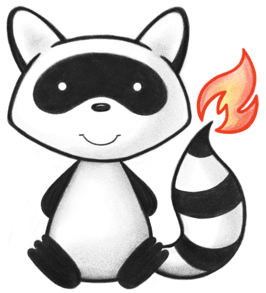
001package org.hl7.fhir.convertors.conv14_40.resources14_40; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext14_40; 006import org.hl7.fhir.convertors.conv14_40.VersionConvertor_14_40; 007import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.CodeableConcept14_40; 008import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.ContactPoint14_40; 009import org.hl7.fhir.convertors.conv14_40.datatypes14_40.complextypes14_40.Identifier14_40; 010import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Boolean14_40; 011import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.DateTime14_40; 012import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Id14_40; 013import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.String14_40; 014import org.hl7.fhir.convertors.conv14_40.datatypes14_40.primitivetypes14_40.Uri14_40; 015import org.hl7.fhir.dstu2016may.model.StructureMap; 016import org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType; 017import org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode; 018import org.hl7.fhir.exceptions.FHIRException; 019 020public class StructureMap14_40 { 021 022 public static org.hl7.fhir.dstu2016may.model.StructureMap convertStructureMap(org.hl7.fhir.r4.model.StructureMap src) throws FHIRException { 023 if (src == null || src.isEmpty()) 024 return null; 025 org.hl7.fhir.dstu2016may.model.StructureMap tgt = new org.hl7.fhir.dstu2016may.model.StructureMap(); 026 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 027 if (src.hasUrlElement()) 028 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 029 if (src.hasVersion()) 030 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 031 if (src.hasNameElement()) 032 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 033 if (src.hasStatus()) 034 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 035 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 036 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 037 if (src.hasExperimental()) 038 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 039 if (src.hasPublisher()) 040 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 041 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 042 tgt.addContact(convertStructureMapContactComponent(t)); 043 if (src.hasDate()) 044 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 045 if (src.hasDescription()) 046 tgt.setDescription(src.getDescription()); 047 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 048 if (t.hasValueCodeableConcept()) 049 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t.getValueCodeableConcept())); 050 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 051 tgt.addUseContext(CodeableConcept14_40.convertCodeableConcept(t)); 052 if (src.hasPurpose()) 053 tgt.setRequirements(src.getPurpose()); 054 if (src.hasCopyright()) 055 tgt.setCopyright(src.getCopyright()); 056 for (org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 057 tgt.addStructure(convertStructureMapStructureComponent(t)); 058 for (org.hl7.fhir.r4.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 059 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 060 tgt.addGroup(convertStructureMapGroupComponent(t)); 061 return tgt; 062 } 063 064 public static org.hl7.fhir.r4.model.StructureMap convertStructureMap(org.hl7.fhir.dstu2016may.model.StructureMap src) throws FHIRException { 065 if (src == null || src.isEmpty()) 066 return null; 067 org.hl7.fhir.r4.model.StructureMap tgt = new org.hl7.fhir.r4.model.StructureMap(); 068 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyDomainResource(src, tgt); 069 if (src.hasUrlElement()) 070 tgt.setUrlElement(Uri14_40.convertUri(src.getUrlElement())); 071 if (src.hasVersion()) 072 tgt.setVersionElement(String14_40.convertString(src.getVersionElement())); 073 if (src.hasNameElement()) 074 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 075 if (src.hasStatus()) 076 tgt.setStatusElement(Enumerations14_40.convertConformanceResourceStatus(src.getStatusElement())); 077 for (org.hl7.fhir.dstu2016may.model.Identifier t : src.getIdentifier()) 078 tgt.addIdentifier(Identifier14_40.convertIdentifier(t)); 079 if (src.hasExperimental()) 080 tgt.setExperimentalElement(Boolean14_40.convertBoolean(src.getExperimentalElement())); 081 if (src.hasPublisher()) 082 tgt.setPublisherElement(String14_40.convertString(src.getPublisherElement())); 083 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContactComponent t : src.getContact()) 084 tgt.addContact(convertStructureMapContactComponent(t)); 085 if (src.hasDate()) 086 tgt.setDateElement(DateTime14_40.convertDateTime(src.getDateElement())); 087 if (src.hasDescription()) 088 tgt.setDescription(src.getDescription()); 089 for (org.hl7.fhir.dstu2016may.model.CodeableConcept t : src.getUseContext()) 090 if (VersionConvertor_14_40.isJurisdiction(t)) 091 tgt.addJurisdiction(CodeableConcept14_40.convertCodeableConcept(t)); 092 else 093 tgt.addUseContext(CodeableConcept14_40.convertCodeableConceptToUsageContext(t)); 094 if (src.hasRequirements()) 095 tgt.setPurpose(src.getRequirements()); 096 if (src.hasCopyright()) 097 tgt.setCopyright(src.getCopyright()); 098 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 099 tgt.addStructure(convertStructureMapStructureComponent(t)); 100 for (org.hl7.fhir.dstu2016may.model.UriType t : src.getImport()) tgt.addImport(t.getValue()); 101 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 102 tgt.addGroup(convertStructureMapGroupComponent(t)); 103 return tgt; 104 } 105 106 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContactComponent convertStructureMapContactComponent(org.hl7.fhir.r4.model.ContactDetail src) throws FHIRException { 107 if (src == null || src.isEmpty()) 108 return null; 109 