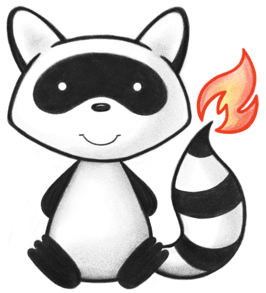
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import java.util.List; 004 005import org.hl7.fhir.convertors.VersionConvertorConstants; 006import org.hl7.fhir.convertors.context.ConversionContext14_40; 007import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Reference14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Code14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 015import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 016import org.hl7.fhir.dstu2016may.model.ImplementationGuide; 017import org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuidePageKind; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.CanonicalType; 020import org.hl7.fhir.r4.model.Enumeration; 021import org.hl7.fhir.r4.model.ImplementationGuide.GuidePageGeneration; 022import org.hl7.fhir.r4.model.Type; 023 024public class ImplementationGuide14_40 { 025 026 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.r4.model.ImplementationGuide src) throws FHIRException { 027 if (src == null || src.isEmpty()) 028 return null; 029 org.hl7.fhir.dstu2016may.model.ImplementationGuide tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide(); 030 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 031 if (src.hasUrlElement()) 032 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 033 if (src.hasVersion()) 034 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 035 if (src.hasNameElement()) 036 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 037 if (src.hasStatus()) 038 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 039 if (src.hasExperimental()) 040 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 041 if (src.hasPublisher()) 042 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 043 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 044 tgt.addContact(convertImplementationGuideContactComponent(t)); 045 if (src.hasDate()) 046 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 047 if (src.hasDescription()) 048 tgt.setDescription(src.getDescription()); 049 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 050 if (t.hasValueCodeableConcept()) 051 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 052 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 053 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 054 if (src.hasCopyright()) 055 tgt.setCopyright(src.getCopyright()); 056 if (src.hasFhirVersion()) 057 for (Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> v : src.getFhirVersion()) { 058 tgt.setFhirVersion(v.asStringValue()); 059 } 060 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent t : src.getDependsOn()) 061 tgt.addDependency(convertImplementationGuideDependencyComponent(t)); 062 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent t : src.getDefinition().getGrouping()) 063 tgt.addPackage(convertImplementationGuidePackageComponent(t)); 064 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent t : src.getDefinition().getResource()) 065 findPackage(tgt.getPackage(), t.getGroupingId()).addResource(convertImplementationGuidePackageResourceComponent(t)); 066 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 067 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 068 tgt.setPage(convertImplementationGuidePageComponent(src.getDefinition().getPage())); 069 return tgt; 070 } 071 072 public static org.hl7.fhir.r4.model.ImplementationGuide convertImplementationGuide(org.hl7.fhir.dstu2016may.model.ImplementationGuide src) throws FHIRException { 073 if (src == null || src.isEmpty()) 074 return null; 075 org.hl7.fhir.r4.model.ImplementationGuide tgt = new org.hl7.fhir.r4.model.ImplementationGuide(); 076 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 077 if (src.hasUrlElement()) 078 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 079 if (src.hasVersion()) 080 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 081 if (src.hasNameElement()) 082 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 083 if (src.hasStatus()) 084 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 085 if (src.hasExperimental()) 086 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 087 if (src.hasPublisher()) 088 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 089 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent t : src.getContact()) 090 tgt.addContact(convertImplementationGuideContactComponent(t)); 091 if (src.hasDate()) 092 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 093 if (src.hasDescription()) 094 tgt.setDescription(src.getDescription()); 095 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 096 if (VersionConvertor_14_40.isJurisdiction(t)) 097 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 098 else 099 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 100 if (src.hasCopyright()) 101 tgt.setCopyright(src.getCopyright()); 102 if (src.hasFhirVersion()) 103 tgt.addFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 104 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent t : src.getDependency()) 105 tgt.addDependsOn(convertImplementationGuideDependencyComponent(t)); 106 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t : src.getPackage()) 107 