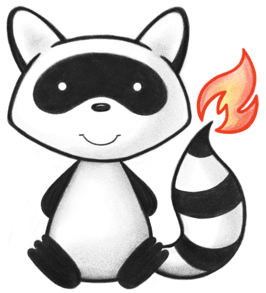
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.Reference14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Identifier14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Id14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Integer14_30; 014import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 015import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 016import org.hl7.fhir.dstu3.model.Enumeration; 017import org.hl7.fhir.dstu3.model.TestScript; 018import org.hl7.fhir.exceptions.FHIRException; 019 020public class TestScript14_30 { 021 022 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType> src) throws FHIRException { 023 if (src == null || src.isEmpty()) 024 return null; 025 Enumeration<TestScript.AssertionDirectionType> tgt = new Enumeration<>(new TestScript.AssertionDirectionTypeEnumFactory()); 026 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 027 if (src.getValue() == null) { 028 tgt.setValue(null); 029 } else { 030 switch (src.getValue()) { 031 case RESPONSE: 032 tgt.setValue(TestScript.AssertionDirectionType.RESPONSE); 033 break; 034 case REQUEST: 035 tgt.setValue(TestScript.AssertionDirectionType.REQUEST); 036 break; 037 default: 038 tgt.setValue(TestScript.AssertionDirectionType.NULL); 039 break; 040 } 041 } 042 return tgt; 043 } 044 045 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType> convertAssertionDirectionType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionDirectionType> src) throws FHIRException { 046 if (src == null || src.isEmpty()) 047 return null; 048 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionTypeEnumFactory()); 049 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 050 if (src.getValue() == null) { 051 tgt.setValue(null); 052 } else { 053 switch (src.getValue()) { 054 case RESPONSE: 055 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType.RESPONSE); 056 break; 057 case REQUEST: 058 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType.REQUEST); 059 break; 060 default: 061 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionDirectionType.NULL); 062 break; 063 } 064 } 065 return tgt; 066 } 067 068 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> src) throws FHIRException { 069 if (src == null || src.isEmpty()) 070 return null; 071 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorTypeEnumFactory()); 072 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 073 if (src.getValue() == null) { 074 tgt.setValue(null); 075 } else { 076 switch (src.getValue()) { 077 case EQUALS: 078 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.EQUALS); 079 break; 080 case NOTEQUALS: 081 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.NOTEQUALS); 082 break; 083 case IN: 084 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.IN); 085 break; 086 case NOTIN: 087 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.NOTIN); 088 break; 089 case GREATERTHAN: 090 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.GREATERTHAN); 091 break; 092 case LESSTHAN: 093 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.LESSTHAN); 094 break; 095 case EMPTY: 096 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.EMPTY); 097 break; 098 case NOTEMPTY: 099 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.NOTEMPTY); 100 break; 101 case CONTAINS: 102 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.CONTAINS); 103 break; 104 case NOTCONTAINS: 105 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.NOTCONTAINS); 106 break; 107 default: 108 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType.NULL); 109 break; 110 } 111 } 112 return tgt; 113 } 114 115 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionOperatorType> convertAssertionOperatorType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionOperatorType> src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 Enumeration<TestScript.AssertionOperatorType> tgt = new Enumeration<>(new TestScript.AssertionOperatorTypeEnumFactory()); 119 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 120 if (src.getValue() == null) { 121 tgt.setValue(null); 122 } else { 123 switch (src.getValue()) { 124 case EQUALS: 125 tgt.setValue(TestScript.AssertionOperatorType.EQUALS); 126 break; 127 case NOTEQUALS: 128 tgt.setValue(TestScript.AssertionOperatorType.NOTEQUALS); 129 break; 130 case IN: 131 tgt.setValue(TestScript.AssertionOperatorType.IN); 132 break; 133 case NOTIN: 134 tgt.setValue(TestScript.AssertionOperatorType.NOTIN); 135 break; 136 case GREATERTHAN: 137 tgt.setValue(TestScript.AssertionOperatorType.GREATERTHAN); 138 break; 139 case LESSTHAN: 140 tgt.setValue(TestScript.AssertionOperatorType.LESSTHAN); 141 break; 142 case EMPTY: 143 tgt.setValue(TestScript.AssertionOperatorType.EMPTY); 144 break; 145 case NOTEMPTY: 146 tgt.setValue(TestScript.AssertionOperatorType.NOTEMPTY); 147 break; 148 case CONTAINS: 149 tgt.setValue(TestScript.AssertionOperatorType.CONTAINS); 150 break; 151 case NOTCONTAINS: 152 tgt.setValue(TestScript.AssertionOperatorType.NOTCONTAINS); 153 break; 154 default: 155 tgt.setValue(TestScript.AssertionOperatorType.NULL); 156 break; 157 } 158 } 159 return tgt; 160 } 161 162 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 163 if (src == null || src.isEmpty()) 164 return null; 165 Enumeration<TestScript.AssertionResponseTypes> tgt = new Enumeration<>(new TestScript.AssertionResponseTypesEnumFactory()); 166 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 167 if (src.getValue() == null) { 168 tgt.setValue(null); 169 } else { 170 switch (src.getValue()) { 171 case OKAY: 172 tgt.setValue(TestScript.AssertionResponseTypes.OKAY); 173 break; 174 case CREATED: 175 tgt.setValue(TestScript.AssertionResponseTypes.CREATED); 176 break; 177 case NOCONTENT: 178 tgt.setValue(TestScript.AssertionResponseTypes.NOCONTENT); 179 break; 180 case NOTMODIFIED: 181 tgt.setValue(TestScript.AssertionResponseTypes.NOTMODIFIED); 182 break; 183 case BAD: 184 tgt.setValue(TestScript.AssertionResponseTypes.BAD); 185 break; 186 case FORBIDDEN: 187 tgt.setValue(TestScript.AssertionResponseTypes.FORBIDDEN); 188 break; 189 case NOTFOUND: 190 tgt.setValue(TestScript.AssertionResponseTypes.NOTFOUND); 191 break; 192 case METHODNOTALLOWED: 193 tgt.setValue(TestScript.AssertionResponseTypes.METHODNOTALLOWED); 194 break; 195 case CONFLICT: 196 tgt.setValue(TestScript.AssertionResponseTypes.CONFLICT); 197 break; 198 case GONE: 199 tgt.setValue(TestScript.AssertionResponseTypes.GONE); 200 break; 201 case PRECONDITIONFAILED: 202 tgt.setValue(TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 203 break; 204 case UNPROCESSABLE: 205 tgt.setValue(TestScript.AssertionResponseTypes.UNPROCESSABLE); 206 break; 207 default: 208 tgt.setValue(TestScript.AssertionResponseTypes.NULL); 209 break; 210 } 211 } 212 return tgt; 213 } 214 215 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes> convertAssertionResponseTypes(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.AssertionResponseTypes> src) throws FHIRException { 216 if (src == null || src.isEmpty()) 217 return null; 218 