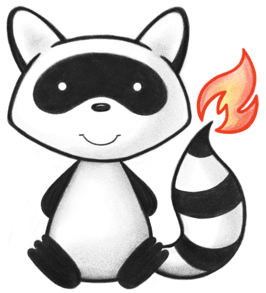
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Signature14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Decimal14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Instant14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.UnsignedInt14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 010import org.hl7.fhir.exceptions.FHIRException; 011 012public class Bundle14_30 { 013 014 public static org.hl7.fhir.dstu3.model.Bundle convertBundle(org.hl7.fhir.dstu2016may.model.Bundle src) throws FHIRException { 015 if (src == null || src.isEmpty()) 016 return null; 017 org.hl7.fhir.dstu3.model.Bundle tgt = new org.hl7.fhir.dstu3.model.Bundle(); 018 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyResource(src, tgt); 019 if (src.hasType()) 020 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 021 if (src.hasTotal()) 022 tgt.setTotalElement(UnsignedInt14_30.convertUnsignedInt(src.getTotalElement())); 023 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 024 tgt.addLink(convertBundleLinkComponent(t)); 025 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent t : src.getEntry()) 026 tgt.addEntry(convertBundleEntryComponent(t)); 027 if (src.hasSignature()) 028 tgt.setSignature(Signature14_30.convertSignature(src.getSignature())); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu2016may.model.Bundle convertBundle(org.hl7.fhir.dstu3.model.Bundle src) throws FHIRException { 033 if (src == null || src.isEmpty()) 034 return null; 035 org.hl7.fhir.dstu2016may.model.Bundle tgt = new org.hl7.fhir.dstu2016may.model.Bundle(); 036 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyResource(src, tgt); 037 if (src.hasType()) 038 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 039 if (src.hasTotal()) 040 tgt.setTotalElement(UnsignedInt14_30.convertUnsignedInt(src.getTotalElement())); 041 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 042 tgt.addLink(convertBundleLinkComponent(t)); 043 for (org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent t : src.getEntry()) 044 tgt.addEntry(convertBundleEntryComponent(t)); 045 if (src.hasSignature()) 046 tgt.setSignature(Signature14_30.convertSignature(src.getSignature())); 047 return tgt; 048 } 049 050 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent src) throws FHIRException { 051 if (src == null || src.isEmpty()) 052 return null; 053 org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent(); 054 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 055 for (org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent t : src.getLink()) 056 tgt.addLink(convertBundleLinkComponent(t)); 057 if (src.hasFullUrl()) 058 tgt.setFullUrlElement(Uri14_30.convertUri(src.getFullUrlElement())); 059 if (src.hasResource()) 060 tgt.setResource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertResource(src.getResource())); 061 if (src.hasSearch()) 062 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 063 if (src.hasRequest()) 064 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 065 if (src.hasResponse()) 066 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 067 return tgt; 068 } 069 070 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent src) throws FHIRException { 071 if (src == null || src.isEmpty()) 072 return null; 073 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryComponent(); 074 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 075 for (org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent t : src.getLink()) 076 tgt.addLink(convertBundleLinkComponent(t)); 077 if (src.hasFullUrl()) 078 tgt.setFullUrlElement(Uri14_30.convertUri(src.getFullUrlElement())); 079 if (src.hasResource()) 080 tgt.setResource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertResource(src.getResource())); 081 if (src.hasSearch()) 082 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 083 if (src.hasRequest()) 084 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 085 if (src.hasResponse()) 086 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 091 if (src == null || src.isEmpty()) 092 return null; 093 org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent(); 094 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 095 if (src.hasMethod()) 096 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 097 if (src.hasUrlElement()) 098 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 099 if (src.hasIfNoneMatch()) 100 tgt.setIfNoneMatchElement(String14_30.convertString(src.getIfNoneMatchElement())); 101 if (src.hasIfModifiedSince()) 102 tgt.setIfModifiedSinceElement(Instant14_30.convertInstant(src.getIfModifiedSinceElement())); 103 if (src.hasIfMatch()) 104 tgt.setIfMatchElement(String14_30.convertString(src.getIfMatchElement())); 105 if (src.hasIfNoneExist()) 106 tgt.setIfNoneExistElement(String14_30.convertString(src.getIfNoneExistElement())); 107 return tgt; 108 } 109 110 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryRequestComponent(); 