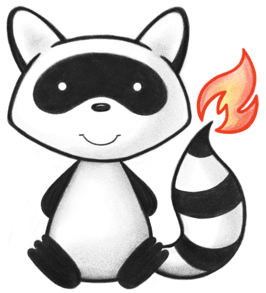
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 005import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Reference14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Coding14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Identifier14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Integer14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r4.model.*; 017import org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator; 018 019public class Questionnaire14_40 { 020 021 public static org.hl7.fhir.r4.model.Questionnaire convertQuestionnaire(org.hl7.fhir.dstu2016may.model.Questionnaire src) throws FHIRException { 022 if (src == null || src.isEmpty()) 023 return null; 024 org.hl7.fhir.r4.model.Questionnaire tgt = new org.hl7.fhir.r4.model.Questionnaire(); 025 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 026 if (src.hasUrl()) 027 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 028 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 029 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 030 if (src.hasVersion()) 031 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 032 if (src.hasStatus()) 033 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 034 if (src.hasDate()) 035 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 036 if (src.hasPublisher()) 037 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 038 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 039 tgt.addContact(convertQuestionnaireContactComponent(t)); 040 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 041 if (VersionConvertor_14_40.isJurisdiction(t)) 042 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 043 else 044 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 045 if (src.hasTitle()) 046 tgt.setTitleElement(String14_40.convertString(src.getTitleElement())); 047 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Coding14_40.convertCoding(t)); 048 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 049 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 050 tgt.addItem(convertQuestionnaireItemComponent(t)); 051 return tgt; 052 } 053 054 public static org.hl7.fhir.dstu2016may.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r4.model.Questionnaire src) throws FHIRException { 055 if (src == null || src.isEmpty()) 056 return null; 057 org.hl7.fhir.dstu2016may.model.Questionnaire tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire(); 058 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 059 if (src.hasUrl()) 060 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 061 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 062 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 063 if (src.hasVersion()) 064 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 065 if (src.hasStatus()) 066 tgt.setStatusElement(convertQuestionnaireStatus(src.getStatusElement())); 067 if (src.hasDate()) 068 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 069 if (src.hasPublisher()) 070 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 071 for (ContactDetail t : src.getContact()) 072 for (org.hl7.fhir.r4.model.ContactPoint t1 : t.getTelecom()) 073 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t1)); 074 for (UsageContext t : src.getUseContext()) 075 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 076 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 077 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 078 if (src.hasTitle()) 079 tgt.setTitleElement(String14_40.convertString(src.getTitleElement())); 080 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addConcept(Coding14_40.convertCoding(t)); 081 for (org.hl7.fhir.r4.model.CodeType t : src.getSubjectType()) tgt.addSubjectType(t.getValue()); 082 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 083 tgt.addItem(convertQuestionnaireItemComponent(t)); 084 return tgt; 085 } 086 087 public static org.hl7.fhir.r4.model.ContactDetail convertQuestionnaireContactComponent(org.hl7.fhir.dstu2016may.model.ContactPoint src) throws FHIRException { 088 if (src == null || src.isEmpty()) 089 return null; 090 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 091 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 092 tgt.addTelecom(ContactPoint14_40.convertContactPoint(src)); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 097 if (src == null || src.isEmpty()) 098 return null; 099 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent(); 100 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 101 if (src.hasLinkId()) 102 tgt.setLinkIdElement(String14_40.convertString(src.getLinkIdElement())); 103 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getConcept()) tgt.addCode(Coding14_40.convertCoding(t)); 104 if (src.hasPrefix()) 105 tgt.setPrefixElement(String14_40.convertString(src.getPrefixElement())); 106 if (src.hasText()) 107 tgt.setTextElement(String14_40.convertString(src.getTextElement())); 108 if (src.hasType()) 109 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 110 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 111 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 112 if (src.hasRequired()) 113 tgt.setRequiredElement(Boolean14_40.convertBoolean(src.getRequiredElement())); 114 if (src.hasRepeats()) 115 