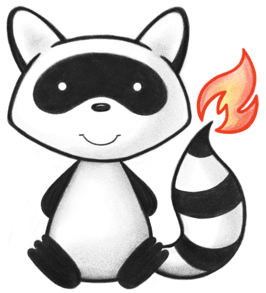
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.ElementDefinition14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Identifier14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Id14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 014import org.hl7.fhir.dstu3.model.DataElement; 015import org.hl7.fhir.dstu3.model.Enumeration; 016import org.hl7.fhir.exceptions.FHIRException; 017 018public class DataElement14_30 { 019 020 public static org.hl7.fhir.dstu3.model.DataElement convertDataElement(org.hl7.fhir.dstu2016may.model.DataElement src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.dstu3.model.DataElement tgt = new org.hl7.fhir.dstu3.model.DataElement(); 024 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 025 if (src.hasUrl()) 026 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 027 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 028 tgt.addIdentifier(Identifier14_30.convertIdentifier(t)); 029 if (src.hasVersion()) 030 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 031 if (src.hasStatus()) 032 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 033 if (src.hasExperimental()) 034 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 035 if (src.hasPublisher()) 036 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 037 if (src.hasDate()) 038 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 039 if (src.hasName()) 040 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 041 for (org.hl7.fhir.dstu2016may.model.DataElement.DataElementContactComponent t : src.getContact()) 042 tgt.addContact(convertDataElementContactComponent(t)); 043 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 044 if (VersionConvertor_14_30.isJurisdiction(t)) 045 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 046 else 047 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 048 if (src.hasCopyright()) 049 tgt.setCopyright(src.getCopyright()); 050 if (src.hasStringency()) 051 tgt.setStringencyElement(convertDataElementStringency(src.getStringencyElement())); 052 for (org.hl7.fhir.dstu2016may.model.DataElement.DataElementMappingComponent t : src.getMapping()) 053 tgt.addMapping(convertDataElementMappingComponent(t)); 054 for (org.hl7.fhir.dstu2016may.model.ElementDefinition t : src.getElement()) 055 tgt.addElement(ElementDefinition14_30.convertElementDefinition(t)); 056 return tgt; 057 } 058 059 public static org.hl7.fhir.dstu2016may.model.DataElement convertDataElement(org.hl7.fhir.dstu3.model.DataElement src) throws FHIRException { 060 if (src == null || src.isEmpty()) 061 return null; 062 org.hl7.fhir.dstu2016may.model.DataElement tgt = new org.hl7.fhir.dstu2016may.model.DataElement(); 063 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 064 if (src.hasUrl()) 065 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 066 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 067 tgt.addIdentifier(Identifier14_30.convertIdentifier(t)); 068 if (src.hasVersion()) 069 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 070 if (src.hasStatus()) 071 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 072 if (src.hasExperimental()) 073 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 074 if (src.hasPublisher()) 075 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 076 if (src.hasDate()) 077 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 078 if (src.hasName()) 079 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 080 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 081 tgt.addContact(convertDataElementContactComponent(t)); 082 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 083 if (t.hasValueCodeableConcept()) 084 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 085 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 086 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 087 if (src.hasCopyright()) 088 tgt.setCopyright(src.getCopyright()); 089 if (src.hasStringency()) 090 tgt.setStringencyElement(convertDataElementStringency(src.getStringencyElement())); 091 for (org.hl7.fhir.dstu3.model.DataElement.DataElementMappingComponent t : src.getMapping()) 092 tgt.addMapping(convertDataElementMappingComponent(t)); 093 for (org.hl7.fhir.dstu3.model.ElementDefinition t : src.getElement()) 094 tgt.addElement(ElementDefinition14_30.convertElementDefinition(t)); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.dstu3.model.ContactDetail convertDataElementContactComponent(org.hl7.fhir.dstu2016may.model.DataElement.DataElementContactComponent src) throws FHIRException { 099 if (src == null || src.isEmpty()) 100 return null; 101 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 102 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 103 if (src.hasName()) 104 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 105 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 106 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 