPONSE: 056 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionDirectionType.RESPONSE); 057 break; 058 case REQUEST: 059 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionDirectionType.REQUEST); 060 break; 061 default: 062 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionDirectionType.NULL); 063 break; 064 } 065 } 066 return tgt; 067 } 068 069 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 Enumeration<TestScript.AssertionOperatorType> tgt = new Enumeration<>(new TestScript.AssertionOperatorTypeEnumFactory()); 073 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 074 if (src.getValue() == null) { 075 tgt.setValue(null); 076 } else { 077 switch (src.getValue()) { 078 case EQUALS: 079 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 080 break; 081 case NOTEQUALS: 082 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 083 break; 084 case IN: 085 tgt.setValue(TestScript.AssertionOperatorType.IN); 086 break; 087 case NOTIN: 088 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 089 break; 090 case GREATERTHAN: 091 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 092 break; 093 case LESSTHAN: 094 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 095 break; 096 case EMPTY: 097 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 098 break; 099 case NOTEMPTY: 100 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 101 break; 102 case CONTAINS: 103 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 104 break; 105 case NOTCONTAINS: 106 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 107 break; 108 default: 109 tgt.setValue(TestScript.AssertionOperatorType.NULL); 110 break; 111 } 112 } 113 return tgt; 114 } 115 116 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionOperatorType> src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionOperatorTypeEnumFactory()); 120 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 121 if (src.getValue() == null) { 122 tgt.setValue(null); 123 } else { 124 switch (src.getValue()) { 125 case EQUALS: 126 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.EQUALS); 127 break; 128 case NOTEQUALS: 129 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.NOTEQUALS); 130 break; 131 case IN: 132 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.IN); 133 break; 134 case NOTIN: 135 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.NOTIN); 136 break; 137 case GREATERTHAN: 138 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.GREATERTHAN); 139 break; 140 case LESSTHAN: 141 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.LESSTHAN); 142 break; 143 case EMPTY: 144 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.EMPTY); 145 break; 146 case NOTEMPTY: 147 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.NOTEMPTY); 148 break; 149 case CONTAINS: 150 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.CONTAINS); 151 break; 152 case NOTCONTAINS: 153 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.NOTCONTAINS); 154 break; 155 default: 156 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionOperatorType.NULL); 157 break; 158 } 159 } 160 return tgt; 161 } 162 163 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 164 if (src == null || src.isEmpty()) 165 return null; 166 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.TestScript.AssertionResponseTypesEnumFactory()); 167 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 168 if (src.getValue() == null) { 169 tgt.setValue(null); 170 } else { 171 switch (src.getValue()) { 172 case OKAY: 173 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.OKAY); 174 break; 175 case CREATED: 176 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.CREATED); 177 break; 178 case NOCONTENT: 179 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.NOCONTENT); 180 break; 181 case NOTMODIFIED: 182 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 183 break; 184 case BAD: 185 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.BADREQUEST); 186 break; 187 case FORBIDDEN: 188 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.FORBIDDEN); 189 break; 190 case NOTFOUND: 191 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.NOTFOUND); 192 break; 193 case METHODNOTALLOWED: 194 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 195 break; 196 case CONFLICT: 197 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.CONFLICT); 198 break; 199 case GONE: 200 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.GONE); 201 break; 202 case PRECONDITIONFAILED: 203 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 204 break; 205 case UNPROCESSABLE: 206 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.UNPROCESSABLECONTENT); 207 break; 208 default: 209 tgt.setValue(org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes.NULL); 210 break; 211 } 212 } 213 return tgt; 214 } 215 216 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 Enumeration<TestScript.AssertionResponseTypes> tgt = new Enumeration<>(new