114 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 115 if (src.hasMethod()) 116 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 117 if (src.hasUrlElement()) 118 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 119 if (src.hasIfNoneMatch()) 120 tgt.setIfNoneMatchElement(String14_30.convertString(src.getIfNoneMatchElement())); 121 if (src.hasIfModifiedSince()) 122 tgt.setIfModifiedSinceElement(Instant14_30.convertInstant(src.getIfModifiedSinceElement())); 123 if (src.hasIfMatch()) 124 tgt.setIfMatchElement(String14_30.convertString(src.getIfMatchElement())); 125 if (src.hasIfNoneExist()) 126 tgt.setIfNoneExistElement(String14_30.convertString(src.getIfNoneExistElement())); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent(); 134 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 135 if (src.hasStatusElement()) 136 tgt.setStatusElement(String14_30.convertString(src.getStatusElement())); 137 if (src.hasLocation()) 138 tgt.setLocationElement(Uri14_30.convertUri(src.getLocationElement())); 139 if (src.hasEtag()) 140 tgt.setEtagElement(String14_30.convertString(src.getEtagElement())); 141 if (src.hasLastModified()) 142 tgt.setLastModifiedElement(Instant14_30.convertInstant(src.getLastModifiedElement())); 143 return tgt; 144 } 145 146 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 147 if (src == null || src.isEmpty()) 148 return null; 149 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntryResponseComponent(); 150 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 151 if (src.hasStatusElement()) 152 tgt.setStatusElement(String14_30.convertString(src.getStatusElement())); 153 if (src.hasLocation()) 154 tgt.setLocationElement(Uri14_30.convertUri(src.getLocationElement())); 155 if (src.hasEtag()) 156 tgt.setEtagElement(String14_30.convertString(src.getEtagElement())); 157 if (src.hasLastModified()) 158 tgt.setLastModifiedElement(Instant14_30.convertInstant(src.getLastModifiedElement())); 159 return tgt; 160 } 161 162 public static org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 163 if (src == null || src.isEmpty()) 164 return null; 165 org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent(); 166 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 167 if (src.hasMode()) 168 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 169 if (src.hasScore()) 170 tgt.setScoreElement(Decimal14_30.convertDecimal(src.getScoreElement())); 171 return tgt; 172 } 173 174 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.dstu3.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleEntrySearchComponent(); 178 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 179 if (src.hasMode()) 180 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 181 if (src.hasScore()) 182 tgt.setScoreElement(Decimal14_30.convertDecimal(src.getScoreElement())); 183 return tgt; 184 } 185 186 public static org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent src) throws FHIRException { 187 if (src == null || src.isEmpty()) 188 return null; 189 org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent(); 190 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 191 if (src.hasRelationElement()) 192 tgt.setRelationElement(String14_30.convertString(src.getRelationElement())); 193 if (src.hasUrlElement()) 194 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 195 return tgt; 196 } 197 198 public static org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.dstu2016may.model.Bundle.BundleLinkComponent src) throws FHIRException { 199 if (src == null || src.isEmpty()) 200 return null; 201 org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.dstu3.model.Bundle.BundleLinkComponent(); 202 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 203 if (src.hasRelationElement()) 204 tgt.setRelationElement(String14_30.convertString(src.getRelationElement())); 205 if (src.hasUrlElement()) 206 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 207 return tgt; 208 } 209 210 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> src) throws FHIRException { 211 if (src == null || src.isEmpty()) 212 return null; 213 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.BundleTypeEnumFactory()); 214 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 215 switch (src.getValue()) { 216 case DOCUMENT: 217 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.DOCUMENT); 218 break; 219 case MESSAGE: 220 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.MESSAGE); 221 break; 222 case TRANSACTION: 223 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.TRANSACTION); 224 break; 225 case TRANSACTIONRESPONSE: 226 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.TRANSACTIONRESPONSE); 227 break; 228 case BATCH: 229 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.BATCH); 230 break; 231 case BATCHRESPONSE: 232 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.BATCHRESPONSE); 233 break; 234 case HISTORY: 235 