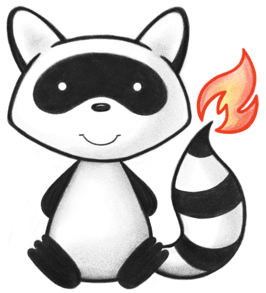
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_40; 004import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 005import org.hl7.fhir.convertors.conv14_40.datatypes14_40.ElementDefinition14_40; 006import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Coding14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Identifier14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Id14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r4.model.ElementDefinition; 017import org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule; 018import org.hl7.fhir.utilities.Utilities; 019 020public class StructureDefinition14_40 { 021 022 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> convertExtensionContext(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> src) throws FHIRException { 023 if (src == null || src.isEmpty()) 024 return null; 025 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextTypeEnumFactory()); 026 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 027 switch (src.getValue()) { 028 case RESOURCE: 029 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.ELEMENT); 030 break; 031 case DATATYPE: 032 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.ELEMENT); 033 break; 034 case EXTENSION: 035 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.EXTENSION); 036 break; 037 default: 038 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.NULL); 039 break; 040 } 041 return tgt; 042 } 043 044 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> convertExtensionContext(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> src, String expression) throws FHIRException { 045 if (src == null || src.isEmpty()) 046 return null; 047 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContextEnumFactory()); 048 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 049 switch (src.getValue()) { 050 case FHIRPATH: 051 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.RESOURCE); 052 break; 053 case ELEMENT: 054 String tn = expression.contains(".") ? expression.substring(0, expression.indexOf(".")) : expression; 055 if (isResource140(tn)) { 056 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.RESOURCE); 057 } else { 058 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.DATATYPE); 059 } 060 break; 061 case EXTENSION: 062 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.EXTENSION); 063 break; 064 default: 065 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.ExtensionContext.NULL); 066 break; 067 } 068 return tgt; 069 } 070 071 public static org.hl7.fhir.dstu2016may.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r4.model.StructureDefinition src) throws FHIRException { 072 if (src == null || src.isEmpty()) 073 return null; 074 org.hl7.fhir.dstu2016may.model.StructureDefinition tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition(); 075 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 076 if (src.hasUrl()) 077 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 078 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 079 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 080 if (src.hasVersion()) 081 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 082 if (src.hasNameElement()) 083 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 084 if (src.hasTitle()) 085 tgt.setDisplayElement(String14_40.convertString(src.getTitleElement())); 086 if (src.hasStatus()) 087 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 088 if (src.hasExperimental()) 089 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 090 if (src.hasPublisher()) 091 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 092 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 093 tgt.addContact(convertStructureDefinitionContactComponent(t)); 094 if (src.hasDate()) 095 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 096 if (src.hasDescription()) 097 tgt.setDescription(src.getDescription()); 098 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 099 if (t.hasValueCodeableConcept()) 100 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 101 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 102 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 103 if (src.hasPurpose()) 104 tgt.setRequirements(src.getPurpose()); 105 if (src.hasCopyright()) 106 tgt.setCopyright(src.getCopyright()); 107 for (org.hl7.fhir.r4.model.Coding t : src.getKeyword()) tgt.addCode(Coding14_40.convertCoding(t)); 108 if (src.hasFhirVersion()) 109 tgt.setFhirVersion(src.getFhirVersion().toCode()); 110 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 111 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 112 if (src.hasKind()) 113 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 114 if (src.hasAbstractElement()) 115 tgt.setAbstractElement(Boolean14_40.convertBoolean(src.getAbstractElement())); 116 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) { 117 if (!tgt.hasContextType()) 118 tgt.setContextTypeElement(convertExtensionContext(t.getTypeElement(), t.getExpression())); 119 tgt.addContext("Element".equals(t.getExpression()) ? "*" : t.getExpression()); 120 } 121 if (src.hasBaseDefinition()) 122 tgt.setBaseDefinition(src.getBaseDefinition()); 123 if (src.hasType() && src.getDerivation() == org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.CONSTRAINT) 124 tgt.setBaseType(src.getType()); 125 if (src.hasDerivation()) 126 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 127 if (src.hasSnapshot()) 128 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 129 if (src.hasDifferential()) 130 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 131 return tgt; 132 } 133 134 public static org.hl7.fhir.r4.