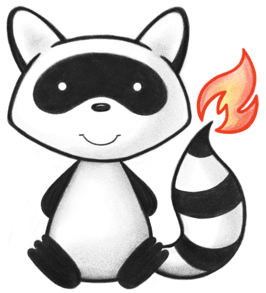
001package org.hl7.fhir.convertors.conv14_50.datatypes14_50; 002 003import java.util.List; 004import java.util.stream.Collectors; 005 006import org.apache.commons.lang3.StringUtils; 007import org.hl7.fhir.convertors.VersionConvertorConstants; 008import org.hl7.fhir.convertors.context.ConversionContext14_50; 009import org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50.Coding14_50; 010import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Boolean14_50; 011import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 012import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Id14_50; 013import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Integer14_50; 014import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.MarkDown14_50; 015import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 016import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Uri14_50; 017import org.hl7.fhir.convertors.conv14_50.resources14_50.Enumerations14_50; 018import org.hl7.fhir.dstu2016may.model.ElementDefinition; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r5.conformance.profile.ProfileUtilities; 021import org.hl7.fhir.utilities.Utilities; 022 023public class ElementDefinition14_50 { 024 public static org.hl7.fhir.r5.model.ElementDefinition convertElementDefinition(org.hl7.fhir.dstu2016may.model.ElementDefinition src, List<ElementDefinition> context, int pos) throws FHIRException { 025 if (src == null || src.isEmpty()) return null; 026 org.hl7.fhir.r5.model.ElementDefinition tgt = new org.hl7.fhir.r5.model.ElementDefinition(); 027 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt, VersionConvertorConstants.EXT_MUST_VALUE, VersionConvertorConstants.EXT_VALUE_ALT); 028 if (src.hasPathElement()) tgt.setPathElement(String14_50.convertString(src.getPathElement())); 029 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition14_50::convertPropertyRepresentation).collect(Collectors.toList())); 030 if (src.hasName()) tgt.setSliceNameElement(String14_50.convertString(src.getNameElement())); 031 if (src.hasLabel()) tgt.setLabelElement(String14_50.convertString(src.getLabelElement())); 032 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getCode()) tgt.addCode(Coding14_50.convertCoding(t)); 033 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing(), context, pos)); 034 if (src.hasShort()) tgt.setShortElement(String14_50.convertString(src.getShortElement())); 035 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown14_50.convertMarkdown(src.getDefinitionElement())); 036 if (src.hasComments()) tgt.setCommentElement(MarkDown14_50.convertMarkdown(src.getCommentsElement())); 037 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown14_50.convertMarkdown(src.getRequirementsElement())); 038 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 039 if (src.hasMin()) tgt.setMin(src.getMin()); 040 if (src.hasMax()) tgt.setMaxElement(String14_50.convertString(src.getMaxElement())); 041 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 042 if (src.hasContentReference()) 043 tgt.setContentReferenceElement(Uri14_50.convertUri(src.getContentReferenceElement())); 044 // work around for problem in R2B definitions: 045 if (!src.hasContentReference()) { 046 for (ElementDefinition.TypeRefComponent t : src.getType()) 047 convertTypeRefComponent(t, tgt.getType()); 048 if (src.hasDefaultValue()) 049 tgt.setDefaultValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getDefaultValue())); 050 if (src.hasMeaningWhenMissing()) 051 tgt.setMeaningWhenMissingElement(MarkDown14_50.convertMarkdown(src.getMeaningWhenMissingElement())); 052 if (src.hasFixed()) 053 tgt.setFixed(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getFixed())); 054 if (src.hasPattern()) 055 tgt.setPattern(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getPattern())); 056 if (src.hasExample()) 057 tgt.addExample().setLabel("General").setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getExample())); 058 if (src.hasMinValue()) 059 tgt.setMinValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getMinValue())); 060 if (src.hasMaxValue()) 061 tgt.setMaxValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getMaxValue())); 062 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer14_50.convertInteger(src.getMaxLengthElement())); 063 for (org.hl7.fhir.dstu2016may.