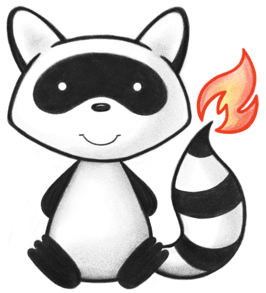
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Id14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Integer14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.MarkDown14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 014import org.hl7.fhir.convertors.conv14_30.resources14_30.Enumerations14_30; 015import org.hl7.fhir.dstu3.conformance.ProfileUtilities; 016import org.hl7.fhir.dstu3.model.ElementDefinition; 017import org.hl7.fhir.exceptions.FHIRException; 018 019public class ElementDefinition14_30 { 020 public static org.hl7.fhir.dstu3.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu2016may.model.ElementDefinition src) throws FHIRException { 021 if (src == null || src.isEmpty()) return null; 022 org.hl7.fhir.dstu3.model.ElementDefinition tgt = new org.hl7.fhir.dstu3.model.ElementDefinition(); 023 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 024 if (src.hasPathElement()) tgt.setPathElement(String14_30.convertString(src.getPathElement())); 025 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition14_30::convertPropertyRepresentation).collect(Collectors.toList())); 026 if (src.hasName()) tgt.setSliceNameElement(String14_30.convertString(src.getNameElement())); 027 if (src.hasLabel()) tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 028 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getCode()) tgt.addCode(Code14_30.convertCoding(t)); 029 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 030 if (src.hasShort()) tgt.setShortElement(String14_30.convertString(src.getShortElement())); 031 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown14_30.convertMarkdown(src.getDefinitionElement())); 032 if (src.hasComments()) tgt.setCommentElement(MarkDown14_30.convertMarkdown(src.getCommentsElement())); 033 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown14_30.convertMarkdown(src.getRequirementsElement())); 034 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 035 if (src.hasMin()) tgt.setMin(src.getMin()); 036 if (src.hasMax()) tgt.setMaxElement(String14_30.convertString(src.getMaxElement())); 037 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 038 if (src.hasContentReference()) 039 tgt.setContentReferenceElement(Uri14_30.convertUri(src.getContentReferenceElement())); 040 for (org.hl7.fhir.dstu2016may.model.ElementDefinition.TypeRefComponent t : src.getType()) 041 tgt.addType(convertTypeRefComponent(t)); 042 if (src.hasDefaultValue()) 043 tgt.setDefaultValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getDefaultValue())); 044 if (src.hasMeaningWhenMissing()) 045 tgt.setMeaningWhenMissingElement(MarkDown14_30.convertMarkdown(src.getMeaningWhenMissingElement())); 046 if (src.hasFixed()) 047 tgt.setFixed(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getFixed())); 048 if (src.hasPattern()) 049 tgt.setPattern(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getPattern())); 050 if (src.hasExample()) 051 tgt.addExample().setLabel("General").setValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getExample())); 052 if (src.hasMinValue()) 053 tgt.setMinValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getMinValue())); 054 if (src.hasMaxValue()) 055 tgt.setMaxValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getMaxValue())); 056 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer14_30.convertInteger(src.getMaxLengthElement())); 057 for (org.hl7.fhir.dstu2016may.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 058 for (org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 059 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 060 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean14_30.convertBoolean(src.getMustSupportElement())); 061 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean14_30.convertBoolean(src.getIsModifierElement())); 062 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean14_30.convertBoolean(src.getIsSummaryElement())); 063 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 064 for (org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 065 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.dstu2016may.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu3.model.ElementDefinition src) throws FHIRException { 070 if (src == null || src.isEmpty()) return null; 071 org.hl7.fhir.dstu2016may.model.ElementDefinition tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition(); 072 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 073 if (src.hasPathElement()) tgt.setPathElement(String14_30.convertString(src.getPathElement())); 074 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition14_30::convertPropertyRepresentation).collect(Collectors.toList())); 075 if (src.hasSliceName()) tgt.setNameElement(String14_30.convertString(src.getSliceNameElement())); 076 if (src.hasLabel()) tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 077 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addCode(Code14_30.convertCoding(t)); 078 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 079 if (src.hasShort()) tgt.setShortElement(String14_30.convertString(src.getShortElement())); 080 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown14_30.convertMarkdown(src.getDefinitionElement())); 081 if (src.hasComment()) tgt.setCommentsElement(MarkDown14_30.convertMarkdown(src.getCommentElement())); 082 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown14_30.convertMarkdown(src.getRequirementsElement())); 083 for (org.hl7.fhir.dstu3.