107 return tgt; 108 } 109 110 public static org.hl7.fhir.dstu2016may.model.DataElement.DataElementContactComponent convertDataElementContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 org.hl7.fhir.dstu2016may.model.DataElement.DataElementContactComponent tgt = new org.hl7.fhir.dstu2016may.model.DataElement.DataElementContactComponent(); 114 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 115 if (src.hasName()) 116 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 117 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 118 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 119 return tgt; 120 } 121 122 public static org.hl7.fhir.dstu3.model.DataElement.DataElementMappingComponent convertDataElementMappingComponent(org.hl7.fhir.dstu2016may.model.DataElement.DataElementMappingComponent src) throws FHIRException { 123 if (src == null || src.isEmpty()) 124 return null; 125 org.hl7.fhir.dstu3.model.DataElement.DataElementMappingComponent tgt = new org.hl7.fhir.dstu3.model.DataElement.DataElementMappingComponent(); 126 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 127 if (src.hasIdentityElement()) 128 tgt.setIdentityElement(Id14_30.convertId(src.getIdentityElement())); 129 if (src.hasUri()) 130 tgt.setUriElement(Uri14_30.convertUri(src.getUriElement())); 131 if (src.hasName()) 132 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 133 if (src.hasComment()) 134 tgt.setCommentElement(String14_30.convertString(src.getCommentElement())); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.dstu2016may.model.DataElement.DataElementMappingComponent convertDataElementMappingComponent(org.hl7.fhir.dstu3.model.DataElement.DataElementMappingComponent src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.dstu2016may.model.DataElement.DataElementMappingComponent tgt = new org.hl7.fhir.dstu2016may.model.DataElement.DataElementMappingComponent(); 142 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 143 if (src.hasIdentityElement()) 144 tgt.setIdentityElement(Id14_30.convertId(src.getIdentityElement())); 145 if (src.hasUri()) 146 tgt.setUriElement(Uri14_30.convertUri(src.getUriElement())); 147 if (src.hasName()) 148 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 149 if (src.hasComment()) 150 tgt.setCommentElement(String14_30.convertString(src.getCommentElement())); 151 return tgt; 152 } 153 154 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DataElement.DataElementStringency> convertDataElementStringency(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency> src) throws FHIRException { 155 if (src == null || src.isEmpty()) 156 return null; 157 Enumeration<DataElement.DataElementStringency> tgt = new Enumeration<>(new DataElement.DataElementStringencyEnumFactory()); 158 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 159 if (src.getValue() == null) { 160 tgt.setValue(null); 161 } else { 162 switch (src.getValue()) { 163 case COMPARABLE: 164 tgt.setValue(DataElement.DataElementStringency.COMPARABLE); 165 break; 166 case FULLYSPECIFIED: 167 tgt.setValue(DataElement.DataElementStringency.FULLYSPECIFIED); 168 break; 169 case EQUIVALENT: 170 tgt.setValue(DataElement.DataElementStringency.EQUIVALENT); 171 break; 172 case CONVERTABLE: 173 tgt.setValue(DataElement.DataElementStringency.CONVERTABLE); 174 break; 175 case SCALEABLE: 176 tgt.setValue(DataElement.DataElementStringency.SCALEABLE); 177 break; 178 case FLEXIBLE: 179 tgt.setValue(DataElement.DataElementStringency.FLEXIBLE); 180 break; 181 default: 182 tgt.setValue(DataElement.DataElementStringency.NULL); 183 break; 184 } 185 } 186 return tgt; 187 } 188 189 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency> convertDataElementStringency(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.DataElement.DataElementStringency> src) throws FHIRException { 190 if (src == null || src.isEmpty()) 191 return null; 192 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringencyEnumFactory()); 193 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 194 if (src.getValue() == null) { 195 tgt.setValue(null); 196 } else { 197 switch (src.getValue()) { 198 case COMPARABLE: 199 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.COMPARABLE); 200 break; 201 case FULLYSPECIFIED: 202 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.FULLYSPECIFIED); 203 break; 204 case EQUIVALENT: 205 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.EQUIVALENT); 206 break; 207 case CONVERTABLE: 208 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.CONVERTABLE); 209 break; 210 case SCALEABLE: 211 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.SCALEABLE); 212 break; 213 case FLEXIBLE: 214 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.FLEXIBLE); 215 break; 216 default: 217 tgt.setValue(org.hl7.fhir.dstu2016may.model.DataElement.DataElementStringency.NULL); 218 break; 219 } 220 } 221 return tgt; 222 } 223}