tgt.setRepeatsElement(Boolean14_40.convertBoolean(src.getRepeatsElement())); 116 if (src.hasReadOnly()) 117 tgt.setReadOnlyElement(Boolean14_40.convertBoolean(src.getReadOnlyElement())); 118 if (src.hasMaxLength()) 119 tgt.setMaxLengthElement(Integer14_40.convertInteger(src.getMaxLengthElement())); 120 if (src.hasOptions()) 121 tgt.setAnswerValueSetElement(Reference14_40.convertReferenceToCanonical(src.getOptions())); 122 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent t : src.getOption()) 123 tgt.addAnswerOption(convertQuestionnaireItemOptionComponent(t)); 124 if (src.hasInitial()) 125 tgt.addInitial().setValue(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getInitial())); 126 for (org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 127 tgt.addItem(convertQuestionnaireItemComponent(t)); 128 return tgt; 129 } 130 131 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemComponent(); 135 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 136 if (src.hasLinkId()) 137 tgt.setLinkIdElement(String14_40.convertString(src.getLinkIdElement())); 138 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addConcept(Coding14_40.convertCoding(t)); 139 if (src.hasPrefix()) 140 tgt.setPrefixElement(String14_40.convertString(src.getPrefixElement())); 141 if (src.hasText()) 142 tgt.setTextElement(String14_40.convertString(src.getTextElement())); 143 if (src.hasType()) 144 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 145 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 146 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 147 if (src.hasRequired()) 148 tgt.setRequiredElement(Boolean14_40.convertBoolean(src.getRequiredElement())); 149 if (src.hasRepeats()) 150 tgt.setRepeatsElement(Boolean14_40.convertBoolean(src.getRepeatsElement())); 151 if (src.hasReadOnly()) 152 tgt.setReadOnlyElement(Boolean14_40.convertBoolean(src.getReadOnlyElement())); 153 if (src.hasMaxLength()) 154 tgt.setMaxLengthElement(Integer14_40.convertInteger(src.getMaxLengthElement())); 155 if (src.hasAnswerValueSetElement()) 156 tgt.setOptions(Reference14_40.convertCanonicalToReference(src.getAnswerValueSetElement())); 157 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 158 tgt.addOption(convertQuestionnaireItemOptionComponent(t)); 159 if (src.hasInitial()) 160 tgt.setInitial(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getInitialFirstRep().getValue())); 161 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 162 tgt.addItem(convertQuestionnaireItemComponent(t)); 163 return tgt; 164 } 165 166 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 167 if (src == null || src.isEmpty()) 168 return null; 169 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 170 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 171 if (src.hasQuestionElement()) 172 tgt.setQuestionElement(String14_40.convertString(src.getQuestionElement())); 173 if (src.hasAnswered()) { 174 tgt.setOperator(QuestionnaireItemOperator.EXISTS); 175 if (src.hasAnsweredElement()) 176 tgt.setAnswer(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getAnsweredElement())); 177 } 178 if (src.hasAnswer()) 179 tgt.setAnswer(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getAnswer())); 180 return tgt; 181 } 182 183 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 184 if (src == null || src.isEmpty()) 185 return null; 186 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 187 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 188 if (src.hasQuestionElement()) 189 tgt.setQuestionElement(String14_40.convertString(src.getQuestionElement())); 190 if (src.hasOperator() && src.getOperator() == QuestionnaireItemOperator.EXISTS) 191 tgt.setAnswered(src.getAnswerBooleanType().getValue()); 192 else 193 tgt.setAnswer(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getAnswer())); 194 return tgt; 195 } 196 197 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 201 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 202 if (src.hasValue()) 203 tgt.setValue(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getValue())); 204 return tgt; 205 } 206 207 public static org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent convertQuestionnaireItemOptionComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 208 if (src == null || src.isEmpty()) 209 return null; 210 org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent tgt = new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemOptionComponent(); 211 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 212 if (src.hasValue()) 213 tgt.setValue(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getValue())); 214 return tgt; 215 } 216 217 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 218 if (src == null || src.isEmpty()) 219 return null; 220 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 221 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 222 if (src.getValue() == null) { 223 tgt.setValue(null); 224 } else { 225 switch (src.getValue()) { 226 case GROUP: 227 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 228 break; 229 case DISPLAY: 230 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 231 break; 232 case QUESTION: 233 tgt.setValue(Questionnaire.QuestionnaireItemType.QUESTION); 234 break; 235 case BOOLEAN: 236 