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 064 for (ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 065 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 066 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean14_50.convertBoolean(src.getMustSupportElement())); 067 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean14_50.convertBoolean(src.getIsModifierElement())); 068 if (tgt.getIsModifier()) { 069 String reason = org.hl7.fhir.dstu2016may.utils.ToolingExtensions.readStringExtension(src, VersionConvertorConstants.EXT_MODIFIER_REASON_EXTENSION); 070 if (Utilities.noString(reason)) reason = VersionConvertorConstants.EXT_MODIFIER_REASON_LEGACY; 071 tgt.setIsModifierReason(reason); 072 } 073 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean14_50.convertBoolean(src.getIsSummaryElement())); 074 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 075 for (ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 076 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 077 } 078 079 if (src.hasExtension(VersionConvertorConstants.EXT_MUST_VALUE)) { 080 tgt.setMustHaveValueElement(Boolean14_50.convertBoolean((org.hl7.fhir.dstu2016may.model.BooleanType) src.getExtensionByUrl(VersionConvertorConstants.EXT_MUST_VALUE).getValue())); 081 } 082 for (org.hl7.fhir.dstu2016may.model.Extension ext : src.getExtensionsByUrl(VersionConvertorConstants.EXT_VALUE_ALT)) { 083 tgt.addValueAlternative(Uri14_50.convertCanonical((org.hl7.fhir.dstu2016may.model.UriType)ext.getValue())); 084 } 085 086 return tgt; 087 } 088 089 public static ElementDefinition convertElementDefinition(org.hl7.fhir.r5.model.ElementDefinition src) throws FHIRException { 090 if (src == null || src.isEmpty()) return null; 091 ElementDefinition tgt = new ElementDefinition(); 092 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 093 if (src.hasPathElement()) tgt.setPathElement(String14_50.convertString(src.getPathElement())); 094 tgt.setRepresentation(src.getRepresentation().stream().map(ElementDefinition14_50::convertPropertyRepresentation).collect(Collectors.toList())); 095 if (src.hasSliceName()) tgt.setNameElement(String14_50.convertString(src.getSliceNameElement())); 096 if (src.hasLabel()) tgt.setLabelElement(String14_50.convertString(src.getLabelElement())); 097 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding14_50.convertCoding(t)); 098 if (src.hasSlicing()) tgt.setSlicing(convertElementDefinitionSlicingComponent(src.getSlicing())); 099 if (src.hasShort()) tgt.setShortElement(String14_50.convertString(src.getShortElement())); 100 if (src.hasDefinition()) tgt.setDefinitionElement(MarkDown14_50.convertMarkdown(src.getDefinitionElement())); 101 if (src.hasComment()) tgt.setCommentsElement(MarkDown14_50.convertMarkdown(src.getCommentElement())); 102 if (src.hasRequirements()) tgt.setRequirementsElement(MarkDown14_50.convertMarkdown(src.getRequirementsElement())); 103 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.addAlias(t.getValue()); 104 if (src.hasMin()) tgt.setMin(src.getMin()); 105 if (src.hasMax()) tgt.setMaxElement(String14_50.convertString(src.getMaxElement())); 106 if (src.hasBase()) tgt.setBase(convertElementDefinitionBaseComponent(src.getBase())); 107 if (src.hasContentReference()) 108 tgt.setContentReferenceElement(Uri14_50.convertUri(src.getContentReferenceElement())); 109 for (org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent t : src.getType()) 110 convertTypeRefComponent(t, tgt.getType()); 111 if (src.hasDefaultValue()) 112 tgt.setDefaultValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getDefaultValue())); 113 if (src.hasMeaningWhenMissing()) 114 tgt.setMeaningWhenMissingElement(MarkDown14_50.convertMarkdown(src.getMeaningWhenMissingElement())); 115 if (src.hasFixed()) 116 tgt.setFixed(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getFixed())); 117 if (src.hasPattern()) 118 tgt.setPattern(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getPattern())); 119 if (src.hasExample()) 120 tgt.setExample(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getExample().get(0).getValue())); 121 if (src.hasMinValue()) 122 tgt.setMinValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getMinValue())); 123 if (src.hasMaxValue()) 124 tgt.setMaxValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getMaxValue())); 125 if (src.hasMaxLength()) tgt.setMaxLengthElement(Integer14_50.convertInteger(src.getMaxLengthElement())); 126 for (org.hl7.fhir.r5.model.IdType t : src.getCondition()) tgt.addCondition(t.getValue()); 127 