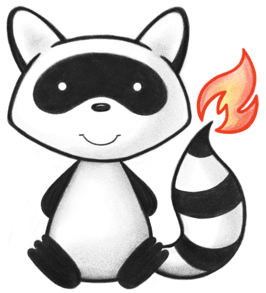
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.Reference14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Identifier14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Integer14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 014import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 015import org.hl7.fhir.dstu3.model.*; 016import org.hl7.fhir.exceptions.FHIRException; 017 018public class Questionnaire14_30 { 019 020 public static org.hl7.fhir.dstu2016may.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu3.model.Questionnaire src) throws FHIRException { 021 if (src == null || src.isEmpty()) 022 return null; 023 org.hl7.fhir.dstu2016may.model.Questionnaire tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire(); 024 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 025 if (src.hasUrl()) 026 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 027 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 028 tgt.addIdentifier(Identifier14_30.convertIdentifier(t)); 029 if (src.hasVersion()) 030 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 031 if (src.hasStatus()) 032 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 033 if (src.hasDate()) 034 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 035 if (src.hasPublisher()) 036 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 037 for (ContactDetail t : src.getContact()) 038 for (org.hl7.fhir.dstu3.model.ContactPoint t1 : t.getTelecom()) 039 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t1)); 040 for (UsageContext t : src.getUseContext()) 041 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 042 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 043 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 044 if (src.hasTitle()) 045 tgt.setTitleElement(String14_30.convertString(src.getTitleElement())); 046 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addConcept(Code14_30.convertCoding(t)); 047 for (org.hl7.fhir.dstu3.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 048 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 049 tgt.addItem(convertQuestionnaireItemComponent(t)); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.dstu3.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2016may.model.Questionnaire src) throws FHIRException { 054 if (src == null || src.isEmpty()) 055 return null; 056 org.hl7.fhir.dstu3.model.Questionnaire tgt = new org.hl7.fhir.dstu3.model.Questionnaire(); 057 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 058 if (src.hasUrl()) 059 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 060 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 061 tgt.addIdentifier(Identifier14_30.convertIdentifier(t)); 062 if (src.hasVersion()) 063 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 064 if (src.hasStatus()) 065 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 066 if (src.hasDate()) 067 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 068 if (src.hasPublisher()) 069 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 070 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 071 tgt.addContact(convertQuestionnaireContactComponent(t)); 072 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 073 if (VersionConvertor_14_30.isJurisdiction(t)) 074 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 075 else 076 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 077 if (src.hasTitle()) 078 tgt.setTitleElement(String14_30.convertString(src.getTitleElement())); 079 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Code14_30.convertCoding(t)); 080 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 081 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 082 tgt.addItem(convertQuestionnaireItemComponent(t)); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.dstu3.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2016may.model.ContactPoint src) throws FHIRException { 087 if (src == null || src.isEmpty()) 088 return null; 089 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 090 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 091 tgt.addTelecom(ContactPoint14_30.convertContactPoint(src)); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 096 if (src == null || src.isEmpty()) 097 return null; 098 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent(); 099 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 100 if (src.hasLinkId()) 101 tgt.setLinkIdElement(String14_30.convertString(src.getLinkIdElement())); 102 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Code14_30.convertCoding(t)); 103 if (src.hasPrefix()) 104 tgt.setPrefixElement(String14_30.convertString(src.getPrefixElement())); 105 if (src.hasText()) 106 tgt.setTextElement(String14_30.convertString(src.getTextElement())); 107 if (src.hasType()) 108 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 109 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 110 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 111 if (src.hasRequired()) 112 tgt.setRequiredElement(Boolean14_30.convertBoolean(src.getRequiredElement())); 113 if (src.hasRepeats()) 114 tgt.setRepeatsElement(Boolean14_30.convertBoolean(src.getRepeatsElement())); 115 if (src.hasReadOnly()) 116 tgt.setReadOnlyElement(Boolean14_30.convertBoolean(src.getReadOnlyElement())); 117 if (src.hasMaxLength()) 118 tgt.setMaxLengthElement(Integer14_30.convertInteger(src.getMaxLengthElement())); 119 if (src.hasOptions()) 120 tgt.setOptions(Reference14_30.convertReference(src.getOptions())); 121 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 122 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 123 if (src.hasInitial()) 124 tgt.setInitial(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getInitial())); 125 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 126 tgt.addItem(convertQuestionnaireItemComponent(t)); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent(); 134 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 135 if (src.hasLinkId()) 136 tgt.setLinkIdElement(String14_30.convertString(src.getLinkIdElement())); 137 for (org.hl7.fhir.dstu3.model.Coding t : src.getCode()) tgt.addConcept(Code14_30.convertCoding(t)); 138 if (src.hasPrefix()) 139 tgt.setPrefixElement(String14_30.convertString(src.getPrefixElement())); 140 if (src.hasText()) 141 tgt.setTextElement(String14_30.convertString(src.getTextElement())); 142 if (src.hasType()) 143 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 144 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 145 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 146 if (src.hasRequired()) 147 tgt.setRequiredElement(Boolean14_30.convertBoolean(src.getRequiredElement())); 148 if (src.hasRepeats()) 149 tgt.setRepeatsElement(Boolean14_30.convertBoolean(src.getRepeatsElement())); 150 if (src.hasReadOnly()) 151 tgt.setReadOnlyElement(Boolean14_30.convertBoolean(src.getReadOnlyElement())); 152 if (src.hasMaxLength()) 153 tgt.setMaxLengthElement(Integer14_30.convertInteger(src.getMaxLengthElement())); 154 if (src.hasOptions()) 155 tgt.setOptions(Reference14_30.convertReference(src.getOptions())); 156 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 157 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 158 if (src.hasInitial()) 159 tgt.setInitial(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getInitial())); 160 for (org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 161 tgt.addItem(convertQuestionnaireItemComponent(t)); 162 