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 237 break; 238 case DECIMAL: 239 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 240 break; 241 case INTEGER: 242 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 243 break; 244 case DATE: 245 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 246 break; 247 case DATETIME: 248 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 249 break; 250 case INSTANT: 251 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 252 break; 253 case TIME: 254 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 255 break; 256 case STRING: 257 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 258 break; 259 case TEXT: 260 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 261 break; 262 case URL: 263 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 264 break; 265 case CHOICE: 266 tgt.setValue(Questionnaire.QuestionnaireItemType.CHOICE); 267 break; 268 case OPENCHOICE: 269 tgt.setValue(Questionnaire.QuestionnaireItemType.OPENCHOICE); 270 break; 271 case ATTACHMENT: 272 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 273 break; 274 case REFERENCE: 275 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 276 break; 277 case QUANTITY: 278 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 279 break; 280 default: 281 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 282 break; 283 } 284 } 285 return tgt; 286 } 287 288 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 289 if (src == null || src.isEmpty()) 290 return null; 291 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 292 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 293 if (src.getValue() == null) { 294 tgt.setValue(null); 295 } else { 296 switch (src.getValue()) { 297 case GROUP: 298 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.GROUP); 299 break; 300 case DISPLAY: 301 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DISPLAY); 302 break; 303 case QUESTION: 304 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.QUESTION); 305 break; 306 case BOOLEAN: 307 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.BOOLEAN); 308 break; 309 case DECIMAL: 310 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DECIMAL); 311 break; 312 case INTEGER: 313 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.INTEGER); 314 break; 315 case DATE: 316 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DATE); 317 break; 318 case DATETIME: 319 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.DATETIME); 320 break; 321 case TIME: 322 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.TIME); 323 break; 324 case STRING: 325 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.STRING); 326 break; 327 case TEXT: 328 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.TEXT); 329 break; 330 case URL: 331 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.URL); 332 break; 333 case CHOICE: 334 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.CHOICE); 335 break; 336 case OPENCHOICE: 337 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.OPENCHOICE); 338 break; 339 case ATTACHMENT: 340 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.ATTACHMENT); 341 break; 342 case REFERENCE: 343 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.REFERENCE); 344 break; 345 case QUANTITY: 346 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.QUANTITY); 347 break; 348 default: 349 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireItemType.NULL); 350 break; 351 } 352 } 353 return tgt; 354 } 355 356 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> convertQuestionnaireStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> src) throws FHIRException { 357 if (src == null || src.isEmpty()) 358 return null; 359 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatusEnumFactory()); 360 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 361 if (src.getValue() == null) { 362 tgt.setValue(null); 363 } else { 364 switch (src.getValue()) { 365 case DRAFT: 366 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.DRAFT); 367 break; 368 case ACTIVE: 369 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.PUBLISHED); 370 break; 371 case RETIRED: 372 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.RETIRED); 373 break; 374 default: 375 tgt.setValue(org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus.NULL); 376 break; 377 } 378 } 379 return tgt; 380 } 381 382 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> convertQuestionnaireStatus(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.Questionnaire.QuestionnaireStatus> src) throws FHIRException { 383 if (src == null || src.isEmpty()) 384 return null; 385 Enumeration<Enumerations.PublicationStatus> tgt = new Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 386 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 387 if (src.getValue() == null) { 388 tgt.setValue(null); 389 } else { 390 switch (src.getValue()) { 391 case DRAFT: 392 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 393 break; 394 case PUBLISHED: 395 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 396 break; 397 case RETIRED: 398 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 399 break; 400 default: 401 tgt.setValue(Enumerations.PublicationStatus.NULL); 402 break; 403 } 404 } 405 return tgt; 406 } 407}