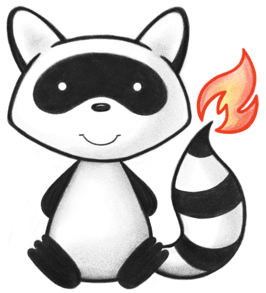
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.Reference14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Id14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 014import org.hl7.fhir.dstu3.model.Enumeration; 015import org.hl7.fhir.dstu3.model.ImplementationGuide; 016import org.hl7.fhir.exceptions.FHIRException; 017 018public class ImplementationGuide14_30 { 019 020 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ImplementationGuide.GuideDependencyType> convertGuideDependencyType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType> src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 Enumeration<ImplementationGuide.GuideDependencyType> tgt = new Enumeration<>(new ImplementationGuide.GuideDependencyTypeEnumFactory()); 024 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 025 if (src.getValue() == null) { 026 tgt.setValue(null); 027 } else { 028 switch (src.getValue()) { 029 case REFERENCE: 030 tgt.setValue(ImplementationGuide.GuideDependencyType.REFERENCE); 031 break; 032 case INCLUSION: 033 tgt.setValue(ImplementationGuide.GuideDependencyType.INCLUSION); 034 break; 035 default: 036 tgt.setValue(ImplementationGuide.GuideDependencyType.NULL); 037 break; 038 } 039 } 040 return tgt; 041 } 042 043 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType> convertGuideDependencyType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ImplementationGuide.GuideDependencyType> src) throws FHIRException { 044 if (src == null || src.isEmpty()) 045 return null; 046 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyTypeEnumFactory()); 047 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 048 if (src.getValue() == null) { 049 tgt.setValue(null); 050 } else { 051 switch (src.getValue()) { 052 case REFERENCE: 053 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType.REFERENCE); 054 break; 055 case INCLUSION: 056 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType.INCLUSION); 057 break; 058 default: 059 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType.NULL); 060 break; 061 } 062 } 063 return tgt; 064 } 065 066 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind> convertGuidePageKind(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind> src) throws FHIRException { 067 if (src == null || src.isEmpty()) 068 return null; 069 Enumeration<ImplementationGuide.GuidePageKind> tgt = new Enumeration<>(new ImplementationGuide.GuidePageKindEnumFactory()); 070 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 071 if (src.getValue() == null) { 072 tgt.setValue(null); 073 } else { 074 switch (src.getValue()) { 075 case PAGE: 076 tgt.setValue(ImplementationGuide.GuidePageKind.PAGE); 077 break; 078 case EXAMPLE: 079 tgt.setValue(ImplementationGuide.GuidePageKind.EXAMPLE); 080 break; 081 case LIST: 082 tgt.setValue(ImplementationGuide.GuidePageKind.LIST); 083 break; 084 case INCLUDE: 085 tgt.setValue(ImplementationGuide.GuidePageKind.INCLUDE); 086 break; 087 case DIRECTORY: 088 tgt.setValue(ImplementationGuide.GuidePageKind.DIRECTORY); 089 break; 090 case DICTIONARY: 091 tgt.setValue(ImplementationGuide.GuidePageKind.DICTIONARY); 092 break; 093 case TOC: 094 tgt.setValue(ImplementationGuide.GuidePageKind.TOC); 095 break; 096 case RESOURCE: 097 tgt.setValue(ImplementationGuide.GuidePageKind.RESOURCE); 098 break; 099 default: 100 tgt.setValue(ImplementationGuide.GuidePageKind.NULL); 101 break; 102 } 103 } 104 return tgt; 105 } 106 107 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind> convertGuidePageKind(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ImplementationGuide.GuidePageKind> src) throws FHIRException { 108 if (src == null || src.isEmpty()) 109 return null; 110 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKindEnumFactory()); 111 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 112 if (src.getValue() == null) { 113 tgt.setValue(null); 114 } else { 115 switch (src.getValue()) { 116 case PAGE: 117 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.PAGE); 118 break; 119 case EXAMPLE: 120 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.EXAMPLE); 121 break; 122 case LIST: 123 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.LIST); 124 break; 125 case INCLUDE: 126 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.INCLUDE); 127 break; 128 case DIRECTORY: 129 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.DIRECTORY); 130 break; 131 case DICTIONARY: 132 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.DICTIONARY); 133 break; 134 case TOC: 135 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.TOC); 136 break; 137 case RESOURCE: 138 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.RESOURCE); 139 break; 140 default: 141 tgt.setValue(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind.NULL); 142 break; 143 } 144 } 145 return tgt; 146 } 147 148 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu3.model.ImplementationGuide src) throws FHIRException { 149 if (src == null || src.isEmpty()) 150 return null; 151 org.hl7.fhir.dstu2016may.model.ImplementationGuide tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide(); 152 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 153 if (src.hasUrlElement()) 154 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 155 if (src.hasVersion()) 156 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 157 if (src.hasNameElement()) 158 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 159 if (src.hasStatus()) 160 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 161 if (src.hasExperimental()) 162 