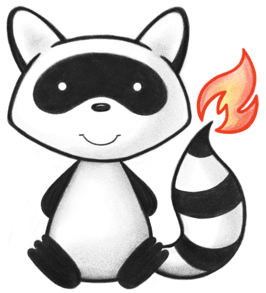
001package org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40; 002 003import java.util.Collections; 004 005import org.hl7.fhir.convertors.context.ConversionContext14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Decimal14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.UnsignedInt14_40; 008import org.hl7.fhir.exceptions.FHIRException; 009import org.hl7.fhir.r4.model.Timing; 010 011public class Timing14_40 { 012 public static org.hl7.fhir.r4.model.Timing convertTiming(org.hl7.fhir.dstu2016may.model.Timing src) throws FHIRException { 013 if (src == null || src.isEmpty()) return null; 014 org.hl7.fhir.r4.model.Timing tgt = new org.hl7.fhir.r4.model.Timing(); 015 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 016 for (org.hl7.fhir.dstu2016may.model.DateTimeType t : src.getEvent()) tgt.addEvent(t.getValue()); 017 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 018 if (src.hasCode()) tgt.setCode(CodeableConcept14_40.convertCodeableConcept(src.getCode())); 019 return tgt; 020 } 021 022 public static org.hl7.fhir.dstu2016may.model.Timing convertTiming(org.hl7.fhir.r4.model.Timing src) throws FHIRException { 023 if (src == null || src.isEmpty()) return null; 024 org.hl7.fhir.dstu2016may.model.Timing tgt = new org.hl7.fhir.dstu2016may.model.Timing(); 025 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 026 for (org.hl7.fhir.r4.model.DateTimeType t : src.getEvent()) tgt.addEvent(t.getValue()); 027 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 028 if (src.hasCode()) tgt.setCode(CodeableConcept14_40.convertCodeableConcept(src.getCode())); 029 return tgt; 030 } 031 032 public static org.hl7.fhir.r4.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.dstu2016may.model.Timing.TimingRepeatComponent src) throws FHIRException { 033 if (src == null || src.isEmpty()) return null; 034 org.hl7.fhir.r4.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.r4.model.Timing.TimingRepeatComponent(); 035 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 036 if (src.hasBounds()) 037 tgt.setBounds(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getBounds())); 038 if (src.hasCount()) tgt.setCount(src.getCount()); 039 if (src.hasCountMax()) tgt.setCountMax(src.getCountMax()); 040 if (src.hasDuration()) tgt.setDurationElement(Decimal14_40.convertDecimal(src.getDurationElement())); 041 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal14_40.convertDecimal(src.getDurationMaxElement())); 042 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 043 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 044 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 045 if (src.hasPeriod()) tgt.setPeriodElement(Decimal14_40.convertDecimal(src.getPeriodElement())); 046 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal14_40.convertDecimal(src.getPeriodMaxElement())); 047 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 048 if (src.hasWhen()) tgt.setWhen(Collections.singletonList(convertEventTiming(src.getWhenElement()))); 049 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt14_40.convertUnsignedInt(src.getOffsetElement())); 050 return tgt; 051 } 052 053 public static org.hl7.fhir.dstu2016may.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.r4.model.Timing.TimingRepeatComponent src) throws FHIRException { 054 if (src == null || src.isEmpty()) return null; 055 org.hl7.fhir.dstu2016may.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.dstu2016may.model.Timing.TimingRepeatComponent(); 056 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 057 if (src.hasBounds()) 058 tgt.setBounds(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getBounds())); 059 if (src.hasCount()) tgt.setCount(src.getCount()); 060 if (src.hasCountMax()) tgt.setCountMax(src.getCountMax()); 061 if (src.hasDuration()) tgt.setDurationElement(Decimal14_40.convertDecimal(src.getDurationElement())); 062 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal14_40.convertDecimal(src.getDurationMaxElement())); 063 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 064 if (src.hasFrequency()) tgt.setFrequency(src.getFrequency()); 065 if (src.hasFrequencyMax()) tgt.setFrequencyMax(src.getFrequencyMax()); 066 if (src.hasPeriod()) tgt.setPeriodElement(Decimal14_40.convertDecimal(src.getPeriodElement())); 067 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal14_40.convertDecimal(src.getPeriodMaxElement())); 068 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 069 if (src.hasWhen()) tgt.setWhenElement(convertEventTiming(src.getWhen().get(0))); 070 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt14_40.convertUnsignedInt(src.getOffsetElement())); 071 return tgt; 072 } 073 074 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime> src) throws FHIRException { 075 if (src == null || src.isEmpty()) return null; 076 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.UnitsOfTimeEnumFactory()); 077 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 078 if (src.getValue() == null) { 