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.HISTORY); 236 break; 237 case SEARCHSET: 238 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.SEARCHSET); 239 break; 240 case COLLECTION: 241 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.COLLECTION); 242 break; 243 default: 244 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.BundleType.NULL); 245 break; 246 } 247 return tgt; 248 } 249 250 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.BundleType> src) throws FHIRException { 251 if (src == null || src.isEmpty()) 252 return null; 253 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.BundleType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.BundleTypeEnumFactory()); 254 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 255 switch (src.getValue()) { 256 case DOCUMENT: 257 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.DOCUMENT); 258 break; 259 case MESSAGE: 260 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.MESSAGE); 261 break; 262 case TRANSACTION: 263 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTION); 264 break; 265 case TRANSACTIONRESPONSE: 266 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.TRANSACTIONRESPONSE); 267 break; 268 case BATCH: 269 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCH); 270 break; 271 case BATCHRESPONSE: 272 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.BATCHRESPONSE); 273 break; 274 case HISTORY: 275 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.HISTORY); 276 break; 277 case SEARCHSET: 278 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.SEARCHSET); 279 break; 280 case COLLECTION: 281 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.COLLECTION); 282 break; 283 default: 284 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.BundleType.NULL); 285 break; 286 } 287 return tgt; 288 } 289 290 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> src) throws FHIRException { 291 if (src == null || src.isEmpty()) 292 return null; 293 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.HTTPVerbEnumFactory()); 294 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 295 switch (src.getValue()) { 296 case GET: 297 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.GET); 298 break; 299 case POST: 300 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.POST); 301 break; 302 case PUT: 303 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.PUT); 304 break; 305 case DELETE: 306 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.DELETE); 307 break; 308 default: 309 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.HTTPVerb.NULL); 310 break; 311 } 312 return tgt; 313 } 314 315 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.HTTPVerb> src) throws FHIRException { 316 if (src == null || src.isEmpty()) 317 return null; 318 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerbEnumFactory()); 319 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 320 switch (src.getValue()) { 321 case GET: 322 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.GET); 323 break; 324 case POST: 325 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.POST); 326 break; 327 case PUT: 328 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.PUT); 329 break; 330 case DELETE: 331 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.DELETE); 332 break; 333 default: 334 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.HTTPVerb.NULL); 335 break; 336 } 337 return tgt; 338 } 339 340 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> src) throws FHIRException { 341 if (src == null || src.isEmpty()) 342 return null; 343 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Bundle.SearchEntryModeEnumFactory()); 344 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 345 switch (src.getValue()) { 346 case MATCH: 347 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.MATCH); 348 break; 349 case INCLUDE: 350 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.INCLUDE); 351 break; 352 case OUTCOME: 353 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.OUTCOME); 354 break; 355 default: 356 tgt.setValue(org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode.NULL); 357 break; 358 } 359 return tgt; 360 } 361 362 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Bundle.SearchEntryMode> src) throws FHIRException { 363 if (src == null || src.isEmpty()) 364 return null; 365 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryModeEnumFactory()); 366 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 367 switch (src.getValue()) { 368 case MATCH: 369 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.MATCH); 370 break; 371 case INCLUDE: 372 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.INCLUDE); 373 break; 374 case OUTCOME: 375 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.OUTCOME); 376 break; 377 default: 378 tgt.setValue(org.hl7.fhir.dstu2016may.model.Bundle.SearchEntryMode.NULL); 379 break; 380 } 381 return tgt; 382 } 383}