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent t : src.getConstraint()) 128 tgt.addConstraint(convertElementDefinitionConstraintComponent(t)); 129 if (src.hasMustSupport()) tgt.setMustSupportElement(Boolean14_50.convertBoolean(src.getMustSupportElement())); 130 if (src.hasIsModifier()) tgt.setIsModifierElement(Boolean14_50.convertBoolean(src.getIsModifierElement())); 131 if (src.hasIsModifierReason() && !VersionConvertorConstants.EXT_MODIFIER_REASON_LEGACY.equals(src.getIsModifierReason())) 132 org.hl7.fhir.dstu2016may.utils.ToolingExtensions.setStringExtension(tgt, VersionConvertorConstants.EXT_MODIFIER_REASON_EXTENSION, src.getIsModifierReason()); 133 if (src.hasIsSummary()) tgt.setIsSummaryElement(Boolean14_50.convertBoolean(src.getIsSummaryElement())); 134 if (src.hasBinding()) tgt.setBinding(convertElementDefinitionBindingComponent(src.getBinding())); 135 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent t : src.getMapping()) 136 tgt.addMapping(convertElementDefinitionMappingComponent(t)); 137 if (src.hasMustHaveValue()) { 138 tgt.addExtension(VersionConvertorConstants.EXT_MUST_VALUE, Boolean14_50.convertBoolean(src.getMustHaveValueElement())); 139 } 140 for (org.hl7.fhir.r5.model.CanonicalType ct : src.getValueAlternatives()) { 141 tgt.addExtension(VersionConvertorConstants.EXT_VALUE_ALT, Uri14_50.convertCanonical(ct)); 142 } 143 144 145 return tgt; 146 } 147 148 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.PropertyRepresentation> src) throws FHIRException { 149 if (src == null || src.isEmpty()) return null; 150 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentationEnumFactory()); 151 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 152 if (src.getValue() == null) { 153 tgt.setValue(null); 154} else { 155 switch(src.getValue()) { 156 case XMLATTR: 157 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.XMLATTR); 158 break; 159 case XMLTEXT: 160 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.XMLTEXT); 161 break; 162 case TYPEATTR: 163 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.TYPEATTR); 164 break; 165 case CDATEXT: 166 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.CDATEXT); 167 break; 168 default: 169 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation.NULL); 170 break; 171 } 172} 173 return tgt; 174 } 175 176 static public org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.PropertyRepresentation> convertPropertyRepresentation(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.PropertyRepresentation> src) throws FHIRException { 177 if (src == null || src.isEmpty()) return null; 178 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.PropertyRepresentation> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new ElementDefinition.PropertyRepresentationEnumFactory()); 179 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 180 if (src.getValue() == null) { 181 tgt.setValue(null); 182} else { 183 switch(src.getValue()) { 184 case XMLATTR: 185 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLATTR); 186 break; 187 case XMLTEXT: 188 tgt.setValue(ElementDefinition.PropertyRepresentation.XMLTEXT); 189 break; 190 case TYPEATTR: 191 tgt.setValue(ElementDefinition.PropertyRepresentation.TYPEATTR); 192 break; 193 case CDATEXT: 194 tgt.setValue(ElementDefinition.PropertyRepresentation.CDATEXT); 195 break; 196 default: 197 tgt.setValue(ElementDefinition.PropertyRepresentation.NULL); 198 break; 199 } 200} 201 return tgt; 202 } 203 204 /* 205 * This process deals with the fact that 'exists' slicing didn't have a mechanism to flag it in 2016May. 206 * (Pattern and profile both had '@' flags in the discriminator to distinguish this, but exists did not.) 207 * 'Exists' can thus only be determined by looking at the available slices - and checking to see that there 208 * are exactly two slices, one which is mandatory and one which is prohibited. We need to do that check 209 * at the level where the slices are defined, rather than only inside the 'slicing' element where we don't 210 * have access to the slices themselves. 211 * 212 * This process checks to see if we have a 'value' discriminator (i.e. no '@') and if so, checks for all 213 * matching slices. If there are exactly two and one's required and one's prohibited, then it sets a flag 214 * so that the converter will declare a discriminator.type of 'exists'. 