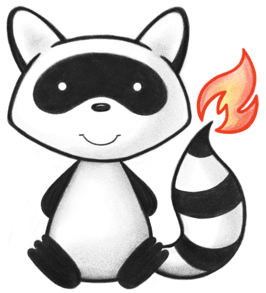
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 005import org.hl7.fhir.dstu3.model.Address; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class Address14_30 { 009 public static org.hl7.fhir.dstu3.model.Address convertAddress(org.hl7.fhir.dstu2016may.model.Address src) throws FHIRException { 010 if (src == null || src.isEmpty()) return null; 011 org.hl7.fhir.dstu3.model.Address tgt = new org.hl7.fhir.dstu3.model.Address(); 012 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 013 if (src.hasUse()) tgt.setUseElement(convertAddressUse(src.getUseElement())); 014 if (src.hasType()) tgt.setTypeElement(convertAddressType(src.getTypeElement())); 015 if (src.hasText()) tgt.setTextElement(String14_30.convertString(src.getTextElement())); 016 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getLine()) tgt.getLine().add(String14_30.convertString(t)); 017 if (src.hasCity()) tgt.setCityElement(String14_30.convertString(src.getCityElement())); 018 if (src.hasDistrict()) tgt.setDistrictElement(String14_30.convertString(src.getDistrictElement())); 019 if (src.hasState()) tgt.setStateElement(String14_30.convertString(src.getStateElement())); 020 if (src.hasPostalCode()) tgt.setPostalCodeElement(String14_30.convertString(src.getPostalCodeElement())); 021 if (src.hasCountry()) tgt.setCountryElement(String14_30.convertString(src.getCountryElement())); 022 if (src.hasPeriod()) tgt.setPeriod(Period14_30.convertPeriod(src.getPeriod())); 023 return tgt; 024 } 025 026 public static org.hl7.fhir.dstu2016may.model.Address convertAddress(org.hl7.fhir.dstu3.model.Address src) throws FHIRException { 027 if (src == null || src.isEmpty()) return null; 028 org.hl7.fhir.dstu2016may.model.Address tgt = new org.hl7.fhir.dstu2016may.model.Address(); 029 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 030 if (src.hasUse()) tgt.setUseElement(convertAddressUse(src.getUseElement())); 031 if (src.hasType()) tgt.setTypeElement(convertAddressType(src.getTypeElement())); 032 if (src.hasText()) tgt.setTextElement(String14_30.convertString(src.getTextElement())); 033 for (org.hl7.fhir.dstu3.model.StringType t : src.getLine()) tgt.getLine().add(String14_30.convertString(t)); 034 if (src.hasCity()) tgt.setCityElement(String14_30.convertString(src.getCityElement())); 035 if (src.hasDistrict()) tgt.setDistrictElement(String14_30.convertString(src.getDistrictElement())); 036 if (src.hasState()) tgt.setStateElement(String14_30.convertString(src.getStateElement())); 037 if (src.hasPostalCode()) tgt.setPostalCodeElement(String14_30.convertString(src.getPostalCodeElement())); 038 if (src.hasCountry()) tgt.setCountryElement(String14_30.convertString(src.getCountryElement())); 039 if (src.hasPeriod()) tgt.setPeriod(Period14_30.convertPeriod(src.getPeriod())); 040 return tgt; 041 } 042 043 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressUse> convertAddressUse(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressUse> src) throws FHIRException { 044 if (src == null || src.isEmpty()) return null; 045 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Address.AddressUseEnumFactory()); 046 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 047 if (src.getValue() == null) { 048 tgt.setValue(null); 049} else { 050 switch(src.getValue()) { 051 case HOME: 052 tgt.setValue(Address.AddressUse.HOME); 053 break; 054 case WORK: 055 tgt.setValue(Address.AddressUse.WORK); 056 break; 057 case TEMP: 058 tgt.setValue(Address.AddressUse.TEMP); 059 break; 060 case OLD: 061 tgt.setValue(Address.AddressUse.OLD); 062 break; 063 default: 064 tgt.setValue(Address.AddressUse.NULL); 065 break; 066 } 067} 068 return tgt; 069 } 070 071 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressUse> convertAddressUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressUse> src) throws FHIRException { 072 if (src == null || src.isEmpty()) return null; 073 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressUse> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Address.AddressUseEnumFactory()); 074 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 075 if (src.getValue() == null) { 076 tgt.setValue(null); 077} else { 078 switch(src.getValue()) { 079 case HOME: 080 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressUse.HOME); 081 break; 082 case WORK: 083 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressUse.WORK); 084 break; 085 case TEMP: 086 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressUse.TEMP); 087 break; 088 case OLD: 089 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressUse.OLD); 090 break; 091 default: 092 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressUse.NULL); 093 break; 094 } 095} 096 return tgt; 097 } 098 099 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressType> convertAddressType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressType> src) throws FHIRException { 100 if (src == null || src.isEmpty()) return null; 101 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Address.AddressTypeEnumFactory()); 102 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 103 if (src.getValue() == null) { 104 tgt.setValue(null); 105} else { 106 switch(src.getValue()) { 107 case POSTAL: 108 tgt.setValue(Address.AddressType.POSTAL); 109 break; 110 case PHYSICAL: 111 tgt.setValue(Address.AddressType.PHYSICAL); 112 break; 113 case BOTH: 114 tgt.setValue(Address.AddressType.BOTH); 115 break; 116 default: 117 tgt.setValue(Address.AddressType.NULL); 118 break; 119 } 120} 121 return tgt; 122 } 123 124 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressType> convertAddressType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Address.AddressType> src) throws FHIRException { 125 if (src == null || src.isEmpty()) return null; 126 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Address.AddressType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Address.AddressTypeEnumFactory()); 127 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 128 if (src.getValue() == null) { 129 tgt.setValue(null); 130} else { 131 switch(src.getValue()) { 132 case POSTAL: 133 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressType.POSTAL); 134 break; 135 case PHYSICAL: 136 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressType.PHYSICAL); 137 break; 138 case BOTH: 139 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressType.BOTH); 140 break; 141 default: 142 tgt.setValue(org.hl7.fhir.dstu2016may.model.Address.AddressType.NULL); 143 break; 144 } 145} 146 return tgt; 147 } 148}