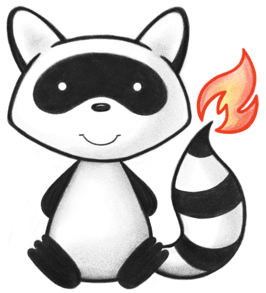
001package org.hl7.fhir.convertors.conv14_50.datatypes14_50.complextypes14_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext14_50; 004import org.hl7.fhir.convertors.conv14_50.datatypes14_50.Reference14_50; 005import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Base64Binary14_50; 006import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Code14_50; 007import org.hl7.fhir.convertors.conv14_50.datatypes14_50.primitivetypes14_50.Instant14_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Signature14_50 { 011 public static org.hl7.fhir.r5.model.Signature convertSignature(org.hl7.fhir.dstu2016may.model.Signature src) throws FHIRException { 012 if (src == null || src.isEmpty()) return null; 013 org.hl7.fhir.r5.model.Signature tgt = new org.hl7.fhir.r5.model.Signature(); 014 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 015 for (org.hl7.fhir.dstu2016may.model.Coding t : src.getType()) tgt.addType(Coding14_50.convertCoding(t)); 016 if (src.hasWhenElement()) tgt.setWhenElement(Instant14_50.convertInstant(src.getWhenElement())); 017 if (src.hasWhoUriType()) tgt.setWho(new org.hl7.fhir.r5.model.Reference(src.getWhoUriType().getValue())); 018 else tgt.setWho(Reference14_50.convertReference(src.getWhoReference())); 019 if (src.hasContentType()) tgt.setSigFormatElement(Code14_50.convertCode(src.getContentTypeElement())); 020 if (src.hasBlob()) tgt.setDataElement(Base64Binary14_50.convertBase64Binary(src.getBlobElement())); 021 return tgt; 022 } 023 024 public static org.hl7.fhir.dstu2016may.model.Signature convertSignature(org.hl7.fhir.r5.model.Signature src) throws FHIRException { 025 if (src == null || src.isEmpty()) return null; 026 org.hl7.fhir.dstu2016may.model.Signature tgt = new org.hl7.fhir.dstu2016may.model.Signature(); 027 ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().copyElement(src, tgt); 028 for (org.hl7.fhir.r5.model.Coding t : src.getType()) tgt.addType(Coding14_50.convertCoding(t)); 029 if (src.hasWhenElement()) tgt.setWhenElement(Instant14_50.convertInstant(src.getWhenElement())); 030 if (src.hasWho()) tgt.setWho(ConversionContext14_50.INSTANCE.getVersionConvertor_14_50().convertType(src.getWho())); 031 if (src.hasSigFormat()) tgt.setContentTypeElement(Code14_50.convertCode(src.getSigFormatElement())); 032 if (src.hasData()) tgt.setBlobElement(Base64Binary14_50.convertBase64Binary(src.getDataElement())); 033 return tgt; 034 } 035}