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypesEnumFactory()); 219 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 220 if (src.getValue() == null) { 221 tgt.setValue(null); 222 } else { 223 switch (src.getValue()) { 224 case OKAY: 225 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.OKAY); 226 break; 227 case CREATED: 228 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.CREATED); 229 break; 230 case NOCONTENT: 231 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.NOCONTENT); 232 break; 233 case NOTMODIFIED: 234 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.NOTMODIFIED); 235 break; 236 case BAD: 237 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.BAD); 238 break; 239 case FORBIDDEN: 240 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.FORBIDDEN); 241 break; 242 case NOTFOUND: 243 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.NOTFOUND); 244 break; 245 case METHODNOTALLOWED: 246 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.METHODNOTALLOWED); 247 break; 248 case CONFLICT: 249 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.CONFLICT); 250 break; 251 case GONE: 252 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.GONE); 253 break; 254 case PRECONDITIONFAILED: 255 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.PRECONDITIONFAILED); 256 break; 257 case UNPROCESSABLE: 258 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.UNPROCESSABLE); 259 break; 260 default: 261 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.AssertionResponseTypes.NULL); 262 break; 263 } 264 } 265 return tgt; 266 } 267 268 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.ContentType> convertContentType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.ContentType> src) throws FHIRException { 269 if (src == null || src.isEmpty()) 270 return null; 271 Enumeration<TestScript.ContentType> tgt = new Enumeration<>(new TestScript.ContentTypeEnumFactory()); 272 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 273 if (src.getValue() == null) { 274 tgt.setValue(null); 275 } else { 276 switch (src.getValue()) { 277 case XML: 278 tgt.setValue(TestScript.ContentType.XML); 279 break; 280 case JSON: 281 tgt.setValue(TestScript.ContentType.JSON); 282 break; 283 default: 284 tgt.setValue(TestScript.ContentType.NULL); 285 break; 286 } 287 } 288 return tgt; 289 } 290 291 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.ContentType> convertContentType(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.TestScript.ContentType> src) throws FHIRException { 292 if (src == null || src.isEmpty()) 293 return null; 294 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.TestScript.ContentType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.TestScript.ContentTypeEnumFactory()); 295 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 296 if (src.getValue() == null) { 297 tgt.setValue(null); 298 } else { 299 switch (src.getValue()) { 300 case XML: 301 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.ContentType.XML); 302 break; 303 case JSON: 304 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.ContentType.JSON); 305 break; 306 default: 307 tgt.setValue(org.hl7.fhir.dstu2016may.model.TestScript.ContentType.NULL); 308 break; 309 } 310 } 311 return tgt; 312 } 313 314 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 315 if (src == null || src.isEmpty()) 316 return null; 317 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertComponent(); 318 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 319 if (src.hasLabel()) 320 tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 321 if (src.hasDescription()) 322 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 323 if (src.hasDirection()) 324 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 325 if (src.hasCompareToSourceId()) 326 tgt.setCompareToSourceIdElement(String14_30.convertString(src.getCompareToSourceIdElement())); 327 if (src.hasCompareToSourcePath()) 328 tgt.setCompareToSourcePathElement(String14_30.convertString(src.getCompareToSourcePathElement())); 329 if (src.hasContentType()) 330 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 331 if (src.hasHeaderField()) 332 tgt.setHeaderFieldElement(String14_30.convertString(src.getHeaderFieldElement())); 333 if (src.hasMinimumId()) 334 tgt.setMinimumIdElement(String14_30.convertString(src.getMinimumIdElement())); 335 if (src.hasNavigationLinks()) 336 tgt.setNavigationLinksElement(Boolean14_30.convertBoolean(src.getNavigationLinksElement())); 337 if (src.hasOperator()) 338 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 339 if (src.hasPath()) 340 tgt.setPathElement(String14_30.convertString(src.getPathElement())); 341 if (src.hasResource()) 342 tgt.setResourceElement(Code14_30.convertCode(src.getResourceElement())); 343 if (src.hasResponse()) 344 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 345 if (src.hasResponseCode()) 346 tgt.setResponseCodeElement(String14_30.convertString(src.getResponseCodeElement())); 347 if (src.hasRule()) 348 tgt.setRule(convertSetupActionAssertRuleComponent(src.getRule())); 349 if (src.hasRuleset()) 350 tgt.setRuleset(convertSetupActionAssertRulesetComponent(src.getRuleset())); 351 if (src.hasSourceId()) 352 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 353 if (src.hasValidateProfileId()) 354 tgt.setValidateProfileIdElement(Id14_30.convertId(src.getValidateProfileIdElement())); 355 if (src.hasValue()) 356 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 357 if (src.hasWarningOnly()) 358 tgt.setWarningOnlyElement(Boolean14_30.convertBoolean(src.getWarningOnlyElement())); 359 return tgt; 360 } 361 362 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent convertSetupActionAssertComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertComponent src) throws FHIRException { 363 if (src == null || src.isEmpty()) 364 return null; 365 org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionAssertComponent(); 366 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 367 if (src.hasLabel()) 368 tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 369 if (src.hasDescription()) 370 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 371 if (src.hasDirection()) 372 tgt.setDirectionElement(convertAssertionDirectionType(src.getDirectionElement())); 373 if (src.hasCompareToSourceId()) 374 tgt.setCompareToSourceIdElement(String14_30.convertString(src.getCompareToSourceIdElement())); 375 if (src.hasCompareToSourcePath()) 376 tgt.setCompareToSourcePathElement(String14_30.convertString(src.getCompareToSourcePathElement())); 377 if (src.hasContentType()) 378 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 379 if (src.hasHeaderField()) 380 tgt.setHeaderFieldElement(String14_30.convertString(src.getHeaderFieldElement())); 381 if (src.hasMinimumId()) 382 tgt.setMinimumIdElement(String14_30.convertString(src.getMinimumIdElement())); 383 if (src.hasNavigationLinks()) 384 tgt.setNavigationLinksElement(Boolean14_30.convertBoolean(src.getNavigationLinksElement())); 385 if (src.hasOperator()) 386 tgt.setOperatorElement(convertAssertionOperatorType(src.getOperatorElement())); 387 if (src.hasPath()) 