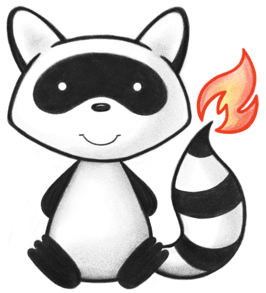
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Expression14_40; 005import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r4.model.Enumeration; 009import org.hl7.fhir.r4.model.OperationOutcome; 010 011public class OperationOutcome14_40 { 012 013 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.OperationOutcome.IssueSeverity> convertIssueSeverity(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity> src) throws FHIRException { 014 if (src == null || src.isEmpty()) 015 return null; 016 Enumeration<OperationOutcome.IssueSeverity> tgt = new Enumeration<>(new OperationOutcome.IssueSeverityEnumFactory()); 017 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 018 if (src.getValue() == null) { 019 tgt.setValue(null); 020 } else { 021 switch (src.getValue()) { 022 case FATAL: 023 tgt.setValue(OperationOutcome.IssueSeverity.FATAL); 024 break; 025 case ERROR: 026 tgt.setValue(OperationOutcome.IssueSeverity.ERROR); 027 break; 028 case WARNING: 029 tgt.setValue(OperationOutcome.IssueSeverity.WARNING); 030 break; 031 case INFORMATION: 032 tgt.setValue(OperationOutcome.IssueSeverity.INFORMATION); 033 break; 034 default: 035 tgt.setValue(OperationOutcome.IssueSeverity.NULL); 036 break; 037 } 038 } 039 return tgt; 040 } 041 042 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity> convertIssueSeverity(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.OperationOutcome.IssueSeverity> src) throws FHIRException { 043 if (src == null || src.isEmpty()) 044 return null; 045 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverityEnumFactory()); 046 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 047 if (src.getValue() == null) { 048 tgt.setValue(null); 049 } else { 050 switch (src.getValue()) { 051 case FATAL: 052 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity.FATAL); 053 break; 054 case ERROR: 055 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity.ERROR); 056 break; 057 case WARNING: 058 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity.WARNING); 059 break; 060 case INFORMATION: 061 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity.INFORMATION); 062 break; 063 default: 064 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueSeverity.NULL); 065 break; 066 } 067 } 068 return tgt; 069 } 070 071 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.OperationOutcome.IssueType> convertIssueType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType> src) throws FHIRException { 072 if (src == null || src.isEmpty()) 073 return null; 074 Enumeration<OperationOutcome.IssueType> tgt = new Enumeration<>(new OperationOutcome.IssueTypeEnumFactory()); 075 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 076 if (src.getValue() == null) { 077 tgt.setValue(null); 078 } else { 079 switch (src.getValue()) { 080 case INVALID: 081 tgt.setValue(OperationOutcome.IssueType.INVALID); 082 break; 083 case STRUCTURE: 084 tgt.setValue(OperationOutcome.IssueType.STRUCTURE); 085 break; 086 case REQUIRED: 087 tgt.setValue(OperationOutcome.IssueType.REQUIRED); 088 break; 089 case VALUE: 090 tgt.setValue(OperationOutcome.IssueType.VALUE); 091 break; 092 case INVARIANT: 093 tgt.setValue(OperationOutcome.IssueType.INVARIANT); 094 break; 095 case SECURITY: 096 tgt.setValue(OperationOutcome.IssueType.SECURITY); 097 break; 098 case LOGIN: 099 tgt.setValue(OperationOutcome.IssueType.LOGIN); 100 break; 101 case UNKNOWN: 102 tgt.setValue(OperationOutcome.IssueType.UNKNOWN); 103 break; 104 case EXPIRED: 105 tgt.setValue(OperationOutcome.IssueType.EXPIRED); 106 break; 107 case FORBIDDEN: 108 tgt.setValue(OperationOutcome.IssueType.FORBIDDEN); 109 break; 110 case SUPPRESSED: 111 tgt.setValue(OperationOutcome.IssueType.SUPPRESSED); 112 break; 113 case PROCESSING: 114 tgt.setValue(OperationOutcome.IssueType.PROCESSING); 115 break; 116 case NOTSUPPORTED: 117 tgt.setValue(OperationOutcome.IssueType.NOTSUPPORTED); 118 break; 119 case DUPLICATE: 120 tgt.setValue(OperationOutcome.IssueType.DUPLICATE); 121 break; 122 case NOTFOUND: 123 tgt.setValue(OperationOutcome.IssueType.NOTFOUND); 124 break; 125 case TOOLONG: 126 