return tgt; 163 } 164 165 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 166 if (src == null || src.isEmpty()) 167 return null; 168 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 169 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 170 if (src.hasQuestionElement()) 171 tgt.setQuestionElement(String14_30.convertString(src.getQuestionElement())); 172 if (src.hasHasAnswer()) 173 tgt.setAnsweredElement(Boolean14_30.convertBoolean(src.getHasAnswerElement())); 174 if (src.hasAnswer()) 175 tgt.setAnswer(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getAnswer())); 176 return tgt; 177 } 178 179 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 183 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 184 if (src.hasQuestionElement()) 185 tgt.setQuestionElement(String14_30.convertString(src.getQuestionElement())); 186 if (src.hasAnswered()) 187 tgt.setHasAnswerElement(Boolean14_30.convertBoolean(src.getAnsweredElement())); 188 if (src.hasAnswer()) 189 tgt.setAnswer(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getAnswer())); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 194 if (src == null || src.isEmpty()) 195 return null; 196 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent(); 197 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 198 if (src.hasValue()) 199 tgt.setValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValue())); 200 return tgt; 201 } 202 203 public static org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 204 if (src == null || src.isEmpty()) 205 return null; 206 org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemOptionComponent(); 207 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 208 if (src.hasValue()) 209 tgt.setValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValue())); 210 return tgt; 211 } 212 213 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 214 if (src == null || src.isEmpty()) 215 return null; 216 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 217 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 218 if (src.getValue() == null) { 219 tgt.setValue(null); 220 } else { 221 switch (src.getValue()) { 222 case GROUP: 223 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 224 break; 225 case DISPLAY: 226 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 227 break; 228 case QUESTION: 229 tgt.setValue(Questionnaire.QuestionnaireItemType.QUESTION); 230 break; 231 case BOOLEAN: 232 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 233 break; 234 case DECIMAL: 235 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 236 break; 237 case INTEGER: 238 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 239 break; 240 case DATE: 241 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 242 break; 243 case DATETIME: 244 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 245 break; 246 case INSTANT: 247 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 248 break; 249 case TIME: 250 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 251 break; 252 case STRING: 253 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 254 break; 255 case TEXT: 256 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 257 break; 258 case URL: 259 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 260 break; 261 case CHOICE: 262 tgt.setValue(Questionnaire.QuestionnaireItemType.CHOICE); 263 break; 264 case OPENCHOICE: 265 tgt.setValue(Questionnaire.QuestionnaireItemType.OPENCHOICE); 266 break; 267 case ATTACHMENT: 268 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 269 break; 270 case REFERENCE: 271 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 272 break; 273 case QUANTITY: 274 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 275 break; 276 default: 277 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 278 break; 279 } 280 } 281 return tgt; 282 } 283 284 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 285 if (src == null || src.isEmpty()) 286 return null; 287 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 288 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 289 if (src.getValue() == null) { 290 tgt.setValue(null); 291 } else { 292 switch (src.getValue()) { 293 case GROUP: 294 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.GROUP); 295 break; 296 case DISPLAY: 297 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DISPLAY); 298 break; 299 case QUESTION: 300 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.QUESTION); 301 break; 302 case BOOLEAN: 303 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.BOOLEAN); 304 break; 305 case DECIMAL: 306 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DECIMAL); 307 break; 308 case INTEGER: 309 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.INTEGER); 310 break; 311 case DATE: 312 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DATE); 313 break; 314 case DATETIME: 315 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DATETIME); 316 break; 317 case TIME: 318 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.TIME); 319 break; 320 case STRING: 321 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.STRING); 322 break; 323 case TEXT: 324 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.TEXT); 325 break; 326 case URL: 327 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.URL); 328 break; 329 case CHOICE: 330 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.CHOICE); 331 break; 332 case OPENCHOICE: 333 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.OPENCHOICE); 334 break; 335 case ATTACHMENT: 336 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.ATTACHMENT); 337 break; 338 case REFERENCE: 339 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.REFERENCE); 340 break; 341 case QUANTITY: 342 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.QUANTITY); 343 break; 344 default: 345 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.NULL); 346 break; 347 } 348 } 349 return tgt; 350 } 351 352 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> src) throws FHIRException { 353 if (src == null || src.isEmpty()) 354 return null; 355 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatusEnumFactory()); 356 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 357 if (src.getValue() == null) { 358 tgt.setValue(null); 359 } else { 360 switch (src.getValue()) { 361 case DRAFT: 362 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.DRAFT); 363 break; 364 case ACTIVE: 365 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.PUBLISHED); 366 break; 367 case RETIRED: 368 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.RETIRED); 369 break; 370 default: 371 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.NULL); 372 break; 373 } 374 } 375 return tgt; 376 } 377 378 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 379 if (src == null || src.isEmpty()) 380 return null; 381 Enumeration<Enumerations.PublicationStatus> tgt = new Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 382 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 383 if (src.getValue() == null) { 384 tgt.setValue(null); 385 } else { 386 switch (src.getValue()) { 387 case DRAFT: 388 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 389 break; 390 case PUBLISHED: 391 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 392 break; 393 case RETIRED: 394 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 395 break; 396 default: 397 tgt.setValue(Enumerations.PublicationStatus.NULL); 398 break; 399 } 400 } 401 return tgt; 402 } 403}