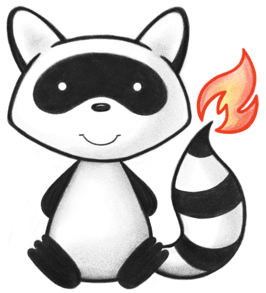
001package org.hl7.fhir.convertors.conv14_30.resources14_30; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_30; 004import org.hl7.fhir.convertors.conv14_30.VersionConvertor_14_30; 005import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.CodeableConcept14_30; 006import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.ContactPoint14_30; 007import org.hl7.fhir.convertors.conv14_30.datatypes14_30.complextypes14_30.Identifier14_30; 008import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Boolean14_30; 009import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Code14_30; 010import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.DateTime14_30; 011import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Integer14_30; 012import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.String14_30; 013import org.hl7.fhir.convertors.conv14_30.datatypes14_30.primitivetypes14_30.Uri14_30; 014import org.hl7.fhir.exceptions.FHIRException; 015 016public class ValueSet14_30 { 017 018 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 019 if (src == null || src.isEmpty()) 020 return null; 021 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent(); 022 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 023 if (src.hasCodeElement()) 024 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 025 if (src.hasDisplay()) 026 tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 027 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 028 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent convertConceptReferenceComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent src) throws FHIRException { 033 if (src == null || src.isEmpty()) 034 return null; 035 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent(); 036 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 037 if (src.hasCodeElement()) 038 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 039 if (src.hasDisplay()) 040 tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 041 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent t : src.getDesignation()) 042 tgt.addDesignation(convertConceptReferenceDesignationComponent(t)); 043 return tgt; 044 } 045 046 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 047 if (src == null || src.isEmpty()) 048 return null; 049 org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent(); 050 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 051 if (src.hasLanguage()) 052 tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 053 if (src.hasUse()) 054 tgt.setUse(Code14_30.convertCoding(src.getUse())); 055 if (src.hasValueElement()) 056 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 057 return tgt; 058 } 059 060 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent convertConceptReferenceDesignationComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceDesignationComponent src) throws FHIRException { 061 if (src == null || src.isEmpty()) 062 return null; 063 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceDesignationComponent(); 064 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 065 if (src.hasLanguage()) 066 tgt.setLanguageElement(Code14_30.convertCode(src.getLanguageElement())); 067 if (src.hasUse()) 068 tgt.setUse(Code14_30.convertCoding(src.getUse())); 069 if (src.hasValueElement()) 070 tgt.setValueElement(String14_30.convertString(src.getValueElement())); 071 return tgt; 072 } 073 074 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent src) throws FHIRException { 075 if (src == null || src.isEmpty()) 076 return null; 077 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent(); 078 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 079 if (src.hasSystemElement()) 080 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 081 if (src.hasVersion()) 082 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 083 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 084 tgt.addConcept(convertConceptReferenceComponent(t)); 085 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 086 tgt.addFilter(convertConceptSetFilterComponent(t)); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent convertConceptSetComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent src) throws FHIRException { 091 if (src == null || src.isEmpty()) 092 return null; 093 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent(); 094 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 095 if (src.hasSystemElement()) 096 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 097 if (src.hasVersion()) 098 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 099 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent t : src.getConcept()) 100 tgt.addConcept(convertConceptReferenceComponent(t)); 101 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent t : src.getFilter()) 102 tgt.addFilter(convertConceptSetFilterComponent(t)); 103 return tgt; 104 } 105 106 public static org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 107 if (src == null || src.isEmpty()) 108 return null; 109 org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent(); 110 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 111 if (src.hasPropertyElement()) 112 tgt.setPropertyElement(Code14_30.convertCode(src.getPropertyElement())); 113 if (src.hasOp()) 114 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 115 if (src.hasValueElement()) 116 tgt.setValueElement(Code14_30.convertCode(src.getValueElement())); 117 return tgt; 118 } 119 120 public static org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent convertConceptSetFilterComponent(org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent src) throws FHIRException { 121 if (src == null || src.isEmpty()) 122 return null; 123 org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetFilterComponent(); 124 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 125 if (src.hasPropertyElement()) 126 tgt.setPropertyElement(Code14_30.convertCode(src.getPropertyElement())); 127 if (src.hasOp()) 128 tgt.setOpElement(convertFilterOperator(src.getOpElement())); 129 if (src.hasValueElement()) 130 tgt.setValueElement(Code14_30.convertCode(src.getValueElement())); 131 return tgt; 132 } 133 134 static public org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> src) throws FHIRException { 135 if (src == null || src.isEmpty()) 136 return null; 137 org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu3.model.Enumeration<>(new org.hl7.fhir.dstu3.model.ValueSet.FilterOperatorEnumFactory()); 138 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 139 switch (src.getValue()) { 140 case EQUAL: 141 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.EQUAL); 142 break; 143 case ISA: 144 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISA); 145 break; 146 case ISNOTA: 147 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.ISNOTA); 148 break; 149 case REGEX: 150 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.REGEX); 151 break; 152 case IN: 153 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.IN); 154 break; 155 case NOTIN: 156 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NOTIN); 157 break; 158 default: 159 tgt.setValue(org.hl7.fhir.dstu3.model.ValueSet.FilterOperator.NULL); 160 break; 161 } 162 return tgt; 163 } 164 165 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> convertFilterOperator(org.hl7.fhir.dstu3.model.Enumeration<org.hl7.fhir.dstu3.model.ValueSet.FilterOperator> src) throws FHIRException { 166 if (src == null || src.isEmpty()) 167 return null; 168 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperatorEnumFactory()); 169 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 170 switch (src.getValue()) { 171 case EQUAL: 172 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.EQUAL); 173 break; 174 case ISA: 175 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.ISA); 176 break; 177 case ISNOTA: 178 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.ISNOTA); 179 break; 180 case REGEX: 181 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.REGEX); 182 break; 183 case IN: 184 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.IN); 185 break; 186 case NOTIN: 187 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.NOTIN); 188 break; 189 default: 190 tgt.setValue(org.hl7.fhir.dstu2016may.model.ValueSet.FilterOperator.NULL); 191 break; 192 } 193 return tgt; 194 } 195 196 public static org.hl7.fhir.dstu2016may.model.ValueSet convertValueSet(org.hl7.fhir.dstu3.model.ValueSet src) throws FHIRException { 197 if (src == null || src.isEmpty()) 198 return null; 199 org.hl7.fhir.dstu2016may.model.ValueSet tgt = new org.hl7.fhir.dstu2016may.model.ValueSet(); 200 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 201 if (src.hasUrl()) 202 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 203 for (org.hl7.fhir.dstu3.model.Identifier t : src.getIdentifier()) 204 tgt.setIdentifier(Identifier14_30.convertIdentifier(t)); 205 if (src.hasVersion()) 206 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 207 if (src.hasName()) 208 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 209 if (src.hasStatus()) 210 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 211 if (src.hasExperimental()) 212 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 213 if (src.hasPublisher()) 214 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 215 for (org.hl7.fhir.dstu3.model.ContactDetail t : src.getContact()) 216 tgt.addContact(convertValueSetContactComponent(t)); 217 if (src.hasDate()) 218 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 219 if (src.getCompose().hasLockedDate()) 220 tgt.setLockedDate(src.getCompose().getLockedDate()); 221 if (src.hasDescription()) 222 tgt.setDescription(src.getDescription()); 223 for (org.hl7.fhir.dstu3.model.UsageContext t : src.getUseContext()) 224 if (t.hasValueCodeableConcept()) 225 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t.getValueCodeableConcept())); 226 for (org.hl7.fhir.dstu3.model.CodeableConcept t : src.getJurisdiction()) 227 tgt.addUseContext(CodeableConcept14_30.convertCodeableConcept(t)); 228 if (src.hasImmutable()) 229 tgt.setImmutableElement(Boolean14_30.convertBoolean(src.getImmutableElement())); 230 if (src.hasPurpose()) 231 tgt.setRequirements(src.getPurpose()); 232 if (src.hasCopyright()) 233 tgt.setCopyright(src.getCopyright()); 234 if (src.hasExtensible()) 235 