tgt.setValue(OperationOutcome.IssueType.TOOLONG); 127 break; 128 case CODEINVALID: 129 tgt.setValue(OperationOutcome.IssueType.CODEINVALID); 130 break; 131 case EXTENSION: 132 tgt.setValue(OperationOutcome.IssueType.EXTENSION); 133 break; 134 case TOOCOSTLY: 135 tgt.setValue(OperationOutcome.IssueType.TOOCOSTLY); 136 break; 137 case BUSINESSRULE: 138 tgt.setValue(OperationOutcome.IssueType.BUSINESSRULE); 139 break; 140 case CONFLICT: 141 tgt.setValue(OperationOutcome.IssueType.CONFLICT); 142 break; 143 case INCOMPLETE: 144 tgt.setValue(OperationOutcome.IssueType.INCOMPLETE); 145 break; 146 case TRANSIENT: 147 tgt.setValue(OperationOutcome.IssueType.TRANSIENT); 148 break; 149 case LOCKERROR: 150 tgt.setValue(OperationOutcome.IssueType.LOCKERROR); 151 break; 152 case NOSTORE: 153 tgt.setValue(OperationOutcome.IssueType.NOSTORE); 154 break; 155 case EXCEPTION: 156 tgt.setValue(OperationOutcome.IssueType.EXCEPTION); 157 break; 158 case TIMEOUT: 159 tgt.setValue(OperationOutcome.IssueType.TIMEOUT); 160 break; 161 case THROTTLED: 162 tgt.setValue(OperationOutcome.IssueType.THROTTLED); 163 break; 164 case INFORMATIONAL: 165 tgt.setValue(OperationOutcome.IssueType.INFORMATIONAL); 166 break; 167 default: 168 tgt.setValue(OperationOutcome.IssueType.NULL); 169 break; 170 } 171 } 172 return tgt; 173 } 174 175 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType> convertIssueType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.OperationOutcome.IssueType> src) throws FHIRException { 176 if (src == null || src.isEmpty()) 177 return null; 178 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueTypeEnumFactory()); 179 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 180 if (src.getValue() == null) { 181 tgt.setValue(null); 182 } else { 183 switch (src.getValue()) { 184 case INVALID: 185 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.INVALID); 186 break; 187 case STRUCTURE: 188 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.STRUCTURE); 189 break; 190 case REQUIRED: 191 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.REQUIRED); 192 break; 193 case VALUE: 194 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.VALUE); 195 break; 196 case INVARIANT: 197 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.INVARIANT); 198 break; 199 case SECURITY: 200 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.SECURITY); 201 break; 202 case LOGIN: 203 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.LOGIN); 204 break; 205 case UNKNOWN: 206 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.UNKNOWN); 207 break; 208 case EXPIRED: 209 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.EXPIRED); 210 break; 211 case FORBIDDEN: 212 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.FORBIDDEN); 213 break; 214 case SUPPRESSED: 215 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.SUPPRESSED); 216 break; 217 case PROCESSING: 218 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.PROCESSING); 219 break; 220 case NOTSUPPORTED: 221 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.NOTSUPPORTED); 222 break; 223 case DUPLICATE: 224 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.DUPLICATE); 225 break; 226 case NOTFOUND: 227 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.NOTFOUND); 228 break; 229 case TOOLONG: 230 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.TOOLONG); 231 break; 232 case CODEINVALID: 233 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.CODEINVALID); 234 break; 235 case EXTENSION: 236 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.EXTENSION); 237 break; 238 case TOOCOSTLY: 239 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.TOOCOSTLY); 240 break; 241 case BUSINESSRULE: 242 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.BUSINESSRULE); 243 break; 244 case CONFLICT: 245 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.CONFLICT); 246 break; 247 case INCOMPLETE: 248 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.INCOMPLETE); 249 break; 250 case TRANSIENT: 251 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.TRANSIENT); 252 break; 253 case LOCKERROR: 254 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.LOCKERROR); 