215 * 216 * Note that we only need complex processing on the R2B -> newer release, not on the reverse. On the reverse, 217 * we just strip the discriminator type. What slices exist is still the same. In theory, that means that the 218 * exists type is unnecessary, but it's far more efficient (and clear) to have it. 219 */ 220 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(ElementDefinition.ElementDefinitionSlicingComponent src, List<ElementDefinition> context, int pos) throws FHIRException { 221 if (src == null || src.isEmpty()) return null; 222 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent(); 223 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 224 ElementDefinition slicingElement = context.get(pos); 225 for (org.hl7.fhir.dstu2016may.model.StringType t : src.getDiscriminator()) { 226 boolean isExists = false; 227 if (!t.asStringValue().contains("@")) { 228 int slices = 0; 229 boolean existsSlicePresent = false; 230 boolean notExistsSlicePresent = false; 231 String url = null; 232 String existsPath = slicingElement.getPath() + "." + t.asStringValue(); 233 if (existsPath.contains(".extension(")) { 234 String suffix = StringUtils.substringAfter(existsPath, "(").substring(1); 235 existsPath = StringUtils.substringBefore(existsPath, "("); 236 suffix = StringUtils.substringBefore(suffix, ")"); 237 url = suffix.substring(0, suffix.length() - 1); 238 } 239 for (int i = pos + 1; i < context.size(); i++) { 240 ElementDefinition e = context.get(i); 241 if (e.getPath().equals(slicingElement.getPath())) slices++; 242 else if (!e.getPath().startsWith(slicingElement.getPath() + ".")) break; 243 else if (e.getPath().equals(existsPath)) { 244 if (url == null || (e.getType().get(0).hasProfile() && e.getType().get(0).getProfile().get(0).equals(url))) { 245 if (e.hasMin() && e.getMin() > 0 && !e.hasFixed()) existsSlicePresent = true; 246 else if (e.hasMax() && e.getMax().equals("0")) notExistsSlicePresent = true; 247 } 248 } 249 } 250 isExists = (slices == 2 && existsSlicePresent && notExistsSlicePresent) || (slices == 1 && existsSlicePresent != notExistsSlicePresent); 251 } 252 tgt.addDiscriminator(ProfileUtilities.interpretR2Discriminator(t.getValue(), isExists)); 253 } 254 if (src.hasDescription()) tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 255 if (src.hasOrdered()) tgt.setOrderedElement(Boolean14_50.convertBoolean(src.getOrderedElement())); 256 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 257 return tgt; 258 } 259 260 public static ElementDefinition.ElementDefinitionSlicingComponent convertElementDefinitionSlicingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingComponent src) throws FHIRException { 261 if (src == null || src.isEmpty()) return null; 262 ElementDefinition.ElementDefinitionSlicingComponent tgt = new ElementDefinition.ElementDefinitionSlicingComponent(); 263 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 264 for (org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionSlicingDiscriminatorComponent t : src.getDiscriminator()) 265 tgt.addDiscriminator(ProfileUtilities.buildR2Discriminator(t)); 266 if (src.hasDescription()) tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 267 if (src.hasOrdered()) tgt.setOrderedElement(Boolean14_50.convertBoolean(src.getOrderedElement())); 268 if (src.hasRules()) tgt.setRulesElement(convertSlicingRules(src.getRulesElement())); 269 return tgt; 270 } 271 272 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.SlicingRules> src) throws FHIRException { 273 if (src == null || src.isEmpty()) return null; 274 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.SlicingRulesEnumFactory()); 275 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 276 if (src.getValue() == null) { 277 tgt.setValue(null); 278} else { 279 switch(src.getValue()) { 280 case CLOSED: 281 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.CLOSED); 282 break; 283 case OPEN: 284 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.OPEN); 285 break; 286 case OPENATEND: 287 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.OPENATEND); 288 break; 289 default: 290 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.SlicingRules.NULL); 291 break; 292 } 293} 294 return tgt; 295 } 296 297 static public org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.SlicingRules> convertSlicingRules(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.SlicingRules> src) throws FHIRException { 298 if (src == null || src.isEmpty()) return null; 299 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.SlicingRules> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new