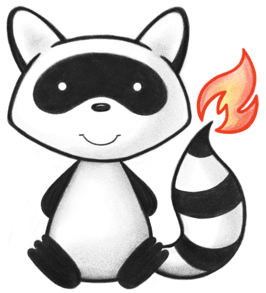
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Base64Binary14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Instant14_30; 007import org.hl7.fhir.exceptions.FHIRException; 008 009public class Signature14_30 { 010 public static org.hl7.fhir.dstu3.model.Signature convertSignature(org.hl7.fhir.dstu2016may.model.Signature src) throws FHIRException { 011 if (src == null || src.isEmpty()) return null; 012 org.hl7.fhir.dstu3.model.Signature tgt = new org.hl7.fhir.dstu3.model.Signature(); 013 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 014 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getType()) tgt.addType(Code14_30.convertCoding(t)); 015 if (src.hasWhenElement()) tgt.setWhenElement(Instant14_30.convertInstant(src.getWhenElement())); 016 if (src.hasWho()) tgt.setWho(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getWho())); 017 if (src.hasContentType()) tgt.setContentTypeElement(Code14_30.convertCode(src.getContentTypeElement())); 018 if (src.hasBlob()) tgt.setBlobElement(Base64Binary14_30.convertBase64Binary(src.getBlobElement())); 019 return tgt; 020 } 021 022 public static org.hl7.fhir.dstu2016may.model.Signature convertSignature(org.hl7.fhir.dstu3.model.Signature src) throws FHIRException { 023 if (src == null || src.isEmpty()) return null; 024 org.hl7.fhir.dstu2016may.model.Signature tgt = new org.hl7.fhir.dstu2016may.model.Signature(); 025 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 026 for (org.hl7.fhir.dstu3.model.Coding t : src.getType()) tgt.addType(Code14_30.convertCoding(t)); 027 if (src.hasWhenElement()) tgt.setWhenElement(Instant14_30.convertInstant(src.getWhenElement())); 028 if (src.hasWho()) tgt.setWho(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getWho())); 029 if (src.hasContentType()) tgt.setContentTypeElement(Code14_30.convertCode(src.getContentTypeElement())); 030 if (src.hasBlob()) tgt.setBlobElement(Base64Binary14_30.convertBase64Binary(src.getBlobElement())); 031 return tgt; 032 } 033}