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContactComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContactComponent(); 110 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 111 if (src.hasName()) 112 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 113 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 114 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 115 return tgt; 116 } 117 118 public static org.hl7.fhir.r4.model.ContactDetail convertStructureMapContactComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContactComponent src) throws FHIRException { 119 if (src == null || src.isEmpty()) 120 return null; 121 org.hl7.fhir.r4.model.ContactDetail tgt = new org.hl7.fhir.r4.model.ContactDetail(); 122 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 123 if (src.hasName()) 124 tgt.setNameElement(String14_40.convertString(src.getNameElement())); 125 for (org.hl7.fhir.dstu2016may.model.ContactPoint t : src.getTelecom()) 126 tgt.addTelecom(ContactPoint14_40.convertContactPoint(t)); 127 return tgt; 128 } 129 130 public static org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapContextType> convertStructureMapContextType(org.hl7.fhir.dstu2016may.model.Enumeration<StructureMapContextType> src) throws FHIRException { 131 if (src == null || src.isEmpty()) 132 return null; 133 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapContextType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapContextTypeEnumFactory()); 134 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 135 switch (src.getValue()) { 136 case TYPE: 137 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapContextType.TYPE); 138 case VARIABLE: 139 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapContextType.VARIABLE); 140 default: 141 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapContextType.NULL); 142 } 143 return tgt; 144 } 145 146 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType> convertStructureMapContextType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapContextType> src) throws FHIRException { 147 if (src == null || src.isEmpty()) 148 return null; 149 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextTypeEnumFactory()); 150 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 151 switch (src.getValue()) { 152 case TYPE: 153 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType.TYPE); 154 break; 155 case VARIABLE: 156 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType.VARIABLE); 157 break; 158 default: 159 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapContextType.NULL); 160 break; 161 } 162 return tgt; 163 } 164 165 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 166 if (src == null || src.isEmpty()) 167 return null; 168 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupComponent(); 169 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 170 if (src.hasNameElement()) 171 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 172 if (src.hasExtends()) 173 tgt.setExtendsElement(Id14_40.convertId(src.getExtendsElement())); 174 if (!src.getTypeMode().equals(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode.NONE)) 175 throw new FHIRException("Unable to downgrade structure map with group.typeMode other than 'None': " + src.getTypeMode().getDisplay()); 176 if (src.hasDocumentation()) 177 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 178 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 179 tgt.addInput(convertStructureMapGroupInputComponent(t)); 180 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 181 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 182 return tgt; 183 } 184 185 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 186 if (src == null || src.isEmpty()) 187 return null; 188 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent(); 189 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 190 if (src.hasNameElement()) 191 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 192 if (src.hasExtends()) 193 tgt.setExtendsElement(Id14_40.convertId(src.getExtendsElement())); 194 tgt.setTypeMode(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode.NONE); 195 if (src.hasDocumentation()) 196 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 197 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 198 tgt.addInput(convertStructureMapGroupInputComponent(t)); 199 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 200 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupInputComponent(); 208 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 209 if (src.hasNameElement()) 210 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 211 if (src.hasType()) 212 tgt.setTypeElement(String14_40.convertString(src.getTypeElement())); 213 if (src.hasMode()) 214 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 215 if (src.hasDocumentation()) 216 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 221 if (src == null || src.isEmpty()) 222 return null; 223 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent(); 224 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 225 if (src.hasNameElement()) 226 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 227 if (src.hasType()) 228 tgt.setTypeElement(String14_40.convertString(src.getTypeElement())); 229 if (src.hasMode()) 230 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 231 if (src.hasDocumentation()) 232 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 237 if (src == null || src.isEmpty()) 238 return null; 239 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent(); 240 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 241 if (src.hasNameElement()) 242 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 243 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 244 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 245 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 