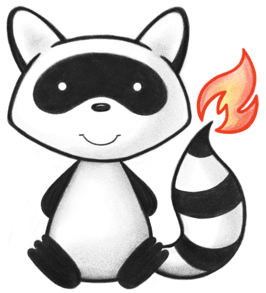
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Base64Binary14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.UnsignedInt14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Attachment14_30 { 013 public static org.hl7.fhir.dstu3.model.Attachment convertAttachment(org.hl7.fhir.dstu2016may.model.Attachment src) throws FHIRException { 014 if (src == null || src.isEmpty()) return null; 015 org.hl7.fhir.dstu3.model.Attachment tgt = new org.hl7.fhir.dstu3.model.Attachment(); 016 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 017 if (src.hasContentType()) tgt.setContentTypeElement(Code14_30.convertCode(src.getContentTypeElement())); 018 if (src.hasLanguage()) tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 019 if (src.hasData()) tgt.setDataElement(Base64Binary14_30.convertBase64Binary(src.getDataElement())); 020 if (src.hasUrl()) tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 021 if (src.hasSize()) tgt.setSizeElement(UnsignedInt14_30.convertUnsignedInt(src.getSizeElement())); 022 if (src.hasHash()) tgt.setHashElement(Base64Binary14_30.convertBase64Binary(src.getHashElement())); 023 if (src.hasTitle()) tgt.setTitleElement(String14_30.convertString(src.getTitleElement())); 024 if (src.hasCreation()) tgt.setCreationElement(DateTime14_30.convertDateTime(src.getCreationElement())); 025 return tgt; 026 } 027 028 public static org.hl7.fhir.dstu2016may.model.Attachment convertAttachment(org.hl7.fhir.dstu3.model.Attachment src) throws FHIRException { 029 if (src == null || src.isEmpty()) return null; 030 org.hl7.fhir.dstu2016may.model.Attachment tgt = new org.hl7.fhir.dstu2016may.model.Attachment(); 031 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 032 if (src.hasContentType()) tgt.setContentTypeElement(Code14_30.convertCode(src.getContentTypeElement())); 033 if (src.hasLanguage()) tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 034 if (src.hasData()) tgt.setDataElement(Base64Binary14_30.convertBase64Binary(src.getDataElement())); 035 if (src.hasUrl()) tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 036 if (src.hasSize()) tgt.setSizeElement(UnsignedInt14_30.convertUnsignedInt(src.getSizeElement())); 037 if (src.hasHash()) tgt.setHashElement(Base64Binary14_30.convertBase64Binary(src.getHashElement())); 038 if (src.hasTitle()) tgt.setTitleElement(String14_30.convertString(src.getTitleElement())); 039 if (src.hasCreation()) tgt.setCreationElement(DateTime14_30.convertDateTime(src.getCreationElement())); 040 return tgt; 041 } 042}