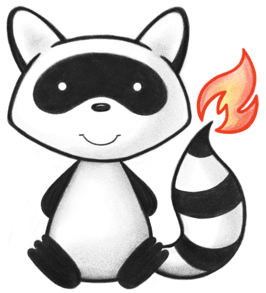
001package org.hl7.fhir.convertors.conv14_50.datatypes14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.String14_50; 005import org.hl7.fhir.dstu2016may.model.Reference; 006import org.hl7.fhir.exceptions.FHIRException; 007import org.hl7.fhir.r5.model.CanonicalType; 008 009public class Reference14_50 { 010 public static org.hl7.fhir.r5.model.Reference convertReference(org.hl7.fhir.dstu2016may.model.Reference src) throws FHIRException { 011 if (src == null || src.isEmpty()) return null; 012 org.hl7.fhir.r5.model.Reference tgt = new org.hl7.fhir.r5.model.Reference(); 013 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 014 if (src.hasReference()) tgt.setReference(src.getReference()); 015 if (src.hasDisplay()) tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 016 return tgt; 017 } 018 019 public static org.hl7.fhir.dstu2016may.model.Reference convertReference(org.hl7.fhir.r5.model.Reference src) throws FHIRException { 020 if (src == null || src.isEmpty()) return null; 021 org.hl7.fhir.dstu2016may.model.Reference tgt = new org.hl7.fhir.dstu2016may.model.Reference(); 022 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 023 if (src.hasReference()) tgt.setReference(src.getReference()); 024 if (src.hasDisplay()) tgt.setDisplayElement(String14_50.convertString(src.getDisplayElement())); 025 return tgt; 026 } 027 028 static public CanonicalType convertReferenceToCanonical(Reference src) throws FHIRException { 029 CanonicalType dst = new CanonicalType(src.getReference()); 030 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, dst); 031 return dst; 032 } 033 034 static public Reference convertCanonicalToReference(CanonicalType src) throws FHIRException { 035 Reference dst = new Reference(src.getValue()); 036 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, dst); 037 return dst; 038 } 039}