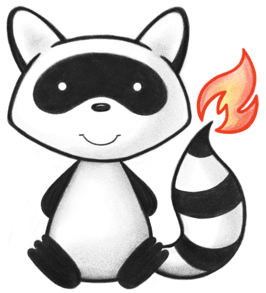
001package org.hl7.fhir.convertors.conv14_50; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import javax.annotation.Nonnull; 007 008import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_14_50; 009import org.hl7.fhir.convertors.context.ConversionContext14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.BackboneElement14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Element14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Type14_50; 013import org.hl7.fhir.convertors.conv14_50.resources14_50.Resource14_50; 014import org.hl7.fhir.exceptions.FHIRException; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 */ 043 044public class VersionConvertor_14_50 { 045 static public List<String> CANONICAL_URLS = new ArrayList<String>(); 046 047 static { 048 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/11179-permitted-value-conceptmap"); 049 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/11179-permitted-value-valueset"); 050 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/codesystem-map"); 051 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/cqif-library"); 052 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-allowedUnits"); 053 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-inheritedExtensibleValueSet"); 054 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-maxValueSet"); 055 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/elementdefinition-minValueSet"); 056 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/event-instantiatesCanonical"); 057 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-allowedProfile"); 058 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-deMap"); 059 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-sourceStructureMap"); 060 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-targetStructureMap"); 061 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/questionnaire-unit-valueSet"); 062 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-map"); 063 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-supplement"); 064 CANONICAL_URLS.add("http://hl7.org/fhir/StructureDefinition/valueset-system"); 065 } 066 067 private final BaseAdvisor_14_50 advisor; 068 private final Element14_50 elementConvertor; 069 070 private final BackboneElement14_50 backboneElementConvertor; 071 private final Resource14_50 resourceConvertor; 072 private final Type14_50 typeConvertor; 073 074 public VersionConvertor_14_50(@Nonnull BaseAdvisor_14_50 advisor) { 075 this.advisor = advisor; 076 this.elementConvertor = new Element14_50(advisor); 077 this.backboneElementConvertor = new BackboneElement14_50(); 078 this.resourceConvertor = new Resource14_50(advisor); 079 this.typeConvertor = new Type14_50(advisor); 080 } 081 082 public BaseAdvisor_14_50 advisor() { 083 return advisor; 084 } 085 086 public void copyResource(@Nonnull org.hl7.fhir.dstu2016may.model.Resource src, 087 @Nonnull org.hl7.fhir.r5.model.Resource tgt) throws FHIRException { 088 resourceConvertor.copyResource(src, tgt); 089 } 090 091 public void copyResource(@Nonnull org.hl7.fhir.r5.model.Resource src, 092 @Nonnull org.hl7.fhir.dstu2016may.model.Resource tgt) throws FHIRException { 093 resourceConvertor.copyResource(src, tgt); 094 } 095 096 public org.hl7.fhir.r5.model.Resource convertResource(@Nonnull org.hl7.fhir.dstu2016may.model.Resource src) throws FHIRException { 097 ConversionContext14_50.INSTANCE.init(this, src.fhirType()); 098 try { 099 return resourceConvertor.convertResource(src); 100 } finally { 101 ConversionContext14_50.INSTANCE.close(src.fhirType()); 102 } 103 } 104 105 public org.hl7.fhir.dstu2016may.model.Resource convertResource(@Nonnull org.hl7.fhir.r5.model.Resource src) throws FHIRException { 106 ConversionContext14_50.INSTANCE.init(this, src.fhirType()); 107 try { 108 return resourceConvertor.convertResource(src); 109 } finally { 110 ConversionContext14_50.INSTANCE.close(src.fhirType()); 111 } 112 } 113 114 public org.hl7.fhir.r5.model.DataType convertType(@Nonnull org.hl7.fhir.dstu2016may.model.Type src) throws FHIRException { 115 ConversionContext14_50.INSTANCE.init(this, src.fhirType()); 116 try { 117 return typeConvertor.convertType(src); 118 } finally { 119 ConversionContext14_50.INSTANCE.close(src.fhirType()); 120 } 121 } 122 123 public org.hl7.fhir.dstu2016may.model.Type convertType(@Nonnull org.hl7.fhir.r5.model.DataType src) throws FHIRException { 124 ConversionContext14_50.INSTANCE.init(this, src.fhirType()); 125 try { 126 return typeConvertor.convertType(src); 127 } finally { 128 ConversionContext14_50.INSTANCE.close(src.fhirType()); 129 } 130 } 131 132 public void copyDomainResource( 133 @Nonnull org.hl7.fhir.dstu2016may.model.DomainResource src, 134 @Nonnull org.hl7.fhir.r5.model.DomainResource tgt, 135 String... extensionUrlsToIgnore) throws FHIRException { 136 resourceConvertor.copyDomainResource(src, tgt, extensionUrlsToIgnore); 137 } 138 139 public void copyDomainResource( 140 @Nonnull org.hl7.fhir.r5.model.DomainResource src, 141 @Nonnull org.hl7.fhir.dstu2016may.model.DomainResource tgt, 142 String... extensionUrlsToIgnore) throws FHIRException { 143 resourceConvertor.copyDomainResource(src, tgt, extensionUrlsToIgnore); 144 } 145 146 public void copyElement( 147 @Nonnull org.hl7.fhir.dstu2016may.model.Element src, 148 @Nonnull org.hl7.fhir.r5.model.Element tgt, 149 String... extensionUrlsToIgnore) throws FHIRException { 150 elementConvertor.copyElement(src, tgt, ConversionContext14_50.INSTANCE.path(), extensionUrlsToIgnore); 151 } 152 153 public void copyElement( 154 @Nonnull org.hl7.fhir.r5.model.Element src, 155 @Nonnull org.hl7.fhir.dstu2016may.model.Element tgt, 156 String... extensionUrlsToIgnore) throws FHIRException { 157 elementConvertor.copyElement(src, tgt, ConversionContext14_50.INSTANCE.path(), extensionUrlsToIgnore); 158 } 159 160 public void copyBackboneElement( 161 @Nonnull org.hl7.fhir.r5.model.BackboneElement src, 162 @Nonnull org.hl7.fhir.dstu2016may.model.BackboneElement tgt, 163 String... extensionUrlsToIgnore) throws FHIRException { 164 backboneElementConvertor.copyBackboneElement(src, tgt, extensionUrlsToIgnore); 165 } 166 167 public void copyBackboneElement( 168 @Nonnull org.hl7.fhir.dstu2016may.model.BackboneElement src, 169 @Nonnull org.hl7.fhir.r5.model.BackboneElement tgt, 170 String... extensionUrlsToIgnore) throws FHIRException { 171 backboneElementConvertor.copyBackboneElement(src, tgt, extensionUrlsToIgnore); 172 } 173}