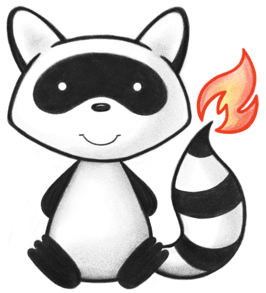
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.SourceElementComponentWrapper; 007import org.hl7.fhir.convertors.context.ConversionContext14_40; 008import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Reference14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Identifier14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Code14_40; 015import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 016import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 017import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.CanonicalType; 020import org.hl7.fhir.r4.model.ConceptMap; 021import org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent; 022import org.hl7.fhir.r4.model.Enumeration; 023import org.hl7.fhir.r4.model.Enumerations; 024 025public class ConceptMap14_40 { 026 027 public static org.hl7.fhir.r4.model.ConceptMap convertConceptMap(org.hl7.fhir.dstu2016may.model.ConceptMap src) throws FHIRException { 028 if (src == null || src.isEmpty()) 029 return null; 030 org.hl7.fhir.r4.model.ConceptMap tgt = new org.hl7.fhir.r4.model.ConceptMap(); 031 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 032 if (src.hasUrl()) 033 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 034 if (src.hasIdentifier()) 035 tgt.setIdentifier(Identifier14_40.convertIdentifier(src.getIdentifier())); 036 if (src.hasVersion()) 037 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 038 if (src.hasName()) 039 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 040 if (src.hasStatus()) 041 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 042 if (src.hasExperimental()) 043 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 044 if (src.hasPublisher()) 045 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 046 for (org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent t : src.getContact()) 047 tgt.addContact(convertConceptMapContactComponent(t)); 048 if (src.hasDate()) 049 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 050 if (src.hasDescription()) 051 tgt.setDescription(src.getDescription()); 052 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 053 if (VersionConvertor_14_40.isJurisdiction(t)) 054 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 055 else 056 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 057 if (src.hasRequirements()) 058 tgt.setPurpose(src.getRequirements()); 059 if (src.hasCopyright()) 060 tgt.setCopyright(src.getCopyright()); 061 org.hl7.fhir.r4.model.Type tt = ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getSource()); 062 tgt.setSource(tt instanceof org.hl7.fhir.r4.model.Reference ? new CanonicalType(((org.hl7.fhir.r4.model.Reference) tt).getReference()) : tt); 063 tt = ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getTarget()); 064 tgt.setTarget(tt instanceof org.hl7.fhir.r4.model.Reference ? new CanonicalType(((org.hl7.fhir.r4.model.Reference) tt).getReference()) : tt); 065 for (org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent t : src.getElement()) { 066 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> ws = convertSourceElementComponent(t); 067 for (SourceElementComponentWrapper<ConceptMap.SourceElementComponent> w : ws) 068 getGroup(tgt, w.getSource(), w.getTarget()).addElement(w.getComp()); 069 } 070 return tgt; 071 } 072 073 public static org.hl7.fhir.dstu2016may.model.ConceptMap convertConceptMap(org.hl7.fhir.r4.model.ConceptMap src) throws FHIRException { 074 if (src == null || src.isEmpty()) 075 return null; 076 org.hl7.fhir.dstu2016may.model.ConceptMap tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap(); 077 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 078 if (src.hasUrl()) 079 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 080 if (src.hasIdentifier()) 081 tgt.setIdentifier(Identifier14_40.convertIdentifier(src.getIdentifier())); 082 if (src.hasVersion()) 083 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 084 if (src.hasName()) 085 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 086 if (src.hasStatus()) 087 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 088 if (src.hasExperimental()) 089 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 090 if (src.hasPublisher()) 091 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 092 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) tgt.addContact(convertConceptMapContactComponent(t)); 093 if (src.hasDate()) 094 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 095 if (src.hasDescription()) 096 tgt.setDescription(src.getDescription()); 097 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 098 if (t.hasValueCodeableConcept()) 099 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 100 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 101 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 