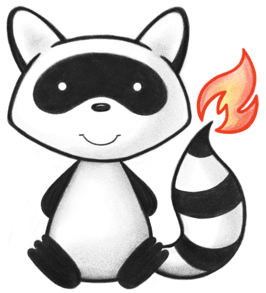
001package org.hl7.fhir.convertors.conv10_50.resources10_50; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_10_50; 007import org.hl7.fhir.convertors.context.ConversionContext10_50; 008import org.hl7.fhir.convertors.conv10_50.VersionConvertor_10_50; 009import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.CodeableConcept10_50; 010import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Coding10_50; 011import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.ContactPoint10_50; 012import org.hl7.fhir.convertors.conv10_50.datatypes10_50.complextypes10_50.Identifier10_50; 013import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Boolean10_50; 014import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Code10_50; 015import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.DateTime10_50; 016import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Integer10_50; 017import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.String10_50; 018import org.hl7.fhir.convertors.conv10_50.datatypes10_50.primitivetypes10_50.Uri10_50; 019import org.hl7.fhir.dstu2.model.Enumeration; 020import org.hl7.fhir.dstu2.model.ValueSet; 021import org.hl7.fhir.exceptions.FHIRException; 022import org.hl7.fhir.r5.model.BooleanType; 023import org.hl7.fhir.r5.model.CodeSystem; 024import org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent; 025import org.hl7.fhir.r5.model.Enumerations; 026import org.hl7.fhir.r5.model.Enumerations.CodeSystemContentMode; 027import org.hl7.fhir.r5.terminologies.CodeSystemUtilities; 028 029public class ValueSet10_50 { 030 031 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 032 if (src == null || src.isEmpty()) 033 return null; 034 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent(); 035 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 036 if (src.hasCodeElement()) 037 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 038 if (src.hasDisplayElement()) 039 tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 040 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent t : src.getDesignation()) 041 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 042 return tgt; 043 } 044 045 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 046 if (src == null || src.isEmpty()) 047 return null; 048 org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent(); 049 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 050 if (src.hasCodeElement()) 051 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 052 if (src.hasDisplayElement()) 053 tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 054 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 055 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 056 return tgt; 057 } 058 059 public static org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent src) throws FHIRException { 060 if (src == null || src.isEmpty()) 061 return null; 062 org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent(); 063 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 064 if (src.hasLanguageElement()) 065 tgt.setLanguageElement(Code10_50.convertCode(src.getLanguageElement())); 066 if (src.hasUse()) 067 tgt.setUse(Coding10_50.convertCoding(src.getUse())); 068 if (src.hasValueElement()) 069 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 070 return tgt; 071 } 072 073 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.r5.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 074 if (src == null || src.isEmpty()) 075 return null; 076 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 077 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 078 if (src.hasLanguageElement()) 079 tgt.setLanguageElement(Code10_50.convertCode(src.getLanguageElement())); 080 if (src.hasUse()) 081 tgt.setUse(Coding10_50.convertCoding(src.getUse())); 082 if (src.hasValueElement()) 083 tgt.setValueElement(String10_50.convertString(src.getValueElement())); 084 return tgt; 085 } 086 087 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent src) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent(); 091 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 092 if (src.hasSystemElement()) 093 tgt.setSystemElement(Uri10_50.convertUri(src.getSystemElement())); 094 if (src.hasVersionElement()) 095 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 096 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 097 tgt.addConcept(convertConceptReferenceComponent(t)); 098 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 099 tgt.addFilter(convertConceptSetFilterComponent(t)); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent src) throws FHIRException { 104 if (src == null || src.isEmpty()) 105 return null; 106 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent(); 107 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 108 if (src.hasSystemElement()) 109 tgt.setSystemElement(Uri10_50.convertUri(src.getSystemElement())); 110 if (src.hasVersionElement()) 111 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 112 for (org.hl7.fhir.r5.