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 084 if (src.hasMin()) tgt.setMin(src.getMin()); 085 if (src.hasMax()) tgt.setMaxElement(String14_30.convertString(src.getMaxElement())); 086 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 087 if (src.hasContentReference()) 088 tgt.setContentReferenceElement(Uri14_30.convertUri(src.getContentReferenceElement())); 089 for (org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent t : src.getType()) 090 tgt.addType(convertTypeRefComponent(t)); 091 if (src.hasDefaultValue()) 092 tgt.setDefaultValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getDefaultValue())); 093 if (src.hasMeaningWhenMissing()) 094 tgt.setMeaningWhenMissingElement(MarkDown14_30.convertMarkdown(src.getMeaningWhenMissingElement())); 095 if (src.hasFixed()) 096 tgt.setFixed(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getFixed())); 097 if (src.hasPattern()) 098 tgt.setPattern(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getPattern())); 099 if (src.hasExample()) 100 tgt.setExample(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getExample().get(0).getValue())); 101 if (src.hasMinValue()) 102 tgt.setMinValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getMinValue())); 103 if (src.hasMaxValue()) 104 tgt.setMaxValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getMaxValue())); 105 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer14_30.convertInteger(src.getMaxLengthElement())); 106 for (org.hl7.fhir.dstu3.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 107 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 108 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 109 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean14_30.convertBoolean(src.getMustSupportElement())); 110 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean14_30.convertBoolean(src.getIsModifierElement())); 111 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean14_30.convertBoolean(src.getIsSummaryElement())); 112 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 113 for (org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 114 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 115 return tgt; 116 } 117 118 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 119 if (src == null || src.isEmpty()) return null; 120 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentationEnumFactory()); 121 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 122 if (src.getValue() == null) { 123 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.NULL); 124 } else { 125 switch (src.getValue()) { 126 case XMLATTR: 127 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLATTR); 128 break; 129 case XMLTEXT: 130 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 131 break; 132 case TYPEATTR: 133 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 134 break; 135 case CDATEXT: 136 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.CDATEXT); 137 break; 138 default: 139 tgt.setValue(org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation.NULL); 140 break; 141 } 142 } 143 return tgt; 144 } 145 146 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 147 if (src == null || src.isEmpty()) return null; 148 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentationEnumFactory()); 149 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 150 if (src.getValue() == null) { 151 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.NULL); 152 } else { 153 switch (src.getValue()) { 154 case XMLATTR: 155 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.XMLATTR); 156 break; 157 case XMLTEXT: 158 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 159 break; 160 case TYPEATTR: 161 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 162 break; 163 case CDATEXT: 164 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.CDATEXT); 165 break; 166 default: 167 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.PropertyRepresentation.NULL); 168 break; 169 } 170 } 171 return tgt; 172 } 173 174 public static org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 175 if (src == null || src.isEmpty()) return null; 176 org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent(); 177 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 178 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getDiscriminator()) 179 tgt.addDiscriminator(ProfileUtilities.interpretR2Discriminator(t.getValue())); 180 if (src.hasDescription()) tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 181 if (src.hasOrdered()) tgt.setOrderedElement(Boolean14_30.convertBoolean(src.getOrderedElement())); 182 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 183 return tgt; 184 } 185 186 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.dstu3.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 187 if (src == null || src.isEmpty()) return null; 188 org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionSlicingComponent(); 189 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 190 for (ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 191 tgt.addDiscriminator(ProfileUtilities.buildR2Discriminator(t)); 192 if (src.hasDescription()) tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 193 if (src.hasOrdered()) tgt.setOrderedElement(Boolean14_30.convertBoolean(src.getOrderedElement())); 194 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 195 return tgt; 196 } 197 198 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules> src) throws FHIRException { 199 if (src == null || src.isEmpty()) return null; 200 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.SlicingRulesEnumFactory()); 201 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 202 if (src.getValue() == null) { 203 tgt.setValue(ElementDefinition.SlicingRules.NULL); 204 } else { 205 switch (src.getValue()) { 206 case CLOSED: 207 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 208 break; 209 case OPEN: 210 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 211 break; 212 case OPENATEND: 213 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 214 break; 215 default: 216 tgt.setValue(ElementDefinition.SlicingRules.NULL); 217 break; 218 } 219 } 220 return tgt; 221 } 222 223 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.SlicingRules> src) throws FHIRException { 224 if (src == null || src.isEmpty()) return null; 225 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRulesEnumFactory()); 226 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 227 if (src.getValue() == null) { 228 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules.NULL); 229 } else { 230 switch (src.getValue()) { 231 case CLOSED: 232 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules.CLOSED); 233 break; 234 case OPEN: 235 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules.OPEN); 