246 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 247 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 248 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 249 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 250 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 251 if (src.hasDocumentation()) 252 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 253 return tgt; 254 } 255 256 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 257 if (src == null || src.isEmpty()) 258 return null; 259 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent(); 260 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 261 if (src.hasNameElement()) 262 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 263 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 264 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 265 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 266 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 267 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 268 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 269 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 270 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 271 if (src.hasDocumentation()) 272 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 273 return tgt; 274 } 275 276 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 277 if (src == null || src.isEmpty()) 278 return null; 279 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleDependentComponent(); 280 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 281 if (src.hasNameElement()) 282 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 283 for (org.hl7.fhir.r4.model.StringType t : src.getVariable()) tgt.addVariable(t.asStringValue()); 284 return tgt; 285 } 286 287 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 288 if (src == null || src.isEmpty()) 289 return null; 290 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent(); 291 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 292 if (src.hasNameElement()) 293 tgt.setNameElement(Id14_40.convertId(src.getNameElement())); 294 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getVariable()) tgt.addVariable(t.asStringValue()); 295 return tgt; 296 } 297 298 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 299 if (src == null || src.isEmpty()) 300 return null; 301 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent(); 302 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 303 tgt.setMin(src.getRequired() ? 1 : 0); 304 if (src.getContextType().equals(StructureMap.StructureMapContextType.TYPE)) 305 tgt.setType(src.getContext()); 306 if (src.hasElement()) 307 tgt.setElementElement(String14_40.convertString(src.getElementElement())); 308 if (src.hasListMode()) 309 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 310 if (src.hasVariable()) 311 tgt.setVariableElement(Id14_40.convertId(src.getVariableElement())); 312 if (src.hasCondition()) 313 tgt.setConditionElement(String14_40.convertString(src.getConditionElement())); 314 if (src.hasCheck()) 315 tgt.setCheckElement(String14_40.convertString(src.getCheckElement())); 316 return tgt; 317 } 318 319 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 320 if (src == null || src.isEmpty()) 321 return null; 322 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleSourceComponent(); 323 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 324 tgt.setContextType(StructureMapContextType.TYPE); 325 if (src.hasContextElement()) 326 tgt.setContextElement(Id14_40.convertId(src.getContextElement())); 327 if (src.hasElement()) 328 tgt.setElementElement(String14_40.convertString(src.getElementElement())); 329 if (src.hasListMode()) 330 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 331 if (src.hasVariable()) 332 tgt.setVariableElement(Id14_40.convertId(src.getVariableElement())); 333 if (src.hasCondition()) 334 tgt.setConditionElement(String14_40.convertString(src.getConditionElement())); 335 if (src.hasCheck()) 336 tgt.setCheckElement(String14_40.convertString(src.getCheckElement())); 337 return tgt; 338 } 339 340 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 341 if (src == null || src.isEmpty()) 342 return null; 343 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetComponent(); 344 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 345 if (src.hasContext()) 346 tgt.setContextElement(Id14_40.convertId(src.getContextElement())); 347 if (src.hasContextType()) 348 tgt.setContextTypeElement(convertStructureMapContextType(src.getContextTypeElement())); 349 if (src.hasElement()) 350 tgt.setElementElement(String14_40.convertString(src.getElementElement())); 351 if (src.hasVariable()) 352 tgt.setVariableElement(Id14_40.convertId(src.getVariableElement())); 353 tgt.setListMode(src.getListMode().stream() 354 .map(StructureMap14_40::convertStructureMapTargetListMode) 355 .collect(Collectors.toList())); 356 if (src.hasListRuleId()) 357 tgt.setListRuleIdElement(Id14_40.convertId(src.getListRuleIdElement())); 358 if (src.hasTransform()) 359 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 360 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 361 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 362 return tgt; 363 } 364 365 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 366 if (src == null || src.isEmpty()) 367 return null; 368 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent(); 369 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 370 if (src.hasContext()) 371 tgt.setContextElement(Id14_40.convertId(src.getContextElement())); 372 if (src.hasContextType()) 373 tgt.setContextTypeElement(convertStructureMapContextType(src.getContextTypeElement())); 374 if (src.hasElement()) 375 tgt.setElementElement(String14_40.convertString(src.getElementElement())); 