388 tgt.setPathElement(String14_30.convertString(src.getPathElement())); 389 if (src.hasResource()) 390 tgt.setResourceElement(Code14_30.convertCode(src.getResourceElement())); 391 if (src.hasResponse()) 392 tgt.setResponseElement(convertAssertionResponseTypes(src.getResponseElement())); 393 if (src.hasResponseCode()) 394 tgt.setResponseCodeElement(String14_30.convertString(src.getResponseCodeElement())); 395 if (src.hasRule()) 396 tgt.setRule(convertSetupActionAssertRuleComponent(src.getRule())); 397 if (src.hasRuleset()) 398 tgt.setRuleset(convertSetupActionAssertRulesetComponent(src.getRuleset())); 399 if (src.hasSourceId()) 400 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 401 if (src.hasValidateProfileId()) 402 tgt.setValidateProfileIdElement(Id14_30.convertId(src.getValidateProfileIdElement())); 403 if (src.hasValue()) 404 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 405 if (src.hasWarningOnly()) 406 tgt.setWarningOnlyElement(Boolean14_30.convertBoolean(src.getWarningOnlyElement())); 407 return tgt; 408 } 409 410 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleComponent convertSetupActionAssertRuleComponent(org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleComponent src) throws FHIRException { 411 if (src == null || src.isEmpty()) 412 return null; 413 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleComponent(); 414 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 415 for (org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleParamComponent t : src.getParam()) 416 tgt.addParam(convertSetupActionAssertRuleParamComponent(t)); 417 return tgt; 418 } 419 420 public static org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleComponent convertSetupActionAssertRuleComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleComponent src) throws FHIRException { 421 if (src == null || src.isEmpty()) 422 return null; 423 org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleComponent(); 424 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 425 for (org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleParamComponent t : src.getParam()) 426 tgt.addParam(convertSetupActionAssertRuleParamComponent(t)); 427 return tgt; 428 } 429 430 public static org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleParamComponent convertSetupActionAssertRuleParamComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleParamComponent src) throws FHIRException { 431 if (src == null || src.isEmpty()) 432 return null; 433 org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleParamComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleParamComponent(); 434 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 435 if (src.hasNameElement()) 436 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 437 if (src.hasValue()) 438 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 439 return tgt; 440 } 441 442 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleParamComponent convertSetupActionAssertRuleParamComponent(org.hl7.fhir.dstu3.model.TestScript.ActionAssertRuleParamComponent src) throws FHIRException { 443 if (src == null || src.isEmpty()) 444 return null; 445 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleParamComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRuleParamComponent(); 446 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 447 if (src.hasNameElement()) 448 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 449 if (src.hasValue()) 450 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 451 return tgt; 452 } 453 454 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetComponent convertSetupActionAssertRulesetComponent(org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetComponent src) throws FHIRException { 455 if (src == null || src.isEmpty()) 456 return null; 457 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetComponent(); 458 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 459 for (org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleComponent t : src.getRule()) 460 tgt.addRule(convertSetupActionAssertRulesetRuleComponent(t)); 461 return tgt; 462 } 463 464 public static org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetComponent convertSetupActionAssertRulesetComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetComponent src) throws FHIRException { 465 if (src == null || src.isEmpty()) 466 return null; 467 org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetComponent(); 468 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 469 for (org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleComponent t : src.getRule()) 470 tgt.addRule(convertSetupActionAssertRulesetRuleComponent(t)); 471 return tgt; 472 } 473 474 public static org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleComponent convertSetupActionAssertRulesetRuleComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleComponent src) throws FHIRException { 475 if (src == null || src.isEmpty()) 476 return null; 477 org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleComponent(); 478 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 479 for (org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleParamComponent t : src.getParam()) 480 tgt.addParam(convertSetupActionAssertRulesetRuleParamComponent(t)); 481 return tgt; 482 } 483 484 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleComponent convertSetupActionAssertRulesetRuleComponent(org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleComponent src) throws FHIRException { 485 if (src == null || src.isEmpty()) 486 return null; 487 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleComponent(); 488 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 489 for (org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleParamComponent t : src.getParam()) 490 tgt.addParam(convertSetupActionAssertRulesetRuleParamComponent(t)); 491 return tgt; 492 } 493 494 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleParamComponent convertSetupActionAssertRulesetRuleParamComponent(org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleParamComponent src) throws FHIRException { 495 if (src == null || src.isEmpty()) 496 return null; 497 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleParamComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleParamComponent(); 498 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 499 if (src.hasNameElement()) 500 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 501 if (src.hasValue()) 502 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 503 return tgt; 504 } 505 506 public static org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleParamComponent convertSetupActionAssertRulesetRuleParamComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionAssertRulesetRuleParamComponent src) throws FHIRException { 507 if (src == null || src.isEmpty()) 508 return null; 509 org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleParamComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.ActionAssertRulesetRuleParamComponent(); 510 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 