236 break; 237 case OPENATEND: 238 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules.OPENATEND); 239 break; 240 default: 241 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.SlicingRules.NULL); 242 break; 243 } 244 } 245 return tgt; 246 } 247 248 public static ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 249 if (src == null || src.isEmpty()) return null; 250 ElementDefinition.ElementDefinitionBaseComponent tgt = new ElementDefinition.ElementDefinitionBaseComponent(); 251 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 252 if (src.hasPathElement()) tgt.setPathElement(String14_30.convertString(src.getPathElement())); 253 tgt.setMin(src.getMin()); 254 if (src.hasMaxElement()) tgt.setMaxElement(String14_30.convertString(src.getMaxElement())); 255 return tgt; 256 } 257 258 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 259 if (src == null || src.isEmpty()) return null; 260 org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBaseComponent(); 261 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 262 if (src.hasPathElement()) tgt.setPathElement(String14_30.convertString(src.getPathElement())); 263 tgt.setMin(src.getMin()); 264 if (src.hasMaxElement()) tgt.setMaxElement(String14_30.convertString(src.getMaxElement())); 265 return tgt; 266 } 267 268 public static ElementDefinition.TypeRefComponent convertTypeRefComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.TypeRefComponent src) throws FHIRException { 269 if (src == null || src.isEmpty()) return null; 270 ElementDefinition.TypeRefComponent tgt = new ElementDefinition.TypeRefComponent(); 271 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 272 tgt.setCode(src.getCode()); 273 for (org.hl7.fhir.dstu2016may.model.UriType u : src.getProfile()) { 274 if (src.getCode().equals("Reference")) tgt.setTargetProfile(u.getValue()); 275 else tgt.setProfile(u.getValue()); 276 } 277 for (org.hl7.fhir.dstu2016may.model.Extension t : src.getExtensionsByUrl(VersionConvertorConstants.PROFILE_EXTENSION)) { 278 String s = ((org.hl7.fhir.dstu2016may.model.PrimitiveType<String>) t.getValue()).getValue(); 279 tgt.setProfile(s); 280 } 281 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition14_30::convertAggregationMode).collect(Collectors.toList())); 282 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 283 return tgt; 284 } 285 286 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.TypeRefComponent convertTypeRefComponent(ElementDefinition.TypeRefComponent src) throws FHIRException { 287 if (src == null || src.isEmpty()) return null; 288 org.hl7.fhir.dstu2016may.model.ElementDefinition.TypeRefComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.TypeRefComponent(); 289 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 290 tgt.setCode(src.getCode()); 291 if (src.hasTarget()) { 292 if (src.hasTargetProfile()) { 293 tgt.addProfile(src.getTargetProfile()); 294 } 295 if (src.hasProfile()) { 296 if (src.getCode().equals("Reference")) { 297 org.hl7.fhir.dstu2016may.model.Extension t = new org.hl7.fhir.dstu2016may.model.Extension(VersionConvertorConstants.PROFILE_EXTENSION); 298 t.setValue(new org.hl7.fhir.dstu2016may.model.StringType(src.getProfile())); 299 tgt.addExtension(t); 300 } else tgt.addProfile(src.getProfile()); 301 } 302 } else tgt.addProfile(src.getProfile()); 303 tgt.setAggregation(src.getAggregation().stream().map(ElementDefinition14_30::convertAggregationMode).collect(Collectors.toList())); 304 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 305 return tgt; 306 } 307 308 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode> src) throws FHIRException { 309 if (src == null || src.isEmpty()) return null; 310 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 311 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 312 if (src.getValue() == null) { 313 tgt.setValue(ElementDefinition.AggregationMode.NULL); 314 } else { 315 switch (src.getValue()) { 316 case CONTAINED: 317 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 318 break; 319 case REFERENCED: 320 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 321 break; 322 case BUNDLED: 323 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 324 break; 325 default: 326 tgt.setValue(ElementDefinition.AggregationMode.NULL); 327 break; 328 } 329 } 330 return tgt; 331 } 332 333 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 334 if (src == null || src.isEmpty()) return null; 335 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationModeEnumFactory()); 336 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 337 if (src.getValue() == null) { 338 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode.NULL); 339 } else { 340 switch (src.getValue()) { 341 case CONTAINED: 342 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode.CONTAINED); 343 break; 344 case REFERENCED: 345 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode.REFERENCED); 346 break; 347 case BUNDLED: 348 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode.BUNDLED); 349 break; 350 default: 351 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.AggregationMode.NULL); 352 break; 353 } 354 } 355 return tgt; 356 } 357 358 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 359 if (src == null || src.isEmpty()) return null; 360 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.ReferenceVersionRulesEnumFactory()); 361 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 362 if (src.getValue() == null) { 363 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 364 } else { 365 switch (src.getValue()) { 366 case EITHER: 367 tgt.setValue(ElementDefinition.ReferenceVersionRules.EITHER); 368 break; 369 case INDEPENDENT: 370 tgt.setValue(ElementDefinition.ReferenceVersionRules.INDEPENDENT); 371 break; 372 case SPECIFIC: 373 tgt.setValue(ElementDefinition.ReferenceVersionRules.SPECIFIC); 374 break; 375 default: 376 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 377 break; 378 } 379 } 380 return tgt; 381 } 382 383 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 384 if (src == null || src.isEmpty()) return null; 385 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 