ElementDefinition.SlicingRulesEnumFactory()); 300 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 301 if (src.getValue() == null) { 302 tgt.setValue(null); 303} else { 304 switch(src.getValue()) { 305 case CLOSED: 306 tgt.setValue(ElementDefinition.SlicingRules.CLOSED); 307 break; 308 case OPEN: 309 tgt.setValue(ElementDefinition.SlicingRules.OPEN); 310 break; 311 case OPENATEND: 312 tgt.setValue(ElementDefinition.SlicingRules.OPENATEND); 313 break; 314 default: 315 tgt.setValue(ElementDefinition.SlicingRules.NULL); 316 break; 317 } 318} 319 return tgt; 320 } 321 322 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 323 if (src == null || src.isEmpty()) return null; 324 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent(); 325 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 326 if (src.hasPathElement()) tgt.setPathElement(String14_50.convertString(src.getPathElement())); 327 tgt.setMin(src.getMin()); 328 if (src.hasMaxElement()) tgt.setMaxElement(String14_50.convertString(src.getMaxElement())); 329 return tgt; 330 } 331 332 public static ElementDefinition.ElementDefinitionBaseComponent convertElementDefinitionBaseComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBaseComponent src) throws FHIRException { 333 if (src == null || src.isEmpty()) return null; 334 ElementDefinition.ElementDefinitionBaseComponent tgt = new ElementDefinition.ElementDefinitionBaseComponent(); 335 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 336 if (src.hasPathElement()) tgt.setPathElement(String14_50.convertString(src.getPathElement())); 337 tgt.setMin(src.getMin()); 338 if (src.hasMaxElement()) tgt.setMaxElement(String14_50.convertString(src.getMaxElement())); 339 return tgt; 340 } 341 342 static public void convertTypeRefComponent(ElementDefinition.TypeRefComponent src, List<org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent> list) throws FHIRException { 343 if (src == null) return; 344 org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent tgt = null; 345 for (org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent t : list) 346 if (t.getCode().equals(src.getCode())) tgt = t; 347 if (tgt == null) { 348 tgt = new org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent(); 349 list.add(tgt); 350 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 351 tgt.setCodeElement(Code14_50.convertCodeToUri(src.getCodeElement())); 352 } 353 if (tgt.hasTarget()) { 354 for (org.hl7.fhir.dstu2016may.model.UriType u : src.getProfile()) { 355 if (src.getCode().equals("Reference")) tgt.addTargetProfile(u.getValue()); 356 else tgt.addProfile(u.getValue()); 357 } 358 for (org.hl7.fhir.dstu2016may.model.Extension t : src.getExtensionsByUrl(VersionConvertorConstants.EXT_PROFILE_EXTENSION)) { 359 String s = ((org.hl7.fhir.dstu2016may.model.PrimitiveType<String>) t.getValue()).getValue(); 360 tgt.addProfile(s); 361 } 362 } else { 363 for (org.hl7.fhir.dstu2016may.model.UriType u : src.getProfile()) tgt.addProfile(u.getValue()); 364 } 365 for (org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.AggregationMode> t : src.getAggregation()) { 366 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> a = convertAggregationMode(t); 367 if (!tgt.hasAggregation(a.getValue())) 368 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(t, tgt.addAggregation(a.getValue())); 369 } 370 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 371 } 372 373 public static void convertTypeRefComponent(org.hl7.fhir.r5.model.ElementDefinition.TypeRefComponent src, List<ElementDefinition.TypeRefComponent> list) throws FHIRException { 374 if (src == null) return; 375 ElementDefinition.TypeRefComponent tgt = new ElementDefinition.TypeRefComponent(); 376 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 377 tgt.setCodeElement(Code14_50.convertCode(src.getCodeElement())); 378 list.add(tgt); 379 if (src.hasTarget()) { 380 for (org.hl7.fhir.r5.model.UriType u : src.getProfile()) { 381 org.hl7.fhir.dstu2016may.model.Extension t = new org.hl7.fhir.dstu2016may.model.Extension(VersionConvertorConstants.EXT_PROFILE_EXTENSION); 382 t.setValue(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(u)); 383 tgt.addExtension(t); 384 } 385 for (org.hl7.fhir.r5.model.UriType u : src.getTargetProfile()) { 386 if (!u.equals(src.getTargetProfile().get(0))) { 387 tgt = tgt.copy(); 388 tgt.getProfile().clear(); 389 list.add(tgt); 390 } 391 tgt.addProfile(u.getValue()); 392 } 393 } else { 394 for (org.hl7.fhir.r5.model.UriType u : src.getProfile()) { 395 tgt.addProfile(u.getValue()); 396 } 397 } 398 for (org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> t : src.getAggregation()) { 399 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.AggregationMode> a = convertAggregationMode(t); 400 if (!tgt.hasAggregation(a.getValue())) 401 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(t, tgt.addAggregationElement().setValue(a.getValue())); 402 } 403 if (src.hasVersioning()) tgt.setVersioningElement(convertReferenceVersionRules(src.getVersioningElement())); 404 } 405 406 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.AggregationMode> src) throws FHIRException { 407 if (src == null || src.isEmpty()) return null; 408 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.AggregationModeEnumFactory()); 409 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 410 if (src.getValue() == null) { 411 tgt.setValue(null); 412} else { 413 switch(src.getValue()) { 414 case CONTAINED: 415 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.AggregationMode.CONTAINED); 416 break; 417 case REFERENCED: 418 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.AggregationMode.REFERENCED); 419 break; 420 case BUNDLED: 421 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.AggregationMode.BUNDLED); 422 break; 423 default: 424 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.AggregationMode.NULL); 425 break; 426 } 427} 428 return tgt; 429 } 430 431 static public org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.AggregationMode> convertAggregationMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.AggregationMode> src) throws FHIRException { 432 if (src == null || src.isEmpty()) return null; 433 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.AggregationMode> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new ElementDefinition.AggregationModeEnumFactory()); 434 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 435 if (src.getValue() == null) { 436 tgt.setValue(null); 437} else { 438 switch(src.getValue()) { 439 case CONTAINED: 440 tgt.setValue(ElementDefinition.AggregationMode.CONTAINED); 441 break; 442 case REFERENCED: 443 tgt.setValue(ElementDefinition.AggregationMode.REFERENCED); 444 break; 445 case BUNDLED: 446 tgt.setValue(ElementDefinition.AggregationMode.BUNDLED); 447 break; 448 default: 449 tgt.setValue(ElementDefinition.AggregationMode.NULL); 450 break; 451 } 452} 453 return tgt; 454 } 455 456 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 457 if (src == null || src.isEmpty()) return null; 458 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRulesEnumFactory()); 459 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 460 if (src.getValue() == null) { 461 tgt.setValue(null); 462} else { 463 switch(src.getValue()) { 464 case EITHER: 465 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules.EITHER); 466 break; 467 case INDEPENDENT: 468 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules.INDEPENDENT); 469 break; 470 case SPECIFIC: 471 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules.SPECIFIC); 472 break; 473 default: 474 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules.NULL); 475 break; 476 } 477} 478 return tgt; 479 } 480 481 static public org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ReferenceVersionRules> convertReferenceVersionRules(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ReferenceVersionRules> src) throws FHIRException { 482 if (src == null || src.isEmpty()) return null; 483 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ReferenceVersionRules> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new ElementDefinition.ReferenceVersionRulesEnumFactory()); 484 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 485 if (src.getValue() == null) { 486 tgt.setValue(null); 487} else { 488 switch(src.getValue()) { 489 case EITHER: 490 tgt.setValue(ElementDefinition.ReferenceVersionRules.EITHER); 491 break; 492 case INDEPENDENT: 493 tgt.setValue(ElementDefinition.ReferenceVersionRules.INDEPENDENT); 494 break; 495 case SPECIFIC: 496 tgt.setValue(ElementDefinition.ReferenceVersionRules.SPECIFIC); 497 break; 498 default: 499 tgt.setValue(ElementDefinition.ReferenceVersionRules.NULL); 500 break; 501 } 502} 503 return tgt; 504 } 505 506 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 507 if (src == null || src.isEmpty()) return null; 508 