tgt.setExtensibleElement(Boolean14_30.convertBoolean(src.getExtensibleElement())); 236 if (src.hasCompose()) 237 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 238 if (src.hasExpansion()) 239 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 240 return tgt; 241 } 242 243 public static org.hl7.fhir.dstu3.model.ValueSet convertValueSet(org.hl7.fhir.dstu2016may.model.ValueSet src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 org.hl7.fhir.dstu3.model.ValueSet tgt = new org.hl7.fhir.dstu3.model.ValueSet(); 247 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyDomainResource(src, tgt); 248 if (src.hasUrl()) 249 tgt.setUrlElement(Uri14_30.convertUri(src.getUrlElement())); 250 if (src.hasIdentifier()) 251 tgt.addIdentifier(Identifier14_30.convertIdentifier(src.getIdentifier())); 252 if (src.hasVersion()) 253 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 254 if (src.hasName()) 255 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 256 if (src.hasStatus()) 257 tgt.setStatusElement(Enumerations14_30.convertConformanceResourceStatus(src.getStatusElement())); 258 if (src.hasExperimental()) 259 tgt.setExperimentalElement(Boolean14_30.convertBoolean(src.getExperimentalElement())); 260 if (src.hasPublisher()) 261 tgt.setPublisherElement(String14_30.convertString(src.getPublisherElement())); 262 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent t : src.getContact()) 263 tgt.addContact(convertValueSetContactComponent(t)); 264 if (src.hasDate()) 265 tgt.setDateElement(DateTime14_30.convertDateTime(src.getDateElement())); 266 if (src.hasDescription()) 267 tgt.setDescription(src.getDescription()); 268 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 269 if (VersionConvertor_14_30.isJurisdiction(t)) 270 tgt.addJurisdiction(CodeableConcept14_30.convertCodeableConcept(t)); 271 else 272 tgt.addUseContext(CodeableConcept14_30.convertCodeableConceptToUsageContext(t)); 273 if (src.hasImmutable()) 274 tgt.setImmutableElement(Boolean14_30.convertBoolean(src.getImmutableElement())); 275 if (src.hasRequirements()) 276 tgt.setPurpose(src.getRequirements()); 277 if (src.hasCopyright()) 278 tgt.setCopyright(src.getCopyright()); 279 if (src.hasExtensible()) 280 tgt.setExtensibleElement(Boolean14_30.convertBoolean(src.getExtensibleElement())); 281 if (src.hasCompose()) 282 tgt.setCompose(convertValueSetComposeComponent(src.getCompose())); 283 if (src.hasLockedDate()) 284 tgt.getCompose().setLockedDate(src.getLockedDate()); 285 if (src.hasExpansion()) 286 tgt.setExpansion(convertValueSetExpansionComponent(src.getExpansion())); 287 return tgt; 288 } 289 290 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 291 if (src == null || src.isEmpty()) 292 return null; 293 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent(); 294 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 295 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getInclude()) { 296 for (org.hl7.fhir.dstu3.model.UriType ti : t.getValueSet()) tgt.addImport(ti.getValue()); 297 tgt.addInclude(convertConceptSetComponent(t)); 298 } 299 for (org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent t : src.getExclude()) 300 tgt.addExclude(convertConceptSetComponent(t)); 301 return tgt; 302 } 303 304 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent convertValueSetComposeComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetComposeComponent src) throws FHIRException { 305 if (src == null || src.isEmpty()) 306 return null; 307 org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetComposeComponent(); 308 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 309 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getImport()) tgt.addInclude().addValueSet(t.getValue()); 310 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent t : src.getInclude()) 311 tgt.addInclude(convertConceptSetComponent(t)); 312 for (org.hl7.fhir.dstu2016may.model.ValueSet.ConceptSetComponent t : src.getExclude()) 313 tgt.addExclude(convertConceptSetComponent(t)); 314 return tgt; 315 } 316 317 public static org.hl7.fhir.dstu3.model.ContactDetail convertValueSetContactComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent src) throws FHIRException { 318 if (src == null || src.isEmpty()) 319 return null; 320 org.hl7.fhir.dstu3.model.ContactDetail tgt = new org.hl7.fhir.dstu3.model.ContactDetail(); 321 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 322 if (src.hasName()) 323 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 324 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 325 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 326 return tgt; 327 } 328 329 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent convertValueSetContactComponent(org.hl7.fhir.dstu3.model.ContactDetail src) throws FHIRException { 330 if (src == null || src.isEmpty()) 331 return null; 332 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetContactComponent(); 333 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyElement(src, tgt); 334 if (src.hasName()) 335 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 336 for (org.hl7.fhir.dstu3.model.ContactPoint t : src.getTelecom()) 337 tgt.addTelecom(ContactPoint14_30.convertContactPoint(t)); 338 