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 113 tgt.addConcept(convertConceptReferenceComponent(t)); 114 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 115 tgt.addFilter(convertConceptSetFilterComponent(t)); 116 return tgt; 117 } 118 119 public static org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 120 if (src == null || src.isEmpty()) 121 return null; 122 org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent(); 123 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 124 if (src.hasPropertyElement()) 125 tgt.setPropertyElement(Code10_50.convertCode(src.getPropertyElement())); 126 if (src.hasOp()) 127 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 128 if (src.hasValue()) 129 tgt.setValue(src.getValue()); 130 return tgt; 131 } 132 133 public static org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu2.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ConceptSetFilterComponent(); 137 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 138 if (src.hasPropertyElement()) 139 tgt.setPropertyElement(Code10_50.convertCode(src.getPropertyElement())); 140 if (src.hasOp()) 141 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 142 if (src.hasValue()) 143 tgt.setValue(src.getValue()); 144 return tgt; 145 } 146 147 static public org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> src) throws FHIRException { 148 if (src == null || src.isEmpty()) 149 return null; 150 Enumeration<ValueSet.FilterOperator> tgt = new Enumeration<>(new ValueSet.FilterOperatorEnumFactory()); 151 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 152 if (src.getValue() == null) { 153 tgt.setValue(null); 154 } else { 155 switch (src.getValue()) { 156 case EQUAL: 157 tgt.setValue(ValueSet.FilterOperator.EQUAL); 158 break; 159 case ISA: 160 tgt.setValue(ValueSet.FilterOperator.ISA); 161 break; 162 case ISNOTA: 163 tgt.setValue(ValueSet.FilterOperator.ISNOTA); 164 break; 165 case REGEX: 166 tgt.setValue(ValueSet.FilterOperator.REGEX); 167 break; 168 case IN: 169 tgt.setValue(ValueSet.FilterOperator.IN); 170 break; 171 case NOTIN: 172 tgt.setValue(ValueSet.FilterOperator.NOTIN); 173 break; 174 default: 175 tgt.setValue(ValueSet.FilterOperator.NULL); 176 break; 177 } 178 } 179 return tgt; 180 } 181 182 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu2.model.Enumeration<org.hl7.fhir.dstu2.model.ValueSet.FilterOperator> src) throws FHIRException { 183 if (src == null || src.isEmpty()) 184 return null; 185 org.hl7.fhir.r5.model.Enumeration<Enumerations.FilterOperator> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new Enumerations.FilterOperatorEnumFactory()); 186 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 187 if (src.getValue() == null) { 188 tgt.setValue(null); 189 } else { 190 switch (src.getValue()) { 191 case EQUAL: 192 tgt.setValue(Enumerations.FilterOperator.EQUAL); 193 break; 194 case ISA: 195 tgt.setValue(Enumerations.FilterOperator.ISA); 196 break; 197 case ISNOTA: 198 tgt.setValue(Enumerations.FilterOperator.ISNOTA); 199 break; 200 case REGEX: 201 tgt.setValue(Enumerations.FilterOperator.REGEX); 202 break; 203 case IN: 204 tgt.setValue(Enumerations.FilterOperator.IN); 205 break; 206 case NOTIN: 207 tgt.setValue(Enumerations.FilterOperator.NOTIN); 208 break; 209 default: 210 tgt.setValue(Enumerations.FilterOperator.NULL); 211 break; 212 } 213 } 214 return tgt; 215 } 216 217 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.r5.model.ValueSet src, BaseAdvisor_10_50 advisor) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 org.hl7.fhir.dstu2.model.ValueSet tgt = new org.hl7.fhir.dstu2.model.ValueSet(); 221 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 222 if (src.hasUrlElement()) 223 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 224 for (org.hl7.fhir.r5.model.Identifier i : src.getIdentifier()) 225 tgt.setIdentifier(Identifier10_50.convertIdentifier(i)); 226 if (src.hasVersionElement()) 227 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 228 if (src.hasNameElement()) 229 