511 if (src.hasNameElement()) 512 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 513 if (src.hasValue()) 514 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 515 return tgt; 516 } 517 518 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent src) throws FHIRException { 519 if (src == null || src.isEmpty()) 520 return null; 521 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionComponent(); 522 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 523 if (src.hasOperation()) 524 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 525 if (src.hasAssert()) 526 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 527 return tgt; 528 } 529 530 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent convertSetupActionComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionComponent src) throws FHIRException { 531 if (src == null || src.isEmpty()) 532 return null; 533 org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent(); 534 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 535 if (src.hasOperation()) 536 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 537 if (src.hasAssert()) 538 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 539 return tgt; 540 } 541 542 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 543 if (src == null || src.isEmpty()) 544 return null; 545 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent(); 546 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 547 if (src.hasType()) 548 tgt.setType(Code14_30.convertCoding(src.getType())); 549 if (src.hasResource()) 550 tgt.setResourceElement(Code14_30.convertCode(src.getResourceElement())); 551 if (src.hasLabel()) 552 tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 553 if (src.hasDescription()) 554 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 555 if (src.hasAccept()) 556 tgt.setAcceptElement(convertContentType(src.getAcceptElement())); 557 if (src.hasContentType()) 558 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 559 if (src.hasDestination()) 560 tgt.setDestinationElement(Integer14_30.convertInteger(src.getDestinationElement())); 561 if (src.hasEncodeRequestUrl()) 562 tgt.setEncodeRequestUrlElement(Boolean14_30.convertBoolean(src.getEncodeRequestUrlElement())); 563 if (src.hasOrigin()) 564 tgt.setOriginElement(Integer14_30.convertInteger(src.getOriginElement())); 565 if (src.hasParams()) 566 tgt.setParamsElement(String14_30.convertString(src.getParamsElement())); 567 for (org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 568 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 569 if (src.hasResponseId()) 570 tgt.setResponseIdElement(Id14_30.convertId(src.getResponseIdElement())); 571 if (src.hasSourceId()) 572 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 573 if (src.hasTargetId()) 574 tgt.setTargetId(src.getTargetId()); 575 if (src.hasUrl()) 576 tgt.setUrlElement(String14_30.convertString(src.getUrlElement())); 577 return tgt; 578 } 579 580 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationComponent convertSetupActionOperationComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationComponent src) throws FHIRException { 581 if (src == null || src.isEmpty()) 582 return null; 583 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationComponent(); 584 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 585 if (src.hasResource()) 586 tgt.setResourceElement(Code14_30.convertCode(src.getResourceElement())); 587 if (src.hasLabel()) 588 tgt.setLabelElement(String14_30.convertString(src.getLabelElement())); 589 if (src.hasDescription()) 590 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 591 if (src.hasAccept()) 592 tgt.setAcceptElement(convertContentType(src.getAcceptElement())); 593 if (src.hasContentType()) 594 tgt.setContentTypeElement(convertContentType(src.getContentTypeElement())); 595 if (src.hasDestination()) 596 tgt.setDestinationElement(Integer14_30.convertInteger(src.getDestinationElement())); 597 if (src.hasEncodeRequestUrl()) 598 tgt.setEncodeRequestUrlElement(Boolean14_30.convertBoolean(src.getEncodeRequestUrlElement())); 599 if (src.hasOrigin()) 600 tgt.setOriginElement(Integer14_30.convertInteger(src.getOriginElement())); 601 if (src.hasParams()) 602 tgt.setParamsElement(String14_30.convertString(src.getParamsElement())); 603 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent t : src.getRequestHeader()) 604 tgt.addRequestHeader(convertSetupActionOperationRequestHeaderComponent(t)); 605 if (src.hasResponseId()) 606 tgt.setResponseIdElement(Id14_30.convertId(src.getResponseIdElement())); 607 if (src.hasSourceId()) 608 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 609 if (src.hasTargetId()) 610 tgt.setTargetId(src.getTargetId()); 611 if (src.hasUrl()) 612 tgt.setUrlElement(String14_30.convertString(src.getUrlElement())); 613 return tgt; 614 } 615 616 public static org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 617 if (src == null || src.isEmpty()) 618 return null; 619 org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent(); 620 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 621 if (src.hasFieldElement()) 622 tgt.setFieldElement(String14_30.convertString(src.getFieldElement())); 623 if (src.hasValueElement()) 624 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 625 return tgt; 626 } 627 628 public static org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationRequestHeaderComponent convertSetupActionOperationRequestHeaderComponent(org.hl7.fhir.dstu3.model.TestScript.SetupActionOperationRequestHeaderComponent src) throws FHIRException { 629 if (src == null || src.isEmpty()) 630 return null; 631 org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationRequestHeaderComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.SetupActionOperationRequestHeaderComponent(); 632 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 633 if (src.hasFieldElement()) 634 tgt.setFieldElement(String14_30.convertString(src.getFieldElement())); 635 if (src.hasValueElement()) 636 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 637 return tgt; 638 } 639 640 public static org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu2016may.model.TestScript.TeardownActionComponent src) throws FHIRException { 641 if (src == null || src.isEmpty()) 642 return null; 643 org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent(); 644 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 645 if (src.hasOperation()) 646 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 647 return tgt; 648 } 649 650 public static org.hl7.fhir.dstu2016may.model.TestScript.TeardownActionComponent convertTeardownActionComponent(org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent src) throws FHIRException { 651 if (src == null || src.isEmpty()) 652 return null; 653 org.hl7.fhir.dstu2016may.model.TestScript.TeardownActionComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TeardownActionComponent(); 654 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 655 if (src.hasOperation()) 656 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 657 return tgt; 658 } 659 660 public static org.hl7.fhir.dstu2016may.