return tgt; 339 } 340 341 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 342 if (src == null || src.isEmpty()) 343 return null; 344 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent(); 345 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 346 if (src.hasIdentifierElement()) 347 tgt.setIdentifierElement(Uri14_30.convertUri(src.getIdentifierElement())); 348 if (src.hasTimestampElement()) 349 tgt.setTimestampElement(DateTime14_30.convertDateTime(src.getTimestampElement())); 350 if (src.hasTotal()) 351 tgt.setTotalElement(Integer14_30.convertInteger(src.getTotalElement())); 352 if (src.hasOffset()) 353 tgt.setOffsetElement(Integer14_30.convertInteger(src.getOffsetElement())); 354 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 355 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 356 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 357 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 358 return tgt; 359 } 360 361 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent convertValueSetExpansionComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent src) throws FHIRException { 362 if (src == null || src.isEmpty()) 363 return null; 364 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionComponent(); 365 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 366 if (src.hasIdentifierElement()) 367 tgt.setIdentifierElement(Uri14_30.convertUri(src.getIdentifierElement())); 368 if (src.hasTimestampElement()) 369 tgt.setTimestampElement(DateTime14_30.convertDateTime(src.getTimestampElement())); 370 if (src.hasTotal()) 371 tgt.setTotalElement(Integer14_30.convertInteger(src.getTotalElement())); 372 if (src.hasOffset()) 373 tgt.setOffsetElement(Integer14_30.convertInteger(src.getOffsetElement())); 374 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent t : src.getParameter()) 375 tgt.addParameter(convertValueSetExpansionParameterComponent(t)); 376 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 377 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 378 return tgt; 379 } 380 381 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 382 if (src == null || src.isEmpty()) 383 return null; 384 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent(); 385 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 386 if (src.hasSystem()) 387 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 388 if (src.hasAbstract()) 389 tgt.setAbstractElement(Boolean14_30.convertBoolean(src.getAbstractElement())); 390 if (src.hasVersion()) 391 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 392 if (src.hasCode()) 393 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 394 if (src.hasDisplay()) 395 tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 396 for (org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 397 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 398 return tgt; 399 } 400 401 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent convertValueSetExpansionContainsComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent src) throws FHIRException { 402 if (src == null || src.isEmpty()) 403 return null; 404 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionContainsComponent(); 405 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 406 if (src.hasSystem()) 407 tgt.setSystemElement(Uri14_30.convertUri(src.getSystemElement())); 408 if (src.hasAbstract()) 409 tgt.setAbstractElement(Boolean14_30.convertBoolean(src.getAbstractElement())); 410 if (src.hasVersion()) 411 tgt.setVersionElement(String14_30.convertString(src.getVersionElement())); 412 if (src.hasCode()) 413 tgt.setCodeElement(Code14_30.convertCode(src.getCodeElement())); 414 if (src.hasDisplay()) 415 tgt.setDisplayElement(String14_30.convertString(src.getDisplayElement())); 416 for (org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent t : src.getContains()) 417 tgt.addContains(convertValueSetExpansionContainsComponent(t)); 418 return tgt; 419 } 420 421 public static org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 422 if (src == null || src.isEmpty()) 423 return null; 424 org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent(); 425 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 426 if (src.hasNameElement()) 427 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 428 if (src.hasValue()) 429 tgt.setValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValue())); 430 return tgt; 431 } 432 433 public static org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent convertValueSetExpansionParameterComponent(org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionParameterComponent src) throws FHIRException { 434 if (src == null || src.isEmpty()) 435 return null; 436 org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent tgt = new org.hl7.fhir.dstu2016may.model.ValueSet.ValueSetExpansionParameterComponent(); 437 ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().copyBackboneElement(src,tgt); 438 if (src.hasNameElement()) 439 tgt.setNameElement(String14_30.convertString(src.getNameElement())); 440 if (src.hasValue()) 441 tgt.setValue(ConversionContext14_30.INSTANCE.getVersionConvertor_14_30().convertType(src.getValue())); 442 return tgt; 443 } 444}