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 163 if (src.hasPublisher()) 164 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 165 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 166 tgt.addContact(convertImplementationGuideContactComponent(t)); 167 if (src.hasDate()) 168 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 169 if (src.hasDescription()) 170 tgt.setDescription(src.getDescription()); 171 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 172 if (t.hasValueCodeableConcept()) 173 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 174 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 175 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 176 if (src.hasCopyright()) 177 tgt.setCopyright(src.getCopyright()); 178 if (src.hasFhirVersion()) 179 tgt.setFhirVersionElement(Id14_30.convertId(src.getFhirVersionElement())); 180 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 181 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 182 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 183 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 184 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 185 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 186 for (org.hl7.fhir.dstu3.model.UriType t : src.getBinary()) tgt.addBinary(t.getValue()); 187 if (src.hasPage()) 188 tgt.setPage(convertImplementationGuidePageComponent(src.getPage())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.dstu3.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu2016may.model.ImplementationGuide src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.dstu3.model.ImplementationGuide tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide(); 196 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 197 if (src.hasUrlElement()) 198 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 199 if (src.hasVersion()) 200 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 201 if (src.hasNameElement()) 202 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 203 if (src.hasStatus()) 204 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 205 if (src.hasExperimental()) 206 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 207 if (src.hasPublisher()) 208 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 209 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent t : src.getContact()) 210 tgt.addContact(convertImplementationGuideContactComponent(t)); 211 if (src.hasDate()) 212 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 213 if (src.hasDescription()) 214 tgt.setDescription(src.getDescription()); 215 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 216 if (VersionConvertor_14_30.isJurisdiction(t)) 217 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 218 else 219 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 220 if (src.hasCopyright()) 221 tgt.setCopyright(src.getCopyright()); 222 if (src.hasFhirVersion()) 223 tgt.setFhirVersionElement(Id14_30.convertId(src.getFhirVersionElement())); 224 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 225 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 226 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 227 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 228 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 229 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 230 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getBinary()) tgt.addBinary(t.getValue()); 231 if (src.hasPage()) 232 tgt.setPage(convertImplementationGuidePageComponent(src.getPage())); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent convertImplementationGuideContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent(); 240 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 241 if (src.hasName()) 242 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 243 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 244 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 245 return tgt; 246 } 247 248 public static org.hl7.fhir.dstu3.model.ContactDetail convertImplementationGuideContactComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent src) throws FHIRException { 249 if (src == null || src.isEmpty()) 250 return null; 251 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 252 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 253 if (src.hasName()) 254 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 255 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 256 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 257 return tgt; 258 } 259 260 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 261 if (src == null || src.isEmpty()) 262 return null; 263 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 264 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 265 if (src.hasType()) 266 tgt.setTypeElement(convertGuideDependencyType(src.getTypeElement())); 267 if (src.hasUriElement()) 268 tgt.setUriElement(Uri14_30.convertUri(src.getUriElement())); 269 return tgt; 270 } 271 272 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 273 if (src == null || src.isEmpty()) 274 return null; 275 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 276 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 277 if (src.hasType()) 278 tgt.setTypeElement(convertGuideDependencyType(src.getTypeElement())); 279 if (src.hasUriElement()) 280 tgt.setUriElement(Uri14_30.convertUri(src.getUriElement())); 281 return tgt; 282 } 283 284 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 285 if (src == null || src.isEmpty()) 286 return null; 287 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 