TestScript.AssertionResponseTypesEnumFactory()); 220 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 221 if (src.getValue() == null) { 222 tgt.setValue(null); 223 } else { 224 switch (src.getValue()) { 225 case OKAY: 226 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 227 break; 228 case CREATED: 229 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 230 break; 231 case NOCONTENT: 232 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 233 break; 234 case NOTMODIFIED: 235 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 236 break; 237 case BADREQUEST: 238 tgt.setValue(TestScript.AssertionResponseTypes.BAD); 239 break; 240 case FORBIDDEN: 241 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 242 break; 243 case NOTFOUND: 244 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 245 break; 246 case METHODNOTALLOWED: 247 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 248 break; 249 case CONFLICT: 250 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 251 break; 252 case GONE: 253 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 254 break; 255 case PRECONDITIONFAILED: 256 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 257 break; 258 case UNPROCESSABLECONTENT: 259 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLE); 260 break; 261 default: 262 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 263 break; 264 } 265 } 266 return tgt; 267 } 268 269 static public String convertContentType(org.hl7.fhir.dstu2.model.TestScript.ContentType src) throws FHIRException { 270 if (src == null) 271 return null; 272 switch (src) { 273 case XML: 274 return "application/fhir+xml"; 275 case JSON: 276 return "application/fhir+json"; 277 default: 278 return null; 279 } 280 } 281 282 static public org.hl7.fhir.dstu2.model.TestScript.ContentType convertContentType(String src) throws FHIRException { 283 if (src == null) 284 return null; 285 if (src.contains("xml")) 286 return org.hl7.fhir.dstu2.model.TestScript.ContentType.XML; 287 if (src.contains("json")) 288 return org.hl7.fhir.dstu2.model.TestScript.ContentType.JSON; 289 return org.hl7.fhir.dstu2.model.TestScript.ContentType.NULL; 290 } 291 292 public static org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent src) throws FHIRException { 293 if (src == null || src.isEmpty()) 294 return null; 295 org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent(); 296 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 297 if (src.hasLabelElement()) 298 tgt.setLabelElement(String10_50.convertString(src.getLabelElement())); 299 if (src.hasDescriptionElement()) 300 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 301 if (src.hasDirection()) 302 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 303 if (src.hasCompareToSourceIdElement()) 304 tgt.setCompareToSourceIdElement(String10_50.convertString(src.getCompareToSourceIdElement())); 305 if (src.hasCompareToSourcePathElement()) 306 tgt.setCompareToSourcePathElement(String10_50.convertString(src.getCompareToSourcePathElement())); 307 if (src.hasContentType()) 308 tgt.setContentType(convertContentType(src.getContentType())); 309 if (src.hasHeaderFieldElement()) 310 tgt.setHeaderFieldElement(String10_50.convertString(src.getHeaderFieldElement())); 311 if (src.hasMinimumIdElement()) 312 tgt.setMinimumIdElement(String10_50.convertString(src.getMinimumIdElement())); 313 if (src.hasNavigationLinksElement()) 314 tgt.setNavigationLinksElement(Boolean10_50.convertBoolean(src.getNavigationLinksElement())); 315 if (src.hasOperator()) 316 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 317 if (src.hasPathElement()) 318 tgt.setPathElement(String10_50.convertString(src.getPathElement())); 319 if (src.hasResource()) 320 tgt.setResource(src.getResource()); 321 if (src.hasResponse()) 322 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 323 if (src.hasResponseCodeElement()) 324 tgt.setResponseCodeElement(String10_50.convertString(src.getResponseCodeElement())); 325 if (src.hasSourceIdElement()) 326 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 327 if (src.hasValidateProfileIdElement()) 328 tgt.setValidateProfileIdElement(Id10_50.convertId(src.getValidateProfileIdElement())); 329 if (src.hasValueElement()) 330 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 331 if (src.hasWarningOnlyElement()) 332 tgt.setWarningOnlyElement(Boolean10_50.convertBoolean(src.getWarningOnlyElement())); 333 return tgt; 334 } 335 336 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.r5.