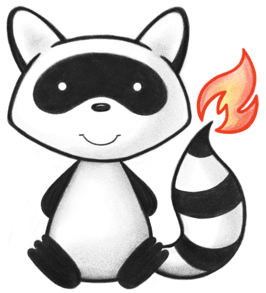
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 005import org.hl7.fhir.convertors.conv14_40.datatypes14_40.Expression14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Code14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r4.model.Enumeration; 015import org.hl7.fhir.r4.model.SearchParameter; 016 017public class SearchParameter14_40 { 018 019 public static org.hl7.fhir.r4.model.SearchParameter convertSearchParameter(org.hl7.fhir.dstu2016may.model.SearchParameter src) throws FHIRException { 020 if (src == null || src.isEmpty()) 021 return null; 022 org.hl7.fhir.r4.model.SearchParameter tgt = new org.hl7.fhir.r4.model.SearchParameter(); 023 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 024 if (src.hasUrlElement()) 025 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 026 if (src.hasNameElement()) 027 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 028 if (src.hasStatus()) 029 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 030 if (src.hasExperimental()) 031 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 032 if (src.hasDate()) 033 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 034 if (src.hasPublisher()) 035 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 036 for (org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent t : src.getContact()) 037 tgt.addContact(convertSearchParameterContactComponent(t)); 038 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 039 if (VersionConvertor_14_40.isJurisdiction(t)) 040 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 041 else 042 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 043 if (src.hasRequirements()) 044 tgt.setPurpose(src.getRequirements()); 045 if (src.hasCodeElement()) 046 tgt.setCodeElement(Code14_40.convertCode(src.getCodeElement())); 047 tgt.addBase(src.getBase()); 048 if (src.hasType()) 049 tgt.setTypeElement(Enumerations14_40.convertSearchParamType(src.getTypeElement())); 050 if (src.hasDescription()) 051 tgt.setDescription(src.getDescription()); 052 if (src.hasExpression()) 053 tgt.setExpression(Expression14_40.convertToR4Expression(src.getExpression())); 054 if (src.hasXpath()) 055 tgt.setXpathElement(String14_40.convertString(src.getXpathElement())); 056 if (src.hasXpathUsage()) 057 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 058 for (org.hl7.fhir.dstu2016may.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 059 return tgt; 060 } 061 062 public static org.hl7.fhir.dstu2016may.model.SearchParameter convertSearchParameter(org.hl7.fhir.r4.model.SearchParameter src) throws FHIRException { 063 if (src == null || src.isEmpty()) 064 return null; 065 org.hl7.fhir.dstu2016may.model.SearchParameter tgt = new org.hl7.fhir.dstu2016may.model.SearchParameter(); 066 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 067 if (src.hasUrlElement()) 068 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 069 if (src.hasNameElement()) 070 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 071 if (src.hasStatus()) 072 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 073 if (src.hasExperimental()) 074 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 075 if (src.hasDate()) 076 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 077 if (src.hasPublisher()) 078 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 079 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 080 tgt.addContact(convertSearchParameterContactComponent(t)); 081 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 082 if (t.hasValueCodeableConcept()) 083 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 084 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 085 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 086 if (src.hasPurpose()) 087 tgt.setRequirements(src.getPurpose()); 088 if (src.hasCodeElement()) 089 tgt.setCodeElement(Code14_40.convertCode(src.getCodeElement())); 090 for (org.hl7.fhir.r4.model.CodeType t : src.getBase()) tgt.setBase(t.asStringValue()); 091 if (src.hasType()) 092 tgt.setTypeElement(Enumerations14_40.convertSearchParamType(src.getTypeElement())); 093 if (src.hasDescription()) 094 tgt.setDescription(src.getDescription()); 095 if (src.hasExpression()) 096 tgt.setExpression(Expression14_40.convertTo2016MayExpression(src.getExpression())); 097 if (src.hasXpath()) 098 tgt.setXpathElement(String14_40.convertString(src.getXpathElement())); 099 if (src.hasXpathUsage()) 100 tgt.setXpathUsageElement(convertXPathUsageType(src.getXpathUsageElement())); 101 for (org.hl7.fhir.r4.model.CodeType t : src.getTarget()) tgt.addTarget(t.getValue()); 102 return tgt; 103 } 104 105 public static org.hl7.fhir.r4.model.ContactDetail convertSearchParameterContactComponent(org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent src) throws FHIRException { 106 if (src == null || src.isEmpty()) 107 return null; 108 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 109 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 110 if (src.hasName()) 111 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 112 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 113 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 114 return tgt; 115 } 116 117 public static org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent convertSearchParameterContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 118 if (src == null || src.isEmpty()) 119 return null; 120 org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent tgt = new org.hl7.fhir.dstu2016may.model.SearchParameter.SearchParameterContactComponent(); 121 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 122 if (src.hasName()) 123 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 124 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 125 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 126 return tgt; 127 } 128 129 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType> src) throws FHIRException { 130 if (src == null || src.isEmpty()) 131 return null; 132 Enumeration<SearchParameter.XPathUsageType> tgt = new Enumeration<>(new SearchParameter.XPathUsageTypeEnumFactory()); 133 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 134 if (src.getValue() == null) { 135 tgt.setValue(null); 136 } else { 137 switch (src.getValue()) { 138 case NORMAL: 139 tgt.setValue(SearchParameter.XPathUsageType.NORMAL); 140 break; 141 case PHONETIC: 142 tgt.setValue(SearchParameter.XPathUsageType.PHONETIC); 143 break; 144 case NEARBY: 145 tgt.setValue(SearchParameter.XPathUsageType.NEARBY); 146 break; 147 case DISTANCE: 148 tgt.setValue(SearchParameter.XPathUsageType.DISTANCE); 149 break; 150 case OTHER: 151 tgt.setValue(SearchParameter.XPathUsageType.OTHER); 152 break; 153 default: 154 tgt.setValue(SearchParameter.XPathUsageType.NULL); 155 break; 156 } 157 } 158 return tgt; 159 } 160 161 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageTypeEnumFactory()); 165 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 166 if (src.getValue() == null) { 167 tgt.setValue(null); 168 } else { 169 switch (src.getValue()) { 170 case NORMAL: 171 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.NORMAL); 172 break; 173 case PHONETIC: 174 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.PHONETIC); 175 break; 176 case NEARBY: 177 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.NEARBY); 178 break; 179 case DISTANCE: 180 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.DISTANCE); 181 break; 182 case OTHER: 183 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.OTHER); 184 break; 185 default: 186 tgt.setValue(org.hl7.fhir.dstu2016may.model.SearchParameter.XPathUsageType.NULL); 187 break; 188 } 189 } 190 return tgt; 191 } 192}