079 tgt.setValue(null); 080} else { 081 switch(src.getValue()) { 082 case S: 083 tgt.setValue(Timing.UnitsOfTime.S); 084 break; 085 case MIN: 086 tgt.setValue(Timing.UnitsOfTime.MIN); 087 break; 088 case H: 089 tgt.setValue(Timing.UnitsOfTime.H); 090 break; 091 case D: 092 tgt.setValue(Timing.UnitsOfTime.D); 093 break; 094 case WK: 095 tgt.setValue(Timing.UnitsOfTime.WK); 096 break; 097 case MO: 098 tgt.setValue(Timing.UnitsOfTime.MO); 099 break; 100 case A: 101 tgt.setValue(Timing.UnitsOfTime.A); 102 break; 103 default: 104 tgt.setValue(Timing.UnitsOfTime.NULL); 105 break; 106 } 107} 108 return tgt; 109 } 110 111 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.UnitsOfTime> src) throws FHIRException { 112 if (src == null || src.isEmpty()) return null; 113 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTimeEnumFactory()); 114 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 115 if (src.getValue() == null) { 116 tgt.setValue(null); 117} else { 118 switch(src.getValue()) { 119 case S: 120 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.S); 121 break; 122 case MIN: 123 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.MIN); 124 break; 125 case H: 126 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.H); 127 break; 128 case D: 129 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.D); 130 break; 131 case WK: 132 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.WK); 133 break; 134 case MO: 135 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.MO); 136 break; 137 case A: 138 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.A); 139 break; 140 default: 141 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.UnitsOfTime.NULL); 142 break; 143 } 144} 145 return tgt; 146 } 147 148 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.EventTiming> src) throws FHIRException { 149 if (src == null || src.isEmpty()) return null; 150 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Timing.EventTimingEnumFactory()); 151 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 152 if (src.getValue() == null) { 153 tgt.setValue(null); 154} else { 155 switch(src.getValue()) { 156 case HS: 157 tgt.setValue(Timing.EventTiming.HS); 158 break; 159 case WAKE: 160 tgt.setValue(Timing.EventTiming.WAKE); 161 break; 162 case C: 163 tgt.setValue(Timing.EventTiming.C); 164 break; 165 case CM: 166 tgt.setValue(Timing.EventTiming.CM); 167 break; 168 case CD: 169 tgt.setValue(Timing.EventTiming.CD); 170 break; 171 case CV: 172 tgt.setValue(Timing.EventTiming.CV); 173 break; 174 case AC: 175 tgt.setValue(Timing.EventTiming.AC); 176 break; 177 case ACM: 178 tgt.setValue(Timing.EventTiming.ACM); 179 break; 180 case ACD: 181 tgt.setValue(Timing.EventTiming.ACD); 182 break; 183 case ACV: 184 tgt.setValue(Timing.EventTiming.ACV); 185 break; 186 case PC: 187 tgt.setValue(Timing.EventTiming.PC); 188 break; 189 case PCM: 190 tgt.setValue(Timing.EventTiming.PCM); 191 break; 192 case PCD: 193 tgt.setValue(Timing.EventTiming.PCD); 194 break; 195 case PCV: 196 tgt.setValue(Timing.EventTiming.PCV); 197 break; 198 default: 199 tgt.setValue(Timing.EventTiming.NULL); 200 break; 201 } 202} 203 return tgt; 204 } 205 206 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Timing.EventTiming> src) throws FHIRException { 207 if (src == null || src.isEmpty()) return null; 208 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Timing.EventTiming> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Timing.EventTimingEnumFactory()); 209 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 210 if (src.getValue() == null) { 211 tgt.setValue(null); 212} else { 213 switch(src.getValue()) { 214 case HS: 215 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.HS); 216 break; 217 case WAKE: 218 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.WAKE); 219 break; 220 case C: 221 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.C); 222 break; 223 case CM: 224 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.CM); 225 break; 226 case CD: 227 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.CD); 228 break; 229 case CV: 230 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.CV); 231 break; 232 case AC: 233 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.AC); 234 break; 235 case ACM: 236 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.ACM); 237 break; 238 case ACD: 239 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.ACD); 240 break; 241 case ACV: 242 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.ACV); 243 break; 244 case PC: 245 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.PC); 246 break; 247 case PCM: 248 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.PCM); 249 break; 250 case PCD: 251 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.PCD); 252 break; 253 case PCV: 254 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.PCV); 255 break; 256 default: 257 tgt.setValue(org.hl7.fhir.dstu2016may.model.Timing.EventTiming.NULL); 258 break; 259 } 260} 261 return tgt; 262 } 263}