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 337 if (src == null || src.isEmpty()) 338 return null; 339 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionAssertComponent(); 340 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 341 if (src.hasLabelElement()) 342 tgt.setLabelElement(String10_50.convertString(src.getLabelElement())); 343 if (src.hasDescriptionElement()) 344 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 345 if (src.hasDirection()) 346 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 347 if (src.hasCompareToSourceIdElement()) 348 tgt.setCompareToSourceIdElement(String10_50.convertString(src.getCompareToSourceIdElement())); 349 if (src.hasCompareToSourcePathElement()) 350 tgt.setCompareToSourcePathElement(String10_50.convertString(src.getCompareToSourcePathElement())); 351 if (src.hasContentType()) 352 tgt.setContentType(convertContentType(src.getContentType())); 353 if (src.hasHeaderFieldElement()) 354 tgt.setHeaderFieldElement(String10_50.convertString(src.getHeaderFieldElement())); 355 if (src.hasMinimumIdElement()) 356 tgt.setMinimumIdElement(String10_50.convertString(src.getMinimumIdElement())); 357 if (src.hasNavigationLinksElement()) 358 tgt.setNavigationLinksElement(Boolean10_50.convertBoolean(src.getNavigationLinksElement())); 359 if (src.hasOperator()) 360 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 361 if (src.hasPathElement()) 362 tgt.setPathElement(String10_50.convertString(src.getPathElement())); 363 tgt.setResource(src.getResource()); 364 if (src.hasResponse()) 365 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 366 if (src.hasResponseCodeElement()) 367 tgt.setResponseCodeElement(String10_50.convertString(src.getResponseCodeElement())); 368 if (src.hasSourceIdElement()) 369 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 370 if (src.hasValidateProfileIdElement()) 371 tgt.setValidateProfileIdElement(Id10_50.convertId(src.getValidateProfileIdElement())); 372 if (src.hasValueElement()) 373 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 374 if (src.hasWarningOnlyElement()) 375 tgt.setWarningOnlyElement(Boolean10_50.convertBoolean(src.getWarningOnlyElement())); 376 return tgt; 377 } 378 379 public static org.hl7.fhir.r5.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent src) throws FHIRException { 380 if (src == null || src.isEmpty()) 381 return null; 382 org.hl7.fhir.r5.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionComponent(); 383 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 384 if (src.hasOperation()) 385 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 386 if (src.hasAssert()) 387 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 388 return tgt; 389 } 390 391 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent convertSetupActionComponent(org.hl7.fhir.r5.model.TestScript.SetupActionComponent src) throws FHIRException { 392 if (src == null || src.isEmpty()) 393 return null; 394 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent(); 395 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 396 if (src.hasOperation()) 397 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 398 if (src.hasAssert()) 399 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 400 return tgt; 401 } 402 403 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 404 if (src == null || src.isEmpty()) 405 return null; 406 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent(); 407 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 408 if (src.hasType()) 409 tgt.setType(Coding10_50.convertCoding(src.getType())); 410 tgt.setResource(src.getResource()); 411 if (src.hasLabelElement()) 412 tgt.setLabelElement(String10_50.convertString(src.getLabelElement())); 413 if (src.hasDescriptionElement()) 414 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 415 if (src.hasAccept()) 416 tgt.setAccept(convertContentType(src.getAccept())); 417 if (src.hasContentType()) 418 tgt.setContentType(convertContentType(src.getContentType())); 419 if (src.hasDestinationElement()) 420 tgt.setDestinationElement(Integer10_50.convertInteger(src.getDestinationElement())); 421 if (src.hasEncodeRequestUrlElement()) 422 tgt.setEncodeRequestUrlElement(Boolean10_50.convertBoolean(src.getEncodeRequestUrlElement())); 423 if (src.hasParamsElement()) 424 tgt.setParamsElement(String10_50.convertString(src.getParamsElement())); 425 for (org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 426 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 427 if (src.hasResponseIdElement()) 428 tgt.setResponseIdElement(Id10_50.convertId(src.getResponseIdElement())); 429 if (src.hasSourceIdElement()) 430 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 431 if (src.hasTargetId()) 432 tgt.setTargetId(src.getTargetId()); 433 if (src.hasUrlElement()) 434 tgt.setUrlElement(String10_50.convertString(src.getUrlElement())); 435 return tgt; 436 } 437 438 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationComponent src) throws FHIRException { 439 if (src == null || src.isEmpty()) 440 return null; 441 org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationComponent(); 442 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 443 if (src.hasType()) 444 tgt.setType(Coding10_50.convertCoding(src.getType())); 445 if (src.hasResource()) 446 tgt.setResource(src.getResource()); 447 if (src.hasLabelElement()) 448 tgt.setLabelElement(String10_50.convertString(src.getLabelElement())); 449 