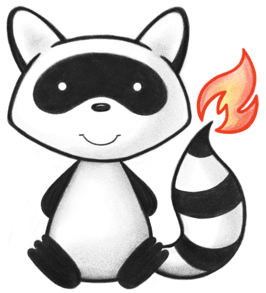
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.SourceElementComponentWrapper; 007import org.hl7.fhir.convertors.context.ConversionContext14_30; 008import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Identifier14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 014import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 015import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 016import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 017import org.hl7.fhir.dstu2016may.model.Enumeration; 018import org.hl7.fhir.dstu2016may.model.Enumerations; 019import org.hl7.fhir.dstu3.model.ConceptMap; 020import org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent; 021import org.hl7.fhir.exceptions.FHIRException; 022 023public class ConceptMap14_30 { 024 025 public static org.hl7.fhir.dstu2016may.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu3.model.ConceptMap src) throws FHIRException { 026 if (src == null || src.isEmpty()) 027 return null; 028 org.hl7.fhir.dstu2016may.model.ConceptMap tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap(); 029 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 030 if (src.hasUrl()) 031 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 032 if (src.hasIdentifier()) 033 tgt.setIdentifier(Identifier14_30.convertIdentifier(src.getIdentifier())); 034 if (src.hasVersion()) 035 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 036 if (src.hasName()) 037 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 038 if (src.hasStatus()) 039 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 040 if (src.hasExperimental()) 041 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 042 if (src.hasPublisher()) 043 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 044 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 045 tgt.addContact(convertConceptMapContactComponent(t)); 046 if (src.hasDate()) 047 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 048 if (src.hasDescription()) 049 tgt.setDescription(src.getDescription()); 050 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 051 if (t.hasValueCodeableConcept()) 052 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 053 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 054 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 055 if (src.hasPurpose()) 056 tgt.setRequirements(src.getPurpose()); 057 if (src.hasCopyright()) 058 tgt.setCopyright(src.getCopyright()); 059 if (src.hasSource()) 060 tgt.setSource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getSource())); 061 if (src.hasTarget()) 062 tgt.setTarget(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getTarget())); 063 for (org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent g : src.getGroup()) 064 for (org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent t : g.getElement()) 065 tgt.addElement(convertSourceElementComponent(t, g)); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.dstu3.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu2016may.model.ConceptMap src) throws FHIRException { 070 if (src == null || src.isEmpty()) 071 return null; 072 org.hl7.fhir.dstu3.model.ConceptMap tgt = new org.hl7.fhir.dstu3.model.ConceptMap(); 073 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 074 if (src.hasUrl()) 075 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 076 if (src.hasIdentifier()) 077 tgt.setIdentifier(Identifier14_30.convertIdentifier(src.getIdentifier())); 078 if (src.hasVersion()) 079 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 080 if (src.hasName()) 081 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 082 if (src.hasStatus()) 083 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 084 if (src.hasExperimental()) 085 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 086 if (src.hasPublisher()) 087 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 088 for (org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent t : src.getContact()) 089 tgt.addContact(convertConceptMapContactComponent(t)); 090 if (src.hasDate()) 091 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 092 if (src.hasDescription()) 093 tgt.setDescription(src.getDescription()); 094 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 095 if (VersionConvertor_14_30.isJurisdiction(t)) 096 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 097 else 098 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 099 if (src.hasRequirements()) 100 tgt.setPurpose(src.getRequirements()); 101 if (src.hasCopyright()) 102 tgt.setCopyright(src.getCopyright()); 103 if (src.hasSource()) 104 tgt.setSource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getSource())); 105 if (src.hasTarget()) 106 tgt.setTarget(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getTarget())); 107 for (org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent t : src.getElement()) { 108 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> ws = convertSourceElementComponent(t); 109 for (SourceElementComponentWrapper<ConceptMap.SourceElementComponent> w : ws) 110 getGroup(tgt, w.getSource(), w.getTarget()).addElement(w.getComp()); 111 } 112 return tgt; 113 } 114 115 public static org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent convertConceptMapContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent(); 119 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 120 if (src.hasName()) 121 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 122 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 123 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 124 return tgt; 125 } 126 127 public static org.hl7.fhir.dstu3.model.ContactDetail convertConceptMapContactComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 131 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 132 if (src.hasName()) 133 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 134 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 135 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 136 return tgt; 137 } 138 139 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 140 if (src == null || src.isEmpty()) 141 return null; 142 Enumeration<Enumerations.ConceptMapEquivalence> tgt = new Enumeration<>(new Enumerations.ConceptMapEquivalenceEnumFactory()); 143 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 144 if (src.getValue() == null) { 145 tgt.setValue(null); 146 } else { 147 switch (src.getValue()) { 148 case EQUIVALENT: 149 tgt.setValue(Enumerations.ConceptMapEquivalence.EQUIVALENT); 150 break; 151 case EQUAL: 152 tgt.setValue(Enumerations.ConceptMapEquivalence.EQUAL); 153 break; 154 case WIDER: 155 tgt.setValue(Enumerations.ConceptMapEquivalence.WIDER); 156 break; 