102 if (src.hasPurpose()) 103 tgt.setRequirements(src.getPurpose()); 104 if (src.hasCopyright()) 105 tgt.setCopyright(src.getCopyright()); 106 if (src.getSource() instanceof CanonicalType) 107 tgt.setSource(Reference14_40.convertCanonicalToReference((CanonicalType) src.getSource())); 108 else if (src.hasSource()) 109 tgt.setSource(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getSource())); 110 if (src.getTarget() instanceof CanonicalType) 111 tgt.setTarget(Reference14_40.convertCanonicalToReference((CanonicalType) src.getTarget())); 112 else if (src.hasTarget()) 113 tgt.setTarget(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getTarget())); 114 if (src.hasSource()) 115 tgt.setSource(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getSource())); 116 if (src.hasTarget()) 117 tgt.setTarget(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getTarget())); 118 for (org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent g : src.getGroup()) 119 for (org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent t : g.getElement()) 120 tgt.addElement(convertSourceElementComponent(t, g)); 121 return tgt; 122 } 123 124 public static org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent convertConceptMapContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 125 if (src == null || src.isEmpty()) 126 return null; 127 org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent(); 128 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 129 if (src.hasName()) 130 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 131 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 132 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 133 return tgt; 134 } 135 136 public static org.hl7.fhir.r4.model.ContactDetail convertConceptMapContactComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.ConceptMapContactComponent src) throws FHIRException { 137 if (src == null || src.isEmpty()) 138 return null; 139 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 140 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 141 if (src.hasName()) 142 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 143 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 144 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 145 return tgt; 146 } 147 148 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 149 if (src == null || src.isEmpty()) 150 return null; 151 Enumeration<Enumerations.ConceptMapEquivalence> tgt = new Enumeration<>(new Enumerations.ConceptMapEquivalenceEnumFactory()); 152 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 153 if (src.getValue() == null) { 154 tgt.setValue(null); 155 } else { 156 switch (src.getValue()) { 157 case EQUIVALENT: 158 tgt.setValue(Enumerations.ConceptMapEquivalence.EQUIVALENT); 159 break; 160 case EQUAL: 161 tgt.setValue(Enumerations.ConceptMapEquivalence.EQUAL); 162 break; 163 case WIDER: 164 tgt.setValue(Enumerations.ConceptMapEquivalence.WIDER); 165 break; 166 case SUBSUMES: 167 tgt.setValue(Enumerations.ConceptMapEquivalence.SUBSUMES); 168 break; 169 case NARROWER: 170 tgt.setValue(Enumerations.ConceptMapEquivalence.NARROWER); 171 break; 172 case SPECIALIZES: 173 tgt.setValue(Enumerations.ConceptMapEquivalence.SPECIALIZES); 174 break; 175 case INEXACT: 176 tgt.setValue(Enumerations.ConceptMapEquivalence.INEXACT); 177 break; 178 case UNMATCHED: 179 tgt.setValue(Enumerations.ConceptMapEquivalence.UNMATCHED); 180 break; 181 case DISJOINT: 182 tgt.setValue(Enumerations.ConceptMapEquivalence.DISJOINT); 183 break; 184 default: 185 tgt.setValue(Enumerations.ConceptMapEquivalence.NULL); 186 break; 187 } 188 } 189 return tgt; 190 } 191 192 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 193 if (src == null || src.isEmpty()) 194 return null; 195 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 196 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 197 if (src.getValue() == null) { 198 tgt.setValue(null); 199 } else { 200 switch (src.getValue()) { 201 case EQUIVALENT: 202 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 203 break; 204 case EQUAL: 205 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.EQUAL); 206 break; 207 case WIDER: 208 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.WIDER); 209 break; 210 case SUBSUMES: 211 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.SUBSUMES); 212 break; 213 case NARROWER: 214 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.NARROWER); 215 break; 216 case SPECIALIZES: 217 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.SPECIALIZES); 218 break; 219 case INEXACT: 220 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.INEXACT); 221 break; 222 case UNMATCHED: 223 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.UNMATCHED); 224 break; 225 case DISJOINT: 226 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.DISJOINT); 