if (src.hasDescriptionElement()) 450 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 451 if (src.hasAccept()) 452 tgt.setAccept(convertContentType(src.getAccept())); 453 if (src.hasContentType()) 454 tgt.setContentType(convertContentType(src.getContentType())); 455 if (src.hasDestinationElement()) 456 tgt.setDestinationElement(Integer10_50.convertInteger(src.getDestinationElement())); 457 if (src.hasEncodeRequestUrlElement()) 458 tgt.setEncodeRequestUrlElement(Boolean10_50.convertBoolean(src.getEncodeRequestUrlElement())); 459 if (src.hasParamsElement()) 460 tgt.setParamsElement(String10_50.convertString(src.getParamsElement())); 461 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 462 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 463 if (src.hasResponseIdElement()) 464 tgt.setResponseIdElement(Id10_50.convertId(src.getResponseIdElement())); 465 if (src.hasSourceIdElement()) 466 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 467 if (src.hasTargetId()) 468 tgt.setTargetId(src.getTargetId()); 469 if (src.hasUrlElement()) 470 tgt.setUrlElement(String10_50.convertString(src.getUrlElement())); 471 return tgt; 472 } 473 474 public static org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent src) throws FHIRException { 475 if (src == null || src.isEmpty()) 476 return null; 477 org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent(); 478 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 479 if (src.hasFieldElement()) 480 tgt.setFieldElement(String10_50.convertString(src.getFieldElement())); 481 if (src.hasValueElement()) 482 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 483 return tgt; 484 } 485 486 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.r5.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 487 if (src == null || src.isEmpty()) 488 return null; 489 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionOperationRequestHeaderComponent(); 490 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 491 if (src.hasFieldElement()) 492 tgt.setFieldElement(String10_50.convertString(src.getFieldElement())); 493 if (src.hasValueElement()) 494 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 495 return tgt; 496 } 497 498 public static org.hl7.fhir.r5.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent src) throws FHIRException { 499 if (src == null || src.isEmpty()) 500 return null; 501 org.hl7.fhir.r5.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TeardownActionComponent(); 502 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 503 if (src.hasOperation()) 504 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 505 return tgt; 506 } 507 508 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.r5.model.TestScript.TeardownActionComponent src) throws FHIRException { 509 if (src == null || src.isEmpty()) 510 return null; 511 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent(); 512 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 513 if (src.hasOperation()) 514 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 515 return tgt; 516 } 517 518 public static org.hl7.fhir.r5.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent src) throws FHIRException { 519 if (src == null || src.isEmpty()) 520 return null; 521 org.hl7.fhir.r5.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestActionComponent(); 522 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 523 if (src.hasOperation()) 524 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 525 if (src.hasAssert()) 526 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 527 return tgt; 528 } 529 530 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent convertTestActionComponent(org.hl7.fhir.r5.model.TestScript.TestActionComponent src) throws FHIRException { 531 if (src == null || src.isEmpty()) 532 return null; 533 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent(); 534 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 535 if (src.hasOperation()) 536 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 537 if (src.hasAssert()) 538 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 539 return tgt; 540 } 541 542 public static org.hl7.fhir.r5.model.TestScript convertTestScript(org.hl7.fhir.dstu2.model.TestScript src) throws FHIRException { 543 if (src == null || src.isEmpty()) 544 return null; 545 org.hl7.fhir.r5.model.TestScript tgt = new org.hl7.fhir.r5.model.TestScript(); 546 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 547 if (src.hasUrlElement()) 548 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 549 if (src.hasVersionElement()) 550 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 551 if (src.hasNameElement()) 552 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 553 if (src.hasStatus()) 554 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 555 if (src.hasIdentifier()) 556 tgt.addIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 557 if (src.hasExperimental()) 558 