386 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 387 if (src.getValue() == null) { 388 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules.NULL); 389 } else { 390 switch (src.getValue()) { 391 case EITHER: 392 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules.EITHER); 393 break; 394 case INDEPENDENT: 395 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules.INDEPENDENT); 396 break; 397 case SPECIFIC: 398 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules.SPECIFIC); 399 break; 400 default: 401 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ReferenceVersionRules.NULL); 402 break; 403 } 404 } 405 return tgt; 406 } 407 408 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 409 if (src == null || src.isEmpty()) return null; 410 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 411 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 412 if (src.hasKeyElement()) tgt.setKeyElement(Id14_30.convertId(src.getKeyElement())); 413 if (src.hasRequirements()) tgt.setRequirementsElement(String14_30.convertString(src.getRequirementsElement())); 414 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 415 if (src.hasHumanElement()) tgt.setHumanElement(String14_30.convertString(src.getHumanElement())); 416 if (src.hasExpression()) tgt.setExpressionElement(String14_30.convertString(src.getExpressionElement())); 417 if (src.hasXpathElement()) tgt.setXpathElement(String14_30.convertString(src.getXpathElement())); 418 return tgt; 419 } 420 421 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 422 if (src == null || src.isEmpty()) return null; 423 org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionConstraintComponent(); 424 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 425 if (src.hasKeyElement()) tgt.setKeyElement(Id14_30.convertId(src.getKeyElement())); 426 if (src.hasRequirements()) tgt.setRequirementsElement(String14_30.convertString(src.getRequirementsElement())); 427 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 428 if (src.hasHumanElement()) tgt.setHumanElement(String14_30.convertString(src.getHumanElement())); 429 if (src.hasExpression()) tgt.setExpressionElement(String14_30.convertString(src.getExpressionElement())); 430 if (src.hasXpathElement()) tgt.setXpathElement(String14_30.convertString(src.getXpathElement())); 431 return tgt; 432 } 433 434 static public org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 435 if (src == null || src.isEmpty()) return null; 436 org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 437 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 438 if (src.getValue() == null) { 439 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 440 } else { 441 switch (src.getValue()) { 442 case ERROR: 443 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 444 break; 445 case WARNING: 446 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 447 break; 448 default: 449 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 450 break; 451 } 452 } 453 return tgt; 454 } 455 456 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu3.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 457 if (src == null || src.isEmpty()) return null; 458 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverityEnumFactory()); 459 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 460 if (src.getValue() == null) { 461 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity.NULL); 462 } else { 463 switch (src.getValue()) { 464 case ERROR: 465 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity.ERROR); 466 break; 467 case WARNING: 468 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity.WARNING); 469 break; 470 default: 471 tgt.setValue(org.hl7.fhir.dstu2016may.model.ElementDefinition.ConstraintSeverity.NULL); 472 break; 473 } 474 } 475 return tgt; 476 } 477 478 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 479 if (src == null || src.isEmpty()) return null; 480 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 481 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 482 if (src.hasStrength()) tgt.setStrengthElement(Enumerations14_30.convertBindingStrength(src.getStrengthElement())); 483 if (src.hasDescription()) tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 484 if (src.hasValueSet()) 485 tgt.setValueSet(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValueSet())); 486 return tgt; 487 } 488 489 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 490 if (src == null || src.isEmpty()) return null; 491 org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionBindingComponent(); 492 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 493 if (src.hasStrength()) tgt.setStrengthElement(Enumerations14_30.convertBindingStrength(src.getStrengthElement())); 494 if (src.hasDescription()) tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 495 if (src.hasValueSet()) 496 tgt.setValueSet(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValueSet())); 497 return tgt; 498 } 499 500 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 501 if (src == null || src.isEmpty()) return null; 502 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 503 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 504 if (src.hasIdentityElement()) tgt.setIdentityElement(Id14_30.convertId(src.getIdentityElement())); 505 if (src.hasLanguage()) tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 506 if (src.hasMapElement()) tgt.setMapElement(String14_30.convertString(src.getMapElement())); 507 return tgt; 508 } 509 510 public static org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 511 if (src == null || src.isEmpty()) return null; 512 org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2016may.model.ElementDefinition.ElementDefinitionMappingComponent(); 513 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 514 if (src.hasIdentityElement()) tgt.setIdentityElement(Id14_30.convertId(src.getIdentityElement())); 515 if (src.hasLanguage()) tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 516 if (src.hasMapElement()) tgt.setMapElement(String14_30.convertString(src.getMapElement())); 517 return tgt; 518 } 519}