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 230 if (src.hasStatus()) 231 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 232 if (src.hasExperimental()) 233 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 234 if (src.hasPublisherElement()) 235 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 236 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) tgt.addContact(convertValueSetContactComponent(t)); 237 if (src.hasDate()) 238 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 239 tgt.setLockedDate(src.getCompose().getLockedDate()); 240 if (src.hasDescription()) 241 tgt.setDescription(src.getDescription()); 242 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 243 if (t.hasValueCodeableConcept()) 244 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t.getValueCodeableConcept())); 245 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 246 tgt.addUseContext(CodeableConcept10_50.convertCodeableConcept(t)); 247 if (src.hasImmutableElement()) 248 tgt.setImmutableElement(Boolean10_50.convertBoolean(src.getImmutableElement())); 249 if (src.hasPurpose()) 250 tgt.setRequirements(src.getPurpose()); 251 if (src.hasCopyright()) 252 tgt.setCopyright(src.getCopyright()); 253 if (src.hasExtension(VersionConvertorConstants.EXT_VS_EXTENSIBLE)) 254 tgt.setExtensible(((BooleanType) src.getExtensionByUrl(VersionConvertorConstants.EXT_VS_EXTENSIBLE).getValue()).booleanValue()); 255 org.hl7.fhir.r5.model.CodeSystem srcCS = (CodeSystem) src.getUserData("r2-cs"); 256 if (srcCS == null && advisor != null) 257 srcCS = advisor.getCodeSystem(src); 258 if (srcCS != null) { 259 tgt.getCodeSystem().setSystem(srcCS.getUrl()); 260 tgt.getCodeSystem().setVersion(srcCS.getVersion()); 261 tgt.getCodeSystem().setCaseSensitive(srcCS.getCaseSensitive()); 262 for (org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent cs : srcCS.getConcept()) 263 processConcept(tgt.getCodeSystem().getConcept(), cs, srcCS); 264 } 265 if (src.hasCompose()) 266 tgt.setCompose(convertValueSetComposeComponent(src.getCompose(), srcCS == null ? null : srcCS.getUrl())); 267 if (src.hasExpansion()) 268 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 269 return tgt; 270 } 271 272 public static org.hl7.fhir.r5.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src) throws FHIRException { 273 return convertValueSet(src, null); 274 } 275 276 public static org.hl7.fhir.r5.model.ValueSet convertValueSet(org.hl7.fhir.dstu2.model.ValueSet src, BaseAdvisor_10_50 advisor) throws FHIRException { 277 if (src == null || src.isEmpty()) 278 return null; 279 org.hl7.fhir.r5.model.ValueSet tgt = new org.hl7.fhir.r5.model.ValueSet(); 280 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgt); 281 if (src.hasUrlElement()) 282 tgt.setUrlElement(Uri10_50.convertUri(src.getUrlElement())); 283 if (src.hasIdentifier()) 284 tgt.addIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 285 if (src.hasVersionElement()) 286 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 287 if (src.hasNameElement()) 288 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 289 if (src.hasStatus()) 290 tgt.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 291 if (src.hasExperimental()) 292 tgt.setExperimentalElement(Boolean10_50.convertBoolean(src.getExperimentalElement())); 293 if (src.hasPublisherElement()) 294 tgt.setPublisherElement(String10_50.convertString(src.getPublisherElement())); 295 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 296 tgt.addContact(convertValueSetContactComponent(t)); 297 if (src.hasDate()) 298 tgt.setDateElement(DateTime10_50.convertDateTime(src.getDateElement())); 299 if (src.hasDescription()) 300 tgt.setDescription(src.getDescription()); 301 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 302 if (VersionConvertor_10_50.isJurisdiction(t)) 303 tgt.addJurisdiction(CodeableConcept10_50.convertCodeableConcept(t)); 304 else 305 tgt.addUseContext(CodeableConcept10_50.convertCodeableConceptToUsageContext(t)); 306 if (src.hasImmutableElement()) 307 tgt.setImmutableElement(Boolean10_50.convertBoolean(src.getImmutableElement())); 308 if (src.hasRequirements()) 309 tgt.setPurpose(src.getRequirements()); 310 if (src.hasCopyright()) 311 tgt.setCopyright(src.getCopyright()); 312 if (src.hasExtensible()) 313 tgt.addExtension(VersionConvertorConstants.EXT_VS_EXTENSIBLE, new BooleanType(src.getExtensible())); 314 if (src.hasCompose()) { 315 if (src.hasCompose()) 316 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 317 tgt.getCompose().setLockedDate(src.getLockedDate()); 318 } 319 if (src.hasCodeSystem() && advisor != null) { 320 org.hl7.fhir.r5.model.CodeSystem tgtcs = new org.hl7.fhir.r5.model.CodeSystem(); 321 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyDomainResource(src, tgtcs); 322 tgtcs.setUrl(src.getCodeSystem().getSystem()); 323 tgtcs.addIdentifier(Identifier10_50.convertIdentifier(src.getIdentifier())); 324 tgtcs.setVersion(src.getCodeSystem().getVersion()); 325 tgtcs.setName(src.getName() + " Code System"); 326 tgtcs.setStatusElement(Enumerations10_50.convertConformanceResourceStatus(src.getStatusElement())); 327 if (src.hasExperimental()) 328 tgtcs.setExperimental(src.getExperimental()); 329 tgtcs.setPublisher(src.getPublisher()); 330 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent t : src.getContact()) 331 tgtcs.addContact(convertValueSetContactComponent(t)); 332 if (src.hasDate()) 333 tgtcs.setDate(src.getDate()); 334 tgtcs.setDescription(src.getDescription()); 335 for (org.hl7.fhir.dstu2.model.CodeableConcept t : src.getUseContext()) 336 if (VersionConvertor_10_50.isJurisdiction(t)) 337 tgtcs.addJurisdiction(CodeableConcept10_50.convertCodeableConcept(t)); 338 else 339 tgtcs.addUseContext(CodeableConcept10_50.convertCodeableConceptToUsageContext(t)); 340 tgtcs.setPurpose(src.getRequirements()); 341 tgtcs.setCopyright(src.getCopyright()); 342 tgtcs.setContent(CodeSystemContentMode.COMPLETE); 343 tgtcs.setCaseSensitive(src.getCodeSystem().getCaseSensitive()); 344 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs : src.getCodeSystem().getConcept()) 345 processConcept(tgtcs.getConcept(), cs, tgtcs); 346 advisor.handleCodeSystem(tgtcs, tgt); 347 tgt.setUserData("r2-cs", tgtcs); 348 tgt.getCompose().addInclude().setSystem(tgtcs.getUrl()); 349 } 350 if (src.hasExpansion()) 351 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 352 return tgt; 353 } 354 355 public static org.hl7.fhir.dstu2.model.ValueSet convertValueSet(org.hl7.fhir.r5.model.ValueSet src) throws FHIRException { 356 return convertValueSet(src, null); 357 } 358 359 public static org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 360 if (src == null || src.isEmpty()) 361 return null; 362 org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent(); 363 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 364 for (org.hl7.fhir.dstu2.model.UriType t : src.getImport()) tgt.addInclude().addValueSet(t.getValue()); 365 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getInclude()) 366 tgt.addInclude(convertConceptSetComponent(t)); 367 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptSetComponent t : src.getExclude()) 368 tgt.addExclude(convertConceptSetComponent(t)); 369 return tgt; 370 } 371 372 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetComposeComponent src, String noSystem) throws FHIRException { 373 if (src == null || src.isEmpty()) 374 return null; 375 org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetComposeComponent(); 376 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 377 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getInclude()) { 378 for (org.hl7.fhir.r5.model.UriType ti : t.getValueSet()) tgt.addImport(ti.getValue()); 379 if (noSystem == null || !t.getSystem().equals(noSystem)) 380 tgt.addInclude(convertConceptSetComponent(t)); 381 } 382 for (org.hl7.fhir.r5.model.ValueSet.ConceptSetComponent t : src.getExclude()) 383 tgt.addExclude(convertConceptSetComponent(t)); 384 return tgt; 385 } 386 387 public static org.hl7.fhir.r5.model.ContactDetail convertValueSetContactComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent src) throws FHIRException { 388 if (src == null || src.isEmpty()) 389 return null; 390 org.hl7.fhir.r5.model.ContactDetail tgt = new org.hl7.fhir.r5.model.ContactDetail(); 391 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 392 if (src.hasNameElement()) 393 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 394 for (org.hl7.fhir.dstu2.model.ContactPoint t : src.getTelecom()) 395 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 396 return tgt; 397 } 398 399 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent convertValueSetContactComponent(org.hl7.fhir.r5.model.ContactDetail src) throws FHIRException { 400 if (src == null || src.isEmpty()) 401 return null; 402 org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetContactComponent(); 403 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 404 if (src.hasNameElement()) 405 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 406 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 407 