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent(); 509 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 510 if (src.hasKeyElement()) tgt.setKeyElement(Id14_50.convertId(src.getKeyElement())); 511 if (src.hasRequirements()) tgt.setRequirementsElement(String14_50.convertStringToMarkdown(src.getRequirementsElement())); 512 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 513 if (src.hasHumanElement()) tgt.setHumanElement(String14_50.convertString(src.getHumanElement())); 514 if (src.hasExpression()) tgt.setExpression(convertToR4Expression(src.getExpression())); 515 if (src.hasXpath()) { 516 tgt.addExtension(new org.hl7.fhir.r5.model.Extension(org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT, new org.hl7.fhir.r5.model.StringType(src.getXpath()))); 517 } 518 return tgt; 519 } 520 521 public static ElementDefinition.ElementDefinitionConstraintComponent convertElementDefinitionConstraintComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionConstraintComponent src) throws FHIRException { 522 if (src == null || src.isEmpty()) return null; 523 ElementDefinition.ElementDefinitionConstraintComponent tgt = new ElementDefinition.ElementDefinitionConstraintComponent(); 524 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT); 525 if (src.hasKeyElement()) tgt.setKeyElement(Id14_50.convertId(src.getKeyElement())); 526 if (src.hasRequirements()) tgt.setRequirementsElement(String14_50.convertString(src.getRequirementsElement())); 527 if (src.hasSeverity()) tgt.setSeverityElement(convertConstraintSeverity(src.getSeverityElement())); 528 if (src.hasHumanElement()) tgt.setHumanElement(String14_50.convertString(src.getHumanElement())); 529 if (src.hasExpression()) tgt.setExpression(convertTo2016MayExpression(src.getExpression())); 530 if (org.hl7.fhir.r5.utils.ToolingExtensions.hasExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)) { 531 tgt.setXpath(org.hl7.fhir.r5.utils.ToolingExtensions.readStringExtension(src, org.hl7.fhir.r5.utils.ToolingExtensions.EXT_XPATH_CONSTRAINT)); 532 } 533 return tgt; 534 } 535 536 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ConstraintSeverity> src) throws FHIRException { 537 if (src == null || src.isEmpty()) return null; 538 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverityEnumFactory()); 539 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 540 if (src.getValue() == null) { 541 tgt.setValue(null); 542} else { 543 switch(src.getValue()) { 544 case ERROR: 545 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity.ERROR); 546 break; 547 case WARNING: 548 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity.WARNING); 549 break; 550 default: 551 tgt.setValue(org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity.NULL); 552 break; 553 } 554} 555 return tgt; 556 } 557 558 static public org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ConstraintSeverity> convertConstraintSeverity(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ElementDefinition.ConstraintSeverity> src) throws FHIRException { 559 if (src == null || src.isEmpty()) return null; 560 org.hl7.fhir.dstu2016may.model.Enumeration<ElementDefinition.ConstraintSeverity> tgt = new org.hl7.fhir.dstu2016may.model.Enumeration<>(new ElementDefinition.ConstraintSeverityEnumFactory()); 561 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 562 if (src.getValue() == null) { 563 tgt.setValue(null); 564} else { 565 switch(src.getValue()) { 566 case ERROR: 567 tgt.setValue(ElementDefinition.ConstraintSeverity.ERROR); 568 break; 569 case WARNING: 570 tgt.setValue(ElementDefinition.ConstraintSeverity.WARNING); 571 break; 572 default: 573 tgt.setValue(ElementDefinition.ConstraintSeverity.NULL); 574 break; 575 } 576} 577 return tgt; 578 } 579 580 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 581 if (src == null || src.isEmpty()) return null; 582 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent(); 583 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 584 if (src.hasStrength()) tgt.setStrengthElement(Enumerations14_50.convertBindingStrength(src.getStrengthElement())); 585 if (src.hasDescription()) tgt.setDescriptionElement(String14_50.convertStringToMarkdown(src.getDescriptionElement())); 586 if (src.hasValueSet()) { 587 org.hl7.fhir.r5.model.DataType t = ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getValueSet()); 588 if (t instanceof org.hl7.fhir.r5.model.Reference) 589 tgt.setValueSet(((org.hl7.fhir.r5.model.Reference) t).getReference()); 590 