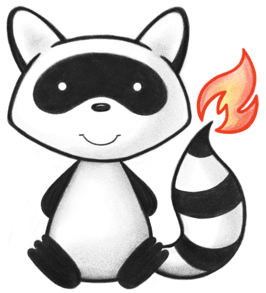
001package org.hl7.fhir.convertors.conv14_40; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import javax.annotation.Nonnull; 007 008import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_14_40; 009import org.hl7.fhir.convertors.context.ConversionContext14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.BackboneElement14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Element14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Type14_40; 013import org.hl7.fhir.convertors.conv14_40.resources14_40.Resource14_40; 014import org.hl7.fhir.dstu2016may.model.CodeableConcept; 015import org.hl7.fhir.exceptions.FHIRException; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 */ 044 045public class VersionConvertor_14_40 { 046 static public List<String> CANONICAL_URLS = new ArrayList<String>(); 047 048 static { 049 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/11179-permitted-value-conceptmap"); 050 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/11179-permitted-value-valueset"); 051 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/codesystem-map"); 052 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/cqif-library"); 053 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-allowedUnits"); 054 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-inheritedExtensibleValueSet"); 055 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-maxValueSet"); 056 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-minValueSet"); 057 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/event-instantiatesCanonical"); 058 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-allowedProfile"); 059 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-deMap"); 060 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-sourceStructureMap"); 061 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-targetStructureMap"); 062 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-unit-valueSet"); 063 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-map"); 064 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-supplement"); 065 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-system"); 066 } 067 068 private final BaseAdvisor_14_40 advisor; 069 private final Element14_40 elementConvertor; 070 071 private final BackboneElement14_40 backboneElementConvertor; 072 private final Resource14_40 resourceConvertor; 073 private final Type14_40 typeConvertor; 074 075 public VersionConvertor_14_40(@Nonnull BaseAdvisor_14_40 advisor) { 076 this.advisor = advisor; 077 this.elementConvertor = new Element14_40(advisor); 078 backboneElementConvertor = new BackboneElement14_40(); 079 this.resourceConvertor = new Resource14_40(advisor); 080 this.typeConvertor = new Type14_40(advisor); 081 } 082 083 static public boolean isJurisdiction(@Nonnull CodeableConcept t) { 084 return t.hasCoding() 085 && ("http://unstats.un.org/unsd/methods/m49/m49.htm".equals(t.getCoding().get(0).getSystem()) 086 || "urn:iso:std:iso:3166".equals(t.getCoding().get(0).getSystem()) 087 || "https://www.usps.com/".equals(t.getCoding().get(0).getSystem())); 088 } 089 090 public BaseAdvisor_14_40 advisor() { 091 return advisor; 092 } 093 094 public void copyResource(@Nonnull org.hl7.fhir.dstu2016may.model.Resource src, 095 @Nonnull org.hl7.fhir.r4.model.Resource tgt) throws FHIRException { 096 resourceConvertor.copyResource(src, tgt); 097 } 098 099 public void copyResource(@Nonnull org.hl7.fhir.r4.model.Resource src, 100 @Nonnull org.hl7.fhir.dstu2016may.model.Resource tgt) throws FHIRException { 101 resourceConvertor.copyResource(src, tgt); 102 } 103 104 public org.hl7.fhir.r4.model.Resource convertResource(@Nonnull org.hl7.fhir.dstu2016may.model.Resource src) throws FHIRException { 105 ConversionContext14_40.INSTANCE.init(this, src.fhirType()); 106 try { 107 return resourceConvertor.convertResource(src); 108 } finally { 109 ConversionContext14_40.INSTANCE.close(src.fhirType()); 110 } 111 } 112 113 public org.hl7.fhir.dstu2016may.model.Resource convertResource(@Nonnull org.hl7.fhir.r4.model.Resource src) throws FHIRException { 114 ConversionContext14_40.INSTANCE.init(this, src.fhirType()); 115 try { 116 return resourceConvertor.convertResource(src); 117 } finally { 118 ConversionContext14_40.INSTANCE.close(src.fhirType()); 119 } 120 } 121 122 public org.hl7.fhir.r4.model.Type convertType(@Nonnull org.hl7.fhir.dstu2016may.model.Type src) throws FHIRException { 123 ConversionContext14_40.INSTANCE.init(this, src.fhirType()); 124 try { 125 return typeConvertor.convertType(src); 126 } finally { 127 ConversionContext14_40.INSTANCE.close(src.fhirType()); 128 } 129 } 130 131 public org.hl7.fhir.dstu2016may.model.Type convertType(@Nonnull org.hl7.fhir.r4.model.Type src) throws FHIRException { 132 ConversionContext14_40.INSTANCE.init(this, src.fhirType()); 133 try { 134 return typeConvertor.convertType(src); 135 } finally { 136 ConversionContext14_40.INSTANCE.close(src.fhirType()); 137 } 138 } 139 140 public void copyDomainResource( 141 @Nonnull org.hl7.fhir.dstu2016may.model.DomainResource src, 142 @Nonnull org.hl7.fhir.r4.model.DomainResource tgt, 143 String... extensionUrlsToIgnore) throws FHIRException { 144 resourceConvertor.copyDomainResource(src, tgt, extensionUrlsToIgnore); 145 } 146 147 public void copyDomainResource( 148 @Nonnull org.hl7.fhir.r4.model.DomainResource src, 149 @Nonnull org.hl7.fhir.dstu2016may.model.DomainResource tgt, 150 String... extensionUrlsToIgnore) throws FHIRException { 151 resourceConvertor.copyDomainResource(src, tgt, extensionUrlsToIgnore); 152 } 153 154 public void copyElement( 155 @Nonnull org.hl7.fhir.dstu2016may.model.Element src, 156 @Nonnull org.hl7.fhir.r4.model.Element tgt, 157 String... extensionUrlsToIgnore) throws FHIRException { 158 elementConvertor.copyElement(src, tgt, ConversionContext14_40.INSTANCE.path(), extensionUrlsToIgnore); 159 } 160 161 public void copyElement( 162 @Nonnull org.hl7.fhir.r4.model.Element src, 163 @Nonnull org.hl7.fhir.dstu2016may.model.Element tgt, 164 String... extensionUrlsToIgnore) throws FHIRException { 165 elementConvertor.copyElement(src, tgt, ConversionContext14_40.INSTANCE.path(), extensionUrlsToIgnore); 166 } 167 168 public void copyBackboneElement( 169 @Nonnull org.hl7.fhir.r4.model.BackboneElement src, 170 @Nonnull org.hl7.fhir.dstu2016may.model.BackboneElement tgt, 171 String... extensionUrlsToIgnore) throws FHIRException { 172 backboneElementConvertor.copyBackboneElement(src, tgt, extensionUrlsToIgnore); 173 } 174 175 public void copyBackboneElement( 176 @Nonnull org.hl7.fhir.dstu2016may.model.BackboneElement src, 177 @Nonnull org.hl7.fhir.r4.model.BackboneElement tgt, 178 String... extensionUrlsToIgnore) throws FHIRException { 179 backboneElementConvertor.copyBackboneElement(src, tgt, extensionUrlsToIgnore); 180 } 181}