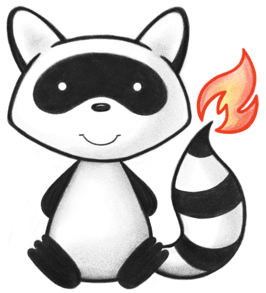
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.exceptions.FHIRException; 005import org.hl7.fhir.r4.model.Enumerations; 006 007public class Enumerations14_40 { 008 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength> src) throws FHIRException { 009 if (src == null || src.isEmpty()) return null; 010 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory()); 011 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 012 if (src.getValue() == null) { 013 tgt.setValue(null); 014} else { 015 switch(src.getValue()) { 016 case REQUIRED: 017 tgt.setValue(Enumerations.BindingStrength.REQUIRED); 018 break; 019 case EXTENSIBLE: 020 tgt.setValue(Enumerations.BindingStrength.EXTENSIBLE); 021 break; 022 case PREFERRED: 023 tgt.setValue(Enumerations.BindingStrength.PREFERRED); 024 break; 025 case EXAMPLE: 026 tgt.setValue(Enumerations.BindingStrength.EXAMPLE); 027 break; 028 default: 029 tgt.setValue(Enumerations.BindingStrength.NULL); 030 break; 031 } 032} 033 return tgt; 034 } 035 036 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> src) throws FHIRException { 037 if (src == null || src.isEmpty()) return null; 038 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrengthEnumFactory()); 039 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 040 if (src.getValue() == null) { 041 tgt.setValue(null); 042} else { 043 switch(src.getValue()) { 044 case REQUIRED: 045 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength.REQUIRED); 046 break; 047 case EXTENSIBLE: 048 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength.EXTENSIBLE); 049 break; 050 case PREFERRED: 051 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength.PREFERRED); 052 break; 053 case EXAMPLE: 054 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength.EXAMPLE); 055 break; 056 default: 057 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.BindingStrength.NULL); 058 break; 059 } 060} 061 return tgt; 062 } 063 064 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> convertConformanceResourceStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus> src) throws FHIRException { 065 if (src == null || src.isEmpty()) return null; 066 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory()); 067 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 068 if (src.getValue() == null) { 069 tgt.setValue(null); 070} else { 071 switch(src.getValue()) { 072 case DRAFT: 073 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 074 break; 075 case ACTIVE: 076 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 077 break; 078 case RETIRED: 079 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 080 break; 081 default: 082 tgt.setValue(Enumerations.PublicationStatus.NULL); 083 break; 084 } 085} 086 return tgt; 087 } 088 089 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus> convertConformanceResourceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> src) throws FHIRException { 090 if (src == null || src.isEmpty()) return null; 091 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatusEnumFactory()); 092 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 093 if (src.getValue() == null) { 094 tgt.setValue(null); 095} else { 096 switch(src.getValue()) { 097 case DRAFT: 098 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus.DRAFT); 099 break; 100 case ACTIVE: 101 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus.ACTIVE); 102 break; 103 case RETIRED: 104 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus.RETIRED); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.ConformanceResourceStatus.NULL); 108 break; 109 } 110} 111 return tgt; 112 } 113 114 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType> src) throws FHIRException { 115 if (src == null || src.isEmpty()) return null; 116 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory()); 117 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 118 if (src.getValue() == null) { 119 tgt.setValue(null); 120} else { 121 switch(src.getValue()) { 122 case NUMBER: 123 tgt.setValue(Enumerations.SearchParamType.NUMBER); 124 break; 125 case DATE: 126 tgt.setValue(Enumerations.SearchParamType.DATE); 127 break; 128 case STRING: 129 tgt.setValue(Enumerations.SearchParamType.STRING); 130 break; 131 case TOKEN: 132 tgt.setValue(Enumerations.SearchParamType.TOKEN); 133 break; 134 case REFERENCE: 135 tgt.setValue(Enumerations.SearchParamType.REFERENCE); 136 break; 137 case COMPOSITE: 138 tgt.setValue(Enumerations.SearchParamType.COMPOSITE); 139 break; 140 case QUANTITY: 141 tgt.setValue(Enumerations.SearchParamType.QUANTITY); 142 break; 143 case URI: 144 tgt.setValue(Enumerations.SearchParamType.URI); 145 break; 146 default: 147 tgt.setValue(Enumerations.SearchParamType.NULL); 148 break; 149 } 150} 151 return tgt; 152 } 153 154 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> src) throws FHIRException { 155 if (src == null || src.isEmpty()) return null; 156 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamTypeEnumFactory()); 157 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 158 if (src.getValue() == null) { 159 tgt.setValue(null); 160} else { 161 switch(src.getValue()) { 162 case NUMBER: 163 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.NUMBER); 164 break; 165 case DATE: 166 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.DATE); 167 break; 168 case STRING: 169 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.STRING); 170 break; 171 case TOKEN: 172 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.TOKEN); 173 break; 174 case REFERENCE: 175 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.REFERENCE); 176 break; 177 case COMPOSITE: 178 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.COMPOSITE); 179 break; 180 case QUANTITY: 181 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.QUANTITY); 182 break; 183 case URI: 184 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.URI); 185 break; 186 default: 187 tgt.setValue(org.hl7.fhir.dstu2016may.model.Enumerations.SearchParamType.NULL); 188 break; 189 } 190} 191 return tgt; 192 } 193}