288 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 289 if (src.hasTypeElement()) 290 tgt.setTypeElement(Code14_30.convertCode(src.getTypeElement())); 291 if (src.hasProfile()) 292 tgt.setProfile(Reference14_30.convertReference(src.getProfile())); 293 return tgt; 294 } 295 296 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 297 if (src == null || src.isEmpty()) 298 return null; 299 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 300 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 301 if (src.hasTypeElement()) 302 tgt.setTypeElement(Code14_30.convertCode(src.getTypeElement())); 303 if (src.hasProfile()) 304 tgt.setProfile(Reference14_30.convertReference(src.getProfile())); 305 return tgt; 306 } 307 308 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 309 if (src == null || src.isEmpty()) 310 return null; 311 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent(); 312 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 313 if (src.hasNameElement()) 314 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 315 if (src.hasDescription()) 316 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 317 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) 318 tgt.addResource(convertImplementationGuidePackageResourceComponent(t)); 319 return tgt; 320 } 321 322 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 323 if (src == null || src.isEmpty()) 324 return null; 325 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageComponent(); 326 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 327 if (src.hasNameElement()) 328 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 329 if (src.hasDescription()) 330 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 331 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) 332 tgt.addResource(convertImplementationGuidePackageResourceComponent(t)); 333 return tgt; 334 } 335 336 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 337 if (src == null || src.isEmpty()) 338 return null; 339 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 340 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 341 if (src.hasExampleElement()) 342 tgt.setExampleElement(Boolean14_30.convertBoolean(src.getExampleElement())); 343 if (src.hasName()) 344 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 345 if (src.hasDescription()) 346 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 347 if (src.hasAcronym()) 348 tgt.setAcronymElement(String14_30.convertString(src.getAcronymElement())); 349 if (src.hasSource()) 350 tgt.setSource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getSource())); 351 if (src.hasExampleFor()) 352 tgt.setExampleFor(Reference14_30.convertReference(src.getExampleFor())); 353 return tgt; 354 } 355 356 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 357 if (src == null || src.isEmpty()) 358 return null; 359 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 360 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 361 if (src.hasExampleElement()) 362 tgt.setExampleElement(Boolean14_30.convertBoolean(src.getExampleElement())); 363 if (src.hasName()) 364 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 365 if (src.hasDescription()) 366 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 367 if (src.hasAcronym()) 368 tgt.setAcronymElement(String14_30.convertString(src.getAcronymElement())); 369 if (src.hasSource()) 370 tgt.setSource(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getSource())); 371 if (src.hasExampleFor()) 372 tgt.setExampleFor(Reference14_30.convertReference(src.getExampleFor())); 373 return tgt; 374 } 375 376 public static org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 377 if (src == null || src.isEmpty()) 378 return null; 379 org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent(); 380 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 381 if (src.hasSourceElement()) 382 tgt.setSourceElement(Uri14_30.convertUri(src.getSourceElement())); 383 if (src.hasNameElement()) 384 tgt.setTitleElement(String14_30.convertString(src.getNameElement())); 385 if (src.hasKind()) 386 tgt.setKindElement(convertGuidePageKind(src.getKindElement())); 387 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getType()) tgt.addType(t.getValue()); 388 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getPackage()) tgt.addPackage(t.getValue()); 389 if (src.hasFormat()) 390 tgt.setFormatElement(Code14_30.convertCode(src.getFormatElement())); 391 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 392 tgt.addPage(convertImplementationGuidePageComponent(t)); 393 return tgt; 394 } 395 396 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 397 if (src == null || src.isEmpty()) 398 return null; 399 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent(); 400 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 401 if (src.hasSourceElement()) 402 tgt.setSourceElement(Uri14_30.convertUri(src.getSourceElement())); 403 if (src.hasTitleElement()) 404 tgt.setNameElement(String14_30.convertString(src.getTitleElement())); 405 if (src.hasKind()) 406 tgt.setKindElement(convertGuidePageKind(src.getKindElement())); 407 for (org.hl7.fhir.dstu3.model.CodeType t : src.getType()) tgt.addType(t.getValue()); 408 for (org.hl7.fhir.dstu3.model.StringType t : src.getPackage()) tgt.addPackage(t.getValue()); 409 if (src.hasFormat()) 410 tgt.setFormatElement(Code14_30.convertCode(src.getFormatElement())); 411 for (org.hl7.fhir.dstu3.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 412 tgt.addPage(convertImplementationGuidePageComponent(t)); 413 return tgt; 414 } 415}