tgt.getDefinition().addGrouping(convertImplementationGuidePackageComponent(tgt.getDefinition(), t)); 108 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent t : src.getGlobal()) 109 tgt.addGlobal(convertImplementationGuideGlobalComponent(t)); 110 tgt.getDefinition().setPage(convertImplementationGuidePageComponent(src.getPage())); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.r4.model.ContactDetail convertImplementationGuideContactComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent src) throws FHIRException { 115 if (src == null || src.isEmpty()) 116 return null; 117 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 118 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 119 if (src.hasName()) 120 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 121 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 122 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 123 return tgt; 124 } 125 126 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent convertImplementationGuideContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 127 if (src == null || src.isEmpty()) 128 return null; 129 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideContactComponent(); 130 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 131 if (src.hasName()) 132 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 133 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 134 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent src) throws FHIRException { 139 if (src == null || src.isEmpty()) 140 return null; 141 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent(); 142 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 143 tgt.setType(org.hl7.fhir.dstu2016may.model.ImplementationGuide.GuideDependencyType.REFERENCE); 144 if (src.hasUri()) 145 tgt.setUri(src.getUri()); 146 return tgt; 147 } 148 149 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent convertImplementationGuideDependencyComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideDependencyComponent src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDependsOnComponent(); 153 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 154 if (src.hasUri()) 155 tgt.setUri(src.getUri()); 156 if (org.hl7.fhir.dstu2016may.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)) { 157 tgt.setPackageId(org.hl7.fhir.dstu2016may.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_PACKAGE_EXTENSION)); 158 } 159 if (org.hl7.fhir.dstu2016may.utils.ToolingExtensions.hasExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)) { 160 tgt.setVersion(org.hl7.fhir.dstu2016may.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_IG_DEPENDSON_VERSION_EXTENSION)); 161 } 162 return tgt; 163 } 164 165 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 166 if (src == null || src.isEmpty()) 167 return null; 168 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 169 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 170 if (src.hasTypeElement()) 171 tgt.setTypeElement(Code14_40.convertCode(src.getTypeElement())); 172 if (src.hasProfile()) 173 tgt.setProfileElement(Reference14_40.convertReferenceToCanonical(src.getProfile())); 174 return tgt; 175 } 176 177 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent convertImplementationGuideGlobalComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideGlobalComponent src) throws FHIRException { 178 if (src == null || src.isEmpty()) 179 return null; 180 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuideGlobalComponent(); 181 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 182 if (src.hasTypeElement()) 183 tgt.setTypeElement(Code14_40.convertCode(src.getTypeElement())); 184 if (src.hasProfileElement()) 185 tgt.setProfile(Reference14_40.convertCanonicalToReference(src.getProfileElement())); 186 return tgt; 187 } 188 189 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionComponent context, org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent src) throws FHIRException { 190 if (src == null || src.isEmpty()) 191 return null; 192 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent(); 193 tgt.setId("p" + (context.getGrouping().size() + 1)); 194 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 195 if (src.hasName()) 196 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 197 if (src.hasDescription()) 198 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 199 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent t : src.getResource()) { 200 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tn = convertImplementationGuidePackageResourceComponent(t); 201 tn.setGroupingId(tgt.getId()); 202 context.addResource(tn); 203 } 204 return tgt; 205 } 206 207 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent convertImplementationGuidePackageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionGroupingComponent src) throws FHIRException { 208 if (src == null || src.isEmpty()) 209 return null; 210 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent(); 211 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 212 tgt.setId(src.getId()); 213 if (src.hasNameElement()) 214 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 215 if (src.hasDescription()) 216 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent(); 224 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 225 if (src.hasExampleCanonicalType()) { 226 if (src.hasExampleCanonicalType()) 227 tgt.setExampleFor(Reference14_40.convertCanonicalToReference(src.getExampleCanonicalType())); 228 tgt.setExample(true); 229 } else if (src.hasExampleBooleanType()) 230 tgt.setExample(src.getExampleBooleanType().getValue()); 231 else 232 tgt.setExample(false); 233 if (src.hasName()) 234 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 235 if (src.hasDescription()) 236 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 237 if (src.hasReference()) 238 tgt.setSource(Reference14_40.convertReference(src.getReference())); 239 return tgt; 240 } 241 242 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent convertImplementationGuidePackageResourceComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageResourceComponent src) throws FHIRException { 243 if (src == null || src.isEmpty()) 244 return null; 245 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionResourceComponent(); 246 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 247 if (src.hasExampleFor()) { 248 Type t = ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getExampleFor()); 249 tgt.setExample(t instanceof org.hl7.fhir.r4.model.Reference ? new CanonicalType(((org.hl7.fhir.r4.model.Reference) t).getReference()) : t); 250 } else if (src.hasExample()) 251 tgt.setExample(new org.hl7.fhir.r4.model.BooleanType(src.getExample())); 252 if (src.hasName()) 253 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 254 if (src.hasDescription()) 255 tgt.setDescriptionElement(String14_40.convertString(src.getDescriptionElement())); 256 if (src.hasSourceReference()) 257 tgt.setReference(Reference14_40.convertReference(src.getSourceReference())); 258 else if (src.hasSourceUriType()) 259 tgt.setReference(new org.hl7.fhir.r4.model.Reference(src.getSourceUriType().getValue())); 260 return tgt; 261 } 262 263 public static org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent convertImplementationGuidePageComponent(org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent src) throws FHIRException { 264 if (src == null || src.isEmpty()) 265 return null; 266 org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent tgt = new org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent(); 267 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 268 if (src.hasSource()) 269 tgt.setName(convertUriToUrl(src.getSourceElement())); 270 if (src.hasNameElement()) 271 tgt.setTitleElement(String14_40.convertString(src.getNameElement())); 272 if (src.hasKind()) 273 tgt.setGeneration(convertPageGeneration(src.getKind())); 274 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent t : src.getPage()) 275 tgt.addPage(convertImplementationGuidePageComponent(t)); 276 return tgt; 277 } 278 279 public static org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent convertImplementationGuidePageComponent(org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent src) throws FHIRException { 280 if (src == null || src.isEmpty()) 281 return null; 282 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent tgt = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePageComponent(); 283 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 284 if (src.hasNameUrlType()) 285 tgt.setSource(src.getNameUrlType().getValue()); 286 if (src.hasTitleElement()) 287 tgt.setNameElement(String14_40.convertString(src.getTitleElement())); 288 if (src.hasGeneration()) 289 tgt.setKind(convertPageGeneration(src.getGeneration())); 290 for (org.hl7.fhir.r4.model.ImplementationGuide.ImplementationGuideDefinitionPageComponent t : src.getPage()) 291 tgt.addPage(convertImplementationGuidePageComponent(t)); 292 return tgt; 293 } 294 295 static public GuidePageKind convertPageGeneration(GuidePageGeneration generation) { 296 switch (generation) { 297 case HTML: 298 return GuidePageKind.PAGE; 299 default: 300 return GuidePageKind.RESOURCE; 301 } 302 } 303 304 static public GuidePageGeneration convertPageGeneration(GuidePageKind kind) { 305 switch (kind) { 306 case PAGE: 307 return GuidePageGeneration.HTML; 308 default: 309 return GuidePageGeneration.GENERATED; 310 } 311 } 312 313 public static org.hl7.fhir.r4.model.UrlType convertUriToUrl(org.hl7.fhir.dstu2016may.model.UriType src) throws FHIRException { 314 org.hl7.fhir.r4.model.UrlType tgt = new org.hl7.fhir.r4.model.UrlType(); 315 if (src.hasValue()) 316 tgt.setValue(src.getValue()); 317 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 318 return tgt; 319 } 320 321 static public org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent findPackage(List<ImplementationGuide.ImplementationGuidePackageComponent> definition, String id) { 322 for (org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t : definition) 323 if (t.hasId() && t.getId().equals(id)) 324 return t; 325 org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent t = new org.hl7.fhir.dstu2016may.model.ImplementationGuide.ImplementationGuidePackageComponent(); 326 t.setName("Default Package"); 327 t.setId(id); 328 return t; 329 } 330}