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu3.model.TestScript.TestActionComponent src) throws FHIRException { 661 if (src == null || src.isEmpty()) 662 return null; 663 org.hl7.fhir.dstu2016may.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestActionComponent(); 664 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 665 if (src.hasOperation()) 666 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 667 if (src.hasAssert()) 668 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 669 return tgt; 670 } 671 672 public static org.hl7.fhir.dstu3.model.TestScript.TestActionComponent convertTestActionComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestActionComponent src) throws FHIRException { 673 if (src == null || src.isEmpty()) 674 return null; 675 org.hl7.fhir.dstu3.model.TestScript.TestActionComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestActionComponent(); 676 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 677 if (src.hasOperation()) 678 tgt.setOperation(convertSetupActionOperationComponent(src.getOperation())); 679 if (src.hasAssert()) 680 tgt.setAssert(convertSetupActionAssertComponent(src.getAssert())); 681 return tgt; 682 } 683 684 public static org.hl7.fhir.dstu3.model.TestScript convertTestScript(org.hl7.fhir.dstu2016may.model.TestScript src) throws FHIRException { 685 if (src == null || src.isEmpty()) 686 return null; 687 org.hl7.fhir.dstu3.model.TestScript tgt = new org.hl7.fhir.dstu3.model.TestScript(); 688 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 689 if (src.hasUrlElement()) 690 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 691 if (src.hasVersion()) 692 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 693 if (src.hasNameElement()) 694 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 695 if (src.hasStatus()) 696 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 697 if (src.hasIdentifier()) 698 tgt.setIdentifier(Identifier14_30.convertIdentifier(src.getIdentifier())); 699 if (src.hasExperimental()) 700 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 701 if (src.hasPublisher()) 702 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 703 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptContactComponent t : src.getContact()) 704 tgt.addContact(convertTestScriptContactComponent(t)); 705 if (src.hasDate()) 706 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 707 if (src.hasDescription()) 708 tgt.setDescription(src.getDescription()); 709 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 710 if (VersionConvertor_14_30.isJurisdiction(t)) 711 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 712 else 713 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 714 if (src.hasRequirements()) 715 tgt.setPurpose(src.getRequirements()); 716 if (src.hasCopyright()) 717 tgt.setCopyright(src.getCopyright()); 718 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 719 tgt.addOrigin(convertTestScriptOriginComponent(t)); 720 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 721 tgt.addDestination(convertTestScriptDestinationComponent(t)); 722 if (src.hasMetadata()) 723 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 724 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 725 tgt.addFixture(convertTestScriptFixtureComponent(t)); 726 for (org.hl7.fhir.dstu2016may.model.Reference t : src.getProfile()) 727 tgt.addProfile(Reference14_30.convertReference(t)); 728 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 729 tgt.addVariable(convertTestScriptVariableComponent(t)); 730 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleComponent t : src.getRule()) 731 tgt.addRule(convertTestScriptRuleComponent(t)); 732 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetComponent t : src.getRuleset()) 733 tgt.addRuleset(convertTestScriptRulesetComponent(t)); 734 if (src.hasSetup()) 735 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 736 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTestComponent t : src.getTest()) 737 tgt.addTest(convertTestScriptTestComponent(t)); 738 if (src.hasTeardown()) 739 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 740 return tgt; 741 } 742 743 public static org.hl7.fhir.dstu2016may.model.TestScript convertTestScript(org.hl7.fhir.dstu3.model.TestScript src) throws FHIRException { 744 if (src == null || src.isEmpty()) 745 return null; 746 org.hl7.fhir.dstu2016may.model.TestScript tgt = new org.hl7.fhir.dstu2016may.model.TestScript(); 747 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 748 if (src.hasUrlElement()) 749 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 750 if (src.hasVersion()) 751 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 752 if (src.hasNameElement()) 753 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 754 if (src.hasStatus()) 755 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 756 if (src.hasIdentifier()) 757 tgt.setIdentifier(Identifier14_30.convertIdentifier(src.getIdentifier())); 758 if (src.hasExperimental()) 759 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 760 if (src.hasPublisher()) 761 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 762 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 763 tgt.addContact(convertTestScriptContactComponent(t)); 764 if (src.hasDate()) 765 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 766 if (src.hasDescription()) 767 tgt.setDescription(src.getDescription()); 768 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 769 if (t.hasValueCodeableConcept()) 770 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 771 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 772 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 773 if (src.hasPurpose()) 774 tgt.setRequirements(src.getPurpose()); 775 if (src.hasCopyright()) 776 tgt.setCopyright(src.getCopyright()); 777 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent t : src.getOrigin()) 778 tgt.addOrigin(convertTestScriptOriginComponent(t)); 779 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent t : src.getDestination()) 780 tgt.addDestination(convertTestScriptDestinationComponent(t)); 781 if (src.hasMetadata()) 782 tgt.setMetadata(convertTestScriptMetadataComponent(src.getMetadata())); 783 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent t : src.getFixture()) 784 tgt.addFixture(convertTestScriptFixtureComponent(t)); 785 for (org.hl7.fhir.dstu3.model.Reference t : src.getProfile()) tgt.addProfile(Reference14_30.convertReference(t)); 786 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent t : src.getVariable()) 787 tgt.addVariable(convertTestScriptVariableComponent(t)); 788 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptRuleComponent t : src.getRule()) 789 tgt.addRule(convertTestScriptRuleComponent(t)); 790 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptRulesetComponent t : src.getRuleset()) 791 tgt.addRuleset(convertTestScriptRulesetComponent(t)); 792 if (src.hasSetup()) 793 tgt.setSetup(convertTestScriptSetupComponent(src.getSetup())); 794 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent t : src.getTest()) 