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 559 if (src.hasPublisherElement()) 560 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 561 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent t : src.getContact()) 562 tgt.addContact(convertTestScriptContactComponent(t)); 563 if (src.hasDate()) 564 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 565 if (src.hasDescription()) 566 tgt.setDescription(src.getDescription()); 567 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 568 if (VersionConvertor_10_50.isJurisdiction(t)) 569 tgt.addJurisdiction(CodeableConcept10_50.convertCodeableConcept(t)); 570 else 571 tgt.addUseContext(CodeableConcept10_50.convertCodeableConceptToUsageContext(t)); 572 if (src.hasRequirements()) 573 tgt.setPurpose(src.getRequirements()); 574 if (src.hasCopyright()) 575 tgt.setCopyright(src.getCopyright()); 576 if (src.hasMetadata()) 577 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 578 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 579 tgt.addFixture(convertTestScriptFixtureComponent(t)); 580 for (org.hl7.fhir.dstu2.model.Reference t : src.getProfile()) tgt.getProfile().add(Reference10_50.convertReferenceToCanonical(t)); 581 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 582 tgt.addVariable(convertTestScriptVariableComponent(t)); 583 if (src.hasSetup()) 584 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 585 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent t : src.getTest()) 586 tgt.addTest(convertTestScriptTestComponent(t)); 587 if (src.hasTeardown()) 588 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 589 return tgt; 590 } 591 592 public static org.hl7.fhir.dstu2.model.TestScript convertTestScript(org.hl7.fhir.r5.model.TestScript src) throws FHIRException { 593 if (src == null || src.isEmpty()) 594 return null; 595 org.hl7.fhir.dstu2.model.TestScript tgt = new org.hl7.fhir.dstu2.model.TestScript(); 596 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 597 if (src.hasUrlElement()) 598 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 599 if (src.hasVersionElement()) 600 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 601 if (src.hasNameElement()) 602 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 603 if (src.hasStatus()) 604 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 605 if (src.hasIdentifier()) { 606 tgt.setIdentifier(Identifier10_50.convertIdentifier(src.getIdentifierFirstRep())); 607 } 608 if (src.hasExperimental()) 609 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 610 if (src.hasPublisherElement()) 611 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 612 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) tgt.addContact(convertTestScriptContactComponent(t)); 613 if (src.hasDate()) 614 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 615 if (src.hasDescription()) 616 tgt.setDescription(src.getDescription()); 617 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 618 if (t.hasValueCodeableConcept()) 619 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t.getValueCodeableConcept())); 620 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 621 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t)); 622 if (src.hasPurpose()) 623 tgt.setRequirements(src.getPurpose()); 624 if (src.hasCopyright()) 625 tgt.setCopyright(src.getCopyright()); 626 if (src.hasMetadata()) 627 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 628 for (org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 629 tgt.addFixture(convertTestScriptFixtureComponent(t)); 630 for (CanonicalType t : src.getProfile()) tgt.addProfile(Reference10_50.convertCanonicalToReference(t)); 631 for (org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 632 tgt.addVariable(convertTestScriptVariableComponent(t)); 633 if (src.hasSetup()) 634 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 635 for (org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent t : src.getTest()) 636 tgt.addTest(convertTestScriptTestComponent(t)); 637 if (src.hasTeardown()) 638 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 639 return tgt; 640 } 641 642 public static org.hl7.fhir.r5.model.ContactDetail convertTestScriptContactComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent src) throws FHIRException { 643 if (src == null || src.isEmpty()) 644 return null; 645 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 646 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 647 if (src.hasNameElement()) 648 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 649 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 650 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 651 return tgt; 652 } 653 654 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent convertTestScriptContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 655 if (src == null || src.isEmpty()) 656 return null; 657 org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptContactComponent(); 658 