157 case SUBSUMES: 158 tgt.setValue(Enumerations.ConceptMapEquivalence.SUBSUMES); 159 break; 160 case NARROWER: 161 tgt.setValue(Enumerations.ConceptMapEquivalence.NARROWER); 162 break; 163 case SPECIALIZES: 164 tgt.setValue(Enumerations.ConceptMapEquivalence.SPECIALIZES); 165 break; 166 case INEXACT: 167 tgt.setValue(Enumerations.ConceptMapEquivalence.INEXACT); 168 break; 169 case UNMATCHED: 170 tgt.setValue(Enumerations.ConceptMapEquivalence.UNMATCHED); 171 break; 172 case DISJOINT: 173 tgt.setValue(Enumerations.ConceptMapEquivalence.DISJOINT); 174 break; 175 default: 176 tgt.setValue(Enumerations.ConceptMapEquivalence.NULL); 177 break; 178 } 179 } 180 return tgt; 181 } 182 183 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 187 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 188 if (src.getValue() == null) { 189 tgt.setValue(null); 190 } else { 191 switch (src.getValue()) { 192 case EQUIVALENT: 193 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 194 break; 195 case EQUAL: 196 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.EQUAL); 197 break; 198 case WIDER: 199 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.WIDER); 200 break; 201 case SUBSUMES: 202 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.SUBSUMES); 203 break; 204 case NARROWER: 205 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.NARROWER); 206 break; 207 case SPECIALIZES: 208 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.SPECIALIZES); 209 break; 210 case INEXACT: 211 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.INEXACT); 212 break; 213 case UNMATCHED: 214 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.UNMATCHED); 215 break; 216 case DISJOINT: 217 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.DISJOINT); 218 break; 219 default: 220 tgt.setValue(org.hl7.fhir.dstu3.model.Enumerations.ConceptMapEquivalence.NULL); 221 break; 222 } 223 } 224 return tgt; 225 } 226 227 public static org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent src) throws FHIRException { 228 if (src == null || src.isEmpty()) 229 return null; 230 org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent(); 231 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 232 if (src.hasPropertyElement()) 233 tgt.setElementElement(Uri14_30.convertUri(src.getPropertyElement())); 234 if (src.hasSystemElement()) 235 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 236 if (src.hasCodeElement()) 237 tgt.setCodeElement(String14_30.convertString(src.getCodeElement())); 238 return tgt; 239 } 240 241 public static org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent src) throws FHIRException { 242 if (src == null || src.isEmpty()) 243 return null; 244 org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent(); 245 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 246 if (src.hasElementElement()) 247 tgt.setPropertyElement(Uri14_30.convertUri(src.getElementElement())); 248 if (src.hasSystemElement()) 249 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 250 if (src.hasCodeElement()) 251 tgt.setCodeElement(String14_30.convertString(src.getCodeElement())); 252 return tgt; 253 } 254 255 public static org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent g) throws FHIRException { 256 if (src == null || src.isEmpty()) 257 return null; 258 org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent(); 259 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 260 if (g.hasSource()) 261 tgt.setSystem(g.getSource()); 262 if (src.hasCode()) 263 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 264 for (org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent t : src.getTarget()) 265 tgt.addTarget(convertTargetElementComponent(t, g)); 266 return tgt; 267 } 268 269 public static List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> convertSourceElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent src) throws FHIRException { 270 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> res = new ArrayList<>(); 271 if (src == null || src.isEmpty()) 272 return res; 273 for (org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent t : src.getTarget()) { 274 org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.SourceElementComponent(); 275 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 276 if (src.hasCode()) 277 tgt.setCode(src.getCode()); 278 tgt.addTarget(convertTargetElementComponent(t)); 279 res.add(new SourceElementComponentWrapper<>(tgt, src.getSystem(), t.getSystem())); 280 } 281 return res; 282 } 283 284 public static org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.dstu3.model.ConceptMap.ConceptMapGroupComponent g) throws FHIRException { 285 if (src == null || src.isEmpty()) 286 return null; 287 org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent(); 288 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 289 if (g.hasTarget()) 290 tgt.setSystem(g.getTarget()); 291 if (src.hasCode()) 292 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 293 if (src.hasEquivalence()) 294 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 295 if (src.hasComment()) 296 tgt.setCommentsElement(String14_30.convertString(src.getCommentElement())); 297 for (org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 298 tgt.addDependsOn(convertOtherElementComponent(t)); 299 for (org.hl7.fhir.dstu3.model.ConceptMap.OtherElementComponent t : src.getProduct()) 300 tgt.addProduct(convertOtherElementComponent(t)); 301 return tgt; 302 } 303 304 public static org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent src) throws FHIRException { 305 if (src == null || src.isEmpty()) 306 return null; 307 org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu3.model.ConceptMap.TargetElementComponent(); 308 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 309 if (src.hasCode()) 310 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 311 if (src.hasEquivalence()) 312 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 313 if (src.hasComments()) 314 tgt.setCommentElement(String14_30.convertString(src.getCommentsElement())); 315 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 316 tgt.addDependsOn(convertOtherElementComponent(t)); 317 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getProduct()) 318 tgt.addProduct(convertOtherElementComponent(t)); 319 return tgt; 320 } 321 322 static public ConceptMapGroupComponent getGroup(ConceptMap map, String srcs, String tgts) { 323 for (ConceptMapGroupComponent grp : map.getGroup()) { 324 if (grp.hasSource() && grp.getSource().equals(srcs) && grp.getTarget().equals(tgts)) 325 return grp; 326 } 327 ConceptMapGroupComponent grp = map.addGroup(); 328 grp.setSource(srcs); 329 grp.setTarget(tgts); 330 return grp; 331 } 332}