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.dstu2016may.model.StructureDefinition src) throws FHIRException { 135 if (src == null || src.isEmpty()) 136 return null; 137 org.hl7.fhir.r4.model.StructureDefinition tgt = new org.hl7.fhir.r4.model.StructureDefinition(); 138 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 139 if (src.hasUrl()) 140 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 141 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 142 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 143 if (src.hasVersion()) 144 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 145 if (src.hasNameElement()) 146 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 147 if (src.hasDisplay()) 148 tgt.setTitleElement(String14_40.convertString(src.getDisplayElement())); 149 if (src.hasStatus()) 150 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 151 if (src.hasExperimental()) 152 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 153 if (src.hasPublisher()) 154 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 155 for (org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent t : src.getContact()) 156 tgt.addContact(convertStructureDefinitionContactComponent(t)); 157 if (src.hasDate()) 158 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 159 if (src.hasDescription()) 160 tgt.setDescription(src.getDescription()); 161 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 162 if (VersionConvertor_14_40.isJurisdiction(t)) 163 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 164 else 165 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 166 if (src.hasRequirements()) 167 tgt.setPurpose(src.getRequirements()); 168 if (src.hasCopyright()) 169 tgt.setCopyright(src.getCopyright()); 170 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getCode()) tgt.addKeyword(Coding14_40.convertCoding(t)); 171 if (src.hasFhirVersion()) 172 tgt.setFhirVersion(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.fromCode(src.getFhirVersion())); 173 for (org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 174 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 175 if (src.hasKind()) 176 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement(), src.getName())); 177 if (src.hasAbstractElement()) 178 tgt.setAbstractElement(Boolean14_40.convertBoolean(src.getAbstractElement())); 179 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getContext()) { 180 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent ec = tgt.addContext(); 181 ec.setTypeElement(convertExtensionContext(src.getContextTypeElement())); 182 ec.setExpression("*".equals(t.getValue()) ? "Element" : t.getValue()); 183 } 184 if (src.getDerivation() == org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.CONSTRAINT) 185 tgt.setType(src.getBaseType()); 186 else 187 tgt.setType(src.getId()); 188 if (src.hasBaseDefinition()) 189 tgt.setBaseDefinition(src.getBaseDefinition()); 190 if (src.hasDerivation()) 191 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 192 if (src.hasSnapshot()) { 193 if (src.hasSnapshot()) 194 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 195 tgt.getSnapshot().getElementFirstRep().getType().clear(); 196 } 197 if (src.hasDifferential()) { 198 if (src.hasDifferential()) 199 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 200 tgt.getDifferential().getElementFirstRep().getType().clear(); 201 } 202 if (tgt.getDerivation() == TypeDerivationRule.SPECIALIZATION) { 203 for (ElementDefinition ed : tgt.getSnapshot().getElement()) { 204 if (!ed.hasBase()) { 205 ed.getBase().setPath(ed.getPath()).setMin(ed.getMin()).setMax(ed.getMax()); 206 } 207 } 208 } 209 return tgt; 210 } 211 212 public static org.hl7.fhir.r4.model.ContactDetail convertStructureDefinitionContactComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent src) throws FHIRException { 213 if (src == null || src.isEmpty()) 214 return null; 215 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 216 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 217 if (src.hasName()) 218 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 219 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 220 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent convertStructureDefinitionContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 225 if (src == null || src.isEmpty()) 226 return null; 227 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionContactComponent(); 228 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 229 if (src.hasName()) 230 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 231 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 232 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 240 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 241 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 242 tgt.addElement(ElementDefinition14_40.convertElementDefinition(t)); 243 return tgt; 244 } 245 246 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 247 if (src == null || src.isEmpty()) 248 return null; 249 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 250 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 251 for (org.hl7.fhir.dstu2016may.model.ElementDefinition t : src.getElement()) 252 tgt.addElement(ElementDefinition14_40.convertElementDefinition(t, src.getElement(), src.getElement().indexOf(t))); 253 return tgt; 254 } 255 256 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 257 if (src == null || src.isEmpty()) 258 return null; 259 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 260 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 261 switch (src.getValue()) { 262 case PRIMITIVETYPE: 263 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 264 break; 265 case COMPLEXTYPE: 266 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.DATATYPE); 267 break; 268 case RESOURCE: 269 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 270 break; 271 case LOGICAL: 272 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 273 break; 274 default: 275 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind.NULL); 276 break; 277 } 278 return tgt; 279 } 280 281 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionKind> src, String name) throws FHIRException { 282 if (src == null || src.isEmpty()) 