376 if (src.hasVariable()) 377 tgt.setVariableElement(Id14_40.convertId(src.getVariableElement())); 378 for (org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode> t : src.getListMode()) 379 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(t, tgt.addListModeElement().setValue(convertStructureMapTargetListMode(t.getValue()))); 380 if (src.hasListRuleId()) 381 tgt.setListRuleIdElement(Id14_40.convertId(src.getListRuleIdElement())); 382 if (src.hasTransform()) 383 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 384 for (org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 385 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 386 return tgt; 387 } 388 389 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 390 if (src == null || src.isEmpty()) 391 return null; 392 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 393 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 394 if (src.hasValue()) 395 tgt.setValue(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getValue())); 396 return tgt; 397 } 398 399 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 400 if (src == null || src.isEmpty()) 401 return null; 402 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 403 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 404 if (src.hasValue()) 405 tgt.setValue(ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().convertType(src.getValue())); 406 return tgt; 407 } 408 409 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 410 if (src == null || src.isEmpty()) 411 return null; 412 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputModeEnumFactory()); 413 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 414 switch (src.getValue()) { 415 case SOURCE: 416 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode.SOURCE); 417 break; 418 case TARGET: 419 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode.TARGET); 420 break; 421 default: 422 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode.NULL); 423 break; 424 } 425 return tgt; 426 } 427 428 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 429 if (src == null || src.isEmpty()) 430 return null; 431 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapInputModeEnumFactory()); 432 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 433 switch (src.getValue()) { 434 case SOURCE: 435 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.SOURCE); 436 break; 437 case TARGET: 438 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.TARGET); 439 break; 440 default: 441 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.NULL); 442 break; 443 } 444 return tgt; 445 } 446 447 public static org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.dstu2016may.model.Enumeration<StructureMapListMode> src) throws FHIRException { 448 if (src == null || src.isEmpty()) 449 return null; 450 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListModeEnumFactory()); 451 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 452 switch (src.getValue()) { 453 case FIRST: 454 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.FIRST); 455 break; 456 case LAST: 457 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.LAST); 458 break; 459 default: 460 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.NULL); 461 break; 462 } 463 return tgt; 464 } 465 466 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode> convertStructureMapSourceListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 467 if (src == null || src.isEmpty()) 468 return null; 469 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListModeEnumFactory()); 470 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 471 switch (src.getValue()) { 472 case FIRST: 473 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.FIRST); 474 break; 475 case LAST: 476 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.LAST); 477 break; 478 default: 479 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.NULL); 480 break; 481 } 482 return tgt; 483 } 484 485 public static org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 486 if (src == null || src.isEmpty()) 487 return null; 488 org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapStructureComponent(); 489 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 490 if (src.hasUrl()) 491 tgt.setUrl(src.getUrl()); 492 if (src.hasMode()) 493 tgt.setModeElement(convertStructureMapStructureMode(src.getModeElement())); 494 if (src.hasDocumentation()) 495 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 496 return tgt; 497 } 498 499 public static org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 500 if (src == null || src.isEmpty()) 501 return null; 502 org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent(); 503 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyBackboneElement(src,tgt); 504 if (src.hasUrl()) 505 tgt.setUrl(src.getUrl()); 506 if (src.hasMode()) 507 tgt.setModeElement(convertStructureMapStructureMode(src.getModeElement())); 508 if (src.hasDocumentation()) 509 tgt.setDocumentationElement(String14_40.convertString(src.getDocumentationElement())); 510 return tgt; 511 } 512 513 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode> convertStructureMapStructureMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 514 if (src == null || src.isEmpty()) 515 return null; 516 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelModeEnumFactory()); 517 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 518 switch (src.getValue()) { 519 case PRODUCED: 520 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode.PRODUCED); 521 break; 522 case QUERIED: 523 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode.QUERIED); 524 break; 525 case SOURCE: 526 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode.SOURCE); 527 break; 528 case TARGET: 529 