795 tgt.addTest(convertTestScriptTestComponent(t)); 796 if (src.hasTeardown()) 797 tgt.setTeardown(convertTestScriptTeardownComponent(src.getTeardown())); 798 return tgt; 799 } 800 801 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptContactComponent convertTestScriptContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 802 if (src == null || src.isEmpty()) 803 return null; 804 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptContactComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptContactComponent(); 805 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 806 if (src.hasName()) 807 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 808 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 809 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 810 return tgt; 811 } 812 813 public static org.hl7.fhir.dstu3.model.ContactDetail convertTestScriptContactComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptContactComponent src) throws FHIRException { 814 if (src == null || src.isEmpty()) 815 return null; 816 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 817 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 818 if (src.hasName()) 819 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 820 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 821 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 822 return tgt; 823 } 824 825 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 826 if (src == null || src.isEmpty()) 827 return null; 828 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptDestinationComponent(); 829 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 830 if (src.hasIndexElement()) 831 tgt.setIndexElement(Integer14_30.convertInteger(src.getIndexElement())); 832 if (src.hasProfile()) 833 tgt.setProfile(Code14_30.convertCoding(src.getProfile())); 834 return tgt; 835 } 836 837 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent convertTestScriptDestinationComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptDestinationComponent src) throws FHIRException { 838 if (src == null || src.isEmpty()) 839 return null; 840 org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptDestinationComponent(); 841 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 842 if (src.hasIndexElement()) 843 tgt.setIndexElement(Integer14_30.convertInteger(src.getIndexElement())); 844 if (src.hasProfile()) 845 tgt.setProfile(Code14_30.convertCoding(src.getProfile())); 846 return tgt; 847 } 848 849 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 850 if (src == null || src.isEmpty()) 851 return null; 852 org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent(); 853 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 854 if (src.hasAutocreate()) 855 tgt.setAutocreateElement(Boolean14_30.convertBoolean(src.getAutocreateElement())); 856 if (src.hasAutodelete()) 857 tgt.setAutodeleteElement(Boolean14_30.convertBoolean(src.getAutodeleteElement())); 858 if (src.hasResource()) 859 tgt.setResource(Reference14_30.convertReference(src.getResource())); 860 return tgt; 861 } 862 863 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptFixtureComponent convertTestScriptFixtureComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptFixtureComponent src) throws FHIRException { 864 if (src == null || src.isEmpty()) 865 return null; 866 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptFixtureComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptFixtureComponent(); 867 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 868 if (src.hasAutocreate()) 869 tgt.setAutocreateElement(Boolean14_30.convertBoolean(src.getAutocreateElement())); 870 if (src.hasAutodelete()) 871 tgt.setAutodeleteElement(Boolean14_30.convertBoolean(src.getAutodeleteElement())); 872 if (src.hasResource()) 873 tgt.setResource(Reference14_30.convertReference(src.getResource())); 874 return tgt; 875 } 876 877 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 878 if (src == null || src.isEmpty()) 879 return null; 880 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent(); 881 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 882 if (src.hasRequired()) 883 tgt.setRequiredElement(Boolean14_30.convertBoolean(src.getRequiredElement())); 884 if (src.hasValidated()) 885 tgt.setValidatedElement(Boolean14_30.convertBoolean(src.getValidatedElement())); 886 if (src.hasDescription()) 887 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 888 for (org.hl7.fhir.dstu2016may.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 889 if (src.hasDestination()) 890 tgt.setDestinationElement(Integer14_30.convertInteger(src.getDestinationElement())); 891 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 892 if (src.hasConformance()) 893 tgt.setCapabilities(Reference14_30.convertReference(src.getConformance())); 894 return tgt; 895 } 896 897 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataCapabilityComponent convertTestScriptMetadataCapabilityComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent src) throws FHIRException { 898 if (src == null || src.isEmpty()) 899 return null; 900 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataCapabilityComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataCapabilityComponent(); 901 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 902 if (src.hasRequired()) 903 tgt.setRequiredElement(Boolean14_30.convertBoolean(src.getRequiredElement())); 904 if (src.hasValidated()) 905 tgt.setValidatedElement(Boolean14_30.convertBoolean(src.getValidatedElement())); 906 if (src.hasDescription()) 907 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 908 for (org.hl7.fhir.dstu3.model.IntegerType t : src.getOrigin()) tgt.addOrigin(t.getValue()); 909 if (src.hasDestination()) 910 tgt.setDestinationElement(Integer14_30.convertInteger(src.getDestinationElement())); 911 for (org.hl7.fhir.dstu3.model.UriType t : src.getLink()) tgt.addLink(t.getValue()); 912 if (src.hasCapabilities()) 913 tgt.setConformance(Reference14_30.convertReference(src.getCapabilities())); 914 return tgt; 915 } 916 917 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 918 if (src == null || src.isEmpty()) 919 return null; 920 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent(); 921 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 922 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 923 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 924 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 925 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 926 return tgt; 927 } 928 929 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataComponent convertTestScriptMetadataComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataComponent src) throws FHIRException { 930 if (src == null || src.isEmpty()) 931 return null; 932 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataComponent(); 933 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 934 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent t : src.getLink()) 935 tgt.addLink(convertTestScriptMetadataLinkComponent(t)); 936 for (org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataCapabilityComponent t : src.getCapability()) 937 tgt.addCapability(convertTestScriptMetadataCapabilityComponent(t)); 938 return tgt; 939 } 940 941 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 942 if (src == null || src.isEmpty()) 943 return null; 944 org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent(); 945 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 946 if (src.hasUrlElement()) 947 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 948 if (src.hasDescription()) 949 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 950 return tgt; 951 } 952 953 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataLinkComponent convertTestScriptMetadataLinkComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptMetadataLinkComponent src) throws FHIRException { 954 if (src == null || src.isEmpty()) 955 return null; 956 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataLinkComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptMetadataLinkComponent(); 957 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 958 if (src.hasUrlElement()) 959 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 960 if (src.hasDescription()) 961 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 962 return tgt; 963 } 964 965 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 966 if (src == null || src.isEmpty()) 967 return null; 968 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptOriginComponent(); 969 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 970 if (src.hasIndexElement()) 971 tgt.setIndexElement(Integer14_30.convertInteger(src.getIndexElement())); 972 if (src.hasProfile()) 973 tgt.setProfile(Code14_30.convertCoding(src.getProfile())); 974 return tgt; 975 } 976 977 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent convertTestScriptOriginComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptOriginComponent src) throws FHIRException { 978 if (src == null || src.isEmpty()) 979 return null; 980 org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptOriginComponent(); 981 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 982 if (src.hasIndexElement()) 983 tgt.setIndexElement(Integer14_30.convertInteger(src.getIndexElement())); 984 if (src.hasProfile()) 985 tgt.setProfile(Code14_30.convertCoding(src.getProfile())); 986 return tgt; 987 } 988 989 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptRuleComponent convertTestScriptRuleComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleComponent src) throws FHIRException { 990 if (src == null || src.isEmpty()) 991 return null; 992 org.hl7.fhir.dstu3.model.TestScript.TestScriptRuleComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptRuleComponent(); 993 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 994 if (src.hasResource()) 995 tgt.setResource(Reference14_30.convertReference(src.getResource())); 996 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleParamComponent t : src.getParam()) 997 tgt.addParam(convertTestScriptRuleParamComponent(t)); 998 return tgt; 999 } 1000 1001 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleComponent convertTestScriptRuleComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptRuleComponent src) throws FHIRException { 1002 if (src == null || src.isEmpty()) 1003 return null; 1004 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleComponent(); 1005 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1006 if (src.hasResource()) 1007 tgt.setResource(Reference14_30.convertReference(src.getResource())); 1008 for (org.hl7.fhir.dstu3.model.TestScript.RuleParamComponent t : src.getParam()) 1009 tgt.addParam(convertTestScriptRuleParamComponent(t)); 1010 return tgt; 1011 } 1012 1013 public static org.hl7.fhir.dstu3.model.TestScript.RuleParamComponent convertTestScriptRuleParamComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleParamComponent src) throws FHIRException { 1014 if (src == null || src.isEmpty()) 1015 return null; 1016 org.hl7.fhir.dstu3.model.TestScript.RuleParamComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.RuleParamComponent(); 1017 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1018 if (src.hasNameElement()) 1019 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1020 if (src.hasValue()) 1021 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 1022 return tgt; 1023 } 1024 1025 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleParamComponent convertTestScriptRuleParamComponent(org.hl7.fhir.dstu3.model.TestScript.RuleParamComponent src) throws FHIRException { 1026 if (src == null || src.isEmpty()) 1027 return null; 1028 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleParamComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRuleParamComponent(); 1029 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1030 if (src.hasNameElement()) 1031 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1032 if (src.hasValue()) 1033 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 1034 return tgt; 1035 } 1036 1037 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptRulesetComponent convertTestScriptRulesetComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetComponent src) throws FHIRException { 1038 if (src == null || src.isEmpty()) 1039 return null; 1040 org.hl7.fhir.dstu3.model.TestScript.TestScriptRulesetComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptRulesetComponent(); 1041 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1042 if (src.hasResource()) 1043 tgt.setResource(Reference14_30.convertReference(src.getResource())); 1044 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleComponent t : src.getRule()) 1045 tgt.addRule(convertTestScriptRulesetRuleComponent(t)); 1046 return tgt; 1047 } 1048 1049 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetComponent convertTestScriptRulesetComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptRulesetComponent src) throws FHIRException { 1050 if (src == null || src.isEmpty()) 1051 return null; 1052 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetComponent(); 1053 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1054 if (src.hasResource()) 1055 tgt.setResource(Reference14_30.convertReference(src.getResource())); 1056 for (org.hl7.fhir.dstu3.model.TestScript.RulesetRuleComponent t : src.getRule()) 1057 tgt.addRule(convertTestScriptRulesetRuleComponent(t)); 1058 return tgt; 1059 } 1060 1061 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleComponent convertTestScriptRulesetRuleComponent(org.hl7.fhir.dstu3.model.TestScript.RulesetRuleComponent src) throws FHIRException { 1062 if (src == null || src.isEmpty()) 1063 return null; 1064 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleComponent(); 1065 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1066 for (org.hl7.fhir.dstu3.model.TestScript.RulesetRuleParamComponent t : src.getParam()) 1067 tgt.addParam(convertTestScriptRulesetRuleParamComponent(t)); 1068 return tgt; 1069 } 1070 1071 public static org.hl7.fhir.dstu3.model.TestScript.RulesetRuleComponent convertTestScriptRulesetRuleComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleComponent src) throws FHIRException { 1072 if (src == null || src.isEmpty()) 1073 return null; 1074 org.hl7.fhir.dstu3.model.TestScript.RulesetRuleComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.RulesetRuleComponent(); 1075 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1076 for (org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleParamComponent t : src.getParam()) 1077 tgt.addParam(convertTestScriptRulesetRuleParamComponent(t)); 1078 return tgt; 1079 } 1080 1081 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleParamComponent convertTestScriptRulesetRuleParamComponent(org.hl7.fhir.dstu3.model.TestScript.RulesetRuleParamComponent src) throws FHIRException { 1082 if (src == null || src.isEmpty()) 1083 return null; 1084 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleParamComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleParamComponent(); 1085 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1086 if (src.hasNameElement()) 1087 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1088 if (src.hasValue()) 1089 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 1090 return tgt; 1091 } 1092 1093 public static org.hl7.fhir.dstu3.model.TestScript.RulesetRuleParamComponent convertTestScriptRulesetRuleParamComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptRulesetRuleParamComponent src) throws FHIRException { 1094 if (src == null || src.isEmpty()) 1095 return null; 1096 org.hl7.fhir.dstu3.model.TestScript.RulesetRuleParamComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.RulesetRuleParamComponent(); 1097 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1098 if (src.hasNameElement()) 1099 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1100 if (src.hasValue()) 1101 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 1102 return tgt; 1103 } 1104 1105 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 1106 if (src == null || src.isEmpty()) 1107 return null; 1108 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptSetupComponent(); 1109 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1110 for (org.hl7.fhir.dstu3.model.TestScript.SetupActionComponent t : src.getAction()) 1111 tgt.addAction(convertSetupActionComponent(t)); 1112 return tgt; 1113 } 1114 1115 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent convertTestScriptSetupComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptSetupComponent src) throws FHIRException { 1116 if (src == null || src.isEmpty()) 1117 return null; 1118 org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptSetupComponent(); 1119 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1120 for (org.hl7.fhir.dstu2016may.model.TestScript.SetupActionComponent t : src.getAction()) 1121 tgt.addAction(convertSetupActionComponent(t)); 1122 return tgt; 1123 } 1124 1125 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 1126 if (src == null || src.isEmpty()) 1127 return null; 1128 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTeardownComponent(); 1129 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1130 for (org.hl7.fhir.dstu3.model.TestScript.TeardownActionComponent t : src.getAction()) 1131 tgt.addAction(convertTeardownActionComponent(t)); 1132 return tgt; 1133 } 1134 1135 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent convertTestScriptTeardownComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTeardownComponent src) throws FHIRException { 1136 if (src == null || src.isEmpty()) 1137 return null; 1138 org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTeardownComponent(); 1139 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1140 for (org.hl7.fhir.dstu2016may.model.TestScript.TeardownActionComponent t : src.getAction()) 1141 tgt.addAction(convertTeardownActionComponent(t)); 1142 return tgt; 1143 } 1144 1145 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent src) throws FHIRException { 1146 if (src == null || src.isEmpty()) 1147 return null; 1148 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTestComponent(); 1149 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1150 if (src.hasName()) 1151 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1152 if (src.hasDescription()) 1153 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 1154 for (org.hl7.fhir.dstu3.model.TestScript.TestActionComponent t : src.getAction()) 1155 tgt.addAction(convertTestActionComponent(t)); 1156 return tgt; 1157 } 1158 1159 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent convertTestScriptTestComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptTestComponent src) throws FHIRException { 1160 if (src == null || src.isEmpty()) 1161 return null; 1162 org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptTestComponent(); 1163 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1164 if (src.hasName()) 1165 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1166 if (src.hasDescription()) 1167 tgt.setDescriptionElement(String14_30.convertString(src.getDescriptionElement())); 1168 for (org.hl7.fhir.dstu2016may.model.TestScript.TestActionComponent t : src.getAction()) 1169 tgt.addAction(convertTestActionComponent(t)); 1170 return tgt; 1171 } 1172 1173 public static org.hl7.fhir.dstu2016may.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 1174 if (src == null || src.isEmpty()) 1175 return null; 1176 org.hl7.fhir.dstu2016may.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu2016may.model.TestScript.TestScriptVariableComponent(); 1177 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1178 if (src.hasNameElement()) 1179 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1180 if (src.hasDefaultValue()) 1181 tgt.setDefaultValueElement(String14_30.convertString(src.getDefaultValueElement())); 1182 if (src.hasHeaderField()) 1183 tgt.setHeaderFieldElement(String14_30.convertString(src.getHeaderFieldElement())); 1184 if (src.hasPath()) 1185 tgt.setPathElement(String14_30.convertString(src.getPathElement())); 1186 if (src.hasSourceId()) 1187 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 1188 return tgt; 1189 } 1190 1191 public static org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent convertTestScriptVariableComponent(org.hl7.fhir.dstu2016may.model.TestScript.TestScriptVariableComponent src) throws FHIRException { 1192 if (src == null || src.isEmpty()) 1193 return null; 1194 org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent tgt = new org.hl7.fhir.dstu3.model.TestScript.TestScriptVariableComponent(); 1195 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 1196 if (src.hasNameElement()) 1197 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 1198 if (src.hasDefaultValue()) 1199 tgt.setDefaultValueElement(String14_30.convertString(src.getDefaultValueElement())); 1200 if (src.hasHeaderField()) 1201 tgt.setHeaderFieldElement(String14_30.convertString(src.getHeaderFieldElement())); 1202 if (src.hasPath()) 1203 tgt.setPathElement(String14_30.convertString(src.getPathElement())); 1204 if (src.hasSourceId()) 1205 tgt.setSourceIdElement(Id14_30.convertId(src.getSourceIdElement())); 1206 return tgt; 1207 } 1208}