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 659 if (src.hasNameElement()) 660 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 661 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 662 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 663 return tgt; 664 } 665 666 public static org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 667 if (src == null || src.isEmpty()) 668 return null; 669 org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent(); 670 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 671 if (src.hasAutocreateElement()) 672 tgt.setAutocreateElement(Boolean10_50.convertBoolean(src.getAutocreateElement())); 673 if (src.hasAutodeleteElement()) 674 tgt.setAutodeleteElement(Boolean10_50.convertBoolean(src.getAutodeleteElement())); 675 if (src.hasResource()) 676 tgt.setResource(Reference10_50.convertReference(src.getResource())); 677 return tgt; 678 } 679 680 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.r5.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 681 if (src == null || src.isEmpty()) 682 return null; 683 org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptFixtureComponent(); 684 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 685 if (src.hasAutocreateElement()) 686 tgt.setAutocreateElement(Boolean10_50.convertBoolean(src.getAutocreateElement())); 687 if (src.hasAutodeleteElement()) 688 tgt.setAutodeleteElement(Boolean10_50.convertBoolean(src.getAutodeleteElement())); 689 if (src.hasResource()) 690 tgt.setResource(Reference10_50.convertReference(src.getResource())); 691 return tgt; 692 } 693 694 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 695 if (src == null || src.isEmpty()) 696 return null; 697 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent(); 698 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 699 if (src.hasRequiredElement()) 700 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 701 if (src.hasValidatedElement()) 702 tgt.setValidatedElement(Boolean10_50.convertBoolean(src.getValidatedElement())); 703 if (src.hasDescriptionElement()) 704 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 705 if (src.hasDestinationElement()) 706 tgt.setDestinationElement(Integer10_50.convertInteger(src.getDestinationElement())); 707 for (org.hl7.fhir.r5.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 708 if (src.hasCapabilitiesElement()) 709 tgt.setConformance(Reference10_50.convertCanonicalToReference(src.getCapabilitiesElement())); 710 return tgt; 711 } 712 713 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 714 if (src == null || src.isEmpty()) 715 return null; 716 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent(); 717 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 718 if (src.hasRequiredElement()) 719 tgt.setRequiredElement(Boolean10_50.convertBoolean(src.getRequiredElement())); 720 if (src.hasValidatedElement()) 721 tgt.setValidatedElement(Boolean10_50.convertBoolean(src.getValidatedElement())); 722 if (src.hasDescriptionElement()) 723 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 724 if (src.hasDestinationElement()) 725 tgt.setDestinationElement(Integer10_50.convertInteger(src.getDestinationElement())); 726 for (org.hl7.fhir.dstu2.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 727 if (src.hasConformance()) 728 tgt.setCapabilitiesElement(Reference10_50.convertReferenceToCanonical(src.getConformance())); 729 return tgt; 730 } 731 732 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 733 if (src == null || src.isEmpty()) 734 return null; 735 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent(); 736 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 737 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 738 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 739 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 740 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 741 return tgt; 742 } 743 744 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 745 if (src == null || src.isEmpty()) 746 return null; 747 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataComponent(); 748 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 749 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 750 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 751 for (org.hl7.fhir.r5.