283 return null; 284 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 285 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 286 switch (src.getValue()) { 287 case DATATYPE: 288 if (name.substring(0, 1).toLowerCase().equals(name.substring(0, 1))) { 289 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 290 } else { 291 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 292 } 293 break; 294 case RESOURCE: 295 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 296 break; 297 case LOGICAL: 298 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 299 break; 300 default: 301 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.NULL); 302 break; 303 } 304 return tgt; 305 } 306 307 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 308 if (src == null || src.isEmpty()) 309 return null; 310 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent(); 311 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 312 if (src.hasIdentityElement()) 313 tgt.setIdentityElement(Id14_40.convertId(src.getIdentityElement())); 314 if (src.hasUri()) 315 tgt.setUriElement(Uri14_40.convertUri(src.getUriElement())); 316 if (src.hasName()) 317 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 318 if (src.hasComment()) 319 tgt.setCommentsElement(String14_40.convertString(src.getCommentElement())); 320 return tgt; 321 } 322 323 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 324 if (src == null || src.isEmpty()) 325 return null; 326 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent(); 327 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 328 if (src.hasIdentityElement()) 329 tgt.setIdentityElement(Id14_40.convertId(src.getIdentityElement())); 330 if (src.hasUri()) 331 tgt.setUriElement(Uri14_40.convertUri(src.getUriElement())); 332 if (src.hasName()) 333 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 334 if (src.hasComments()) 335 tgt.setCommentElement(String14_40.convertString(src.getCommentsElement())); 336 return tgt; 337 } 338 339 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 340 if (src == null || src.isEmpty()) 341 return null; 342 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 343 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 344 for (org.hl7.fhir.dstu2016may.model.ElementDefinition t : src.getElement()) 345 tgt.addElement(ElementDefinition14_40.convertElementDefinition(t, src.getElement(), src.getElement().indexOf(t))); 346 return tgt; 347 } 348 349 public static org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 350 if (src == null || src.isEmpty()) 351 return null; 352 org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 353 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 354 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 355 tgt.addElement(ElementDefinition14_40.convertElementDefinition(t)); 356 return tgt; 357 } 358 359 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 360 if (src == null || src.isEmpty()) 361 return null; 362 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 363 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 364 switch (src.getValue()) { 365 case SPECIALIZATION: 366 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 367 break; 368 case CONSTRAINT: 369 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 370 break; 371 default: 372 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule.NULL); 373 break; 374 } 375 return tgt; 376 } 377 378 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 379 if (src == null || src.isEmpty()) 380 return null; 381 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 382 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 383 switch (src.getValue()) { 384 case SPECIALIZATION: 385 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 386 break; 387 case CONSTRAINT: 388 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 389 break; 390 default: 391 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.NULL); 392 break; 393 } 394 return tgt; 395 } 396 397 static public boolean isResource140(String tn) { 398 return Utilities.existsInList(tn, "Account", "AllergyIntolerance", "Appointment", "AppointmentResponse", "AuditEvent", "Basic", "Binary", "BodySite", "Bundle", "CarePlan", "CareTeam", "Claim", "ClaimResponse", "ClinicalImpression", "CodeSystem", "Communication", "CommunicationRequest", "CompartmentDefinition", "Composition", "ConceptMap", "Condition", "Conformance", "Contract", "Coverage", "DataElement", "DecisionSupportRule", "DecisionSupportServiceModule", "DetectedIssue", "Device", "DeviceComponent", "DeviceMetric", "DeviceUseRequest", "DeviceUseStatement", "DiagnosticOrder", "DiagnosticReport", "DocumentManifest", "DocumentReference", "EligibilityRequest", "EligibilityResponse", "Encounter", "EnrollmentRequest", "EnrollmentResponse", "EpisodeOfCare", "ExpansionProfile", "ExplanationOfBenefit", "FamilyMemberHistory", "Flag", "Goal", "Group", "GuidanceResponse", "HealthcareService", "ImagingExcerpt", "ImagingObjectSelection", "ImagingStudy", "Immunization", "ImmunizationRecommendation", "ImplementationGuide", "Library", "Linkage", "List", "Location", "Measure", "MeasureReport", "Media", "Medication", "MedicationAdministration", "MedicationDispense", "MedicationOrder", "MedicationStatement", "MessageHeader", "ModuleDefinition", "NamingSystem", "NutritionOrder", "Observation", "OperationDefinition", "OperationOutcome", "Order", "OrderResponse", "OrderSet", "Organization", "Parameters", "Patient", "PaymentNotice", "PaymentReconciliation", "Person", "Practitioner", "PractitionerRole", "Procedure", "ProcedureRequest", "ProcessRequest", "ProcessResponse", "Protocol", "Provenance", "Questionnaire", "QuestionnaireResponse", "ReferralRequest", "RelatedPerson", "RiskAssessment", "Schedule", "SearchParameter", "Sequence", "Slot", "Sequence", "Specimen", "StructureDefinition", "StructureMap", "Subscription", "Substance", "SupplyDelivery", "SupplyRequest", "Task", "TestScript", "ValueSet", "VisionPrescription"); 399 } 400}