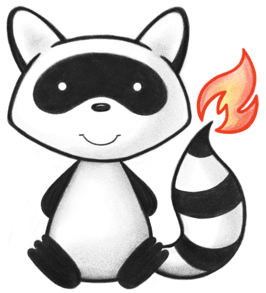
001package org.hl7.fhir.convertors.conv14_50.datatypes14_50; 002 003import java.util.Arrays; 004 005import org.hl7.fhir.convertors.advisors.impl.BaseAdvisor_14_50; 006import org.hl7.fhir.exceptions.FHIRException; 007 008public class Element14_50 { 009 010 public final BaseAdvisor_14_50 advisor; 011 012 public Element14_50(BaseAdvisor_14_50 advisor) { 013 this.advisor = advisor; 014 } 015 016 public boolean isExemptExtension(String url, String[] extensionsToIgnore) { 017 return Arrays.asList(extensionsToIgnore).contains(url); 018 } 019 020 021 public void copyElement(org.hl7.fhir.dstu2016may.model.Element src, 022 org.hl7.fhir.r5.model.Element tgt, 023 String path, 024 String... extensionUrlsToIgnore) throws FHIRException { 025 if (src.hasId()) tgt.setId(src.getId()); 026 src.getExtension().stream() 027 .filter(e -> !isExemptExtension(e.getUrl(), extensionUrlsToIgnore)) 028 .forEach(e -> { 029 if (advisor.useAdvisorForExtension(path, e)) { 030 org.hl7.fhir.r5.model.Extension convertedExtension = new org.hl7.fhir.r5.model.Extension(); 031 advisor.handleExtension(path, e, convertedExtension); 032 tgt.addExtension(convertedExtension); 033 } else { 034 tgt.addExtension(Extension14_50.convertExtension(e)); 035 } 036 }); 037 } 038 039 public void copyElement(org.hl7.fhir.r5.model.Element src, 040 org.hl7.fhir.dstu2016may.model.Element tgt, 041 String path, 042 String... extensionUrlsToIgnore) throws FHIRException { 043 if (src.hasId()) tgt.setId(src.getId()); 044 src.getExtension().stream() 045 .filter(e -> !isExemptExtension(e.getUrl(), extensionUrlsToIgnore)) 046 .forEach(e -> { 047 if (advisor.useAdvisorForExtension(path, e)) { 048 org.hl7.fhir.dstu2016may.model.Extension convertedExtension = new org.hl7.fhir.dstu2016may.model.Extension(); 049 advisor.handleExtension(path, e, convertedExtension); 050 tgt.addExtension(convertedExtension); 051 } else { 052 tgt.addExtension(Extension14_50.convertExtension(e)); 053 } 054 }); 055 } 056}