tgt.addTelecom(ContactPoint10_50.convertContactPoint(t)); 408 return tgt; 409 } 410 411 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 412 if (src == null || src.isEmpty()) 413 return null; 414 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent(); 415 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 416 if (src.hasIdentifierElement()) 417 tgt.setIdentifierElement(Uri10_50.convertUri(src.getIdentifierElement())); 418 if (src.hasTimestampElement()) 419 tgt.setTimestampElement(DateTime10_50.convertDateTime(src.getTimestampElement())); 420 if (src.hasTotalElement()) 421 tgt.setTotalElement(Integer10_50.convertInteger(src.getTotalElement())); 422 if (src.hasOffsetElement()) 423 tgt.setOffsetElement(Integer10_50.convertInteger(src.getOffsetElement())); 424 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 425 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 426 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 427 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 428 return tgt; 429 } 430 431 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 432 if (src == null || src.isEmpty()) 433 return null; 434 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionComponent(); 435 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 436 if (src.hasIdentifierElement()) 437 tgt.setIdentifierElement(Uri10_50.convertUri(src.getIdentifierElement())); 438 if (src.hasTimestampElement()) 439 tgt.setTimestampElement(DateTime10_50.convertDateTime(src.getTimestampElement())); 440 if (src.hasTotalElement()) 441 tgt.setTotalElement(Integer10_50.convertInteger(src.getTotalElement())); 442 if (src.hasOffsetElement()) 443 tgt.setOffsetElement(Integer10_50.convertInteger(src.getOffsetElement())); 444 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 445 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 446 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 447 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 448 return tgt; 449 } 450 451 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 452 if (src == null || src.isEmpty()) 453 return null; 454 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent(); 455 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 456 if (src.hasSystemElement()) 457 tgt.setSystemElement(Uri10_50.convertUri(src.getSystemElement())); 458 if (src.hasAbstractElement()) 459 tgt.setAbstractElement(Boolean10_50.convertBoolean(src.getAbstractElement())); 460 if (src.hasVersionElement()) 461 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 462 if (src.hasCodeElement()) 463 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 464 if (src.hasDisplayElement()) 465 tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 466 for (org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 467 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 468 return tgt; 469 } 470 471 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 472 if (src == null || src.isEmpty()) 473 return null; 474 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionContainsComponent(); 475 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 476 if (src.hasSystemElement()) 477 tgt.setSystemElement(Uri10_50.convertUri(src.getSystemElement())); 478 if (src.hasAbstractElement()) 479 tgt.setAbstractElement(Boolean10_50.convertBoolean(src.getAbstractElement())); 480 if (src.hasVersionElement()) 481 tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 482 if (src.hasCodeElement()) 483 tgt.setCodeElement(Code10_50.convertCode(src.getCodeElement())); 484 if (src.hasDisplayElement()) 485 tgt.setDisplayElement(String10_50.convertString(src.getDisplayElement())); 486 for (org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 487 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 488 return tgt; 489 } 490 491 public static org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 492 if (src == null || src.isEmpty()) 493 return null; 494 org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent(); 495 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 496 if (src.hasNameElement()) 497 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 498 if (src.hasValue()) 499 tgt.setValue(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getValue())); 500 return tgt; 501 } 502 503 public static org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu2.