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 752 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 753 return tgt; 754 } 755 756 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 757 if (src == null || src.isEmpty()) 758 return null; 759 org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent(); 760 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 761 if (src.hasUrlElement()) 762 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 763 if (src.hasDescriptionElement()) 764 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 765 return tgt; 766 } 767 768 public static org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 769 if (src == null || src.isEmpty()) 770 return null; 771 org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptMetadataLinkComponent(); 772 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 773 if (src.hasUrlElement()) 774 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 775 if (src.hasDescriptionElement()) 776 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 777 return tgt; 778 } 779 780 public static org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 781 if (src == null || src.isEmpty()) 782 return null; 783 org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent(); 784 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 785 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupActionComponent t : src.getAction()) 786 tgt.addAction(convertSetupActionComponent(t)); 787 return tgt; 788 } 789 790 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.r5.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 791 if (src == null || src.isEmpty()) 792 return null; 793 org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptSetupComponent(); 794 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 795 for (org.hl7.fhir.r5.model.TestScript.SetupActionComponent t : src.getAction()) 796 tgt.addAction(convertSetupActionComponent(t)); 797 return tgt; 798 } 799 800 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 801 if (src == null || src.isEmpty()) 802 return null; 803 org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent(); 804 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 805 for (org.hl7.fhir.r5.model.TestScript.TeardownActionComponent t : src.getAction()) 806 tgt.addAction(convertTeardownActionComponent(t)); 807 return tgt; 808 } 809 810 public static org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 811 if (src == null || src.isEmpty()) 812 return null; 813 org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTeardownComponent(); 814 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 815 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTeardownActionComponent t : src.getAction()) 816 tgt.addAction(convertTeardownActionComponent(t)); 817 return tgt; 818 } 819 820 public static org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent src) throws FHIRException { 821 if (src == null || src.isEmpty()) 822 return null; 823 org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent(); 824 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 825 if (src.hasNameElement()) 826 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 827 if (src.hasDescriptionElement()) 828 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 829 for (org.hl7.fhir.dstu2.model.TestScript.TestScriptTestActionComponent t : src.getAction()) 830 tgt.addAction(convertTestActionComponent(t)); 831 return tgt; 832 } 833 834 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.r5.model.TestScript.TestScriptTestComponent src) throws FHIRException { 835 if (src == null || src.isEmpty()) 836 return null; 837 org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptTestComponent(); 838 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 839 if (src.hasNameElement()) 840 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 841 if (src.hasDescriptionElement()) 842 tgt.setDescriptionElement(String10_50.convertString(src.getDescriptionElement())); 843 for (org.hl7.fhir.r5.model.TestScript.TestActionComponent t : src.getAction()) 844 tgt.addAction(convertTestActionComponent(t)); 845 return tgt; 846 } 847 848 public static org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 849 if (src == null || src.isEmpty()) 850 return null; 851 org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent(); 852 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 853 if (src.hasNameElement()) 854 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 855 if (src.hasHeaderFieldElement()) 856 tgt.setHeaderFieldElement(String10_50.convertString(src.getHeaderFieldElement())); 857 if (src.hasPathElement()) 858 tgt.setPathElement(String10_50.convertString(src.getPathElement())); 859 if (src.hasSourceIdElement()) 860 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 861 return tgt; 862 } 863 864 public static org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu2.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 865 if (src == null || src.isEmpty()) 866 return null; 867 org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.r5.model.TestScript.TestScriptVariableComponent(); 868 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 869 if (src.hasNameElement()) 870 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 871 if (src.hasHeaderFieldElement()) 872 tgt.setHeaderFieldElement(String10_50.convertString(src.getHeaderFieldElement())); 873 if (src.hasPathElement()) 874 tgt.setPathElement(String10_50.convertString(src.getPathElement())); 875 if (src.hasSourceIdElement()) 876 tgt.setSourceIdElement(Id10_50.convertId(src.getSourceIdElement())); 877 return tgt; 878 } 879}