255 break; 256 case NOSTORE: 257 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.NOSTORE); 258 break; 259 case EXCEPTION: 260 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.EXCEPTION); 261 break; 262 case TIMEOUT: 263 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.TIMEOUT); 264 break; 265 case THROTTLED: 266 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.THROTTLED); 267 break; 268 case INFORMATIONAL: 269 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.INFORMATIONAL); 270 break; 271 default: 272 tgt.setValue(org.hl7.fhir.dstu2016may.model.OperationOutcome.IssueType.NULL); 273 break; 274 } 275 } 276 return tgt; 277 } 278 279 public static org.hl7.fhir.dstu2016may.model.OperationOutcome convertOperationOutcome(org.hl7.fhir.r4.model.OperationOutcome src) throws FHIRException { 280 if (src == null || src.isEmpty()) 281 return null; 282 org.hl7.fhir.dstu2016may.model.OperationOutcome tgt = new org.hl7.fhir.dstu2016may.model.OperationOutcome(); 283 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 284 for (org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent t : src.getIssue()) 285 tgt.addIssue(convertOperationOutcomeIssueComponent(t)); 286 return tgt; 287 } 288 289 public static org.hl7.fhir.r4.model.OperationOutcome convertOperationOutcome(org.hl7.fhir.dstu2016may.model.OperationOutcome src) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 org.hl7.fhir.r4.model.OperationOutcome tgt = new org.hl7.fhir.r4.model.OperationOutcome(); 293 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 294 for (org.hl7.fhir.dstu2016may.model.OperationOutcome.OperationOutcomeIssueComponent t : src.getIssue()) 295 tgt.addIssue(convertOperationOutcomeIssueComponent(t)); 296 return tgt; 297 } 298 299 public static org.hl7.fhir.dstu2016may.model.OperationOutcome.OperationOutcomeIssueComponent convertOperationOutcomeIssueComponent(org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent src) throws FHIRException { 300 if (src == null || src.isEmpty()) 301 return null; 302 org.hl7.fhir.dstu2016may.model.OperationOutcome.OperationOutcomeIssueComponent tgt = new org.hl7.fhir.dstu2016may.model.OperationOutcome.OperationOutcomeIssueComponent(); 303 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 304 if (src.hasSeverity()) 305 tgt.setSeverityElement(convertIssueSeverity(src.getSeverityElement())); 306 if (src.hasCode()) 307 tgt.setCodeElement(convertIssueType(src.getCodeElement())); 308 if (src.hasDetails()) 309 tgt.setDetails(CodeableConcept14_40.convertCodeableConcept(src.getDetails())); 310 if (src.hasDiagnostics()) 311 tgt.setDiagnosticsElement(String14_40.convertString(src.getDiagnosticsElement())); 312 for (org.hl7.fhir.r4.model.StringType t : src.getLocation()) tgt.addLocation(t.getValue()); 313 for (org.hl7.fhir.r4.model.StringType t : src.getExpression()) 314 tgt.addExpression(Expression14_40.convertTo2016MayExpression(t.getValue())); 315 return tgt; 316 } 317 318 public static org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent convertOperationOutcomeIssueComponent(org.hl7.fhir.dstu2016may.model.OperationOutcome.OperationOutcomeIssueComponent src) throws FHIRException { 319 if (src == null || src.isEmpty()) 320 return null; 321 org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent tgt = new org.hl7.fhir.r4.model.OperationOutcome.OperationOutcomeIssueComponent(); 322 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 323 if (src.hasSeverity()) 324 tgt.setSeverityElement(convertIssueSeverity(src.getSeverityElement())); 325 if (src.hasCode()) 326 tgt.setCodeElement(convertIssueType(src.getCodeElement())); 327 if (src.hasDetails()) 328 tgt.setDetails(CodeableConcept14_40.convertCodeableConcept(src.getDetails())); 329 if (src.hasDiagnostics()) 330 tgt.setDiagnosticsElement(String14_40.convertString(src.getDiagnosticsElement())); 331 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getLocation()) tgt.addLocation(t.getValue()); 332 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getExpression()) 333 tgt.addExpression(Expression14_40.convertToR4Expression(t.getValue())); 334 return tgt; 335 } 336}