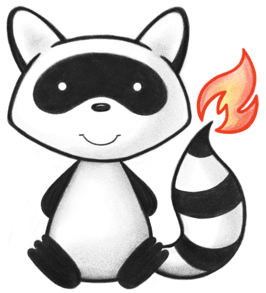
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.PositiveInt14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class ContactPoint14_30 { 009 public static org.hl7.fhir.dstu3.model.ContactPoint convertContactPoint(org.hl7.fhir.dstu2016may.model.ContactPoint src) throws FHIRException { 010 if (src == null || src.isEmpty()) return null; 011 org.hl7.fhir.dstu3.model.ContactPoint tgt = new org.hl7.fhir.dstu3.model.ContactPoint(); 012 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 013 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 014 if (src.hasValue()) tgt.setValueElement(String14_30.convertString(src.getValueElement())); 015 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 016 if (src.hasRank()) tgt.setRankElement(PositiveInt14_30.convertPositiveInt(src.getRankElement())); 017 if (src.hasPeriod()) tgt.setPeriod(Period14_30.convertPeriod(src.getPeriod())); 018 return tgt; 019 } 020 021 public static org.hl7.fhir.dstu2016may.model.ContactPoint convertContactPoint(org.hl7.fhir.dstu3.model.ContactPoint src) throws FHIRException { 022 if (src == null || src.isEmpty()) return null; 023 org.hl7.fhir.dstu2016may.model.ContactPoint tgt = new org.hl7.fhir.dstu2016may.model.ContactPoint(); 024 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 025 if (src.hasSystem()) tgt.setSystemElement(convertContactPointSystem(src.getSystemElement())); 026 if (src.hasValue()) tgt.setValueElement(String14_30.convertString(src.getValueElement())); 027 if (src.hasUse()) tgt.setUseElement(convertContactPointUse(src.getUseElement())); 028 if (src.hasRank()) tgt.setRankElement(PositiveInt14_30.convertPositiveInt(src.getRankElement())); 029 if (src.hasPeriod()) tgt.setPeriod(Period14_30.convertPeriod(src.getPeriod())); 030 return tgt; 031 } 032 033 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 034 if (src == null || src.isEmpty()) return null; 035 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystemEnumFactory()); 036 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 037 if (src.getValue() == null) { 038 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.NULL); 039 } else { 040 switch (src.getValue()) { 041 case PHONE: 042 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.PHONE); 043 break; 044 case FAX: 045 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.FAX); 046 break; 047 case EMAIL: 048 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.EMAIL); 049 break; 050 case PAGER: 051 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.PAGER); 052 break; 053 case OTHER: 054 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.URL); 055 break; 056 default: 057 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem.NULL); 058 break; 059 } 060 } 061 return tgt; 062 } 063 064 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem> convertContactPointSystem(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem> src) throws FHIRException { 065 if (src == null || src.isEmpty()) return null; 066 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystemEnumFactory()); 067 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.NULL); 070 } else { 071 switch (src.getValue()) { 072 case PHONE: 073 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.PHONE); 074 break; 075 case FAX: 076 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.FAX); 077 break; 078 case EMAIL: 079 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.EMAIL); 080 break; 081 case PAGER: 082 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.PAGER); 083 break; 084 case URL: 085 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.OTHER); 086 break; 087 default: 088 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointSystem.NULL); 089 break; 090 } 091 } 092 return tgt; 093 } 094 095 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse> src) throws FHIRException { 096 if (src == null || src.isEmpty()) return null; 097 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUseEnumFactory()); 098 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 099 if (src.getValue() == null) { 100 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.NULL); 101 } else { 102 switch (src.getValue()) { 103 case HOME: 104 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.HOME); 105 break; 106 case WORK: 107 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.WORK); 108 break; 109 case TEMP: 110 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.TEMP); 111 break; 112 case OLD: 113 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.OLD); 114 break; 115 case MOBILE: 116 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.MOBILE); 117 break; 118 default: 119 tgt.setValue(org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse.NULL); 120 break; 121 } 122 } 123 return tgt; 124 } 125 126 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse> convertContactPointUse(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ContactPoint.ContactPointUse> src) throws FHIRException { 127 if (src == null || src.isEmpty()) return null; 128 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUseEnumFactory()); 129 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 130 if (src.getValue() == null) { 131 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.NULL); 132 } else { 133 switch (src.getValue()) { 134 case HOME: 135 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.HOME); 136 break; 137 case WORK: 138 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.WORK); 139 break; 140 case TEMP: 141 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.TEMP); 142 break; 143 case OLD: 144 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.OLD); 145 break; 146 case MOBILE: 147 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.MOBILE); 148 break; 149 default: 150 tgt.setValue(org.hl7.fhir.dstu2016may.model.ContactPoint.ContactPointUse.NULL); 151 break; 152 } 153 } 154 return tgt; 155 } 156}