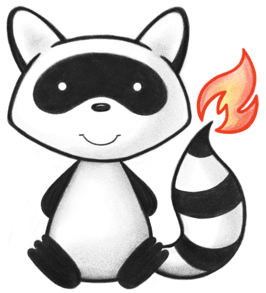
001package org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.exceptions.FHIRException; 005 006public class Code14_30 { 007 public static org.hl7.fhir.dstu3.model.CodeType convertCode(org.hl7.fhir.dstu2016may.model.CodeType src) throws FHIRException { 008 org.hl7.fhir.dstu3.model.CodeType tgt = src.hasValue() ? new org.hl7.fhir.dstu3.model.CodeType(src.getValueAsString()) : new org.hl7.fhir.dstu3.model.CodeType(); 009 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 010 return tgt; 011 } 012 013 public static org.hl7.fhir.dstu2016may.model.CodeType convertCode(org.hl7.fhir.dstu3.model.CodeType src) throws FHIRException { 014 org.hl7.fhir.dstu2016may.model.CodeType tgt = src.hasValue() ? new org.hl7.fhir.dstu2016may.model.CodeType(src.getValueAsString()) : new org.hl7.fhir.dstu2016may.model.CodeType(); 015 if (src.hasValue()) tgt.setValue(src.getValue()); 016 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 017 return tgt; 018 } 019 020 public static org.hl7.fhir.dstu3.model.Coding convertCoding(org.hl7.fhir.dstu2016may.model.Coding src) throws FHIRException { 021 if (src == null || src.isEmpty()) return null; 022 org.hl7.fhir.dstu3.model.Coding tgt = new org.hl7.fhir.dstu3.model.Coding(); 023 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 024 if (src.hasSystem()) tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 025 if (src.hasVersion()) tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 026 if (src.hasCode()) tgt.setCodeElement(convertCode(src.getCodeElement())); 027 if (src.hasDisplay()) tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 028 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean14_30.convertBoolean(src.getUserSelectedElement())); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu2016may.model.Coding convertCoding(org.hl7.fhir.dstu3.model.Coding src) throws FHIRException { 033 if (src == null || src.isEmpty()) return null; 034 org.hl7.fhir.dstu2016may.model.Coding tgt = new org.hl7.fhir.dstu2016may.model.Coding(); 035 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 036 if (src.hasSystem()) tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 037 if (src.hasVersion()) tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 038 if (src.hasCode()) tgt.setCodeElement(convertCode(src.getCodeElement())); 039 if (src.hasDisplay()) tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 040 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean14_30.convertBoolean(src.getUserSelectedElement())); 041 return tgt; 042 } 043}