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode.TARGET); 530 break; 531 default: 532 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode.NULL); 533 break; 534 } 535 return tgt; 536 } 537 538 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> convertStructureMapStructureMode(org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 539 if (src == null || src.isEmpty()) 540 return null; 541 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapModelModeEnumFactory()); 542 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 543 switch (src.getValue()) { 544 case PRODUCED: 545 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.PRODUCED); 546 break; 547 case QUERIED: 548 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.QUERIED); 549 break; 550 case SOURCE: 551 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.SOURCE); 552 break; 553 case TARGET: 554 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.TARGET); 555 break; 556 default: 557 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.NULL); 558 break; 559 } 560 return tgt; 561 } 562 563 public static org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode convertStructureMapTargetListMode(StructureMapListMode src) throws FHIRException { 564 if (src == null) 565 return null; 566 switch (src) { 567 case FIRST: 568 return org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.FIRST; 569 case LAST: 570 return org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.LAST; 571 case SHARE: 572 return org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.SHARE; 573 default: 574 return org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.NULL; 575 } 576 } 577 578 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode> convertStructureMapTargetListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 579 if (src == null || src.isEmpty()) 580 return null; 581 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListModeEnumFactory()); 582 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 583 switch (src.getValue()) { 584 case FIRST: 585 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.FIRST); 586 break; 587 case LAST: 588 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.LAST); 589 break; 590 case SHARE: 591 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.SHARE); 592 break; 593 default: 594 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapListMode.NULL); 595 break; 596 } 597 return tgt; 598 } 599 600 static public org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> src) throws FHIRException { 601 if (src == null || src.isEmpty()) 602 return null; 603 org.hl7.fhir.dstu2016may.model.Enumeration<org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransformEnumFactory()); 604 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 605 switch (src.getValue()) { 606 case APPEND: 607 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.APPEND); 608 break; 609 case CAST: 610 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.CAST); 611 break; 612 case COPY: 613 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.COPY); 614 break; 615 case CREATE: 616 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.CREATE); 617 break; 618 case DATEOP: 619 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.DATEOP); 620 break; 621 case ESCAPE: 622 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.ESCAPE); 623 break; 624 case EVALUATE: 625 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.EVALUATE); 626 break; 627 case POINTER: 628 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.POINTER); 629 break; 630 case REFERENCE: 631 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.REFERENCE); 632 break; 633 case TRANSLATE: 634 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.TRANSLATE); 635 break; 636 case TRUNCATE: 637 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.TRUNCATE); 638 break; 639 case UUID: 640 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.UUID); 641 break; 642 default: 643 tgt.setValue(org.hl7.fhir.dstu2016may.model.StructureMap.StructureMapTransform.NULL); 644 break; 645 } 646 return tgt; 647 } 648 649 public static org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.dstu2016may.model.Enumeration<StructureMap.StructureMapTransform> src) throws FHIRException { 650 if (src == null || src.isEmpty()) 651 return null; 652 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapTransformEnumFactory()); 653 ConversionContext14_40.INSTANCE.getVersionConvertor_14_40().copyElement(src, tgt); 654 switch (src.getValue()) { 655 case APPEND: 656 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.APPEND); 657 break; 658 case CAST: 659 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CAST); 660 break; 661 case COPY: 662 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.COPY); 663 break; 664 case CREATE: 665 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CREATE); 666 break; 667 case DATEOP: 668 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.DATEOP); 669 break; 670 case ESCAPE: 671 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.ESCAPE); 672 break; 673 case EVALUATE: 674 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.EVALUATE); 675 break; 676 case POINTER: 677 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.POINTER); 678 break; 679 case REFERENCE: 680 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.REFERENCE); 681 break; 682 case TRANSLATE: 683 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.TRANSLATE); 684 break; 685 case TRUNCATE: 686 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.TRUNCATE); 687 break; 688 case UUID: 689 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.UUID); 690 break; 691 default: 692 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.NULL); 693 break; 694 } 695 return tgt; 696 } 697}