227 break; 228 default: 229 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConceptMapEquivalence.NULL); 230 break; 231 } 232 } 233 return tgt; 234 } 235 236 public static org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent(); 240 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 241 if (src.hasElementElement()) 242 tgt.setPropertyElement(Uri14_40.convertUri(src.getElementElement())); 243 if (src.hasSystem()) 244 tgt.setSystem(src.getSystem()); 245 if (src.hasCodeElement()) 246 tgt.setValueElement(String14_40.convertString(src.getCodeElement())); 247 return tgt; 248 } 249 250 public static org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent src) throws FHIRException { 251 if (src == null || src.isEmpty()) 252 return null; 253 org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent(); 254 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 255 if (src.hasPropertyElement()) 256 tgt.setElementElement(Uri14_40.convertUri(src.getPropertyElement())); 257 if (src.hasSystem()) 258 tgt.setSystem(src.getSystem()); 259 if (src.hasValueElement()) 260 tgt.setCodeElement(String14_40.convertString(src.getValueElement())); 261 return tgt; 262 } 263 264 public static List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> convertSourceElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent src) throws FHIRException { 265 List<SourceElementComponentWrapper<ConceptMap.SourceElementComponent>> res = new ArrayList<>(); 266 if (src == null || src.isEmpty()) 267 return res; 268 for (org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent t : src.getTarget()) { 269 org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent(); 270 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 271 if (src.hasCode()) 272 tgt.setCode(src.getCode()); 273 tgt.addTarget(convertTargetElementComponent(t)); 274 res.add(new SourceElementComponentWrapper<>(tgt, src.getSystem(), t.getSystem())); 275 } 276 return res; 277 } 278 279 public static org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent g) throws FHIRException { 280 if (src == null || src.isEmpty()) 281 return null; 282 org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.SourceElementComponent(); 283 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 284 if (g.hasSource()) 285 tgt.setSystem(g.getSource()); 286 if (src.hasCode()) 287 tgt.setCodeElement(Code14_40.convertCode(src.getCodeElement())); 288 for (org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent t : src.getTarget()) 289 tgt.addTarget(convertTargetElementComponent(t, g)); 290 return tgt; 291 } 292 293 public static org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent src) throws FHIRException { 294 if (src == null || src.isEmpty()) 295 return null; 296 org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent(); 297 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 298 if (src.hasCode()) 299 tgt.setCodeElement(Code14_40.convertCode(src.getCodeElement())); 300 if (src.hasEquivalence()) 301 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 302 if (src.hasComments()) 303 tgt.setCommentElement(String14_40.convertString(src.getCommentsElement())); 304 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 305 tgt.addDependsOn(convertOtherElementComponent(t)); 306 for (org.hl7.fhir.dstu2016may.model.ConceptMap.OtherElementComponent t : src.getProduct()) 307 tgt.addProduct(convertOtherElementComponent(t)); 308 return tgt; 309 } 310 311 public static org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent g) throws FHIRException { 312 if (src == null || src.isEmpty()) 313 return null; 314 org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.dstu2016may.model.ConceptMap.TargetElementComponent(); 315 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 316 if (g.hasTarget()) 317 tgt.setSystem(g.getTarget()); 318 if (src.hasCode()) 319 tgt.setCodeElement(Code14_40.convertCode(src.getCodeElement())); 320 if (src.hasEquivalence()) 321 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getEquivalenceElement())); 322 if (src.hasComment()) 323 tgt.setCommentsElement(String14_40.convertString(src.getCommentElement())); 324 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 325 tgt.addDependsOn(convertOtherElementComponent(t)); 326 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getProduct()) 327 tgt.addProduct(convertOtherElementComponent(t)); 328 return tgt; 329 } 330 331 static public ConceptMapGroupComponent getGroup(ConceptMap map, String srcs, String tgts) { 332 for (ConceptMapGroupComponent grp : map.getGroup()) { 333 if (grp.hasSource() && grp.getSource().equals(srcs) && grp.getTarget().equals(tgts)) 334 return grp; 335 } 336 ConceptMapGroupComponent grp = map.addGroup(); 337 grp.setSource(srcs); 338 grp.setTarget(tgts); 339 return grp; 340 } 341}