else tgt.setValueSet(t.primitiveValue()); 591 tgt.setValueSet(VersionConvertorConstants.refToVS(tgt.getValueSet())); 592 } 593 return tgt; 594 } 595 596 public static ElementDefinition.ElementDefinitionBindingComponent convertElementDefinitionBindingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionBindingComponent src) throws FHIRException { 597 if (src == null || src.isEmpty()) return null; 598 ElementDefinition.ElementDefinitionBindingComponent tgt = new ElementDefinition.ElementDefinitionBindingComponent(); 599 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 600 if (src.hasStrength()) tgt.setStrengthElement(Enumerations14_50.convertBindingStrength(src.getStrengthElement())); 601 if (src.hasDescription()) tgt.setDescriptionElement(String14_50.convertString(src.getDescriptionElement())); 602 if (src.hasValueSet()) { 603 String vsr = VersionConvertorConstants.vsToRef(src.getValueSet()); 604 if (vsr != null) tgt.setValueSet(new org.hl7.fhir.dstu2016may.model.UriType(vsr)); 605 else tgt.setValueSet(new org.hl7.fhir.dstu2016may.model.Reference(src.getValueSet())); 606 } 607 return tgt; 608 } 609 610 public static String convertToR4Expression(String oldExpression) { 611 String pass1 = oldExpression.replaceAll("\\$context", "%context").replaceAll("\\$resource", "%resource").replaceAll("code\\+profile", "code&profile").replaceAll("path\\+'\\.'", "path&'.'").replaceAll("fullUrl\\+resource", "fullUrl&resource"); 612 String pass2 = pass1; 613 if (pass1.endsWith(".distinct()")) pass2 = pass1.substring(0, pass2.length() - 11) + ".isDistinct()"; 614 String pass3 = pass2; 615 if (pass2.endsWith(".empty() or (type.count() = 1)")) 616 pass3 = pass2.substring(0, pass2.length() - 30) + ".empty() or (type.count() <= 1)"; 617 String pass4 = pass3; 618 if (pass3.equals("duration >= 0")) pass4 = "duration.exists() implies duration >= 0"; 619 else if (pass3.equals("period >= 0")) pass4 = "period.exists() implies period >= 0"; 620 else if (pass3.equals("fullUrl.empty() xor resource")) pass4 = "fullUrl.empty() xor resource.exists()"; 621 return pass4; 622 } 623 624 public static String convertTo2016MayExpression(String newExpression) { 625 String pass1 = newExpression.replaceAll("%context", "\\$context").replaceAll("%resource", "\\$resource").replaceAll("code&profile", "code+profile").replaceAll("path&'\\.'", "path+'.'").replaceAll("fullUrl%resource", "fullUrl+resource"); 626 String pass2 = pass1; 627 if (pass1.endsWith(".isDistinct()")) pass2 = pass1.substring(0, pass1.length() - 13) + ".distinct()"; 628 String pass3 = pass2; 629 if (pass2.endsWith(".empty() or (type.count() <= 1)")) 630 pass3 = pass2.substring(0, pass2.length() - 31) + ".empty() or (type.count() = 1)"; 631 String pass4 = pass3; 632 if (pass3.equals("duration.exists() implies duration >= 0")) pass4 = "duration >= 0"; 633 else if (pass3.equals("period.exists() implies period >= 0")) pass4 = "period >= 0"; 634 else if (pass3.equals("fullUrl.empty() xor resource.exists()")) pass4 = "fullUrl.empty() xor resource"; 635 return pass4; 636 } 637 638 public static org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 639 if (src == null || src.isEmpty()) return null; 640 org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent tgt = new org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent(); 641 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 642 if (src.hasIdentityElement()) tgt.setIdentityElement(Id14_50.convertId(src.getIdentityElement())); 643 if (src.hasLanguage()) tgt.setLanguageElement(Code14_50.convertCode(src.getLanguageElement())); 644 if (src.hasMapElement()) tgt.setMapElement(String14_50.convertString(src.getMapElement())); 645 return tgt; 646 } 647 648 public static ElementDefinition.ElementDefinitionMappingComponent convertElementDefinitionMappingComponent(org.hl7.fhir.r5.model.ElementDefinition.ElementDefinitionMappingComponent src) throws FHIRException { 649 if (src == null || src.isEmpty()) return null; 650 ElementDefinition.ElementDefinitionMappingComponent tgt = new ElementDefinition.ElementDefinitionMappingComponent(); 651 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 652 if (src.hasIdentityElement()) tgt.setIdentityElement(Id14_50.convertId(src.getIdentityElement())); 653 if (src.hasLanguage()) tgt.setLanguageElement(Code14_50.convertCode(src.getLanguageElement())); 654 if (src.hasMapElement()) tgt.setMapElement(String14_50.convertString(src.getMapElement())); 655 return tgt; 656 } 657}