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 504 if (src == null || src.isEmpty()) 505 return null; 506 org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.r5.model.ValueSet.ValueSetExpansionParameterComponent(); 507 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 508 if (src.hasNameElement()) 509 tgt.setNameElement(String10_50.convertString(src.getNameElement())); 510 if (src.hasValue()) 511 tgt.setValue(ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().convertType(src.getValue())); 512 return tgt; 513 } 514 515 static public void processConcept(List<ValueSet.ConceptDefinitionComponent> concepts, ConceptDefinitionComponent cs, CodeSystem srcCS) throws FHIRException { 516 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent ct = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent(); 517 concepts.add(ct); 518 ct.setCode(cs.getCode()); 519 ct.setDisplay(cs.getDisplay()); 520 ct.setDefinition(cs.getDefinition()); 521 if (CodeSystemUtilities.isNotSelectable(srcCS, cs)) 522 ct.setAbstract(true); 523 for (org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 524 org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent(); 525 cst.setLanguage(csd.getLanguage()); 526 cst.setUse(Coding10_50.convertCoding(csd.getUse())); 527 cst.setValue(csd.getValue()); 528 } 529 for (ConceptDefinitionComponent csc : cs.getConcept()) processConcept(ct.getConcept(), csc, srcCS); 530 } 531 532 static public void processConcept(List<ConceptDefinitionComponent> concepts, org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent cs, CodeSystem tgtcs) throws FHIRException { 533 org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent ct = new org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent(); 534 concepts.add(ct); 535 ct.setCode(cs.getCode()); 536 ct.setDisplay(cs.getDisplay()); 537 ct.setDefinition(cs.getDefinition()); 538 if (cs.getAbstract()) 539 CodeSystemUtilities.setNotSelectable(tgtcs, ct); 540 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionDesignationComponent csd : cs.getDesignation()) { 541 org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent cst = new org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent(); 542 cst.setLanguage(csd.getLanguage()); 543 cst.setUse(Coding10_50.convertCoding(csd.getUse())); 544 cst.setValue(csd.getValue()); 545 } 546 for (org.hl7.fhir.dstu2.model.ValueSet.ConceptDefinitionComponent csc : cs.getConcept()) 547 processConcept(ct.getConcept(), csc, tgtcs); 548 } 549 550 public static ValueSet.ValueSetCodeSystemComponent convertCodeSystem(CodeSystem src) throws FHIRException { 551 if (src == null || src.isEmpty()) return null; 552 ValueSet.ValueSetCodeSystemComponent tgt = new ValueSet.ValueSetCodeSystemComponent(); 553 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyElement(src, tgt); 554 if (src.hasUrlElement()) tgt.setSystemElement(Uri10_50.convertUri(src.getUrlElement())); 555 if (src.hasVersionElement()) tgt.setVersionElement(String10_50.convertString(src.getVersionElement())); 556 if (src.hasCaseSensitiveElement()) 557 tgt.setCaseSensitiveElement(Boolean10_50.convertBoolean(src.getCaseSensitiveElement())); 558 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(src, cc)); 559 return tgt; 560 } 561 562 public static ValueSet.ConceptDefinitionComponent convertCodeSystemConcept(CodeSystem cs, ConceptDefinitionComponent src) throws FHIRException { 563 if (src == null || src.isEmpty()) return null; 564 ValueSet.ConceptDefinitionComponent tgt = new ValueSet.ConceptDefinitionComponent(); 565 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 566 tgt.setAbstract(CodeSystemUtilities.isNotSelectable(cs, src)); 567 if (src.hasCode()) tgt.setCode(src.getCode()); 568 if (src.hasDefinition()) tgt.setDefinition(src.getDefinition()); 569 if (src.hasDisplay()) tgt.setDisplay(src.getDisplay()); 570 for (ConceptDefinitionComponent cc : src.getConcept()) tgt.addConcept(convertCodeSystemConcept(cs, cc)); 571 for (CodeSystem.ConceptDefinitionDesignationComponent cc : src.getDesignation()) 572 tgt.addDesignation(convertCodeSystemDesignation(cc)); 573 return tgt; 574 } 575 576 public static ValueSet.ConceptDefinitionDesignationComponent convertCodeSystemDesignation(CodeSystem.ConceptDefinitionDesignationComponent src) throws FHIRException { 577 if (src == null || src.isEmpty()) return null; 578 ValueSet.ConceptDefinitionDesignationComponent tgt = new ValueSet.ConceptDefinitionDesignationComponent(); 579 ConversionContext10_50.INSTANCE.getVersionConvertor_10_50().copyBackboneElement(src,tgt); 580 if (src.hasUse()) tgt.setUse(Coding10_50.convertCoding(src.getUse())); 581 if (src.hasLanguage()) tgt.setLanguage(src.getLanguage()); 582 if (src.hasValue()) tgt.setValue(src.getValue()); 583 return tgt; 584 } 585}